How to Simplify User Authentication with Appwrite: A Step-by-Step Guide
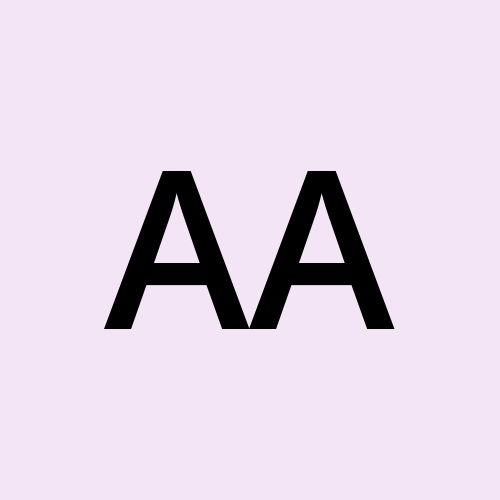
Table of contents

Introduction
Getting started with backend development can be overwhelming dealing with user authentication, setting up databases, managing storage, and writing server-side functions. That’s exactly where Appwrite fit in. It’s a powerful, all-in-one backend platform designed to make building modern applications easier, faster, and more secure.
In this guide, you’ll learn what Appwrite is, what makes it special, and why developers love using it and how to get started.
What is Appwrite?
Appwrite is an open-source backend platform that helps developers build secure, scalable apps faster without worrying about the complexity of backend setup. Whether you’re working on web or mobile apps, Appwrite provides essential backend features like:
User authentication
Databases & file storage
Cloud functions
Messaging & real-time updates
With Appwrite, you can spin up your entire backend in minutes and focus on what matters most: creating great user experiences. It supports a wide range of programming languages and frameworks, making it flexible and easy to integrate into any tech stack.
Trusted by leading companies like Apple, TikTok, IBM, and Deloitte, Appwrite empowers developers from solo hackers to enterprise teams — to move fast, stay secure, and scale confidently.
Why Appwrite?
Appwrite stands out by making backend development fast, secure, and effortless:
Fast Setup: Launch your backend in minutes with a simple command whether on your server or in the cloud.
Robust Security: Built-in user authentication, role-based access, and encryption keep your app safe and secure.
Easy File Handling: Resize, crop, and compress images, manage files with fine-grained control, and store media effortlessly.
Real-Time Sync: Keep users updated instantly with seamless real-time data syncing for features like live chats and notifications.
Developer-Friendly: Supports various programming languages, plus ready-to-use templates and a function marketplace to extend your app easily.
Scalable and Affordable: Start with a generous free tier and scale up as needed without paying for unnecessary features.
Thriving Community: Tap into an open-source, vibrant community that’s always ready to help you build and grow.
With Appwrite, you can focus on what matters most—building amazing apps without the backend headaches.
Getting Started with Appwrite
Now that you know why Appwrite is a great choice, let’s dive into how to get started quickly and effortlessly.
Whether you’re developing for web, iOS, Android, or Native, Appwrite offers SDKs for all major platforms. In this guide, you’ll learn how to get started with Appwrite on the Web using React.
Ready to dive in? Follow the steps below to get your app up and running with Appwrite.
Prerequisites
Before you begin, make sure you have the following:
Node.js and npm installed on your machine.
Basic knowledge of React.js.
An Appwrite account set up. If you haven't already, you can register here.
Step 1: Create a Project
Before you can start building with Appwrite, you need to create a new project in the Appwrite console. Follow these simple steps to get started:
Log in to your Appwrite account: If you haven’t already, visit Appwrite’s console and log in with your credentials.
Create a new project:
In the Appwrite dashboard, click on the "Create Project" button.
Provide a name for your project (e.g., "My First Appwrite App").
Select a region for deployment and click Create to finalize the setup.
Add a Platform:
Select the platform you’re developing for (e.g., Web, iOS, Android).
Provide a name for your hostname registration (e.g., "My Web App") and ensure the hostname is set to localhost.
Skip optional steps.
Click Continue to dashboard after completing setup.
Access the Project Dashboard: After creating the project, you’ll be redirected to your project’s dashboard where you can start adding services like authentication, databases, storage, and more.
With your project created, you’re now ready to connect Appwrite to your application and start using the features it offers!
Step 2: Bootstrap React Project with Vite
Run the following command in your terminal to quickly create a React app with Vite:
npm create vite@latest my-app -- --template react && cd my-app && npm install
Step 3: Install Appwrite JavaScript SDK
Now, install the Appwrite SDK by running:
npm install appwrite@14.0.1
Step 4: Set Up Appwrite
- Create a new file
src/lib/appwrite.js
and add the following code to it. Replace<PROJECT_ID>
with your project ID:
import { Client, Account} from 'appwrite';
export const client = new Client();
client
.setEndpoint('https://cloud.appwrite.io/v1')
.setProject('<PROJECT_ID>'); // Replace with your project ID
export const account = new Account(client);
export { ID } from 'appwrite';
Click on the ID in front of the project name to copy your project ID
Step 5: Create a Login Page
Add the following code to src/App.jsx
to create a simple login and registration page:
import React, { useEffect, useState } from 'react';
import { account, ID } from './lib/appwrite';
const App = () => {
const [loggedInUser, setLoggedInUser] = useState(null);
const [email, setEmail] = useState('');
const [password, setPassword] = useState('');
const [name, setName] = useState('')
const [loginError, setLoginError] = useState('');
const [registerError, setRegisterError] = useState('');
const checkLogin = async () => {
try {
const user = await account.get();
setLoggedInUser(user);
} catch (error) {
setLoggedInUser(null); // Reset if user is not logged in
}
};
useEffect(() => {
checkLogin();
}, []);
const handleLogin = async (e) => {
e.preventDefault();
setLoginError('');
try {
await account.createEmailPasswordSession(email, password);
checkLogin(); // Refresh user info after login
} catch (error) {
console.error('Login failed:', error);
setLoginError(error.message || 'Login failed. Check your credentials.');
}
};
const handleRegister = async (e) => {
e.preventDefault();
setRegisterError('');
try {
await account.create(ID.unique(), email, password, name);
await handleLogin(e);
} catch (error) {
console.error('Registration failed:', error);
setRegisterError(error.message || 'Registration failed. Try again.');
}
};
const handleLogout = async () => {
try {
await account.deleteSession('current');
setLoggedInUser(null);
} catch (error) {
console.error('Logout failed:', error);
}
};
return (
<div>
<div>
{loggedInUser ? (
<div>
<h2>Welcome, {loggedInUser.name}</h2>
<button onClick={handleLogout}>
Logout
</button>
</div>
) : (
<form>
<h2>Appwrite Auth</h2>
<input
type="email"
placeholder="Email"
value={email}
onChange={(e) => setEmail(e.target.value)}
/>
<input
type="password"
placeholder="Password"
value={password}
onChange={(e) => setPassword(e.target.value)}
/>
<input
type="text"
placeholder="Name"
value={name}
onChange={(e) => setName(e.target.value)}
/>
<div>
<button onClick={handleLogin}>
Login
</button>
<button onClick={handleRegister}>
Register
</button>
</div>
{/* Show specific error messages */}
{loginError && <p>{loginError}</p>}
{registerError && <p>{registerError}</p>}
</form>
)}
</div>
</div>
);
};
export default App;
In your main component (App.jsx
), you're using React hooks for handling authentication and updating UI states. You’re calling the account
methods to handle login and registration.
You’ve already set up the necessary methods: handleLogin
, handleRegister
, checkLogin
, and handleLogout
. Here's a breakdown:
checkLogin()
: Checks if the user is logged in by callingaccount.get()
. If logged in, it sets the logged-in user state (loggedInUser
), else it setsnull
.handleLogin()
: This method handles the login process. It sends theemail
andpassword
to Appwrite to create a session. If successful, the user is logged in.handleRegister()
: This method registers a new user. It sendsemail
,password
, andname
to Appwrite. After registration, the user is automatically logged in.handleLogout()
: This method handles logging out by deleting the current session usingaccount.deleteSession('current')
.
Step 6: Styling
Add the following styles to src/App.css
to beautify the page
body{
display: flex;
justify-content: center;
align-items: center;
flex-direction: column;
width: 100%;
height: 100vh;
margin: 0 auto;
}
form{
display: flex;
justify-content: center;
align-items: center;
flex-direction: column;
}
form input{
margin: 0.5em;
padding: 0.5em;
border-radius: 8px;
border: 1px solid transparent;
font-size: 1em;
width: 300px;
font-weight: 500;
font-family: inherit;
background-color: #1a1a1a;
}
Step 7: Run Your Project
Run your project with:
npm run dev
Then, open localhost on port 5173 in your browser to see your app in action!
🎥 Watch: Appwrite Authentication in Minutes (YouTube Walkthrough)
Prefer watching to reading? Here's a step-by-step video where I walk through setting up Appwrite authentication with React.
Conclusion
Appwrite makes it super easy to set up a secure backend in minutes from authentication to databases and more, all without breaking a sweat. Now that you've got the basics down...
But what if you could supercharge it with AI?
Imagine your AI assistant interacting with your Appwrite database in real time. You're going to love what’s coming next.
📘 Check out my article on Appwrite MCP
📚 Explore the official Appwrite Documentation
Build faster. Stay secure. Go further with Appwrite.
Subscribe to my newsletter
Read articles from Abdulrasheed Abdulsalam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
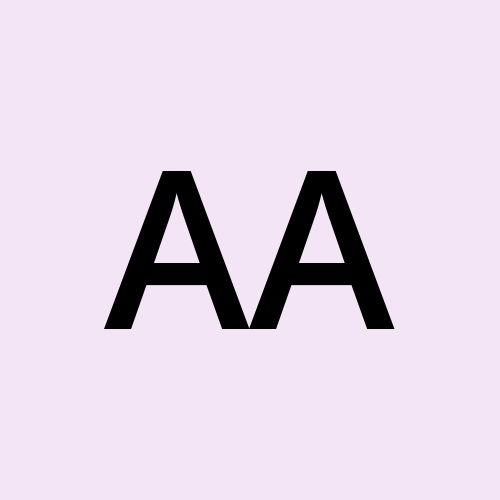