Override MUI component style and related bugs
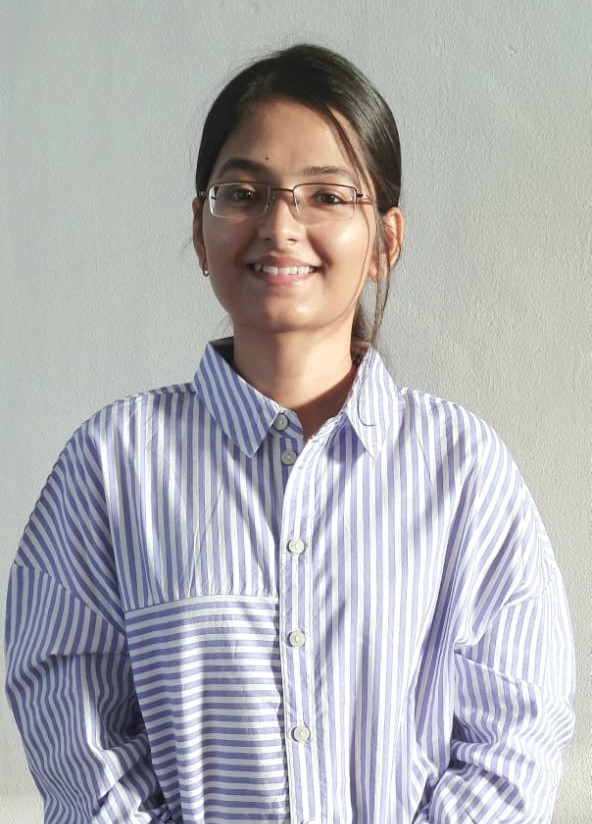

Great topic — overriding Material UI (MUI) component styles is essential in real-world projects, especially large-scale ones. Let’s break it down:
🔹 How to Override MUI Component Styles
There are multiple ways to customize MUI components:
✅ 1. Using sx
Prop (Preferred for quick customizations)
<Button sx={{ backgroundColor: 'red', '&:hover': { backgroundColor: 'darkred' } }}>
Click Me
</Button>
🔸 Simple and scoped to the element.
🔸 Good for on-the-fly style adjustments.
✅ 2. Using styled
API (For reusable custom styles)
import { styled } from '@mui/material/styles';
const MyButton = styled(Button)(({ theme }) => ({
backgroundColor: theme.palette.primary.main,
color: '#fff',
'&:hover': {
backgroundColor: theme.palette.primary.dark,
},
}));
🔸 Great for consistency across components.
🔸 Encouraged for design systems or shared component libraries.
✅ 3. Using Theme Customization (Theme Overrides / Variants)
const theme = createTheme({
components: {
MuiButton: {
styleOverrides: {
root: {
textTransform: 'none',
fontSize: '1rem',
},
},
},
},
});
🔸 Applied globally.
🔸 Useful for app-wide design changes.
✅ 4. CSS Classes or Emotion ClassNames
<Button className="my-custom-btn" />
Use Emotion’s css
if you're sticking with MUI's default styling engine.
⚠️ Issues in Large Projects
When scaling up, here are common challenges:
🔸 1. Style Conflicts & Specificity Issues
Overriding deeply nested styles (like in
Dialog
,Select
, orTable
) may not apply due to CSS specificity.Need to use
!important
sometimes (but it’s better to avoid).
Fix: Use the right class or target specific MUI slots (e.g. .MuiInputBase-root
)
🔸 2. Inconsistent Styling Approaches
Mixing
sx
,styled
, andmakeStyles
can lead to confusion.Harder to maintain and refactor.
Fix: Define and document a consistent styling strategy (e.g., prefer styled
for shared components, sx
for inline tweaks).
🔸 3. Hard to Override Internal MUI Elements
- Some deeply nested MUI components don’t expose easy props for styling.
Fix: Inspect with browser dev tools and target MUI class selectors or use components.MuiX.styleOverrides
.
🔸 4. Dark Mode / Theme Switching Complexity
- Manual overrides may not respect theme mode (light/dark).
Fix: Use theme.palette.mode
or theme.palette
for dynamic theming in styled
.
🔸 5. Build Size & Performance
- Unoptimized custom themes or lots of custom components can bloat your bundle.
Fix: Use tree-shaking, avoid importing entire MUI themes, and keep styling modular.
🚀 15 Real-World Challenges When Using MUI Tables in Scaled React Projects (And How to Fix Them)
Sure! Here's a Markdown-ready blog post version titled and formatted with headings, bullet points, and code snippets — perfect to paste into a blog platform like Hashnode, Medium (with Markdown support), or your dev portfolio.
1. Performance Bottlenecks in Large Tables
Issue: Table re-renders when unrelated parts of the state change.
Fix:
Use
React.memo
or virtualization libraries likereact-window
ormui-x-data-grid-pro
.Use
useCallback
for cell renderers and row actions.Paginate and debounce filters/search.
🎨 2. Inconsistent Styling Across Tables
Issue: Multiple styling approaches (sx
, styled
, theme overrides) lead to conflicts and hard-to-maintain code.
Fix:
Standardize usage (e.g., use
styled
for shared components,sx
for inline tweaks).Use theme overrides for consistency.
const StyledTableCell = styled(TableCell)(({ theme }) => ({
backgroundColor: theme.palette.grey[100],
}));
🧱 3. Messy Column Definitions
Issue: Hard coded, scattered column logic.
Fix:
- Use a centralised column schema:
export const userColumns = [
{ field: 'name', headerName: 'Name', width: 200 },
{
field: 'status',
headerName: 'Status',
renderCell: (params) => <Chip label={params.value} />
}
];
🌀 4. Complex Conditional Rendering Inside Cells
Fix:
- Extract logic into small, reusable components:
const StatusChip = ({ status }) => (
<Chip label={status} color={status === 'active' ? 'success' : 'default'} />
);
🔧 5. Redundant Table Logic (Sorting, Filtering, etc.)
Issue: Same logic written repeatedly across tables.
Fix:
Build a configurable
SmartTable
wrapper that handles:Sorting
Filtering
Pagination
Toolbar actions
Export/CSV download
✏️ 6. Painful Inline Editing in Tables
Issue: Editable rows cause complex state handling and bugs.
Fix:
Use local state per row.
Batch updates before sending to the server.
Validate before updating:
onChange={(e) => setRowData(prev => ({ ...prev, name: e.target.value }))}
🌙 7. Theme Mismatches (Especially in Dark Mode)
Fix:
- Always use values from the
theme
object:
backgroundColor: theme.palette.mode === 'dark' ? '#333' : '#fff'
📱 8. Non-Responsive Table Layouts
Fix:
- Use MUI’s Grid +
useMediaQuery
to show/hide columns or adjust UI on smaller screens.
🔍 9. Unscalable Table Actions
Issue: Each row defines its own action logic.
Fix:
- Use shared action renderers:
const TableActions = ({ row }) => (
<>
<IconButton onClick={() => edit(row)}><Edit /></IconButton>
<IconButton onClick={() => remove(row)}><Delete /></IconButton>
</>
);
💥 10. Lack of Error Boundaries Around Dynamic Tables
Fix:
- Wrap table sections with error boundaries:
<ErrorBoundary fallback={<ErrorTable />}>
<DataGrid {...props} />
</ErrorBoundary>
📡 11. Inefficient API Fetching Strategy
Fix:
Debounce filters and search inputs.
Use server-side filtering/sorting/pagination.
Use SWR/React Query for caching and optimistic updates.
🗃️ 12. Global Redux State Bloat
Issue: Filters, sort, pagination stored globally.
Fix:
Keep local table state using
useReducer
oruseState
.Use Redux only for truly global concerns.
🛑 13. Missing Accessibility (a11y)
Fix:
Use semantic elements (
<thead>
,<tbody>
,<tr>
,<th>
, etc.).Provide ARIA labels for modals, buttons, and inputs.
🧪 14. Difficult to Test Dynamic Tables
Fix:
Add
data-testid
s or usegetByRole
with meaningful labels.Extract logic-heavy actions for isolated unit testing.
🧵 15. Over complicating with Too Many Abstractions
Fix:
Keep logic modular and understandable.
Start simple and refactor only when patterns repeat.
✅ Pro Tip: Build a Reusable Smart Table Component
Instead of repeating logic, build your own abstraction over MUI’s Table or Data Grid:
<SmartTable
data={rows}
columns={userColumns}
onRowClick={handleClick}
enableExport
enableBulkActions
rowKey="id"
/>
🚀 Wrapping Up
MUI Tables are powerful — but they can get messy FAST in scaled applications. The key to success is consistent patterns, reusability, and lean, optimized components.
Building scalable tables with MUI in React is more than just slapping columns together — it's about thoughtful architecture, performance tuning, and a consistent developer experience. The good news? With a few best practices and reusable patterns, you can tame even the most complex UIs.
Thanks for reading! If you found this helpful, consider sharing it with your team or bookmarking it for your next big refactor. 🔁
Subscribe to my newsletter
Read articles from Sonal Diwan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
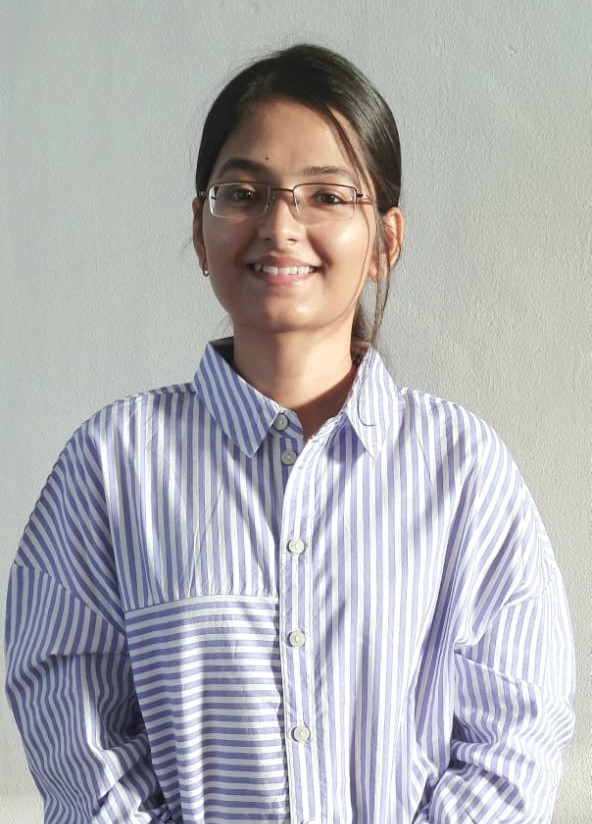
Sonal Diwan
Sonal Diwan
Data-Driven Fresher | Leveraging full stack technologies | Exploring tech-trends