Java Database Connectivity: Beyond Basic JDBC
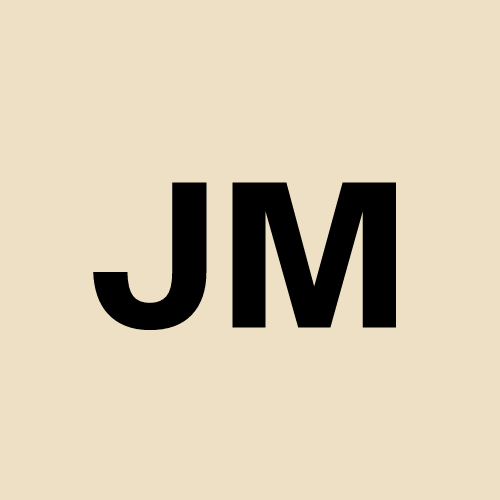

If you’re a Java developer, chances are you’ve worked with databases in some form or another. Java Database Connectivity (JDBC) is the standard API for connecting Java applications to relational databases, like MySQL, PostgreSQL, or Oracle. It’s pretty much the go-to solution for database interaction in Java, but like anything in the world of software development, it has its limits.
So, let’s go beyond the basics of JDBC and explore the more advanced strategies and tools that can make your database interactions faster, more efficient, and—dare we say—fun. If you’re looking to level up your database game, you’ve come to the right place.
What is JDBC, Anyway?
Before we dive into the deep end, let’s do a quick recap for those who might need a refresher. JDBC is the Java API that enables Java applications to interact with relational databases. It provides methods to execute SQL queries, update data, and retrieve results. The basic JDBC workflow looks something like this:
Establish a Connection: Using a
DriverManager
orDataSource
, you get a connection to the database.Execute Queries: You write SQL queries to interact with the database, like
SELECT
,INSERT
, orUPDATE
.Process Results: After executing the queries, you handle the results using
ResultSet
objects.Close Connections: Finally, it’s important to close your resources to avoid memory leaks or connection issues.
Pretty straightforward, right? But JDBC, while powerful, is not always the best solution for every scenario. That’s where things get interesting.
The Limitations of Basic JDBC
Now, don’t get us wrong, JDBC is foundational and still very much used in the industry. But here’s the thing: it’s not the most efficient way to interact with databases, especially for larger applications. The basic JDBC approach doesn’t handle a lot of the behind-the-scenes tasks, such as connection pooling, transaction management, and object-relational mapping (ORM).
In larger applications, this can become a major bottleneck. It’s like trying to use a butter knife to cut through a steak—sure, it works, but it’s not the best tool for the job. That’s why many developers look beyond the basics of JDBC and explore more advanced techniques and tools to streamline database interaction.
Going Beyond JDBC: What Are Our Options?
1. Connection Pooling: Why It Matters
Connection pooling is one of the most important optimizations you can make when working with databases in Java. When you’re using basic JDBC, every time you establish a connection to the database, it involves time-consuming network operations, authentication, and resource allocation. This can quickly lead to performance issues, especially in high-traffic applications.
A connection pool is a collection of reusable connections. Instead of opening a new connection every time you need one, the application pulls an existing one from the pool. This is a massive performance boost, as opening and closing connections are resource-intensive operations.
Here’s where it gets interesting: You don’t have to manually implement connection pooling. There are several libraries out there that do it for you, such as HikariCP and Apache DBCP. These libraries manage the pool of connections, ensuring that resources are used efficiently and that the connections are automatically closed when they’re no longer needed.
Java Homework Help: If you’re struggling with setting up a connection pool in your Java app, don’t sweat it. Whether you’re new to connection pooling or just need a refresher on how it works with JDBC, there are plenty of resources to guide you through the process.
2. ORM (Object-Relational Mapping): Making Things Simpler
JDBC is all about working with SQL directly, which means you have to manually map data from your database to your Java objects. For example, if you have a User
class in your app, and a table in the database that stores user information, you need to map the columns of that table to the fields of your Java class.
But let’s be honest: manually handling this mapping is tedious and error-prone, especially when you have a large database with lots of relationships between tables. That’s where ORM frameworks like Hibernate, EclipseLink, and Spring Data JPA come in. They do all the heavy lifting for you.
ORM tools allow you to map Java objects to database tables using annotations or XML configuration. This means that when you retrieve a User
from the database, it’s automatically mapped to your Java object. No more worrying about manually mapping result sets. The ORM handles all of this under the hood.
Let’s take Hibernate as an example: With Hibernate, you don’t need to write SQL queries for basic CRUD operations (Create, Read, Update, Delete). You can use methods like save()
, find()
, and delete()
, and Hibernate will automatically generate the SQL for you.
While Hibernate is extremely powerful, it also introduces some overhead. It’s not the best option for every project, especially if you need fine-grained control over the SQL. But for many projects, it’s a huge time-saver.
3. JPA (Java Persistence API)
JPA is a Java standard that defines a set of interfaces and annotations for working with ORM. Think of JPA as a specification, and frameworks like Hibernate and EclipseLink as implementations of that specification. If you’re using an ORM framework, you’re probably also using JPA.
JPA defines things like Entity Manager for interacting with the database, and JPQL (Java Persistence Query Language) for querying the database. JPQL is similar to SQL but operates on Java objects rather than database tables, making it more natural to use within Java applications.
One thing that makes JPA stand out is its integration with Java EE (now Jakarta EE) and Spring. It’s the default persistence standard in these frameworks, meaning you don’t have to go hunting for third-party libraries to handle persistence. JPA is standardized, well-documented, and supported by the majority of Java frameworks.
4. Spring Data: Simplifying Database Access
If you’re already using the Spring Framework, then Spring Data is your best friend when it comes to database access. Spring Data builds on JPA and ORM frameworks to simplify the process of interacting with databases.
With Spring Data, you don’t even need to write repository methods. You can create a repository interface and let Spring generate the implementation for you. For example, you could create a UserRepository
interface with methods like findByUsername()
or deleteById()
, and Spring will automatically generate the queries for you. This is huge, as it reduces boilerplate code and lets you focus on the business logic of your application.
Spring Data is also fully compatible with NoSQL databases like MongoDB, so if you decide to use something other than a relational database in the future, Spring Data has got you covered.
5. Database Transactions in Java
Transactions are critical in any database interaction because they ensure that a series of operations are executed atomically—meaning that either all operations succeed, or none of them do. When using JDBC directly, you’ll need to manage transactions manually. You have to handle things like commit and rollback operations, which can get messy.
ORM frameworks like Hibernate and JPA handle transactions for you in most cases, but you still need to be aware of how transactions work in Java. For example, in Spring, transactions are managed using the @Transactional
annotation, which ensures that all database operations within a method are treated as a single unit.
Understanding transactions is crucial because improper transaction handling can lead to data inconsistencies or even corruption. So, make sure you grasp this concept, even if you’re using a framework to simplify things.
Real-World Applications: When to Go Beyond JDBC
So, when should you start looking beyond the basic JDBC? In small-scale projects, using JDBC directly might be perfectly fine. It’s lightweight and gives you complete control. However, as your application grows in complexity, managing connections, transactions, and object mappings becomes more difficult and error-prone.
Here are some scenarios where you’ll benefit from going beyond basic JDBC:
Large Applications: If your application needs to scale, an ORM framework like Hibernate will save you time by automating repetitive database tasks.
Microservices: For microservices, consider using Spring Data JPA or a similar tool. These frameworks make it easy to create and manage the data layer of your microservices.
Complex Relationships: When dealing with many-to-many or one-to-many relationships in your database, using an ORM can simplify the mapping of these relationships.
Wrapping It Up
JDBC is a solid foundation for Java database connectivity, but as applications grow in complexity, it’s essential to move beyond the basics. Connection pooling, ORM frameworks, JPA, and Spring Data are all powerful tools that can make your life as a developer much easier. The key is to choose the right tool for the job—whether you need fine-grained control over your SQL, or you just want to speed up development and avoid repetitive coding.
And hey, if you ever find yourself stuck, or if you just need a little extra guidance, Java Homework Help is out there, ready to help you get over any bumps in the road. You’re not in this alone!
Read More-Ethics in Computing: Preparing for Responsible Tech Careers
Subscribe to my newsletter
Read articles from Jones Miller directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
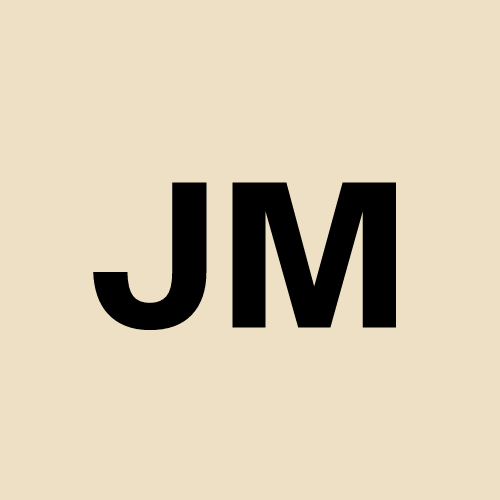