โก Electron.js Cheat Sheet (v30+)
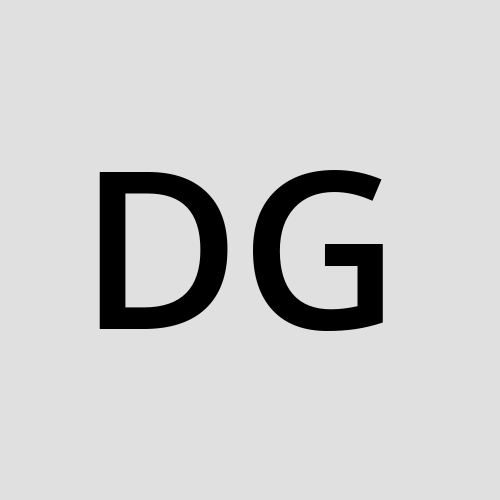

๐ฆ Installation
npm install --save-dev electron
Add to package.json
:
"scripts": {
"start": "electron ."
}
๐ Project Structure
my-app/
โโโ main.js # Main process
โโโ preload.js # Preload script (security)
โโโ index.html # App UI
โโโ renderer.js # Renderer process
โโโ package.json
๐ Entry Point (Main Process)
main.js
const { app, BrowserWindow } = require('electron');
const path = require('path');
const createWindow = () => {
const win = new BrowserWindow({
width: 800,
height: 600,
webPreferences: {
preload: path.join(__dirname, 'preload.js'),
contextIsolation: true,
nodeIntegration: false
}
});
win.loadFile('index.html');
};
app.whenReady().then(createWindow);
app.on('window-all-closed', () => {
if (process.platform !== 'darwin') app.quit();
});
๐ Preload Script (Secure Bridge)
preload.js
const { contextBridge, ipcRenderer } = require('electron');
contextBridge.exposeInMainWorld('electronAPI', {
sendMessage: (msg) => ipcRenderer.send('message', msg),
onReply: (callback) => ipcRenderer.on('reply', (event, data) => callback(data))
});
๐ง Renderer Process (Web)
index.html
<!DOCTYPE html>
<html>
<head><title>Electron App</title></head>
<body>
<h1>Hello from Electron!</h1>
<button id="btn">Send</button>
<script src="renderer.js"></script>
</body>
</html>
renderer.js
document.getElementById('btn').addEventListener('click', () => {
window.electronAPI.sendMessage('Hi from Renderer');
});
window.electronAPI.onReply((data) => {
console.log('Received reply:', data);
});
๐จ IPC Communication
Main Process
const { ipcMain } = require('electron');
ipcMain.on('message', (event, msg) => {
console.log('Received:', msg);
event.reply('reply', 'Got your message!');
});
๐ Useful APIs
File Dialog
const { dialog } = require('electron');
dialog.showOpenDialog({ properties: ['openFile', 'multiSelections'] })
.then(result => console.log(result.filePaths));
System Info
const os = require('os');
console.log(os.platform(), os.cpus());
Notifications
new Notification({ title: 'Hello', body: 'This is a notification!' }).show();
๐ Security Tips
Use
contextIsolation: true
Disable
nodeIntegration
in the rendererUse
preload.js
to safely expose APIsValidate all IPC inputs
Avoid loading remote URLs unless trusted
๐งช Dev Tools
Open DevTools
win.webContents.openDevTools();
Enable Live Reload (for dev)
npm install electron-reload --save-dev
require('electron-reload')(__dirname);
๐ง Cross-Platform Build
Install Electron Forge:
npm install --save-dev @electron-forge/cli
npx electron-forge import
Build App:
npm run make
๐ Resources
Electron Docs
Context Isolation Guide
Security Best Practices
Subscribe to my newsletter
Read articles from David Gostin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
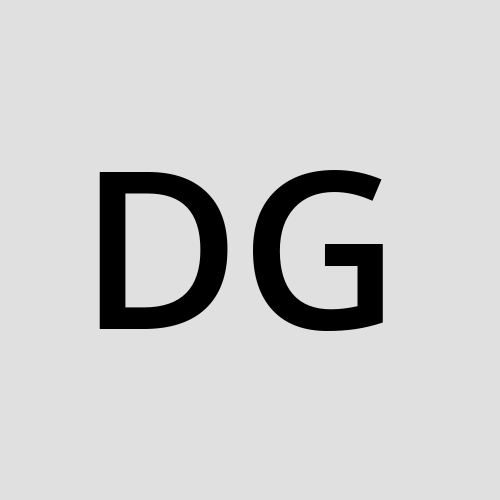
David Gostin
David Gostin
Full-Stack Web Developer with over 25 years of professional experience. I have experience in database development using Oracle, MySQL, and PostgreSQL. I have extensive experience with API and SQL development using PHP and associated frameworks. I am skilled with git/github and CI/CD. I have a good understanding of performance optimization from the server and OS level up to the application and database level. I am skilled with Linux setup, configuration, networking and command line scripting. My frontend experience includes: HTML, CSS, Sass, JavaScript, jQuery, React, Bootstrap and Tailwind CSS. I also have experience with Amazon EC2, RDS and S3.