Top 15 Java & OOPs Interview Questions (With Answers & Examples) for Beginners
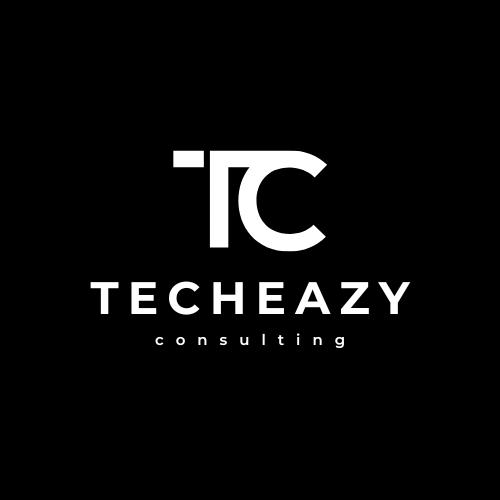
Table of contents
- 1️⃣ What is a Class in Java?
- 2️⃣ What is an Object in Java?
- 3️⃣ What is Encapsulation?
- 4️⃣ What is Abstraction?
- 5️⃣ Difference between Encapsulation and Abstraction?
- 6️⃣ How do Access Modifiers work in Java?
- 7️⃣ How is Encapsulation Implemented in Java?
- 8️⃣ How is Abstraction Implemented in Java?
- 9️⃣ What are Libraries in Java?
- 🔟 Why Can Java Run Across Platforms?
- 1️⃣1️⃣ Procedural vs OOPs – What’s Better?
- 1️⃣2️⃣ One Key Reason for Java's Popularity?
- 1️⃣3️⃣ How Does Java Handle Memory?
- 1️⃣4️⃣ What is Polymorphism?
- 1️⃣5️⃣ What is Inheritance?
- 🚀 Wrap-Up & What’s Next?
Whether you're preparing for your first developer role or brushing up for a technical round, these 15 frequently asked Java and OOPs questions will sharpen your basics and boost your confidence.
🎯 Bonus: For in-depth prep, check out our internship program here:
👉 Java + AWS Internship
1️⃣ What is a Class in Java?
A class is a blueprint for creating objects. It defines properties (variables) and behaviors (methods).
class Car {
String brand;
void drive() {
System.out.println("Car is driving");
}
}
2️⃣ What is an Object in Java?
An object is an instance of a class. It holds data and can perform actions.
Car myCar = new Car(); // Object created
3️⃣ What is Encapsulation?
Encapsulation means binding data with methods and restricting direct access to variables.
✅ Achieved using:
private
fieldspublic
getters/setters
class Person {
private int age;
public void setAge(int age) {
if(age > 0) this.age = age;
}
public int getAge() { return age; }
}
4️⃣ What is Abstraction?
Abstraction means hiding internal logic and exposing only what’s necessary.
✅ Achieved using:
abstract
classesinterfaces
Encapsulated logic with public methods
Example: You see getBalance()
but not how it fetches from the DB.
5️⃣ Difference between Encapsulation and Abstraction?
Feature | Encapsulation | Abstraction |
Focus | Data hiding | Logic hiding |
Achieved by | Access modifiers, getters/setters | Interfaces, abstract classes |
Example | Private age field | Vehicle interface with drive() |
6️⃣ How do Access Modifiers work in Java?
private
: Accessible only in the classdefault
: Package-privateprotected
: Accessible in package + subclasspublic
: Accessible everywhere
Used to control visibility and enforce encapsulation.
7️⃣ How is Encapsulation Implemented in Java?
Using:
Private variables
Public getters/setters
Validation inside setters
This helps secure data and control access.
8️⃣ How is Abstraction Implemented in Java?
Using:
abstract
classes (can have method bodies)interfaces
(pure abstraction until Java 8+)
Example:
interface Animal {
void makeSound(); // Abstract method
}
9️⃣ What are Libraries in Java?
Java comes with rich built-in libraries like:
java.util.*
(collections)java.io.*
(file handling)java.net.*
(networking)
Also, many external libraries like Apache, Jackson, etc.
🔟 Why Can Java Run Across Platforms?
Because of the Java Virtual Machine (JVM).
Java code → Compiled to bytecode → Run by JVM on any OS
"Write once, run anywhere" 🔁
1️⃣1️⃣ Procedural vs OOPs – What’s Better?
Feature | Procedural | OOPs |
Approach | Step-by-step | Object-focused |
Reusability | Hard to reuse | Easy via classes/inheritance |
Real-world Mapping | Not direct | Very close (Person, Vehicle, etc.) |
✅ OOPs makes code scalable, modular, and easier to maintain.
1️⃣2️⃣ One Key Reason for Java's Popularity?
Java's platform independence + automatic memory management (GC) made it ideal for:
Web apps
Enterprise software
Android apps
1️⃣3️⃣ How Does Java Handle Memory?
Java uses Garbage Collection (GC) to automatically free unused memory. No need to manually allocate or deallocate like in C/C++.
1️⃣4️⃣ What is Polymorphism?
Polymorphism means many forms—same method name, different behavior.
Compile-time: Method overloading
Runtime: Method overriding
class Shape {
void draw() { System.out.println("Drawing shape"); }
}
class Circle extends Shape {
void draw() { System.out.println("Drawing circle"); }
}
1️⃣5️⃣ What is Inheritance?
Inheritance allows one class to inherit fields/methods from another.
class Animal {
void sound() { System.out.println("Some sound"); }
}
class Dog extends Animal {
void sound() { System.out.println("Bark"); }
}
✅ Promotes code reuse and hierarchy.
🚀 Wrap-Up & What’s Next?
These 15 questions are must-know for any Java/OOPs interview. Practice writing small programs and understand the concepts deeply—not just definitions.
🧠 Want to go deeper and build real projects?
Join our Java + AWS Internship today:
👉 https://techeazyconsulting.com/class-detail/AWS-Internship
Subscribe to my newsletter
Read articles from TechEazy Consulting directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
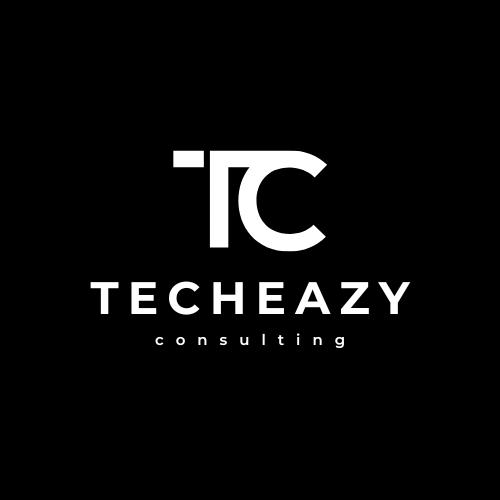