How to Check if a String Contains a Substring in Python
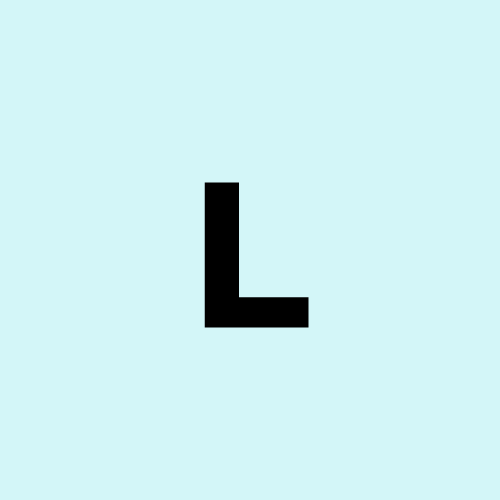

Working with strings is a fundamental part of Python programming. Whether you’re cleaning data, building a chatbot, validating user input, or filtering search results — checking if a string contains a specific substring is one of the most common operations.
In this blog, we'll explore multiple methods to perform the python string contains check effectively, using both simple and advanced techniques.
Why is Substring Matching Important?
String matching helps you determine if a certain sequence of characters (a substring) exists within a larger string. This operation becomes especially powerful when working with user-generated content, text processing, file parsing, or search functionality.
If you’re planning a complex project involving text-based operations and don’t have in-house expertise, it’s always smart to hire a dedicated Python developer who is skilled to handle such logic seamlessly and efficiently.
Using the
in
Operator
The simplest and most Pythonic way to check if a string contains a substring is by using the in
keyword.
```python
6text = "Python is awesome"
7print("awesome" in text) # Output: True
This method returns a boolean — True
if the substring is present and False
otherwise. It’s fast, readable, and preferred for most use cases.
2. Using the find()
Method
The find()
method searches for the substring and returns its index. If the substring is not found, it returns -1
text = "Learning Python is fun"
index = text.find("Python")
print(index) # Output: 9
You can use this index to perform more complex operations, such as slicing or validation.
text = "Learning Python is fun"
if text.find("Python") != -1:
print("Substring found!")
This method is helpful when you not only want to know if a substring exists but where it exists.
3. Using the index()
Method
Similar to find()
, the index()
method returns the position of the substring. However, unlike find()
, it raises a ValueError
if the substring is not found.
text = "Python makes life easier"
try:
pos = text.index("life")
print(f"Substring found at position {pos}")
except ValueError:
print("Substring not found.")
This approach is useful when failure should raise an exception rather than silently returning -1
.
4. Using Regular Expressions
For advanced pattern matching, Python’s re
module is the go-to solution. It allows you to search for substrings using custom rules or wildcards.
import re
text = "Data science and Python go hand in hand"
pattern = "Python"
if re.search(pattern, text):
print("Substring found with regex!")
Regular expressions are extremely powerful when the substring isn’t exact — like searching for variations of a word, patterns, or case-insensitive matches.
5. Case-Insensitive Substring Matching
By default, Python string methods are case-sensitive. To perform a case-insensitive check, you can convert both the string and the substring to lowercase (or uppercase) before comparing.
text = "Python Developers are in demand"
substring = "developers"
if substring.lower() in text.lower():
print("Case-insensitive match found!")
This is a great method when user input or data is not standardized.
6. Checking Multiple Substrings
You can check for multiple substrings using a loop or list comprehension:
text = "Python is powerful and flexible"
substrings = ["powerful", "easy", "fast"]
matches = [word for word in substrings if word in text]
print("Found substrings:", matches)
This is useful in applications like keyword detection, spam filtering, or SEO tools.
Best Practices
Use the
in
operator for quick, readable checks.Use
find()
orindex()
when you need the position of the substring.Use regex (
re.search
) when you need pattern-based matching.Normalize casing for user input before checking.
Avoid redundant checks — optimize your string operations in loops or large datasets.
Summary
Understanding how the python string contains logic works is essential for developers working on modern applications — from natural language processing to search algorithms. With several built-in methods and libraries at your disposal, Python makes it incredibly easy to implement efficient substring checks.
To recap, here are the key techniques we covered:
Using the
in
operatorUsing
find()
andindex()
Leveraging the
re
module for regexCase-insensitive and multi-substring matching
No matter which method you choose, knowing how and when to apply it will make your Python code more efficient and error-free.
If you're building a data-driven or text-heavy application, having expertise in Python’s string manipulation can save hours of debugging. Whether it's a chatbot, a search feature, or data analysis — knowing how to efficiently check if a python string contains something is a must.
Subscribe to my newsletter
Read articles from Lucy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
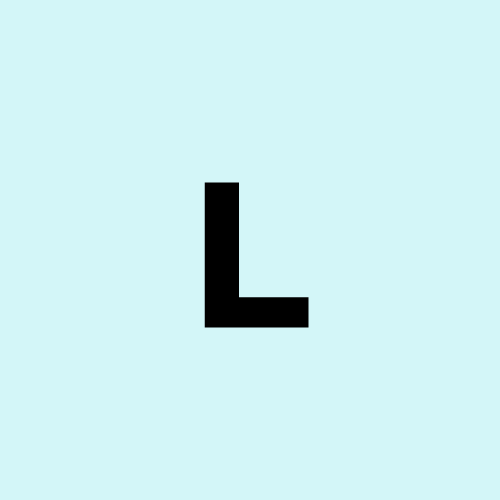