Understanding Complex Features in C++: A Step-by-Step Guide
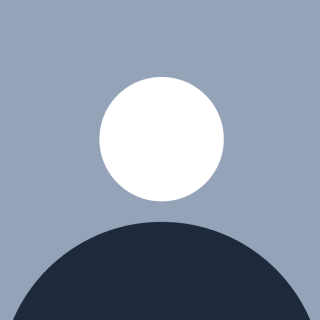
Table of contents
- Concepts we cover
- Anonymous Structs and Unions
- What Are They?
- Basic Syntax
- Accessing Members
- Why Use Anonymous Structs or Unions?
- Real-World Use Case: Hardware Register Mapping
- Nested Anonymous Types
- Compiler Behavior and Portability
- Rules and Restrictions
- Pitfall: Name Conflicts
- Advanced: Anonymous Unions in Unions
- Memory Layout Considerations
- Summary
- Best Practices
- Unnamed Bit-Fields
- Functions with Block Scope Declarations

C++ is a language rich in features, legacy, and complexity. While much of modern C++ has embraced readability and safety, there remain many features that are essential for certain system-level or performance-critical domains. Some of these features are obscure or misunderstood, yet they play critical roles in system behavior and language evolution.
In this article, we’ll thoroughly explore some of the most intricate and powerful constructs in C++, with detailed explanations, examples, use cases, and edge cases. The goal is to take you from beginner understanding to deep internalization of each topic.
Concepts we cover
Anonymous Structs and Unions
Unnamed Bit-Fields
Functions with Block Scope Declarations
Anonymous Structs and Unions
What Are They?
Anonymous structs and unions are structures or unions that are declared without a name. When used as members within another class, struct, or union, the members of the anonymous type are injected directly into the enclosing scope. This means you can access the members of the anonymous type as if they were members of the enclosing type.
Basic Syntax
struct Container {
union {
int i;
float f;
}; // Anonymous union
struct {
char a;
double b;
}; // Anonymous struct
};
In the above example, i
, f
, a
, and b
can be accessed directly through an instance of Container
.
Accessing Members
Container c;
c.i = 42;
c.b = 3.1415;
Why Use Anonymous Structs or Unions?
Anonymous types offer the following benefits:
Memory Layout Control: Useful for mapping memory in systems or embedded programming.
Cleaner Syntax: Allows access to deeply nested members without verbose naming.
Type Flattening: Members of the anonymous type become direct members of the enclosing type.
Real-World Use Case: Hardware Register Mapping
Embedded systems often use memory-mapped hardware registers. You might want to access the same memory region with different views:
struct RegisterMap {
union {
uint32_t raw;
struct {
uint32_t enable : 1;
uint32_t mode : 2;
uint32_t status : 5;
uint32_t : 24; // padding
};
};
};
RegisterMap reg;
reg.raw = 0x01;
std::cout << reg.enable << std::endl;
Here, you can both read/write the whole 32-bit register via raw
and access individual bit fields directly.
Nested Anonymous Types
Anonymous structs and unions can be nested inside each other.
struct Config {
union {
struct {
int x;
int y;
};
float coordinates[2];
};
};
In this case, x
and y
occupy the same memory as coordinates[0]
and coordinates[1]
.
Compiler Behavior and Portability
Different compilers treat anonymous types consistently today, but subtle differences can arise, especially in older compilers or across different platforms:
MSVC allows anonymous structs/unions by default.
GCC/Clang follow the standard strictly but may emit warnings or require specific flags in some cases.
Rules and Restrictions
Anonymous structs/unions must be members of another struct, union, or class.
At namespace scope, anonymous unions are only allowed if marked
static
.static union { int a; float b; }; // OK in global scope
Anonymous structs/unions cannot have member functions, constructors, or destructors.
Access specifiers (public/private/protected) apply from the enclosing class.
Multiple anonymous members that expose the same names lead to ambiguity.
Pitfall: Name Conflicts
struct A {
int value = 1;
};
struct B {
union {
int value; // Conflict with A
};
};
struct Derived : A, B {
void print() {
std::cout << value; // Error: ambiguous
}
};
Advanced: Anonymous Unions in Unions
union Mixed {
int i;
union {
float f;
double d;
}; // Nested anonymous union
};
Mixed m;
m.d = 2.718;
Here, both i
and d
are accessible from m
directly, even though d
is in a nested anonymous union.
Memory Layout Considerations
Anonymous unions are especially useful for defining overlapping memory views. Consider:
struct Packet {
union {
uint32_t all;
struct {
uint8_t header;
uint8_t command;
uint8_t data;
uint8_t checksum;
};
};
};
This allows accessing the whole packet as a single 32-bit value (all
) or byte-wise parts directly.
Summary
Feature | Supported |
Member functions | ❌ Not allowed |
Access specifiers | ✅ Inherited from parent |
Multiple anonymous members | ⚠️ Can cause ambiguity |
Namespace-scope anonymous union | ✅ Only if static |
Best Practices
Avoid name collisions by keeping anonymous members small.
Use in system-level code where memory layout is critical.
Avoid in high-level code unless layout control is needed.
That’s it for the first concept. I tried to provide as much detailed explanation as possible, feel free to explore this if you think you need more of it.
Now, let’s dive into the next concept, another fascinating topic not only in C++, but in the entire System Programming world, The Bit fields.
Unnamed Bit-Fields
What Are Bit-Fields?
Bit-fields allow you to define variables that occupy a specific number of bits rather than the entire size of their type. This is especially useful when working with low-level data representations, such as hardware registers or communication protocols.
struct Flags {
unsigned int ready : 1;
unsigned int error : 1;
unsigned int mode : 2;
};
Here, the Flags
struct only uses 4 bits in total: 1 for ready
, 1 for error
, and 2 for mode
.
What Are Unnamed Bit-Fields?
Unnamed bit-fields are bit-fields that do not have an identifier. They're typically used for padding or alignment purposes inside structures.
struct Example {
unsigned int a : 3;
unsigned int : 5; // unnamed bit-field, 5 bits of padding
unsigned int b : 2;
};
In this case, 5 bits are reserved between a
and b
, but those bits are inaccessible.
Why Use Unnamed Bit-Fields?
Padding: Add space between fields in memory layout.
Alignment: Force a new bit-field to start at a particular boundary.
Compliance: Match externally-defined binary layouts (like hardware or network protocols).
Use Case: Hardware Register Layout
Let’s say a register has specific bits defined and others reserved:
struct ControlRegister {
uint32_t enable : 1;
uint32_t reset : 1;
uint32_t : 6; // reserved bits
uint32_t mode : 2;
uint32_t : 22; // remaining unused bits
};
This layout mirrors a real-world memory-mapped register.
Zero-Width Unnamed Bit-Fields
Zero-width unnamed bit-fields force alignment to the next boundary of the declared type.
struct AlignedExample {
uint16_t a : 3;
uint16_t : 0; // forces alignment to next uint16_t boundary
uint16_t b : 5;
};
This is helpful when packing multiple fields while needing to start a new aligned section.
Edge Cases and Rules
An unnamed bit-field with non-zero width just skips bits.
A zero-width unnamed bit-field forces the next field to start on the next alignment boundary.
Unnamed bit-fields cannot be referenced — they have no names.
Unnamed bit-fields must have a type just like named ones.
Invalid Usage Examples
struct Invalid {
int : 5; // OK
: 3; // ❌ Error: must specify a type
};
Compiler-Specific Behavior
Some compilers align unnamed bit-fields to byte boundaries; others to the type size.
Code that relies on bit-field layout should be tested across compilers or use
#pragma pack
/alignas
.
Portability Warning
Bit-fields, especially unnamed ones, are implementation-defined in layout. The compiler decides how to lay them out in memory. Never assume bit offsets unless you control alignment explicitly.
Inspecting Layout
Use offsetof
and sizeof
to inspect layout behavior:
struct Demo {
uint8_t x : 2;
uint8_t : 0; // force next field to new byte
uint8_t y : 2;
};
std::cout << sizeof(Demo) << std::endl; // May show 2 bytes instead of 1
Best Practices
Use unnamed bit-fields only when absolutely necessary.
Favor named bit-fields and
alignas()
for clarity.For cross-platform binary compatibility, use
std::bitset
or manual shifting/masking.
Summary Table
Feature | Use |
Non-zero unnamed bit-field | Inserts padding bits |
Zero-width bit-field | Forces alignment |
Cannot be accessed | ✅ |
Common in hardware layouts | ✅ |
Yes, it feels really interesting, isn't it? Of course, not if you already know all of this. You are an expert, you know? Anyhow, That’s it for this concept for now, Let’s start with the next one.
Functions with Block Scope Declarations
Introduction
In C++, functions are typically declared at namespace scope or within class definitions. However, it's possible to declare functions within block scope, i.e., inside the body of another function or a compound statement. While rarely used in modern code, this construct is allowed by the C++ standard with several important limitations.
What is a Block Scope Declaration?
Block scope refers to a scope defined by a pair of braces {}
— for instance, inside a function body or an if
, for
, or while
statement. A declaration that occurs inside such a block is said to have block scope.
void outer() {
void inner(); // Function declaration at block scope
}
In this case, inner
is declared inside the function outer
. This is not the same as defining a local function — something which standard C++ does not support. Instead, this syntax is used to declare that such a function exists, possibly declared elsewhere.
Key Rules and Constraints
- Only Declarations Are Allowed: You may only declare a function inside a block. You cannot define the function body inside the block.
void f() {
void g(); // ✅ declaration
void h() {} // ❌ error: cannot define a function at block scope
}
- Visible in the Block Only: The declaration is scoped to the enclosing block. Outside of that block, the function name may be unknown.
void foo();
void outer() {
void foo(); // block scope declaration (shadows outer foo?)
// This may refer to the same foo or another overload — depends on context
}
Name Hiding: A block-scope function declaration may hide an outer declaration, particularly when default arguments are involved (we’ll cover this in the next section).
Linkage Is Still Global: Even though the declaration appears inside a block, the function it refers to must still have global or external linkage — this is not a way to create local functions.
Practical Use Cases
While rare in modern practice, block-scope function declarations can be found in legacy or very low-level code for a few reasons:
Forward Declaration: You might declare a function in a block before calling it, especially in translation units that mix C and C++.
Avoiding Header Pollution: Declare functions locally to indicate they are used only in this context.
Example:
void process() {
void helper(); // Declaration
helper(); // Call to the function defined elsewhere
}
void helper() {
std::cout << "I'm the helper!";
}
Edge Cases and Pitfalls
- Misunderstanding Definition vs Declaration: Developers might try to define a function inside another function, which is not allowed in C++ (unlike in some other languages).
void outer() {
void inner() { // ❌ illegal
// body
}
}
- Conflict with Overloads: If multiple overloads exist, declaring one inside a block can mask others or alter overload resolution unintentionally.
void log(int);
void log(double);
void run() {
void log(int); // this hides the 'log(double)' overload within this block
log(3.14); // ❌ error: no matching overload
}
- Confusion with Lambdas: Beginners often confuse this with lambda expressions, which are legal and common in C++ for defining inline callable objects.
void outer() {
auto inner = [](){ std::cout << "Hi"; }; // ✅ preferred modern style
inner();
}
Summary
Concept | Rule |
Function declaration allowed | ✅ inside a block |
Function definition allowed | ❌ not allowed inside a block |
Block-scoped function shadows outer | ✅ potentially |
Function linkage | Global/external only |
Practical use | Legacy or narrow scenarios |
Best Practices
Prefer to declare functions at namespace or class scope unless a strong reason exists.
Use lambdas when you need local behavior or closures.
Be cautious with overloads and name hiding.
That’s it for now. Hope you enjoyed the article. We will continue this series, covering few more interesting concepts in detail.
Thank you for reading till now.
Feel free to explore my C++ and other Github repositories at:
Until next time,
Keep learning and Have fun experimenting with C++ 😊
Subscribe to my newsletter
Read articles from Bhanuprakash Eagala directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Bhanuprakash Eagala
Bhanuprakash Eagala
Computer Science enthusiast