How to Create Custom WordPress Blocks
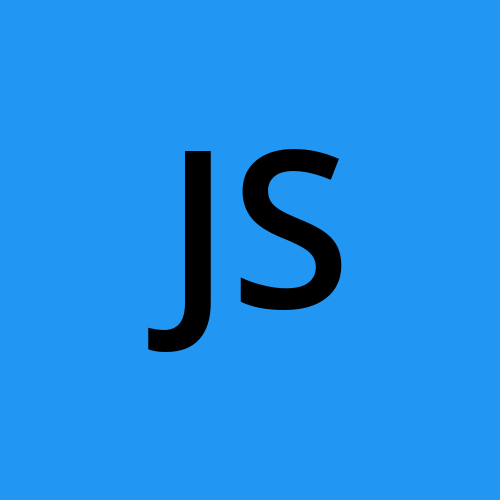
The WordPress block editor, also known as Gutenberg, has transformed content creation by introducing a modular block-based editing experience. While WordPress offers a growing library of core blocks, there are times when you need more flexibility or want to build something tailor-made for your website or client. That’s where custom WordPress blocks come into play.
In this blog, we’ll walk you through how to create custom WordPress blocks from scratch using modern tools like @wordpress/scripts, React, and the Block API. Whether you want to add dynamic content, branding components, or interactive elements, this guide has got you covered.
What is a WordPress Block?
A block is a reusable unit of content in the WordPress editor—such as a paragraph, image, button, or gallery. Blocks are built using JavaScript (mostly React) and registered with PHP. Custom blocks let you define your own functionality and design to extend Gutenberg beyond the default blocks.
Why Create Custom Blocks?
Here’s why you might need to build a custom block:
- Unique functionality: For example, a pricing table, testimonial slider, or product feature block.
- Client branding: Delivering consistent styling and user experience across the editor.
- Reusable elements: Empower content teams to insert complex layouts without touching code.
- Enhanced UX: Provide a better editor interface tailored to your specific use cases.
Prerequisites
To follow along, ensure you have the following:
- A local WordPress development environment (e.g., using Local, MAMP, or DevKinsta)
- Node.js and npm installed
- Familiarity with React and JavaScript ESNext
- Basic understanding of WordPress themes/plugins
Step-by-Step: Creating a Custom WordPress Block
Let’s build a simple custom "Call to Action" block.
1. Set Up a Custom Plugin
First, create a new folder in wp-content/plugins/ called custom-blocks. Inside, create a file named custom-blocks.php.
<?php
/**
* Plugin Name: Custom Blocks
* Description: A plugin to register custom Gutenberg blocks.
* Version: 1.0
*/
defined( 'ABSPATH' ) || exit;
function custom_blocks_register() {
// Automatically loads block files from build/index.js
wp_register_script(
'custom-blocks-editor-script',
plugins_url( 'build/index.js', FILE ),
array( 'wp-blocks', 'wp-element', 'wp-editor', 'wp-components' ),
filemtime( plugin_dir_path( FILE ) . 'build/index.js' )
);
register_block_type( 'custom/cta-block', array(
'editor_script' => 'custom-blocks-editor-script',
));
}
add_action( 'init', 'custom_blocks_register' );
2. Initialize the Block with @wordpress/scripts
Navigate into your plugin directory and initialize the block setup using:
npx @wordpress/create-block cta-block
This scaffolds your block with a default structure and all dependencies pre-configured.
It will create a cta-block folder with files like:
- block.json
- edit.js
- save.js
- style.scss
You can now edit your block functionality inside edit.js and save.js.
3. Customize the Block (Example: Call to Action)
Let’s update the editor interface to add a title and a button.
edit.js:
import { __ } from '@wordpress/i18n';
import { useBlockProps, RichText } from '@wordpress/block-editor';
export default function Edit({ attributes, setAttributes }) {
const { title, buttonText } = attributes;
return (
<div {...useBlockProps()}>
<RichText
tagName="h2"
value={title}
onChange={(value) => setAttributes({ title: value })}
placeholder={__('Add CTA title…', 'cta-block')}
/>
<RichText
tagName="button"
value={buttonText}
onChange={(value) => setAttributes({ buttonText: value })}
placeholder={__('Click Me', 'cta-block')}
/>
</div>
);
}
save.js:
import { useBlockProps, RichText } from '@wordpress/block-editor';
export default function save({ attributes }) {
const { title, buttonText } = attributes;
return (
<div {...useBlockProps.save()}>
<RichText.Content tagName="h2" value={title} />
<RichText.Content tagName="button" value={buttonText} />
</div>
);
}
block.json (simplified):
{
"apiVersion": 2,
"name": "custom/cta-block",
"title": "Call to Action",
"category": "widgets",
"icon": "megaphone",
"attributes": {
"title": { "type": "string" },
"buttonText": { "type": "string" }
},
"editorScript": "file:./index.js"
}
Run npm run build to compile your JavaScript files. Activate the plugin, and you’ll see your custom block in the editor!
Tips and Best Practices
- Use @wordpress/scripts to simplify build tools (webpack, Babel).
- Keep blocks modular by separating concerns (edit/save/styles).
- Use InnerBlocks for nested content like accordions or tabs.
- Add supports in block.json to define custom block behaviors.
- Test responsiveness and accessibility early in development.
- Register block styles using wp_enqueue_style.
Advanced Features to Explore
Once you're comfortable with the basics, explore:
- Dynamic blocks with PHP rendering
- Server-side attributes (great for query blocks)
- Inspector controls (Sidebar UI)
- Reusable components from @wordpress/components
- Custom block styles and variations
Final Thoughts
Creating custom WordPress blocks gives developers the power to tailor content creation exactly to their needs. With Gutenberg's robust APIs and modern JavaScript support, you can build rich, intuitive editing experiences that save time and boost consistency across your website or product.
Whether you're a freelancer, agency, or business owner looking to streamline content management, investing in custom blocks is a future-proof move.
Subscribe to my newsletter
Read articles from John Smith directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
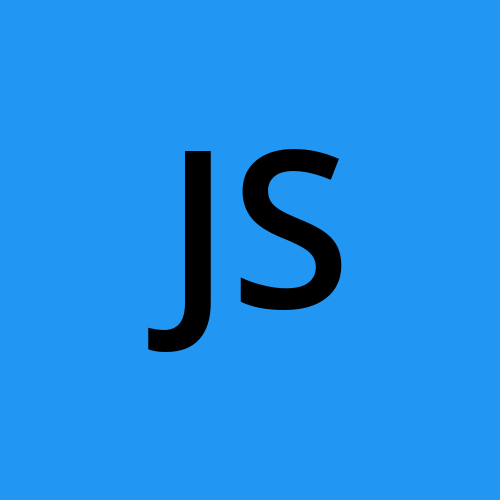