Common Git Mistakes Developers Make and How to Overcome Them

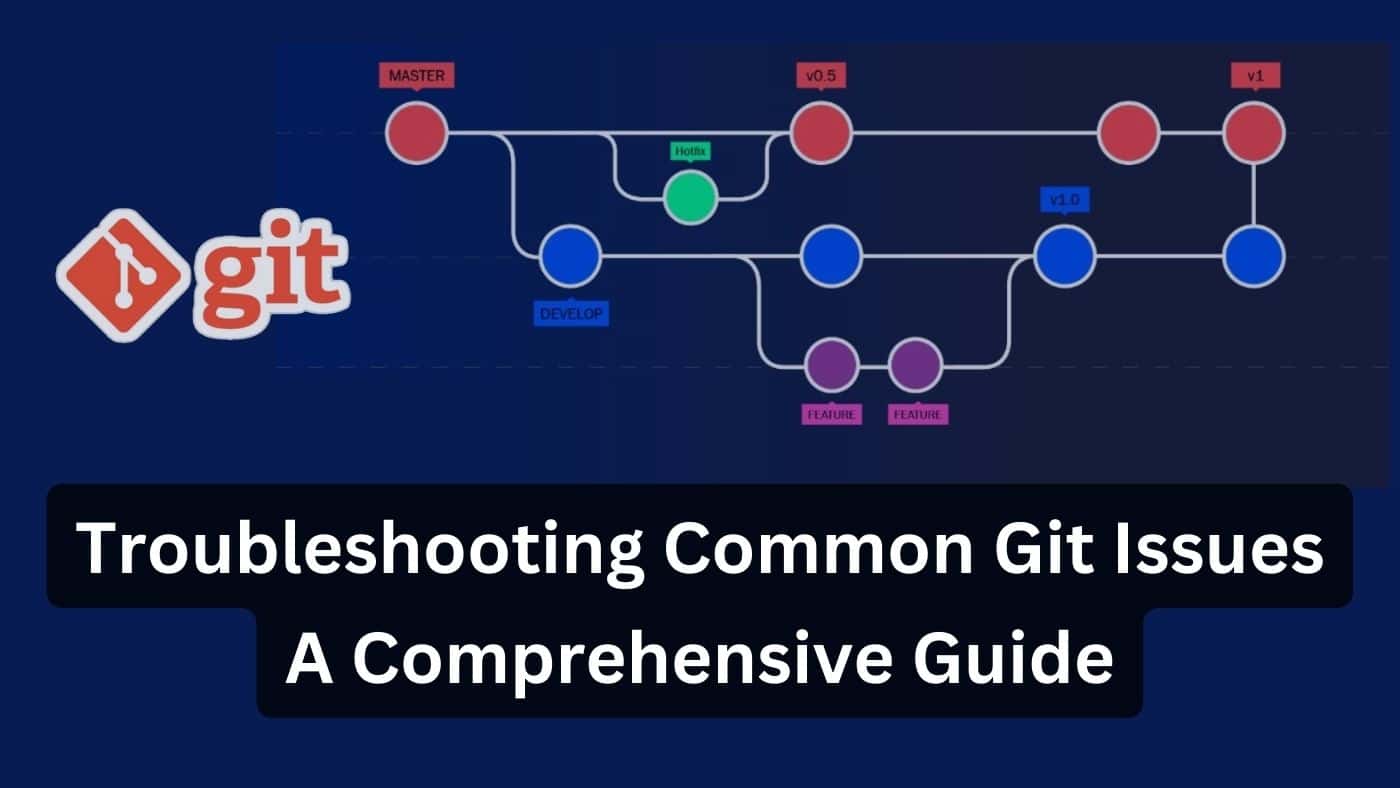
Git is an essential tool for version control in software development. However, many developers—especially beginners—make common mistakes that can lead to frustrating issues, wasted time, and sometimes even lost code. In this article, I will walk you through the most common Git mistakes, how to avoid them, and some personal experiences from my own development journey that taught me the importance of mastering Git.
1. Committing Sensitive Information
The Mistake:
One of the most common Git mistakes developers make is committing sensitive information (like API keys, passwords, and tokens) to the repository. This can lead to security vulnerabilities, especially if the repository is public.
Why This Happens:
In the heat of development, developers may forget to exclude sensitive files from Git. When working with API integrations or configuring development environments, secrets often slip into commits, even if it's unintentional.
How I Overcame This:
I once committed my Firebase credentials directly to the repo without realizing it. Fortunately, I noticed the mistake early and removed them using git filter-branch
to clean the commit history. From that point onwards, I made sure to use .gitignore
to exclude sensitive files and set up environment variables instead of hardcoding sensitive data.
How to Avoid It:
Use
.gitignore
to exclude files like*.env.secret
, or any other file containing credentials.# .gitignore *.env .secret
Store sensitive information in environment variables or use secret management tools.
If sensitive data has been committed, use tools like BFG Repo-Cleaner or
git filter-branch
remove it from the entire repository history.git filter-branch --force --index-filter \ "git rm --cached --ignore-unmatch path/to/sensitive-file" \ --prune-empty --tag-name-filter cat -- --all
2. Not Using Meaningful Commit Messages
The Mistake:
Another common mistake is using vague commit messages like "Fix" or "Update" without providing enough context. This can make it difficult to understand the history of changes, especially when collaborating with a team.
Why This Happens:
Some developers may feel rushed or don’t realise how crucial commit messages are for the development process. As a result, they opt for generic messages that don’t describe the changes clearly.
How I Overcame This:
When I started working in larger teams, I quickly realised that vague commit messages led to confusion. After receiving feedback, I began to write detailed commit messages that explained what and why I made the changes, rather than just how. For example:
Bad:
Fix bug
Good:
Fix login issue by updating authentication flow
How to Avoid It:
Follow the Conventional Commits style (e.g.,
feat
,fix
,chore
), which is widely used for better clarity.git commit -m "feat(auth): add Google login functionality"
Write commit messages that describe why you made the change, not just what was changed.
Start your commit messages with a short, concise summary, followed by a more detailed explanation if necessary.
3. Committing Directly to Master/Main
The Mistake:
Committing directly to the master
main
branch can create chaos, especially when working in a team. This bypasses the code review process and may lead to introducing bugs directly into production code.
Why This Happens:
New developers or those working alone may not see the need for branching. They think committing directly to the main branch is quicker and easier, but it can lead to unreviewed changes in the main codebase.
How I Overcame This:
Early on, I made this mistake, and it caused issues when I pushed changes that broke the app without getting feedback. After realizing the importance of creating feature branches, I switched to a branching strategy. Now, I create a new branch for each new feature or bug fix.
git checkout -b feature/new-login
How to Avoid It:
Always create a feature branch before making any changes.
git checkout -b feature/feature-name
Use a branching strategy such as Git Flow or GitHub Flow to keep your main branch clean.
Before merging any feature branch, ensure code reviews are done.
git merge feature/feature-name
4. Not Pulling Before Pushing
The Mistake:
Pushing changes without first pulling the latest updates from the remote repository can cause merge conflicts or push errors if someone else has updated the repository in the meantime.
Why This Happens:
This usually happens when developers assume they are working on the latest version of the repository without pulling in changes made by others.
How I Overcame This:
In my earlier days as a developer, I would often forget to pull changes from the remote repository before pushing my own updates. This led to frequent merge conflicts. I developed a habit of always pulling before pushing, which minimized these conflicts.
git pull origin main
How to Avoid It:
Always run
git pull origin main
(or your working branch) Before pushing your changes.git pull origin main
Use Git aliases or custom commands to streamline your workflow and avoid forgetting to pull.
Set up your IDE to remind you to pull before pushing.
5. Not Staging Changes Before Committing
The Mistake:
Sometimes, developers forget to stage the modified files before committing them, which results in incomplete or inconsistent commits.
Why This Happens:
This happens because developers may mistakenly assume that all modified files are staged automatically. Without use git add
, untracked or unstaged files won’t be included in the commit.
How I Overcame This:
I used to commit changes without staging them, which caused many issues down the road when I realized certain files were left out of the commit. Now, I run git status
regularly to see which files have been modified and ensure everything is staged before committing.
git status
git add .
How to Avoid It:
Always run
git status
to check the status of your files before committing.Use
git add .
or selectively stage files withgit add <filename>
.git add file1.js
Use an IDE or Git GUI tool that visually shows which files are staged.
6. Merging Without Understanding Conflicts
The Mistake:
Merging without understanding or properly resolving conflicts can lead to broken code or loss of functionality.
Why This Happens:
In the rush to merge branches, developers may not take the time to properly analyze the changes and conflicts, leading to mistakes.
How I Overcame This:
When I first encountered merge conflicts, I didn’t fully understand the cause, and this led to frustrating issues. Over time, I learned how to resolve merge conflicts properly by using git diff
and carefully reading through the conflicted areas before making corrections.
git diff
How to Avoid It:
Always review the changes that caused a conflict by using
git diff
or a visual tool.git diff
Don’t rush the merge process; take time to test everything after resolving conflicts.
If conflicts are complex, ask for help or perform a pair review before merging.
7. Force-Pushing Without Caution
The Mistake:
Force-pushing can overwrite important changes in the remote repository, causing lost work and confusion among team members.
Why This Happens:
Force-pushing is often used to rewrite history or to fix issues, but it can be dangerous, especially when used on shared branches.
How I Overcame This:
I once force-pushed to the main
branch to fix an issue, but I accidentally overwrote important work from my teammates. From then on, I made it a point to only use it git push --force
when necessary and always communicate with my team before doing so.
git push --force
How to Avoid It:
Only use
git push --force
for personal branches or when working solo.If you absolutely must force-push to a shared branch, use
git push --force-with-lease
, which ensures that you don’t overwrite changes made by others.git push --force-with-lease
8. Not Using Tags for Releases
The Mistake:
Not tagging releases can make it difficult to track versions and roll back to a previous state if something goes wrong.
Why This Happens:
Developers may focus on commits and forget the importance of version tags, especially when managing releases.
How I Overcame This:
After experiencing a problem where I needed to roll back to a previous stable release, I realised how crucial tags are. From that point on, I started tagging releases at every major point in development.
git tag -a v1.0.0 -m "First stable release"
git push origin v1.0.0
How to Avoid It:
Use Git tags to mark release versions or important milestones.
git tag -a v1.0.0 -m "Version 1.0 release" git push origin v1.0.0
Conclusion:
Git mistakes are inevitable, but learning from them is what makes you a better developer. By following best practices and becoming more aware of these common pitfalls, you can avoid most issues. Whether you’re working solo or with a team, mastering Git is essential for smooth collaboration and maintaining a clean codebase.
Your Turn!
Have you made any of these Git mistakes? How did you overcome them? Feel free to share your experiences in the comments below!
Subscribe to my newsletter
Read articles from Binshad directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Binshad
Binshad
💻 Exploring the intersection of technology and finance. 📈 Sharing insights on tech dev, Ai,market trends, and innovation. 💡 Simplifying the complex world of investing