How to Create Azure Function Webhooks for Sitecore XM Cloud & Experience Edge Integration
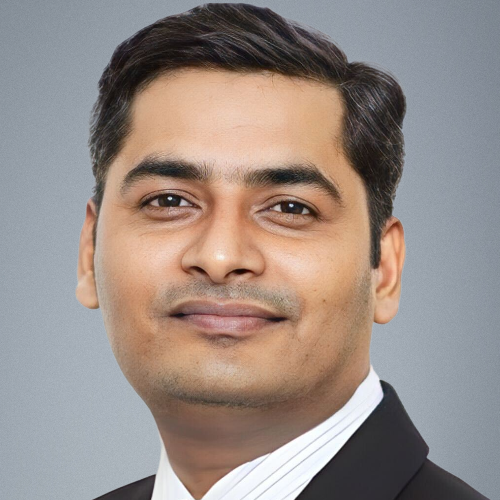
Table of contents
- Introduction: Azure Functions & Sitecore Integration Overview
- Understanding Azure Functions and Webhooks
- How to Create an Azure Functions Webhook for XM Cloud Integration
- Important Considerations for XM Cloud vs Experience Edge Webhooks
- Use Cases for Azure Function Webhooks in XM Cloud
- 💡Conclusion
- 🙏Credit/References
- 🏓Pingback

Introduction: Azure Functions & Sitecore Integration Overview
In the world of digital experiences, connecting Azure Functions with Sitecore XM Cloud and Experience Edge is a great way to automate tasks and react to live events. This guide explains how to create Azure Function webhooks and link them with Sitecore products to manage publishing events, content updates, and more. 🔝
Understanding Azure Functions and Webhooks
What are Azure Functions?
Azure Functions is a robust serverless computing service that empowers developers to create scalable applications effortlessly by eliminating infrastructure management. It excels in event-driven execution, making it perfect for automating workflows and integrating cloud services for real-time communication between applications. By combining Azure Functions with webhooks, you can seamlessly automate processes and connect systems.
These lightweight, event-driven code blocks run in the cloud, perfect for: 🔝
Processing file uploads
Real-time data transformation
Running AI inference tasks
Building scalable APIs
Creating serverless workflows
What are Webhooks in Azure Functions?
Webhooks are dynamic tools that activate Azure Functions in response to specific events, fueling cloud automation and efficient data exchange. These HTTP callbacks facilitate instant communication between applications. Defined by users, webhooks are triggered by events like code pushes or wiki updates or CMS update. When an event occurs, the source site sends an HTTP request to the webhook's configured URL. With Azure Functions, you can craft logic that executes upon receiving a webhook message.
How to Create an Azure Functions Webhook for XM Cloud Integration
Step 1: Set Up Your Azure Functions Webhook
Follow these steps to set up your first Azure Function in C#: 🔝
Create a Resource Group – Sign in to the Azure portal using your MS Learn account and Create a resource group
Create a new project – Watch the video below to learn how to create a new Azure Function project using Visual Studio in C#.
Develop and run Azure Functions locally – Before deploying your Azure Function to Azure, it's a good practice to develop and test your Azure Functions code project locally.
✔ Set your Azure Function project as the startup project and run it. 🔝
Azure Functions HTTP trigger in browser – The
LOCAL
function URL start with LOCALHOST, like this: https://localhost:1234/api/Function1. The Function Name always followsapi
. When running the Azure Function fromAzure
, your Azure Function URL will be like https://my-function-app.azurewebsites.net/api/Function1. 🔝Call Azure Function (HTTP Trigger) from Postman – Use the HTTP
POST
orGET
method, depending on your function's requirements, to trigger the Azure Function. It will return a response from your Azure Function, if there is one. 🔝ℹ️ In the video, you can find a step-by-step guide to creating Azure Function Webhooks
Capture Incoming Webhook JSON Payload using Azure Function – In Sitecore XM Cloud or Sitecore Experience Edge (Experience Edge webhooks have two execution modes: OnEnd and OnUpdate), webhooks can inform other systems about publishing events or workflow changes. They are important for connecting with external services to improve functionality. To do this, we need to update our Azure Function to read the JSON payload, extract the needed data, and change it as required or for use by an external system. 🔝
The video below will show you how to add the new Azure Function to your VS Solution and how to execute and validate the webhook:
Code for the webhook that reads the JSON (JavaScript Object Notation) payload: 🔝
using Microsoft.Azure.Functions.Worker; using Microsoft.AspNetCore.Mvc; using Microsoft.AspNetCore.Http; using Newtonsoft.Json.Linq; namespace XmCloudTriggers { /// <summary> /// This class contains a function that reads a JSON object from the request body and returns it as a string. /// A webhook is a method for a service to give other applications real-time information. /// Webhooks are sometimes called reverse APIs or HTTP callbacks. /// Experience Edge webhooks have two execution modes: OnEnd and OnUpdate /// </summary> public static class ReadJSON { /// <summary> /// This method is triggered by an HTTP request and reads a JSON object from the request body and sends it back as a string. /// </summary> /// <param name="req"></param> /// <returns></returns> [Function("ReadJSON")] public static async Task<IActionResult> Run([HttpTrigger(AuthorizationLevel.Function, "get", "post")] HttpRequest req) { string jsonString = string.Empty; try { string requestBody = await new StreamReader(req.Body).ReadToEndAsync(); dynamic jObject = JObject.Parse(requestBody); jsonString = Convert.ToString(jObject); } catch (Exception ex) { return new BadRequestObjectResult("Error processing request "+ ex.Message); } return new OkObjectResult(jsonString); } } }
This method begins with an HTTP request, reads a JSON object from the request body, and returns it as a string. 🔝
Step 2: Generate Client Credentials for XM Cloud
To use Sitecore’s Experience Edge APIs, you need to create an Edge administration Client ID and Client Secret for your specific XM Cloud Project and XM Cloud Environment at the organization level. You can find the details in the video below:
Step 3: Request an Access Token
To get an access_token
, which is needed later to register your custom Azure Function Webhook with Sitecore’s Experience Edge, you need to make a POST request to https://auth.sitecorecloud.io/oauth/token
with required parameters like audience
, grant_type
, client_id
, and client_secret
🔝
POST
request output: 🔝
{
"access_token": "amit-kumar",
"scope": "edge.caches:clear edge.settings:read edge.settings:update edge.webhooks:create edge.webhooks:read edge.webhooks:update edge.webhooks:delete edge.tokens:create edge.tokens:read edge.tokens:update edge.tokens:delete edge.content:publish edge.content:clearall",
"expires_in": 86400,
"token_type": "Bearer"
}
Step 4: Register Your Webhook with Experience Edge
To create webhooks with Sitecore Experience Edge, Experience Edge offers webhook execution modes that alert your Azure Function when specific events happen. You can set up webhooks using Sitecore’s Experience Edge Admin API 🔝
Experience Edge Execution Modes
OnEnd: Triggered when publishing is completed.
OnUpdate: Includes entity changes in the webhook payload.
To receive detailed updates about entity changes during publishing, we use the OnUpdate
mode. This allows us to get real-time notifications about content updates in Experience Edge. 🔝
Steps to Register Your Webhook
Use the CreateWebhook endpoint.
Provide a secure HTTPS endpoint (your Azure Function URL).
POST
request Body: 🔝// POST: https://edge.sitecorecloud.io/api/admin/v1/webhooks { "label": "Webhook-ReadJSON", "uri": "https://learn-azure-functions.azurewebsites.net/api/ReadJSON", "method": "POST", "headers": { "x-header": "bar" }, "createdBy": "Amit Kumar", "executionMode": "OnUpdate" }
Once registered, use the
ListWebhooks
endpoint to confirm whether your custom Azure Function Webhook has been registered with Sitecore Experience Edge:GET
request output: 🔝// GET: https://edge.sitecorecloud.io/api/admin/v1/webhooks [ { "id": "ak-amit-kumar", "tenantId": "xmctenant", "label": "Webhook-ReadJSON", "uri": "https://learn-azure-functions.azurewebsites.net/api/ReadJSON", "method": "POST", "headers": { "x-header": "bar" }, "body": "", "createdBy": "Amit Kumar", "created": "2025-04-09T11:50:39.0837718+00:00", "bodyInclude": null, "executionMode": "OnUpdate", "lastRuns": [ { "timestamp": "2025-04-10T16:52:59.663868+00:00", "success": true } ], "disabled": null } ]
Important Considerations for XM Cloud vs Experience Edge Webhooks
Sitecore distinguishes between: 🔝
XM Cloud Webhooks – Triggered by internal XM Cloud events.
Experience Edge Webhooks – Triggered by publishing actions on Experience Edge.
Ensure you're using the correct type of webhook depending on your integration scenario.
🔗 Learn more about supported events here:
Use Cases for Azure Function Webhooks in XM Cloud
Azure Function webhooks can be expanded to handle various use cases within XM Cloud and Sitecore composable products: 🔝
🔍 Triggering Sitecore Search Indexing
🧩 Rebuilding XM Cloud Pages
✅ Validating Workflow Changes
🔄 Syncing External Systems
💡Conclusion
By using Azure Functions and webhooks, you can automate tasks, improve integrations, and react instantly to events in XM Cloud and Experience Edge. Begin with a basic HTTP-triggered function, add authentication, and link it to Sitecore’s strong APIs for smooth automation. 🔝
🙏Credit/References
🏓Pingback
How to Set Up Azure Function Webhooks for Sitecore XM Cloud: Learn step-by-step how to create Azure Function webhooks and integrate them with Sitecore XM Cloud for real-time event processing. | Serverless Automation-Using Azure Functions with Sitecore Experience Edge: Discover how to use Azure Functions to automate workflows in Sitecore Experience Edge for seamless content delivery. | Real-Time Publishing Notifications with XM Cloud Webhooks: Configure webhooks in XM Cloud to receive instant notifications when publishing events occur. 🔝 |
Step-by-Step Guide - Integrating Azure Functions with Sitecore Admin API: A detailed tutorial on connecting Azure Functions to Sitecore’s Admin API for custom automation tasks. | OnEnd vs. OnUpdate- Choosing the Right Webhook Mode in Experience Edge: Understand the differences between OnEnd and OnUpdate webhook execution modes in Sitecore Experience Edge. | How to Generate and Use JWT Tokens for Sitecore XM Cloud: Learn how to securely generate and use JWT tokens to authenticate with Sitecore XM Cloud APIs. |
Automating Search Indexing in XM Cloud with Azure Functions: Use Azure Functions to trigger search index updates automatically when content changes in XM Cloud. | Top 5 Use Cases for Webhooks in Sitecore XM Cloud: Explore the best ways to leverage webhooks in XM Cloud for form processing, publishing alerts, and more. | Securing Your Azure Function Webhooks for XM Cloud: Best practices for securing your Azure Function webhooks when integrating with Sitecore XM Cloud. |
Troubleshooting Common Issues with XM Cloud Webhooks: Fix common problems when setting up and using webhooks in Sitecore XM Cloud. | Step-by-Step Guide to Creating Azure Function Webhooks: Learn how to create Azure Function webhooks to enhance serverless computing and integrate with Sitecore XM Cloud for real-time publishing events. | What Are Webhooks? A Beginner’s Guide to Real-Time Integration: Discover the basics of webhooks and how they enable real-time communication between applications like Sitecore XM Cloud and Azure Functions. 🔝 |
Subscribe to my newsletter
Read articles from Amit Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
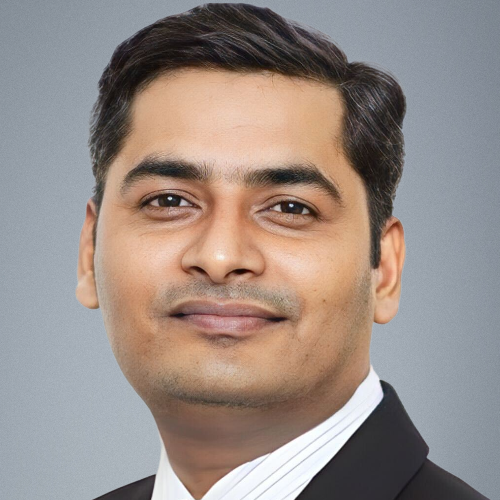
Amit Kumar
Amit Kumar
My name is Amit Kumar. I work as a hands-on Solution Architect. My experience allows me to provide valuable insights and guidance to organizations looking to leverage cutting edge technologies for their digital solutions.As a Solution Architect, I have extensive experience in designing and implementing robust and scalable solutions using server-side and client-side technologies. My expertise lies in architecting complex systems, integrating various modules, and optimizing performance to deliver exceptional user experiences. Additionally, I stay up-to-date with the latest industry trends and best practices to ensure that my solutions are always cutting-edge and aligned with business objectives.