π Creating a Custom Button in Oracle APEX Interactive Grid (IG) to Trigger a Multi-Row Action
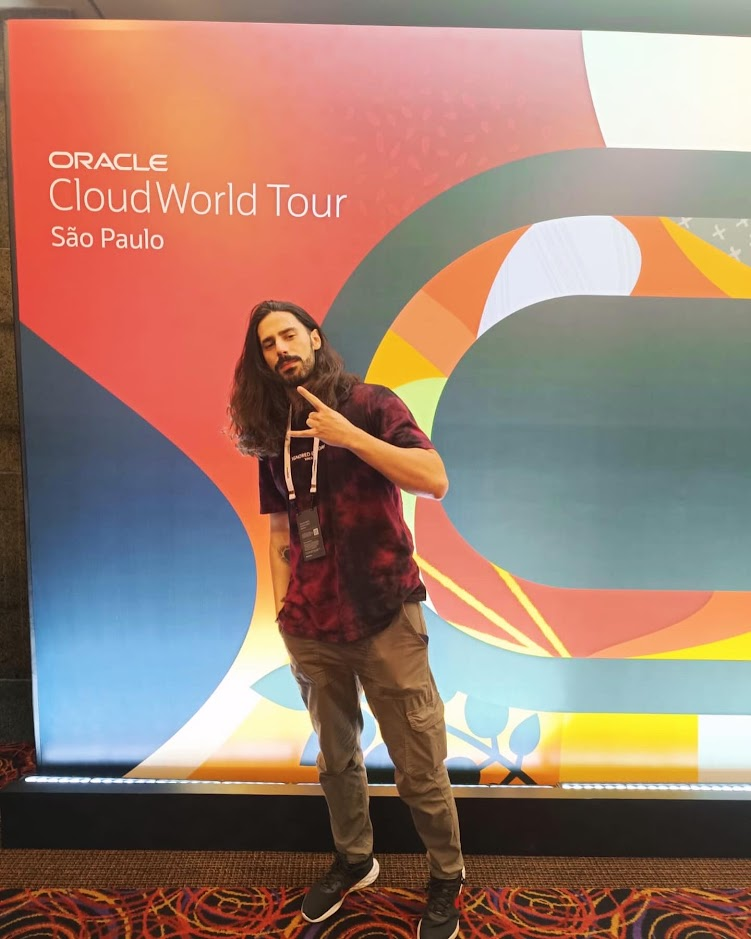
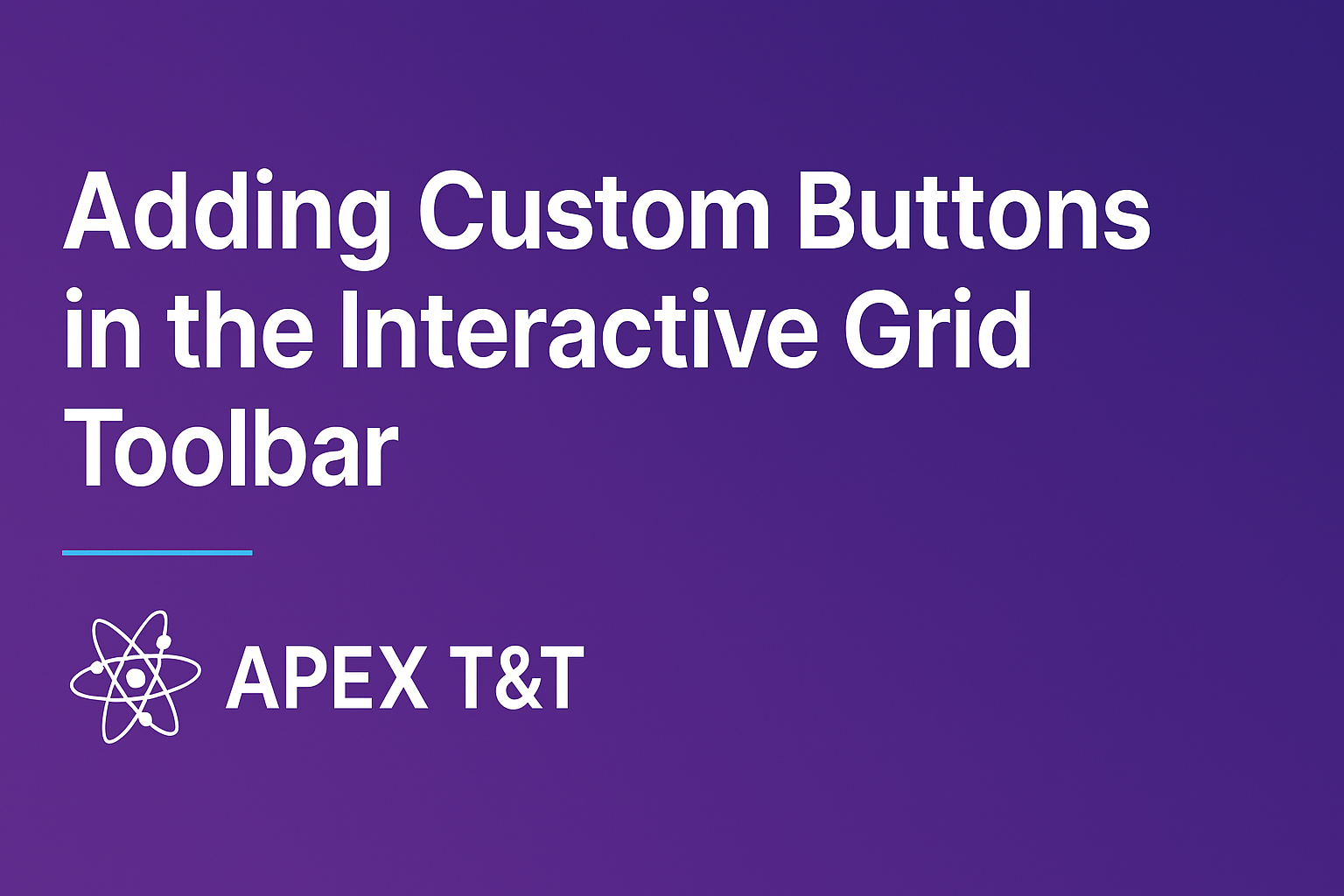
Introduction
Oracle APEX's Interactive Grid (IG) is powerful β but sometimes we need more than the default buttons. A common use case is to allow users to select one or more rows and then perform a custom action using a button.
In this article, we'll walk through how to add a custom button to the Interactive Grid toolbar that triggers a JavaScript function on click β allowing you to get creative and perform any kind of logic you want.
β The Use Case
On my page, I have an Interactive Grid showing a list of countries. The user can select one or more rows and click a button labeled "Search Employees". This button triggers a custom JavaScript function that collects the selected countries, sets a page item, and submits the page to redirect to another report filtering employees based on those countries.
This example may sound silly, but the technique youβll learn is super useful for many real scenarios!
π§ Step 1 β Add a Custom Button to the Grid Toolbar
You can add a new button during the Interactive Grid initialization phase using JavaScript. Here's the code to place in:
Region Attributes β Advanced β Initialization JavaScript Function
function(config){
const toolbarData = $.apex.interactiveGrid.copyDefaultToolbar();
let toolbarGroupExpand = toolbarData.toolbarFind("actions3");
toolbarGroupExpand.controls.push({
type: "BUTTON",
action: "getEmployees",
icon: 'fa fa-user-plus',
iconBeforeLabel: true,
label: 'Search Employees',
id: "btn-get-employees"
});
config.initActions = function(actions) {
actions.add([
{
name: "getEmployees", //must be the same name of action control
action: function(event, focusElement) {
getEmployees();
}
}
]);
};
config.toolbarData = toolbarData;
return config;
}
This snippet:
Clones the default IG toolbar
Adds a new button to the
actions3
groupRegisters a custom action named
getEmployees
linked to the function of the same name
π‘ Tip:
actions3
,actions2
, etc., are toolbar groups that APEX provides by default. If you want to place your button next to another, find the group and push your button into its array.
π§ Step 2 β JavaScript Function to Process Selection
In Page Properties β Function and Global Variable Declaration
Here's the JavaScript function that will be called when the button is clicked:
function getEmployees(){
const regionStaticId = 'countriesIG';
let selectedCountries = [];
// Get selected records from IG
var records = apex.region(regionStaticId)
.widget()
.interactiveGrid("getCurrentView")
.model
.getSelectedRecords();
// Extract country ID (assuming it's at position 0)
records.forEach(function(record) {
var countryName = record[0];
if (countryName) {
selectedCountries.push(countryName);
}
});
// Set the selected values in a hidden item, separated by ':'
apex.item("P14_SELECTED_VALUES").setValue(selectedCountries.join(':'));
// Submit the page to trigger branching
apex.page.submit();
}
π‘ Tip: Make sure your region has a Static ID, e.g.,
countriesIG
.
This function:
Retrieves all selected records from the IG
Collects country names (column at index
0
, adapt as needed)Sets them in a hidden item
P14_SELECTED_VALUES
Submits the page
π Step 3 β Branching to Another Page
After the page is submitted, we can redirect to another page using a branch. Here's how:
Create a hidden item:
P14_SELECTED_VALUES
Create a branch to Page 2
In the branch settings:
Set Items:
Name: P2_COUNTRIES
Value: &P14_SELECTED_VALUES.
Clear Cache:
2,14
(if needed)
This ensures the selected countries are passed to Page 2, where a report or logic can filter based on those values.
β Final Result
Once everything is in place:
The IG will show a "Search Employees" button
You can select one or more countries
On click, the selected values are submitted
Page 2 receives the values in
P2_COUNTRIES
This approach is scalable and user-friendly, especially when will need to perform several actions in multiples rows
π§© Conclusion
Customizing the Interactive Grid toolbar can significantly enhance the user experience in Oracle APEX. With this technique, you have full control of the interface and can build powerful dynamic actions with selected data β all without any plugins. π
π¬ Let me know if you'd like a version that works with modal dialogs or AJAX callbacks.
π And donβt forget to like this article if it helped you!
Subscribe to my newsletter
Read articles from Bruno Cardoso directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
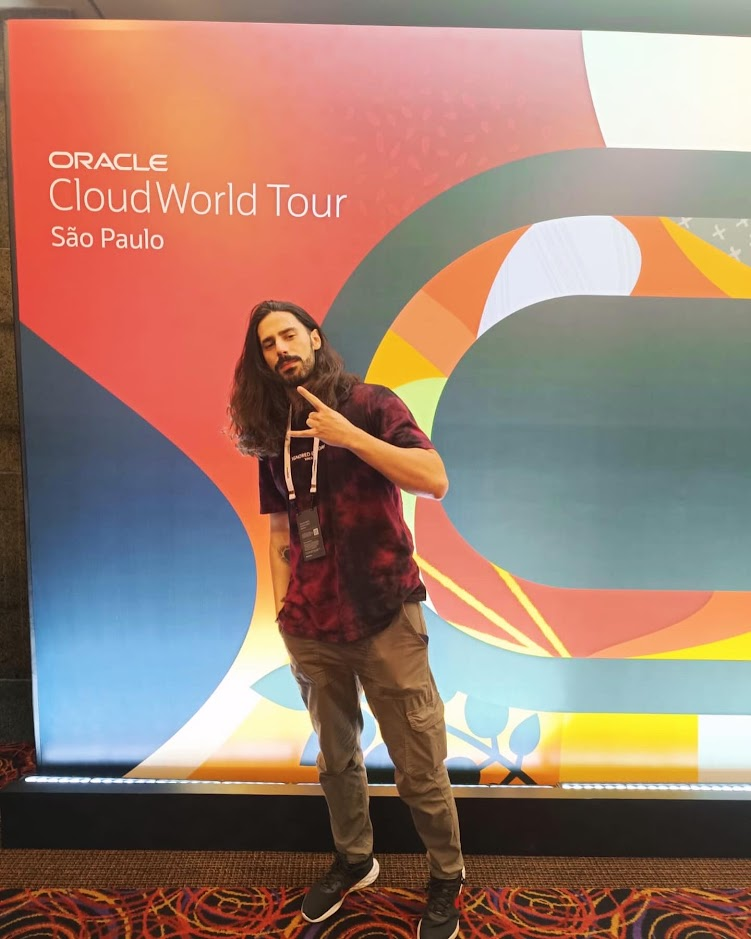
Bruno Cardoso
Bruno Cardoso
I'm a APEX Senior developer who likes write down what I discover to keep a part of my mind stored and share my knowing with others.