Understanding 10 Javascript Array methods as a beginner with Manoj, the Farmer 🧑‍🌾

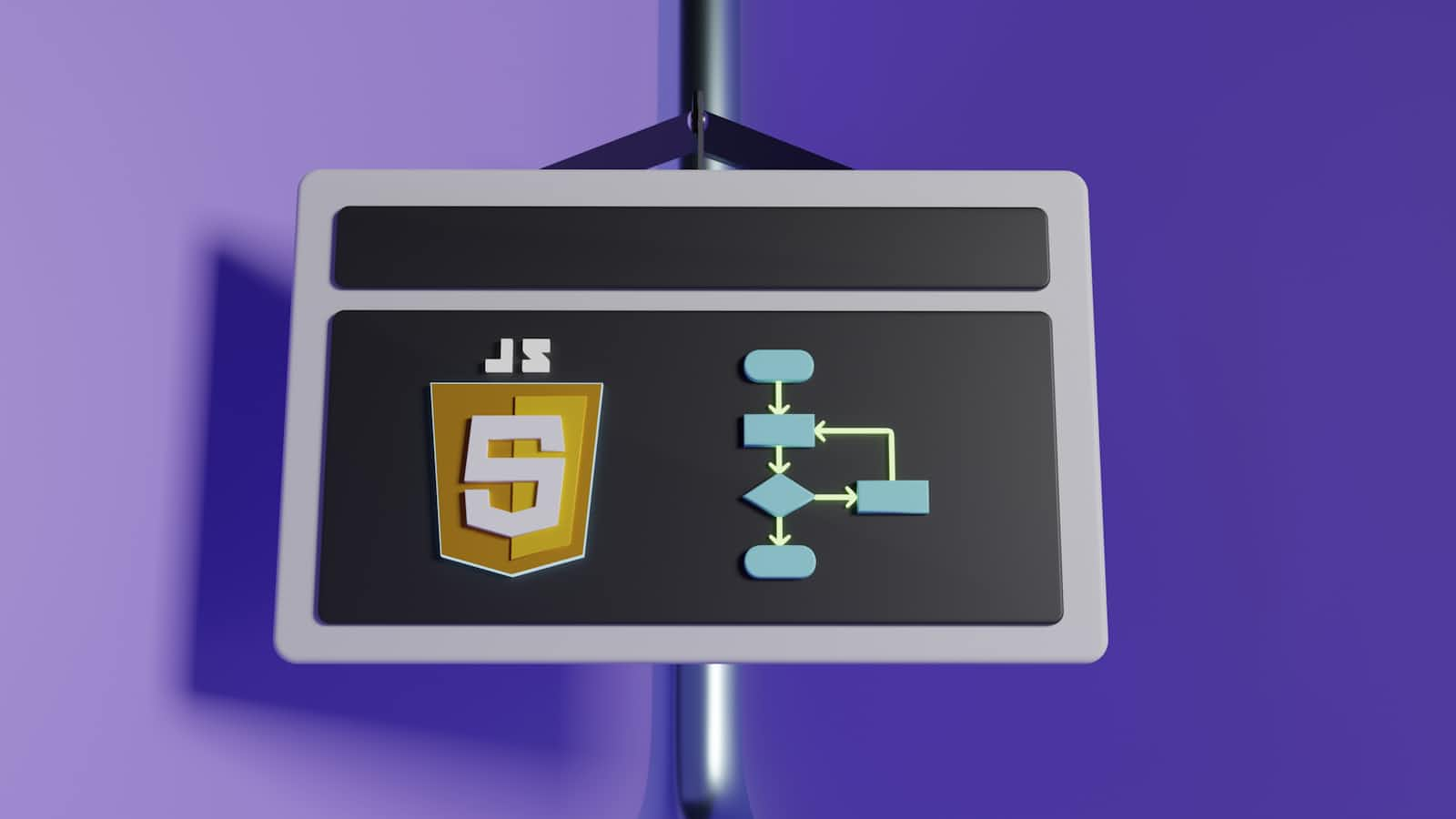
As a Beginner, Javascript can get a bit overwhelming sometimes so as I begin my learning journey on JS, let’s decode some beginner friendly array methods in a fun way 🌿
Introduction
Now as Manoj has to present his products , he has thought of presenting it in a rack. In that rack each level will contain a single fruit of different category.
1) Push Method
For Manoj to successfully implement the first step , he needs to put his desired fruits inside each level of the rack which can be achived by the following :
rack.push("Bananna");// syntax: array_name.push( Entity that you want to insert);
In brief : Push() method pushes element from the back of the array.
2) Pop Method
Manoj: This rack is becoming very heavy to carry on so I will have to remove a fruit from the last of the rack. So I will have to implement the following code to remove the last fruit from the rack so that the size of the rack decreases and the rack feels less heavy .
Therefore for decreasing the weight Manoj implemnts the following method :
rack.pop(); // last element gets removed from the array and array reduces in size
In brief: pop() removes the last elemnent of the array and return it. after removal the memory block where the last elemnt contained is fred.
3) Every method
Manoj : Before showing my fruits to my client for precaution let me check whether all of them are in a good state or not. If anything is rotten then I will remove it !
So for achieving this manoj implements the every() method in the following manner :
//example / pseudo code
const rack = ["Bananna", "Mango", "Strawberry", "Watermelon"];
//function of isRotten
const isRotten = (rack) => condition ; //pseudo code for understanding donot implement it
//every method checks if every value returned is true or not else returns false
console.log(rack.every(isRotten)); // returns true or false
In brief : every() method just checks whether all the array elements satisfy with the given criteria or not , if not then returns false. If we donot use this function then we will have to check using if else , but this method makes the working easy for the coder in one line.
4) findIndex method
Manoj: as I have found that there is a fruit rotten (since every() gave false value), hence I want to change that fruit with another fruit of the same category, for that at first I need to find in which level of the rack the rotten fruit is kept , for that i will use findIndex method. findIndex() returns the first index of the element that satisfies with the condition. Let’s implement it in the following:
// pseudocode
const rack = ["Bananna", "Mango", "Strawberry", "Watermelon"];
const isRotten=(rack)=> condition of being rotten;
console.log(rack.findIndex(isRotten)); // expected output is 3
In brief: findIndex() returns the first index of the element that satisfies the condition. If no elements satisfy the testing function, -1 is returned.
5) Filter method
Manoj : Now as I have found which fruit is rotten then let’s filter (remove) that fruit out of the rack and display them in a clean rack , so that client isn’t displeased. Let’s implement the filter method below :
//considering x is the index that we got in the above findIndex() method
//rack[x] being the element which is rotten , so the rotten elemnt will be filtered out and other gruits satisfying the condition will be copied into a new array
let rack1=rack.filter((fruits)=>(fruits)!=rack[x]); // rack1=[bananna,mango,strawberry]
console.log(rack1);
In brief : if we want to remove a specific array and want afresh shallow copy of the original array without including the elemnt that you want to remove , then use this method.
6) Splice method
Manoj : Manoj delivers it to his client thinking for his feedback.
Client : Manoj, Can you diversify the range of fruits you have sent ? I feel like I should check more categories of fruits to choose the best out of all for my family function.
Manoj : Ok sir ! Then let me add more catgories of fruits into the rack , and then you shall choose as it suits you .
Manoj now will use the splice method which is capable of removing or replacing existing elements and/or adding new elements . Let’s implement and understand it through below explanation :
console.log(rack1.splice(2,0,"Grapes")); // returns rack1 =["Bananna","Mango","Grapes","Strawberry"]
In brief: splice() is used for inserting any elemnt at a certain index , also for removing elemnt at certain index.
7) Includes method
Manoj : For precaution as it’s his client’s order hence let’s recheck whether I have added grapes in the rack or not. If I have added then includes() method will return true else false. Implemntation and code below :
console.log(rack1.includes("Grapes")); // expected output: true
In brief : If we want to check whether the target element is prsesnt in the self declared array.
8) Sort method
Client : I have liked all fruits that you ahve sent . So these are the final ones , I will inform you soon about the quantity Required.
Manoj : Ok so as everything has been completed let me sort all the fruits and keep it again in the rack in sorted manner. For that I will need sort() function :
rack1.sort();
console.log(rack1); //expected output=["Banana","Grapes","Mango","Strawberry"]
9) forEach method
Manoj now wants to make a list of the fruits that he has sent to the client and has been approved , so that he can maintain that in his records for future purposes. Due to that he needs to implement forEach() .
forEach is nothing but a for loop alterantive where the traditional way of checking condition for every array element using for loop is optimized. forEach() executes a provided function once for each array element automatically when it’s used
rack1.forEach((fruits)=> console.log(fruits));
//fruits here referes to a single element of the rack1 array.
/* for Each just performs the function given inside parenthesis for each and every elemnt of the array.
here the function is that we have to print the fruit variable's value. Fruit here refers to the
array element. Hence all the elemnts will be printed*/
//expected output =["Bananna","Grapes","Mango","Strawberry"]
10) indexOf method
Manoj : As I now have the list of all items , let me also record each level where I have stored which fruit. I will have to implement indexOf method that will exactly tell me in which position which fruit is stored ! So let’s see the implementation
Conclusion
Though the story may appear lame/illogical , but I hope some of the scenarios was helpful to any beginner reading this in clearing their concept.
Subscribe to my newsletter
Read articles from Snigdha Datta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Snigdha Datta
Snigdha Datta
EAT , SLEEP , EDIT , CODE and REPEAT