Recap of OOPs in Java with Real-World Examples and a Simple Java Program
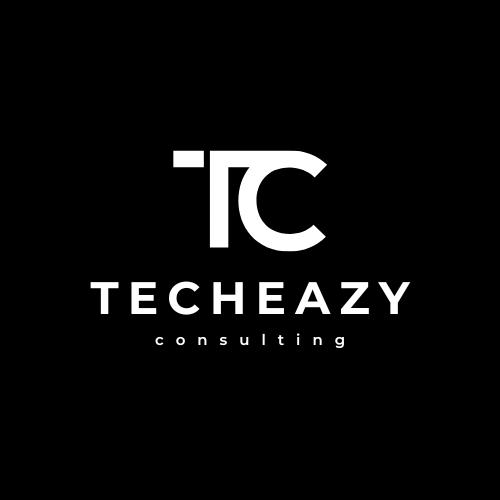
Table of contents
- πΉ What is a Class?
- πΉ Implementing a Person Class in Java
- πΉ OOPs Concepts in Action
- πΉ Sample Java Program (Entry Point)
- π What Happens When You Hit a URL?
- π Simple Java Socket Server Example
- π Web Server vs App Server
- π± Why Spring Boot?
- β Why Java is a High-Level Language (Compared to C/C++)
- π§° Java Libraries in Action
- π Assignment
- π Want to Learn More?
Object-Oriented Programming (OOPs) is the backbone of Java. If you're just starting out with Java or revisiting it after a while, this post will give you a strong foundation in OOPs and how it connects to building real-world applications like Dropbox.
πΉ What is a Class?
A class is a blueprint for creating objects. It defines properties (variables) and behavior (methods). Think of it like a template for a "Person" or a "Document" in a system.
πΉ Implementing a Person
Class in Java
public class Person {
private String name;
private int age;
private String category;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
if (age >= 0 && age <= 120) {
this.age = age;
assignCategory();
} else {
System.out.println("Invalid age!");
}
}
private void assignCategory() {
if (age < 13) {
category = "Child";
} else if (age < 20) {
category = "Teen";
} else {
category = "Adult";
}
}
public String getCategory() {
return category;
}
}
πΉ OOPs Concepts in Action
β Encapsulation
By using private
variables and getters/setters
, we control access and validate input. For example, age must be between 0 and 120.
β Abstraction
The internal logic that assigns a category
is hidden. Consumers of the class just see the result via getCategory()
, without needing to know how itβs calculated.
πΉ Sample Java Program (Entry Point)
public class Main {
public static void main(String[] args) {
Person p = new Person();
p.setName("Alice");
p.setAge(25);
System.out.println(p.getName() + " is an " + p.getCategory());
}
}
π° Entry Point
Java execution starts from the main()
method. When you run this program, you'll see:
Alice is an Adult
π What Happens When You Hit a URL?
When you open a browser and enter a URL:
DNS translates the domain to an IP address.
A request is sent to the server at that IP.
The web server (like Apache or NGINX) handles static files (HTML, CSS).
The application server (like Tomcat or Spring Boot) processes dynamic requests (Java code, database interaction).
A response is returned to your browser.
π Simple Java Socket Server Example
To understand how content can be served, letβs look at a barebones socket server in Java:
import java.io.*;
import java.net.*;
public class SimpleServer {
public static void main(String[] args) throws IOException {
ServerSocket server = new ServerSocket(8080);
System.out.println("Listening on port 8080...");
Socket client = server.accept();
BufferedReader in = new BufferedReader(new InputStreamReader(client.getInputStream()));
PrintWriter out = new PrintWriter(client.getOutputStream(), true);
String request = in.readLine();
System.out.println("Request: " + request);
out.println("HTTP/1.1 200 OK\r\nContent-Type: text/plain\r\n\r\nHello from Java Server!");
in.close();
out.close();
client.close();
server.close();
}
}
π Web Server vs App Server
Feature | Web Server | Application Server |
Serves Static Content | Yes (HTML/CSS/JS) | No |
Serves Dynamic Content | No | Yes (Java, Spring) |
Examples | Apache, NGINX | Tomcat, JBoss, Spring |
π± Why Spring Boot?
Manually writing socket code is funβbut painful! Spring Boot makes serving content, handling requests, managing dependencies, and building REST APIs super simple and productive.
β Why Java is a High-Level Language (Compared to C/C++)
Automatic memory management (no manual
malloc
/free
)Huge standard library (Collections, Networking, File I/O, Security)
Platform-independent via JVM
Built-in multithreading and exception handling
π§° Java Libraries in Action
java.util.ArrayList
β for dynamic listsjava.net.Socket
β for network communicationjava.nio.file
β for file operationsjavax.servlet
/org.springframework.web
β for web apps
π Assignment
β Basic
Design a Document Management System (like Dropbox):
Identify a class:
Document
Properties:
String name
,File file
,String owner
,Date uploadedAt
Modify
main()
to accept command-line arguments: document name & file path
java DocumentUploader "Resume.pdf" "/Users/you/Downloads/Resume.pdf"
β Advanced
Use GPT or Google to find how to read a file and store it in a given location using Java. Weβll discuss error handling and clean code in the next session.
π‘ Teaser: We'll explore file uploads, exception handling, and REST APIs in our upcoming session.
π Want to Learn More?
Join our AWS Internship Program to go from Java basics to cloud deployment.
π Register Now
Subscribe to my newsletter
Read articles from TechEazy Consulting directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
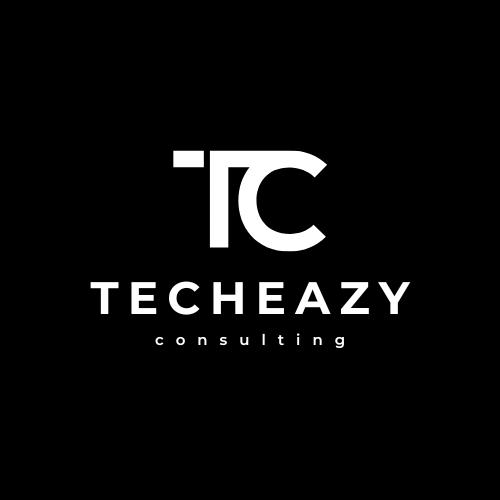