Chapter 2: HTML (Level 1) โ Part B
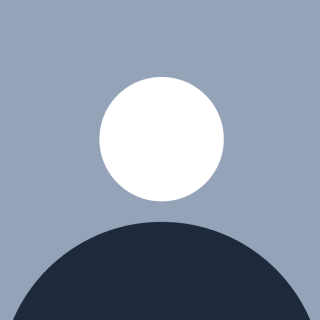
Table of contents
- 1. Lists in HTML
- Unordered List Example
- Ordered List Example
- Ordered List with Type Specification (Alphabetical)
- Nested List Example
- Description List Example
- 2. Attributes in HTML
- ๐ Theory
- ๐ก Technical Terms:
- ๐ Basic Interview Questions:
- ๐ป Small Project: Build a Simple HTML Form with Various Input Attributes
- ๐ Best Practices for Using Attributes Efficiently
- โ Conclusion:
- 3. Anchor Element
- 4. Image Element
- 5. Practice Question
- 6. More HTML Tags
- 7. Comments in HTML
- 8. Is HTML Case Sensitive?
- 9. Practice Question
- 10. Assignment Questions
From the Delta Batch (Web Development) by Shradha Khapra Didi, Apna College
Welcome to Chapter 2: HTML (Level 1) โ Part B in our journey of web development! This chapter is designed to build upon the foundational concepts of HTML (HyperText Markup Language) that youโve learned so far. By the end of this section, youโll have a deeper understanding of how HTML structures web pages and makes them interactive and visually appealing.
In this chapter, weโll explore important elements and features of HTML that are essential for creating user-friendly web pages. Here's what weโll cover:
1. Lists in HTML
In this section, we will cover how to create and use lists in HTML to structure web content efficiently. Lists help organize information in a meaningful way, making it easier for users to consume content.
๐ Theory:
HTML provides three types of lists:
Ordered List (
<ol>
): Displays list items in a specific sequence or order, typically with numbers.Unordered List (
<ul>
): Displays list items in no particular order, often with bullet points.Description List (
<dl>
): Used for pairs of terms and their corresponding descriptions.
Each list uses list items (<li>
) to define individual elements.
๐ก Technical Terms:
List Items: Individual elements in a list, defined using the
<li>
tag.Nested Lists: A list inside another list, used for hierarchical data representation.
Types of List Markers: Different styles such as numbers, alphabets, Roman numerals, and bullet points.
๐ Basic Interview Questions:
What are the different types of lists in HTML?
Answer: HTML provides ordered lists (<ol>
), unordered lists (<ul>
), and description lists (<dl>
).Can lists be nested in HTML? Explain with an example.
Answer: Yes, lists can be nested by placing one list inside a list item (<li>
) of another list.
๐ป Small Project:
Create a simple navigation menu using nested lists to represent categories and subcategories.
๐ง Complete HTML Code Examples:
Unordered List Example
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Unordered List Example</title>
</head>
<body>
<h1>Unordered List Example</h1>
<ul>
<li>Rohit</li>
<li>Shikhar</li>
<li>Virat</li>
<li><b>Shreyash</b></li>
</ul>
</body>
</html>
Ordered List Example
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Ordered List Example</title>
</head>
<body>
<h1>Ordered List Example</h1>
<ol>
<li>Rohit</li>
<li>Shikhar</li>
<li>Virat</li>
</ol>
</body>
</html>
Ordered List with Type Specification (Alphabetical)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Ordered List Type Example</title>
</head>
<body>
<h1>Ordered List with Type "A"</h1>
<ol type="A">
<li>Rohit</li>
<li>Shikhar</li>
<li>Virat</li>
</ol>
</body>
</html>
Nested List Example
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Nested List Example</title>
</head>
<body>
<h1>Nested List Example</h1>
<ul>
<li>Rohit</li>
<li>Shikhar
<ul>
<li>Test Match</li>
<li>ODI</li>
<li>T20</li>
</ul>
</li>
<li>Virat</li>
</ul>
</body>
</html>
Description List Example
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Description List Example</title>
</head>
<body>
<h1>Description List Example</h1>
<dl>
<dt>HTML</dt>
<dd>A markup language for creating web pages.</dd>
<dt>CSS</dt>
<dd>A stylesheet language used for describing the presentation of a document written in HTML.</dd>
</dl>
</body>
</html>
๐ Conclusion:
Lists in HTML play a crucial role in organizing content effectively. They enhance readability and provide a structured way to present information. Mastering lists will make your web content more user-friendly and visually appealing.
2. Attributes in HTML
Attributes are essential for defining additional information or properties for HTML elements, helping developers customize and control the behavior of web elements. They are placed inside the opening tag of an element and typically consist of a name-value pair.
๐ Theory
An attribute provides extra information about an element beyond its basic structure. Attributes can be used to:
Set identifiers or class names for styling (
id
,class
)Control input behaviors (
placeholder
,maxlength
,readonly
)Improve accessibility (
alt
,aria-*
)Specify language or metadata information (
lang
,charset
)
Attributes always have the format:
<element_name attribute_name="value">Content</element_name>
Example:
<html lang="en">
In this example, the lang="en"
attribute specifies that the language of the HTML document is English.
๐ก Technical Terms:
Attributes: Name-value pairs that provide additional details about an element's behavior or properties.
Global Attributes: Attributes that can be applied to any HTML element (
id
,class
,style
,title
).Event Attributes: Attributes that handle events, such as
onclick
andonmouseover
.
๐ Basic Interview Questions:
What are global attributes in HTML?
Answer: Global attributes are attributes that can be applied to any HTML element. Examples include:id
: Unique identifier for an element.class
: Class name for CSS styling.style
: Inline CSS styling.title
: Tooltip text that appears when the mouse hovers over an element.
What is the purpose of the
lang
attribute in HTML?
Answer: Thelang
attribute specifies the language of the document's content, helping search engines and assistive technologies understand the language context.
๐ป Small Project: Build a Simple HTML Form with Various Input Attributes
In this project, we will create a simple HTML form using various input attributes to control user input behavior.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>HTML Form with Attributes</title>
</head>
<body>
<h1>Simple HTML Form Example</h1>
<form action="#" method="POST">
<!-- Text Input with Placeholder and Maxlength -->
<label for="name">Name:</label>
<input type="text" id="name" name="name" placeholder="Enter your name" maxlength="20" required>
<br><br>
<!-- Email Input -->
<label for="email">Email:</label>
<input type="email" id="email" name="email" placeholder="Enter a valid email" required>
<br><br>
<!-- Password Input with Maxlength -->
<label for="password">Password:</label>
<input type="password" id="password" name="password" maxlength="10" placeholder="Create a password">
<br><br>
<!-- Number Input -->
<label for="age">Age:</label>
<input type="number" id="age" name="age" min="1" max="100">
<br><br>
<!-- Submit Button -->
<button type="submit">Submit</button>
</form>
</body>
</html>
Explanation:
placeholder
: Provides hint text inside the input field.maxlength
: Restricts the number of characters a user can input.required
: Ensures that the user must fill out the field before submitting the form.min
andmax
: Define the valid range for number input fields.
๐ Best Practices for Using Attributes Efficiently
Always use meaningful attribute values:
Ensureid
,class
, andalt
values are descriptive and informative.Leverage global attributes:
Usetitle
,lang
, anddata-*
attributes to provide additional information and context.Avoid inline styles:
Prefer using external or internal CSS for styling instead of thestyle
attribute.Follow accessibility guidelines:
Use attributes likearia-label
andalt
to improve web accessibility.
โ Conclusion:
Attributes in HTML play a crucial role in defining how elements behave and appear on a webpage. By understanding and applying them efficiently, developers can create well-structured, user-friendly, and accessible web applications.
3. Anchor Element
The <a>
tag, also known as the anchor element, is used to create hyperlinks.
Internal Links: Navigate within the same website.
External Links: Redirect to another website.
Email Links: Open email applications.
Example:
<a href="https://www.google.com" target="_blank">Visit Google</a>
<a href="#section2">Jump to Section 2</a>
<a href="mailto:example@example.com">Send Email</a>
Youโll also learn about the target
attribute to control where the link opens (_self
, _blank
, etc.).
4. Image Element
The <img>
tag is used to display images.
Key attributes include
src
(source of the image),alt
(alternative text for accessibility), andwidth/height
(dimensions).Learn the difference between relative and absolute paths for image sources.
Example:
<img src="images/logo.png" alt="Website Logo" width="200" height="100">
Bonus topics include:
Handling broken images.
Using responsive images with modern HTML techniques.
5. Practice Question
To reinforce your learning, youโll solve practice problems such as:
Creating a webpage with a list of favorite movies using both ordered and unordered lists.
Adding hyperlinks and images to make the page interactive.
Applying attributes to style and organize content.
6. More HTML Tags
This section introduces additional HTML tags to enrich your web pages.
<hr>
: Adds a horizontal line to separate content.<br>
: Inserts a line break for better readability.<sup>
and<sub>
: Formats text as superscript and subscript.
Example:
<p>Water is H<sub>2</sub>O.</p>
<p>E = mc<sup>2</sup></p>
7. Comments in HTML
Comments are used to add notes or temporarily disable code without affecting the pageโs output.
Syntax:
<!-- This is a comment -->
Comments are essential for documenting code and improving collaboration.
Example:
<!-- This section contains the navigation bar -->
<nav>
<a href="#home">Home</a>
<a href="#about">About</a>
</nav>
8. Is HTML Case Sensitive?
HTML is not case-sensitive, meaning <body>
and <BODY>
are treated the same. However, adhering to lowercase for elements and attributes is a best practice to maintain readability and compatibility.
Example:
<!-- Both are valid -->
<HTML>
<BODY>
<h1>Welcome!</h1>
</BODY>
</HTML>
This section also covers case sensitivity in file paths and its importance in web development.
9. Practice Question
Test your knowledge with scenarios like:
Writing clean and readable code using comments and best practices.
Exploring case sensitivity through real-world examples, such as file path errors.
10. Assignment Questions
Apply everything youโve learned in this chapter with real-world tasks:
Create a personal profile webpage with lists, links, and images.
Use attributes to style the page and make it interactive.
Add comments to document your code.
This chapter is designed to be practical and engaging, with plenty of examples and exercises to strengthen your understanding of HTML concepts. By the end, youโll be well-equipped to create structured, interactive, and visually rich web pagesโsetting a solid foundation for more advanced topics in web development.
Letโs dive in and start building! ๐
Subscribe to my newsletter
Read articles from Rohit Gawande directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Rohit Gawande
Rohit Gawande
๐ Tech Enthusiast | Full Stack Developer | System Design Explorer ๐ป Passionate About Building Scalable Solutions and Sharing Knowledge Hi, Iโm Rohit Gawande! ๐I am a Full Stack Java Developer with a deep interest in System Design, Data Structures & Algorithms, and building modern web applications. My goal is to empower developers with practical knowledge, best practices, and insights from real-world experiences. What Iโm Currently Doing ๐น Writing an in-depth System Design Series to help developers master complex design concepts.๐น Sharing insights and projects from my journey in Full Stack Java Development, DSA in Java (Alpha Plus Course), and Full Stack Web Development.๐น Exploring advanced Java concepts and modern web technologies. What You Can Expect Here โจ Detailed technical blogs with examples, diagrams, and real-world use cases.โจ Practical guides on Java, System Design, and Full Stack Development.โจ Community-driven discussions to learn and grow together. Letโs Connect! ๐ GitHub โ Explore my projects and contributions.๐ผ LinkedIn โ Connect for opportunities and collaborations.๐ LeetCode โ Check out my problem-solving journey. ๐ก "Learning is a journey, not a destination. Letโs grow together!" Feel free to customize or add more based on your preferences! ๐