Protecting User Data: Building a Secure API with Node.js
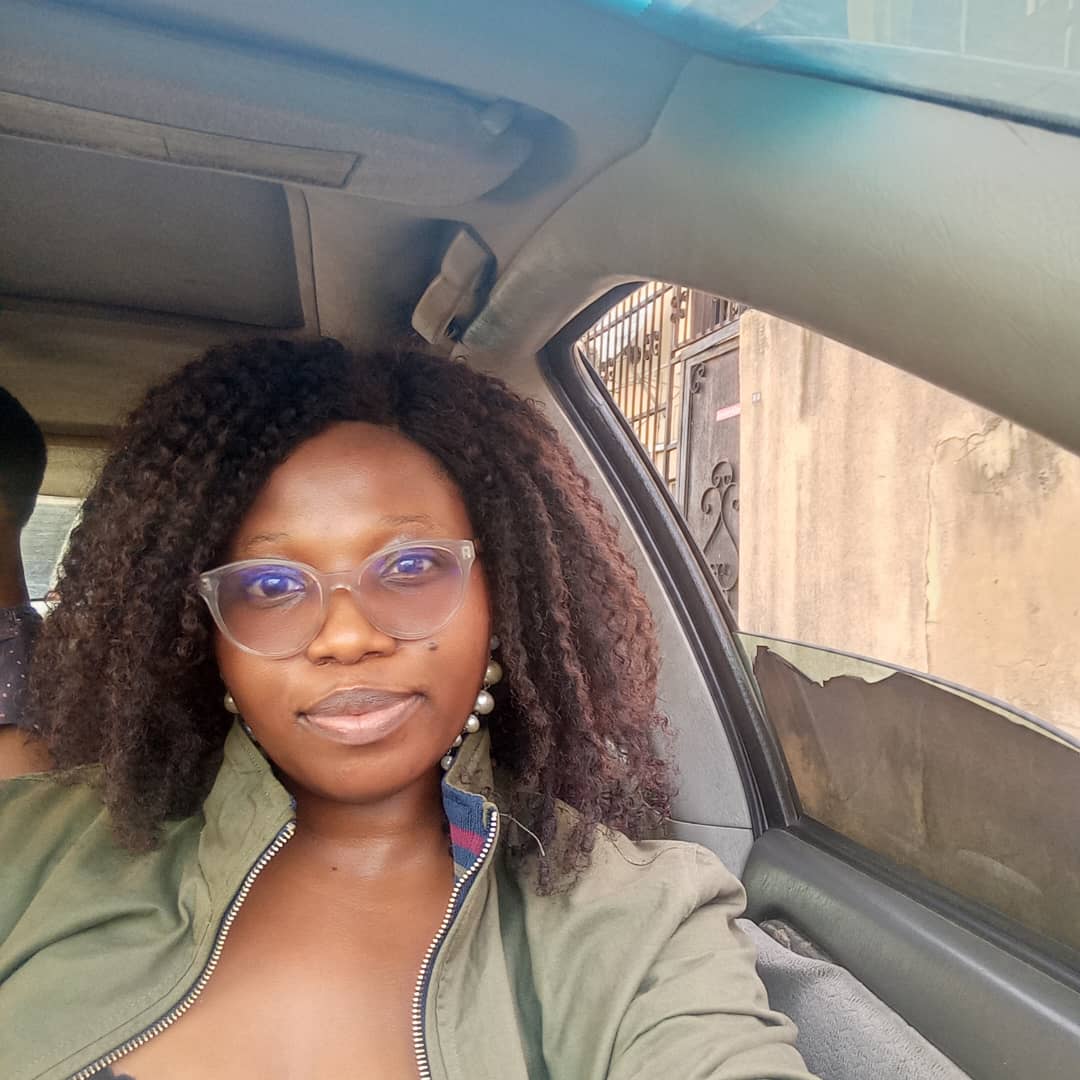

In our interconnected world, safeguarding user data and ensuring that APIs run smoothly are more critical than ever. With frequent headlines about data breaches, it’s evident that tech giants like Google and Amazon invest heavily in robust authentication systems to protect their users while maintaining seamless access to their services. As developers, we have a responsibility to prioritize user authentication and API security to build trust and provide an exceptional user experience.
Why API Security Matters
When developing applications, consider these key aspects of API security:
Safeguard User Data: With the surge in data breaches, protecting user information is essential.
Mitigate Abuse: Implementing rate limiting helps prevent denial-of-service attacks, ensuring your API remains reliable even under heavy traffic.
Build User Trust: A secure API fosters confidence among users, making them feel safe while interacting with your application.
My Project: Developing a Secured API with Node.js
In this article, I'll take you through my recent project where I built a secure API using Node.js. This project focuses on user authentication and rate limiting, both vital for protecting your application from unauthorized access and misuse.
Steps to Create the API
1. Project Structure
I organized my project in the following way:
secured-api/
├── index.js # Main server file
├── package.json # Project metadata
└── .gitignore # Files to ignore
2. Initializing the Project
I started by initializing the project and installing the necessary packages:
mkdir secured-api && cd secured-api
npm init -y
npm install express express-rate-limit body-parser
3. Setting Up the Server
In index.js
, I configured the server to include authentication and rate limiting:
const express = require('express');
const rateLimit = require('express-rate-limit');
const bodyParser = require('body-parser');
const app = express();
// Rate Limiting Middleware
const limiter = rateLimit({
windowMs: 15 * 60 * 1000, // 15 minutes
max: 100, // Limit each IP to 100 requests per window
message: 'Too many requests from this IP, please try again later.'
});
app.use(limiter);
app.use(bodyParser.json());
// Login Endpoint
app.post('/login', (req, res) => {
const { username, password } = req.body;
// Simple authentication logic
if (username === 'admin' && password === 'password') {
const token = 'your_generated_token_here'; // Placeholder for token generation
return res.status(200).json({ token });
}
return res.status(401).json({ message: 'Invalid credentials' });
});
// Home Endpoint
app.get('/', (req, res) => {
res.send('Welcome to the secured API!');
});
const PORT = 3000;
app.listen(PORT, () => console.log(`Server running on port ${PORT}`));
4. Testing the API
After starting the server with node index.js
, I accessed http://localhost:3000
and saw:
Welcome to the secured API!
Using Postman, I sent a POST request to the login endpoint with this JSON body:
{
"username": "admin",
"password": "password"
}
Upon successfully logging in, I received a token, mimicking a real-world authentication scenario.
Conclusion
Creating a secure API with proper authentication and rate limiting is vital for any application. This project reinforced my understanding of how important these elements are in protecting user data and ensuring a responsive service.
Moving forward, I plan to enhance this project by incorporating JWT (JSON Web Tokens) for more robust authentication and exploring user roles.
Final Thoughts
Integrating security best practices into your API development process not only protects user data but also improves the overall user experience. If you’d like to learn more about API security or have any questions, don’t hesitate to leave a comment!
Subscribe to my newsletter
Read articles from Abigeal Afolabi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
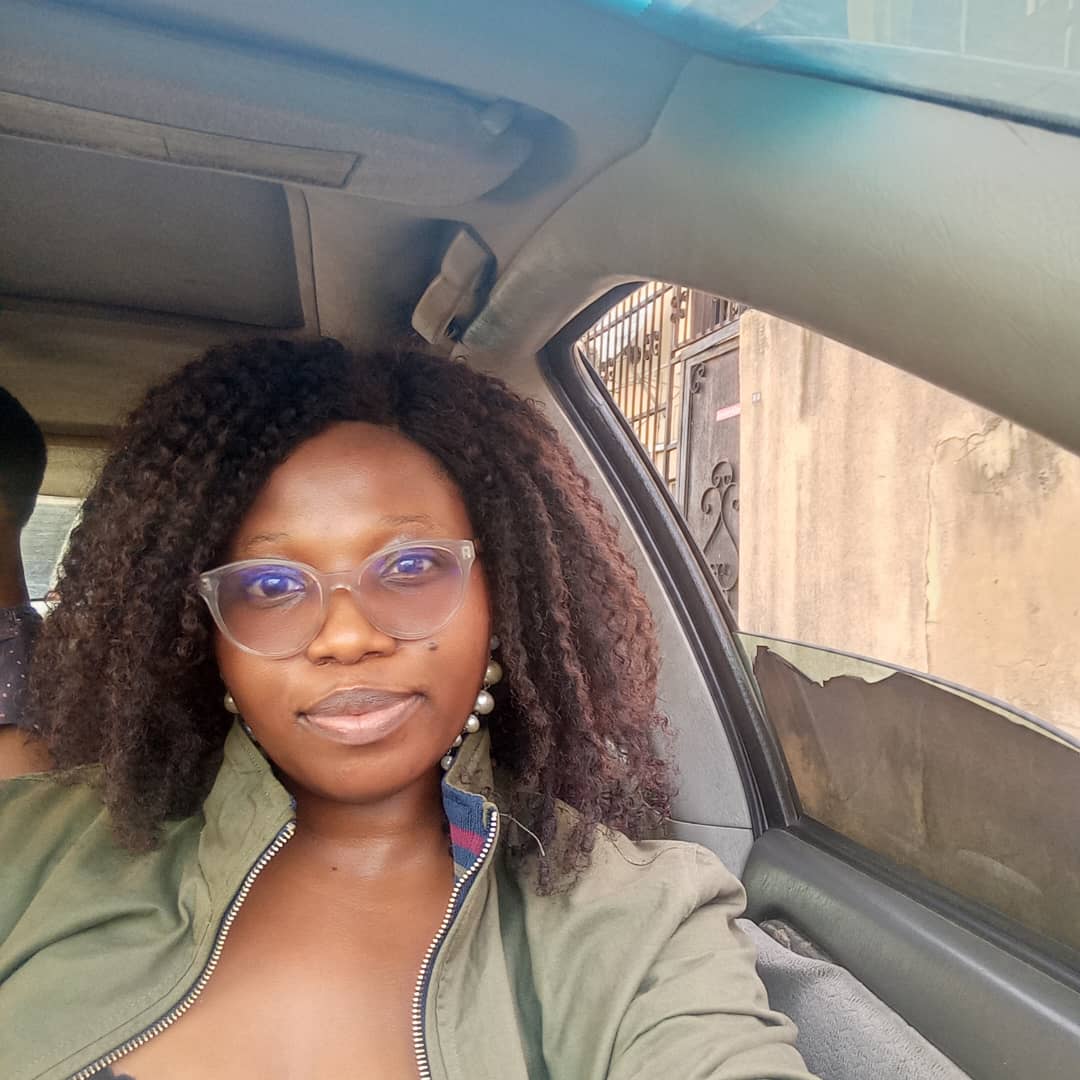
Abigeal Afolabi
Abigeal Afolabi
🚀 Software Engineer by day, SRE magician by night! ✨ Tech enthusiast with an insatiable curiosity for data. 📝 Harvard CS50 Undergrad igniting my passion for code. Currently delving into the MERN stack – because who doesn't love crafting seamless experiences from front to back? Join me on this exhilarating journey of embracing technology, penning insightful tech chronicles, and unraveling the mysteries of data! 🔍🔧 Let's build, let's write, let's explore – all aboard the tech express! 🚂🌟 #CodeAndCuriosity