Git Conflict Resolution Exercises: A Poetic Journey
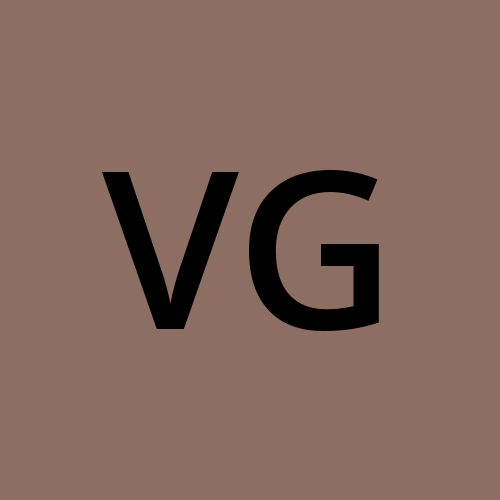
Table of contents
- Setup
- Scenario 1: Adjacent-Line Conflict
- Scenario 2: Binary File Conflict (Revised)
- Scenario 3: Merge vs. Rebase Conflict
- Scenario 4: Cherry-Pick Conflict (Revised)
- Scenario 5: Resolving Conflicts in a Poem with Diverged Branches
- Scenario 6: Resolving Renamed File Conflicts
- Scanerio 7: Resolving Deleted File Conflicts

Setup
Initialize Repository:
mkdir git-poem-exercise
cd git-poem-exercise
git init
echo -e "The sun dips low in twilight's glow,\nShadows stretch across the snow.\nA lone wolf howls a mournful tune,\nBeneath the watchful eye of the moon." > poem.txt
git add poem.txt && git commit -m "Initial poem"
Scenario 1: Adjacent-Line Conflict
Objective: Resolve conflicts in adjacent lines (e.g., line 2 and 3).
Setup:
In
branch1
, edit line 2:git checkout -b branch1 # Change line 2 to emphasize darkness: sed -i '2s/.*/Darkness creeps where shadows grow,/' poem.txt git commit -am "branch1: Rephrase line 2"
In
branch2
, edit line 3:git checkout main git checkout -b branch2 # Add environmental details to line 3: sed -i '3s/.*/A lone wolf howls a mournful tune in the frosty air,/' poem.txt git commit -am "branch2: Expand line 3"
Conflict
Merge branch2
into main
after merging branch1
:
git checkout main
git merge branch1
git merge branch2
Conflict:
Git may flag adjacent changes if the modifications are close enough to overlap in context. Example conflict markers:
Resolution
Identify Changes
Adjust Changes
Commit the Merge:
git add poem.txt git commit -m "Merge branch2 into main: Resolve adjacent-line conflict"
Scenario 2: Binary File Conflict (Revised)
Objective: Resolve conflicts in binary files (e.g., image.png
).
Setup:
Add
image_v1.png
tomain
:cp ~/Downloads/image_v1.png image.png git add image.png git commit -m "Add image_v1.png" # Commit to main
In
branch1
, replaceimage.png
withimage_v2.png
:git checkout -b branch1 cp ~/Downloads/image_v2.png image.png git commit -am "Update image to v2" # Commit to branch1
In
branch2
, replaceimage.png
withimage_v3.png
:git checkout main git checkout -b branch2 cp ~/Downloads/image_v3.png image.png git commit -am "Update image to v3" # Commit to branch2
Conflict:
Merge branch1
into branch2
:
git merge branch1
Git will report a conflict for image.png
because both branches modified it .
Resolution:
Choose which version to keep:
Keep
branch1
'simage_v2.png
:git checkout branch1 -- image.png # Overwrite with v2
OR keep
branch2
'simage_v3.png
:git checkout branch2 -- image.png # Keep v3
Commit the resolved file:
git commit -am "Resolve binary conflict" # Finalize merge
Scenario 3: Merge vs. Rebase Conflict
Objective: Compare merge and rebase workflows.
Setup:
In
feature
, edit line 5:git checkout -b feature # Change "frankincense" to "myrrh" git commit -am "feature: use myrrh"
In
main
, edit line 5 to:# Change "frankincense" to "lavender" git commit -am "main: use lavender"
Conflict:
Merge:
git merge feature # Conflict in line 5
git merge --quit
git switch --force # although this a messed up situation hence better option would be to reolve the conflict manually by accepting eitehr of the two
this is where rebase comes into picture when and if we know that main is ahead of feature branch then we usually rebase or bring main chnages to feature then we merger feature to main
Rebase:
git checkout feature git rebase main # Conflict during rebase
Resolution:
For merge: Resolve conflict and commit.
For rebase: Resolve conflict, then
git rebase --continue
.
Scenario 4: Cherry-Pick Conflict (Revised)
Objective: Resolve conflicts when cherry-picking a commit that modifies the same line.
Setup:
Create
branch1
and modify line 4:git checkout -b branch1 # Edit line 4 to add "lilies" (e.g., "roses lilies") git commit -am "branch1: add lilies to line 4" # Commit to branch1
Switch to
main
, modify the same line:git checkout main # Edit line 4 to replace "leaves" with "petals" (e.g., "roses petals") git commit -am "main: replace leaves with petals in line 4" # Commit to main
Conflict:
Cherry-pick the commit from branch1
into main
:
git cherry-pick <commit-hash> # Replace <commit-hash> with the hash from branch1’s commit
Git will report a conflict in poem.txt
because both branches modified line 4 .
Resolution:
Manually resolve the conflict:
Open
poem.txt
. You’ll see conflict markers:<<<<<<< HEAD roses petals ======= roses lilies >>>>>>> <commit-hash>
Edit line 4 to merge both changes (e.g.,
roses petals lilies
).
Complete the cherry-pick:
git add poem.txt git cherry-pick --continue # Finalize the commit
Scenario 5: Resolving Conflicts in a Poem with Diverged Branches
Objective: Resolve merge conflicts between two branches that modified overlapping lines of a poem.
Poem File: poem.txt
with the following content:
The sun dips low in twilight's glow,
Shadows stretch across the snow.
A lone wolf howls a mournful tune,
Beneath the watchful eye of the moon.
Setup
Initialize Repository:
git init echo -e "The sun dips low in twilight's glow,\nShadows stretch across the snow.\nA lone wolf howls a mournful tune,\nBeneath the watchful eye of the moon." > poem.txt git add poem.txt && git commit -m "Initial poem"
Create
branch1
with 3 commits:Commit 1: Modify line 1 (sun imagery):
git checkout -b branch1 sed -i "1s/.*/The sun sinks low in twilight's haze,/" poem.txt git commit -am "Branch1: Change 'dips' to 'sinks' and 'glow' to 'haze'"
Commit 2: Modify line 3 (wolf's action):
sed -i "3s/.*/A lone wolf sings a lonely song,/" poem.txt git commit -am "Branch1: Change 'howls a mournful tune' to 'sings a lonely song'"
Commit 3: Modify line 4 (moon description):
sed -i "4s/.*/Under the moon\'s soft silver gaze./" poem.txt git commit -am "Branch1: Rewrite line 4"
Create
branch2
with 2 commits:Switch back to
main
and createbranch2
:git checkout main git checkout -b branch2
Commit 1: Modify line 3 (wolf's song):
sed -i "3s/.*/A solitary wolf's sad refrain,/" poem.txt git commit -am "Branch2: Rewrite line 3"
Commit 2: Modify line 4 (moon's role):
sed -i "4s/.*/In the moonlight's gentle, silent reign./" poem.txt git commit -am "Branch2: Rewrite line 4"
Conflict
Merge branch2
into branch1
:
git checkout branch1
git merge branch2
Conflict in poem.txt
:
Lines 3 and 4 are modified in both branches.
Conflict markers will appear:
<<<<<<< HEAD
A lone wolf sings a lonely song,
Under the moon's soft silver gaze.
=======
A solitary wolf's sad refrain,
In the moonlight's gentle, silent reign.
>>>>>>> branch2
Resolution
Identify Overlapping Changes:
Usegit diff
to compare branches:git diff branch1..branch2
Output highlights conflicting changes to lines 3 and 4.
Resolve Conflicts:
Editpoem.txt
to combine changes:A lone wolf sings a sad refrain, Beneath the moon's soft, silent reign.
Line 3: Merge "sings" (branch1) and "sad refrain" (branch2).
Line 4: Combine "soft" (branch1) and "silent reign" (branch2).
Commit the Merge:
git add poem.txt git commit -m "Merge branch2 into branch1: Resolve line 3/4 conflicts"
Final Poem
The sun sinks low in twilight's haze,
Shadows stretch across the snow.
A lone wolf sings a sad refrain,
Beneath the moon's soft, silent reign.
This exercise demonstrates how to resolve overlapping line changes in diverged branches using git diff
and manual conflict resolution.
Scenario 6: Resolving Renamed File Conflicts
Objective: Resolve merge conflicts when a file is renamed in one branch and edited in another.
File: poem.txt
(from previous scenarios).
Setup
Create
branch1
(rename file):git checkout -b branch1 git mv poem.txt elegy.txt git commit -m "Rename poem.txt to elegy.txt"
Create
branch2
(edit original file):git checkout main git checkout -b branch2 echo "The night embraces all in quiet grace." >> poem.txt git commit -am "branch2: Add new line to poem.txt"
Conflict
Merge branch2
into branch1
:
git checkout branch1
git merge branch2
Conflict:
poem.txt
was modified inbranch2
but renamed toelegy.txt
inbranch1
.Git marks
poem.txt
as "deleted by us" (due to rename) and "modified by them" (changes inbranch2
).
Resolution
Identify Changes in
branch2
:
Extract the new line added topoem.txt
inbranch2
:git show branch2:poem.txt > temp.txt tail -n 1 temp.txt # Output: "The night embraces all in quiet grace." rm temp.txt
Apply Changes to Renamed File:
Append the new line toelegy.txt
:echo "The night embraces all in quiet grace." >> elegy.txt
Resolve the Conflict:
Remove the conflictingpoem.txt
(already renamed) and stage changes:git rm poem.txt git add elegy.txt git commit -m "Merge branch2 into branch1: Resolve rename/edit conflict"
Final File (elegy.txt
)
The sun dips low in twilight's glow,
Shadows stretch across the snow.
A lone wolf howls a mournful tune,
Beneath the watchful eye of the moon.
The night embraces all in quiet grace.
Key Takeaways
Git may not automatically detect that a modified file in one branch corresponds to a renamed file in another.
Use
git show
to recover changes from the original file path (branch2:poem.txt
).Manually apply changes to the renamed file (
elegy.txt
) and resolve the conflict by deleting the outdated path.
Scanerio 7: Resolving Deleted File Conflicts
Objective: Resolve merge conflicts when a file is deleted in one branch and modified in another.
File: poem.txt
(from previous scenarios).
Setup
Create
branch1
(delete file):git checkout -b branch1 git rm poem.txt git commit -m "Delete poem.txt in branch1"
Create
branch2
(edit file):git checkout main git checkout -b branch2 echo "The night whispers secrets to the stars." >> poem.txt git commit -am "branch2: Add new line to poem.txt"
Conflict
Merge branch2
into branch1
:
git checkout branch1
git merge branch2
Conflict:
poem.txt
was deleted inbranch1
but modified inbranch2
.Git marks the conflict as:
CONFLICT (modify/delete): poem.txt deleted in HEAD and modified in branch2. Version branch2 of poem.txt left in tree.
Resolution
Option 1: Keep poem.txt
with changes from branch2
Restore the modified file:
git checkout branch2 -- poem.txt
Commit the merge:
git add poem.txt git commit -m "Merge branch2 into branch1: Restore poem.txt with changes"
Option 2: Permanently delete poem.txt
Remove the file and accept deletion:
git rm poem.txt git commit -m "Merge branch2 into branch1: Delete poem.txt"
Final Outcome
If restored (
poem.txt
exists):The sun dips low in twilight's glow, Shadows stretch across the snow. A lone wolf howls a mournful tune, Beneath the watchful eye of the moon. The night whispers secrets to the stars.
If deleted:
poem.txt
no longer exists in the repository.
Key Takeaways
Git flags a conflict when a file is modified in one branch and deleted in another.
Use
git checkout <branch> -- <file>
to recover changes from a specific branch.Use
git rm
to finalize deletion if the file is no longer needed.Always verify changes with
git status
andgit diff
before committing.
Subscribe to my newsletter
Read articles from Vaibhav Gagneja directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
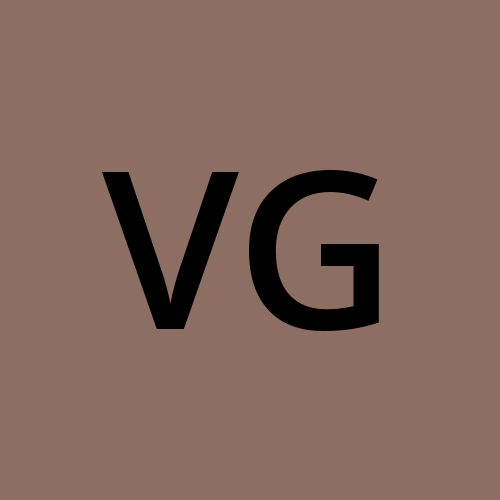