Alignment and Arrangement in Jetpack Compose
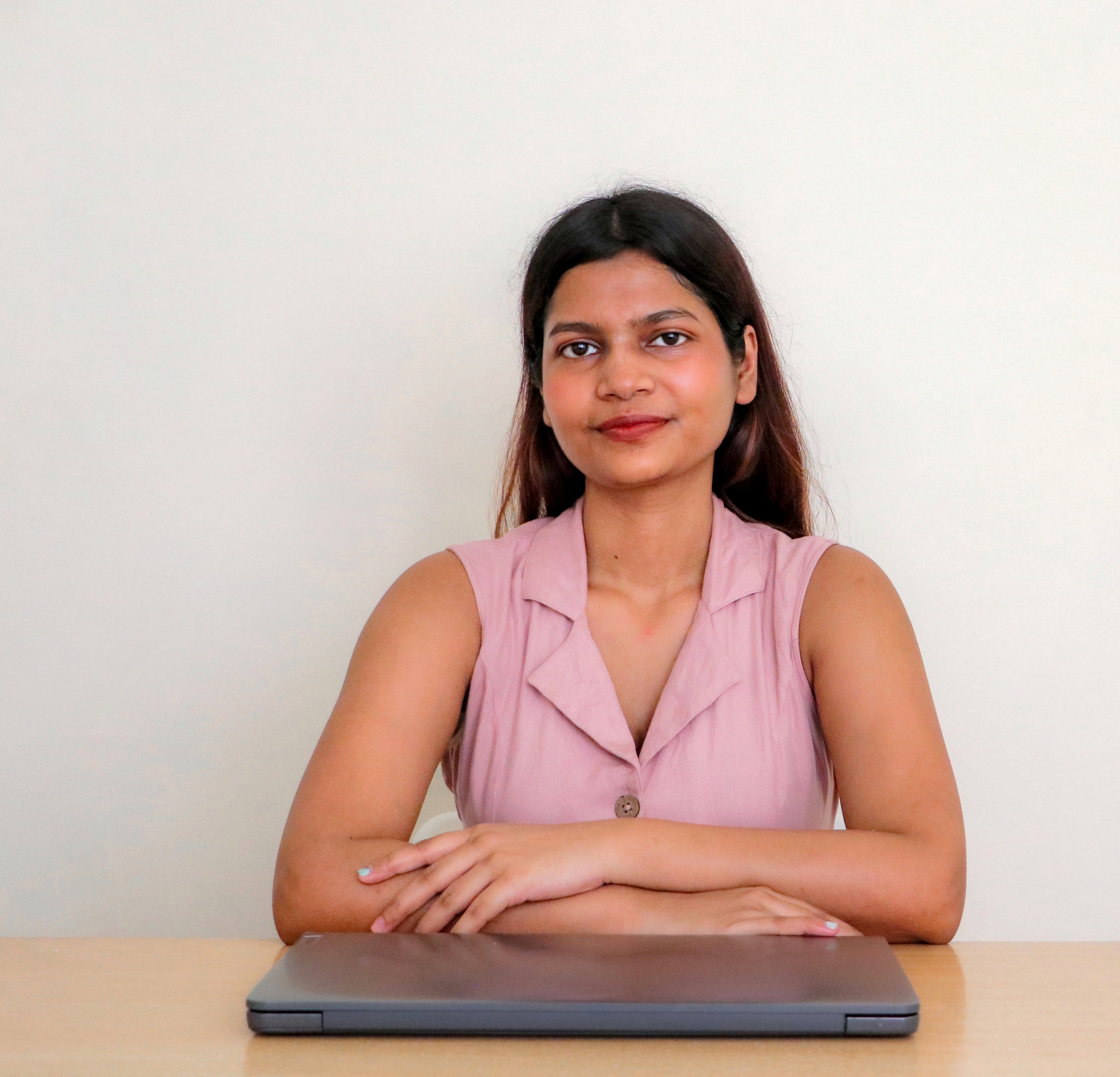

Introduction
When creating layouts in Jetpack Compose, two important things to understand are alignment and arrangement. These help you control where your UI elements appear on the screen — both within a container and in relation to each other.
When you arrange items in a layout like a Row or a Column, Jetpack Compose uses the idea of axes to control how the items are placed.
Main Axis
The main axis is the primary direction in which items are laid out:
In a Row, the main axis is horizontal (left to right)
In a Column, the main axis is vertical (top to bottom)
So, when you use horizontalArrangement in a Row, or verticalArrangement in a Column, you're controlling spacing along the main axis.
Cross Axis
The cross axis is perpendicular to the main axis:
In a Row, the cross axis is vertical
In a Column, the cross axis is horizontal
You control alignment along the cross axis using:
verticalAlignment in a Row (controls cross-axis alignment → vertical)
horizontalAlignment in a Column (controls cross-axis alignment → horizontal)
Arrangement in done on main axis while alignment is done on cross axis in various layouts.
Alignment
Alignment refers to the positioning of a composable element within its parent container, specifying how it should be placed relative to the available space along the horizontal and vertical axes.
Alignment tells Compose where to place a single item inside a layout. For example, if you want a Text to appear in the center of the screen or aligned to the start (left) or end (right), you use alignment.
In a Column or Row, you use:
horizontalAlignment → controls horizontal position of items inside a Column
verticalAlignment → controls vertical position of items inside a Row
In a Box, you use:
- contentAlignment → controls where to place the content (center, top-start, bottom-end, etc.)
Alignment Parameters
Row | Column |
Top | Start |
Center Vertically | center Horizontally |
Bottom | End |
Arrangement
Arrangement defines the distribution of multiple composables within a container, determining their placement along the main axis.
Arrangement is about spacing and positioning multiple items inside a layout. It decides how the children of a layout like Column or Row are spaced.
In a Column, you use:
- verticalArrangement → controls vertical spacing between items
In a Row, you use:
- horizontalArrangement → controls horizontal spacing between items
You can use options like:
Arrangement.Top, Arrangement.Center, Arrangement.Bottom
Arrangement.SpaceEvenly, Arrangement.SpaceBetween, Arrangement.SpaceAround
Arrangement.spacedBy(16.dp) → adds fixed spacing between items
Arrangement Parameters
Row | Column |
Start | Top |
Center | Center |
End | Bottom |
Space between, space around, space evenly, absolute, … | space between, space around, space evenly |
Space Between, Space Evenly, Space Around
Space Between
Distributes the children evenly, but with no space at the start or end of the container. The space is only between items.
Space Evenly
Distributes the children with equal space between them and also equal space before the first and after the last item.
Space Around
Distributes space evenly around each item. This means the edges get half the space compared to the space between items.
Scenario 1: Row Layout
A Row arranges items horizontally, side by side.
Arrangement (horizontal): Controls spacing between items
horizontalArrangement = Arrangement.Center → puts all items in the center
Arrangement.spacedBy(16.dp) → adds fixed space between items
Arrangement.SpaceBetween, SpaceAround, SpaceEvenly → distributes space in different ways
Alignment (vertical): Controls vertical alignment of items inside the row
verticalAlignment = Alignment.CenterVertically → centers items vertically
Alignment.Top, Alignment.Bottom → aligns items to top or bottom
package com.igdtuw.basicsjc
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.activity.enableEdgeToEdge
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.Scaffold
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import com.igdtuw.basicsjc.ui.theme.BasicsJCTheme
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.Row
import androidx.compose.foundation.layout.Arrangement
import androidx.compose.foundation.layout.height
import androidx.compose.foundation.layout.width
import androidx.compose.ui.Alignment
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.unit.dp
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
enableEdgeToEdge()
setContent {
BasicsJCTheme {
Scaffold(modifier = Modifier.fillMaxSize()) { innerPadding ->
RowLayout(innerPadding)
}
}
}
}
}
@Composable
fun RowLayout (innerPadding: androidx.compose.foundation.layout.PaddingValues) {
// Row Alignment (Cross Axis) Options:
// Alignment.Top, Alignment.CenterVertically, Alignment.Bottom
// Row Arrangement (Main Axis) Options:
// Arrangement.Start, Arrangement.Center, Arrangement.End,
// Arrangement.SpaceBetween, Arrangement.SpaceAround, Arrangement.SpaceEvenly
Row( modifier = Modifier.padding(innerPadding)
.fillMaxSize()
.background(Color.Yellow),
verticalAlignment = Alignment.CenterVertically, // Cross axis (vertical)
horizontalArrangement = Arrangement.SpaceBetween // Main axis (horizontal)
) {
Text(text = "Item 1", modifier = Modifier.background(Color.Green).width(50.dp).height(150.dp))
Text(text = "Item 2", modifier = Modifier.background(Color.Cyan).width(50.dp).height(150.dp))
Text(text = "item 3", modifier = Modifier.background(Color.Magenta).width(50.dp).height(150.dp))
}
}
This RowLayout composable creates a horizontal arrangement of three text items using the Row layout in Jetpack Compose. The entire layout fills the available screen and has a yellow background for visibility. Inside the row, each text item ("Item 1", "Item 2", and "Item 3") is displayed inside a colored box (green, cyan, and magenta respectively), with fixed dimensions of 150dp by 150dp. The items are arranged with equal horizontal spacing using Arrangement.SpaceEvenly, meaning equal space is placed before, between, and after each item. They are also vertically aligned to the center of the row using Alignment.CenterVertically, ensuring all items are aligned in the middle of the vertical axis. This example clearly demonstrates how alignment and arrangement can control positioning in a horizontal layout.
Scenario 2: Column Layout
A Column arranges items vertically, one below the other.
Arrangement (vertical): Controls spacing between items
verticalArrangement = Arrangement.Center → centers all items vertically
Arrangement.spacedBy(12.dp) → adds space between items
Arrangement.SpaceBetween, SpaceAround, etc.
Alignment (horizontal): Controls horizontal alignment of items
horizontalAlignment = Alignment.CenterHorizontally → centers items horizontally
Alignment.Start, Alignment.End → aligns items left or right
package com.igdtuw.basicsjc
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.activity.enableEdgeToEdge
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.Scaffold
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import com.igdtuw.basicsjc.ui.theme.BasicsJCTheme
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.Arrangement
import androidx.compose.foundation.layout.height
import androidx.compose.foundation.layout.width
import androidx.compose.ui.Alignment
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.unit.dp
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
enableEdgeToEdge()
setContent {
BasicsJCTheme {
Scaffold(modifier = Modifier.fillMaxSize()) { innerPadding ->
ColumnLayout(innerPadding)
}
}
}
}
}
@Composable
fun ColumnLayout(innerPadding: androidx.compose.foundation.layout.PaddingValues) {
// Column Alignment (Cross Axis) Options:
// Alignment.Start, Alignment.CenterHorizontally, Alignment.End
// Column Arrangement (Main Axis) Options:
// Arrangement.Top, Arrangement.Center, Arrangement.Bottom,
// Arrangement.SpaceBetween, Arrangement.SpaceAround, Arrangement.SpaceEvenly
Column( modifier = Modifier.padding(innerPadding)
.fillMaxSize()
.background(Color.Yellow),
horizontalAlignment = Alignment.CenterHorizontally, // Cross axis (horizontal)
verticalArrangement = Arrangement.SpaceEvenly // Main axis (vertical)
) {
Text(text = "Item 1", modifier = Modifier.background(Color.Green).width(150.dp).height(150.dp))
Text(text = "Item 2", modifier = Modifier.background(Color.Cyan).width(150.dp).height(150.dp))
Text(text = "item 3", modifier = Modifier.background(Color.Magenta).width(150.dp).height(150.dp))
}
}
This ColumnLayout composable creates a vertical arrangement of three text items using the Column layout in Jetpack Compose. The entire layout fills the available screen space and has a yellow background for visibility. Each text item ("Item 1", "Item 2", and "Item 3") is placed inside a colored box (green, cyan, and magenta respectively), with fixed dimensions of 150dp by 150dp. The items are aligned horizontally to the center of the column (Alignment.CenterHorizontally), and spaced evenly from top to bottom using Arrangement.SpaceEvenly, which distributes equal vertical space before, between, and after the items. Padding is applied to ensure the layout respects system UI spaces (like top bars). This example demonstrates how alignment and arrangement properties control the positioning of elements in a column.
Scenario 3: Box Layout
A Box stacks its children on top of each other, like layers.
Alignment (Box): You use contentAlignment to place items inside the Box
Alignment.Center → places content in the center
Alignment.TopEnd, BottomStart, etc. → places content in corners or sides
Child Alignment: Each child can also be individually aligned using Modifier.align(...)
No arrangement available in Box layout
package com.igdtuw.basicsjc
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.activity.enableEdgeToEdge
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.Scaffold
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import com.igdtuw.basicsjc.ui.theme.BasicsJCTheme
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.Box
import androidx.compose.foundation.layout.Arrangement
import androidx.compose.foundation.layout.height
import androidx.compose.foundation.layout.width
import androidx.compose.ui.Alignment
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.unit.dp
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
enableEdgeToEdge()
setContent {
BasicsJCTheme {
Scaffold(modifier = Modifier.fillMaxSize()) { innerPadding ->
BoxLayout(innerPadding)
}
}
}
}
}
@Composable
fun BoxLayout (innerPadding: androidx.compose.foundation.layout.PaddingValues) {
// Alignment Options:
// Alignment.TopStart, Alignment.TopCenter, Alignment.TopEnd
// Alignment.CenterStart, Alignment.Center, Alignment.CenterEnd
// Alignment.BottomStart, Alignment.BottomCenter, Alignment.BottomEnd
Box( modifier = Modifier.padding(innerPadding)
.fillMaxSize()
.background(Color.Yellow)
) {
Text(text = "Item 1", modifier = Modifier.align(Alignment.TopStart).background(Color.Green).width(50.dp).height(150.dp))
Text(text = "Item 2", modifier = Modifier.align(Alignment.Center).background(Color.Cyan).width(50.dp).height(150.dp))
Text(text = "item 3", modifier = Modifier.align(Alignment.BottomEnd).background(Color.Magenta).width(50.dp).height(150.dp))
}
}
This Jetpack Compose code demonstrates the use of the Box layout with different alignment options to position child elements. Inside the Box, which fills the entire screen and has a yellow background, three Text components are placed using Modifier.align() to show alignment behavior. The first item is aligned to the top-start corner, the second is perfectly centered, and the third is aligned to the bottom-end corner. Each item is given a specific size and background color for clear visual distinction. This example effectively illustrates how Box allows overlapping or independently aligned elements within the same container.
All options for alignment in box layout are shown below
After words
Understanding arrangement and alignment in Jetpack Compose is essential for creating well-structured and visually appealing user interfaces. Arrangement controls how multiple items are distributed along the main axis of layouts like Row and Column, enabling flexible spacing strategies such as centered, evenly spaced, or spaced by a fixed distance. Alignment, on the other hand, positions individual elements along the cross axis or within containers like Box, allowing precise control over where content appears. By mastering these concepts and their corresponding parameters—like horizontalArrangement, verticalAlignment, and contentAlignment—developers can design dynamic, responsive, and readable layouts with ease. Whether you're working with rows, columns, or overlapping layers in a box, the clear understanding of how items align and arrange ensures a consistent and polished UI across different screen sizes and orientations.
Happy Coding!!!
Subscribe to my newsletter
Read articles from Jyoti Maurya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
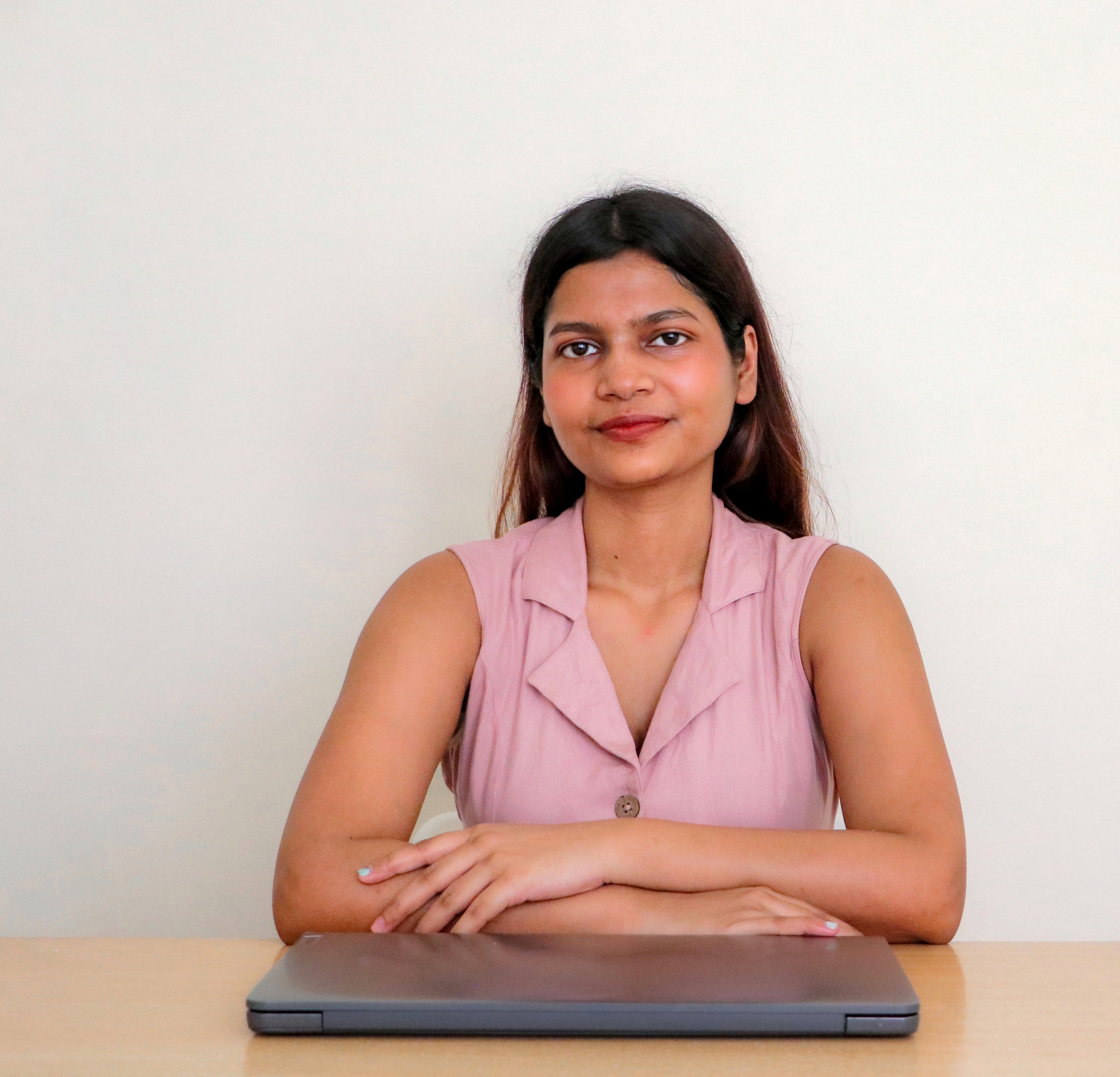
Jyoti Maurya
Jyoti Maurya
I create cross platform mobile apps with AI functionalities. Currently a PhD Scholar at Indira Gandhi Delhi Technical University for Women, Delhi. M.Tech in Artificial Intelligence (AI).