Generating PDF Files in Java: A Complete Guide with Best Practices
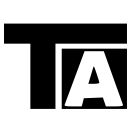
4 min read
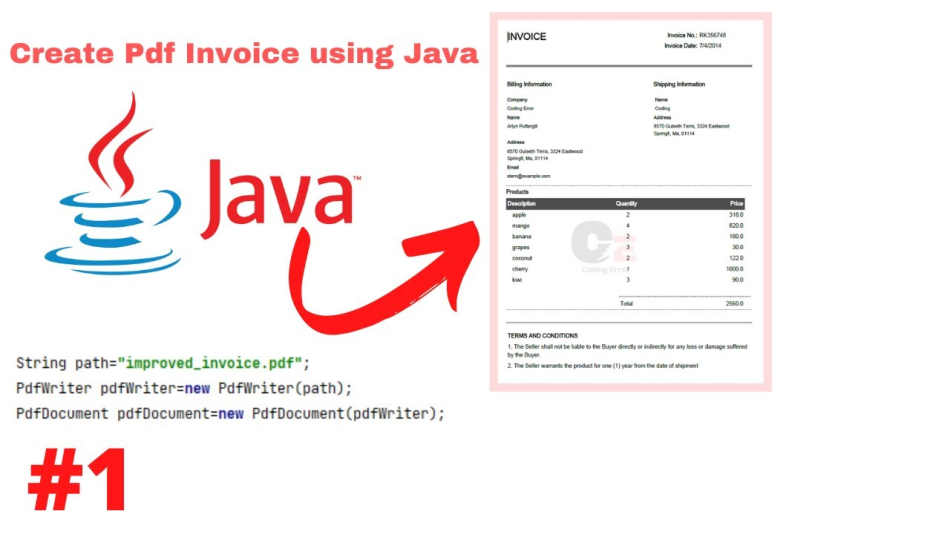
1. Understanding PDF Generation in Java
Generating a PDF in Java typically involves the use of libraries since Java doesn’t have a built-in PDF generation mechanism. The most popular libraries used for this task are iText, Apache PDFBox, and JasperReports. Each library comes with its own set of features, pros, and cons, making it important to choose the right one based on your needs.
1.1 iText Library
iText is one of the most powerful and flexible PDF generation libraries in the Java ecosystem. It allows developers to create, inspect, and modify PDF documents programmatically. Despite being commercial for extended use cases, iText is known for its reliability in creating dynamic and interactive PDFs.
Code Example Using iText:
import com.itextpdf.text.Document;
import com.itextpdf.text.DocumentException;
import com.itextpdf.text.Paragraph;
import com.itextpdf.text.pdf.PdfWriter;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
public class PdfGenerator {
public static void main(String[] args) {
Document document = new Document();
try {
PdfWriter.getInstance(document, new FileOutputStream("example.pdf"));
document.open();
document.add(new Paragraph("Hello, this is a sample PDF generated using iText."));
} catch (DocumentException | FileNotFoundException e) {
e.printStackTrace();
} finally {
document.close();
}
}
}
This code will generate a PDF file called example.pdf containing a simple "Hello" message. You can open the file using any PDF viewer to confirm the output.
1.2 Apache PDFBox
PDFBox is another popular open-source library for creating, editing, and inspecting PDF files. It’s less feature-rich compared to iText but is fully open source, making it a suitable choice for developers working on open-source projects or those with simpler PDF generation needs.
import org.apache.pdfbox.pdmodel.PDDocument;
import org.apache.pdfbox.pdmodel.PDPage;
import org.apache.pdfbox.pdmodel.PDPageContentStream;
import org.apache.pdfbox.pdmodel.font.PDType1Font;
import java.io.IOException;
public class PdfBoxGenerator {
public static void main(String[] args) {
PDDocument document = new PDDocument();
PDPage page = new PDPage();
document.addPage(page);
try {
PDPageContentStream contentStream = new PDPageContentStream(document, page);
contentStream.beginText();
contentStream.setFont(PDType1Font.HELVETICA_BOLD, 12);
contentStream.newLineAtOffset(100, 700);
contentStream.showText("Hello, this is a sample PDF generated using PDFBox.");
contentStream.endText();
contentStream.close();
document.save("example_pdfbox.pdf");
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
document.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
This code will generate a PDF file named example_pdfbox.pdf, displaying a "Hello" message in bold font at the top of the page.
2. Best Practices for Generating PDFs in Java
Once you've chosen the right library for your use case, it's important to consider best practices that ensure your PDF generation is efficient and maintains high-quality output.
2.1 Optimize Performance
PDF generation can become a resource-intensive operation, especially when dealing with large datasets or images. To optimize the performance of your PDF generation process, consider:
- Streaming Large PDFs: Instead of generating the entire PDF in memory, use streaming APIs to write chunks of data to the file system progressively.
- Use Memory Efficient Libraries: Libraries like iText offer mechanisms for managing memory better when working with large PDFs.
2.2 Secure Your PDFs
Security is an often-overlooked aspect of PDF generation. If your PDFs contain sensitive data, it’s critical to encrypt them or add passwords to prevent unauthorized access. iText provides built-in methods for setting encryption.
PdfWriter writer = PdfWriter.getInstance(document, new FileOutputStream("secure.pdf"));
writer.setEncryption("user_password".getBytes(), "owner_password".getBytes(),
PdfWriter.ALLOW_PRINTING, PdfWriter.ENCRYPTION_AES_128);
2.3 Embed Fonts Correctly
Embedding fonts is crucial for ensuring that the PDF looks consistent across different devices and readers. Without embedding fonts, some viewers may not render your PDF correctly, leading to layout issues. Both iText and PDFBox allow you to embed fonts with ease.
3. Choosing the Right Library for Your Needs
3.1 iText vs. PDFBox
The choice between iText and PDFBox often depends on the complexity of your PDF requirements:
- iText is ideal for applications needing advanced features such as interactive forms, encryption, or dynamic content generation. It’s also more mature and provides extensive documentation.
- PDFBox, being open-source and more lightweight, is perfect for projects that don’t need complex PDF functionality but still need a reliable library for generating documents.
3.2 JasperReports for Reporting
If you’re working in an enterprise setting and need to generate dynamic reports from databases or other data sources, JasperReports can be an excellent option. JasperReports integrates well with Java frameworks and can produce high-quality PDFs along with other formats such as Excel and Word.
4. Conclusion
Generating PDFs in Java may seem like a daunting task, but with the right tools and best practices, it becomes a manageable and efficient process. Whether you're using iText, PDFBox, or JasperReports, it’s important to choose the right library based on your specific use case, optimize performance, and secure your PDFs when necessary.
Feel free to comment below if you have any questions, or if you’d like further clarification on PDF generation in Java. Happy coding!
Read more at : Generating PDF Files in Java: A Complete Guide with Best Practices
0
Subscribe to my newsletter
Read articles from Tuanhdotnet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
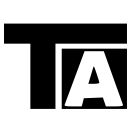
Tuanhdotnet
Tuanhdotnet
I am Tuanh.net. As of 2024, I have accumulated 8 years of experience in backend programming. I am delighted to connect and share my knowledge with everyone.