AWS CDK - test and production environments
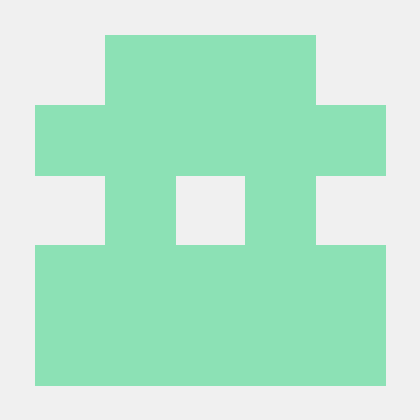

Last week, I used ChatGPT to write Python code that converts a CSV file uploaded to an S3 bucket into a table in an RDS (transactional database). Once the code was ready, I wanted to deploy it in a test environment before moving it to the production environment.
It turned out there is a convenient way to do that. First, we need to create environment.
env = Environment(account=os.environ["CDK_DEFAULT_ACCOUNT"], region=os.environ["CDK_DEFAULT_REGION"])
While writing this post, I noticed that in the above code line generated by ChatGPT, my AWS account ID was hardcoded, which is not a good practice and exposes sensitive data. This is a good reminder to review what AI creates. To avoid this mistake, we should read the AWS account ID and region from our local AWS account configuration files located in ~/.aws/credentials
and ~/.aws/config
.
When we deploy our app, AWS CDK will use our AWS account from the default or profiled AWS CLI configuration to set the CDK_DEFAULT_ACCOUNT
and CDK_DEFAULT_REGION
environment variables.
In the next step, we need to create two stacks for each environment.
app = cdk.App()
BudgetCsvTransformStack(app, "BudgetCsvTransformStack-Test", stage="test", env=env)
BudgetCsvTransformStack(app, "BudgetCsvTransformStack-Prod", stage="prod", env=env)
It is important to note that we are using a suffix in the stack name:
-Test for the test environment
-Prod for the production environment
Additionally, we define the stage argument in the BudgetCsvTransformStack constructor:
stage="test" for the test environment
stage="prod" for the production environment
It's important to note that the stage argument will be used in each AWS resource defined in the BudgetCsvTransformStack constructor to specify environment-specific resource names, for example.
bucket = s3.Bucket(
self, f"CsvUploadBucket-{stage}",
bucket_name=f"budget-csv-uploads-{stage}",
removal_policy=RemovalPolicy.DESTROY,
auto_delete_objects=True
)
As the last step, we pass the env
variable to the BudgetCsvTransformStack
constructor using the environment defined earlier
Now, when we want to deploy our app to a specific environment, we can use a command like the following:
cdk deploy BudgetCsvTransformStack-Test
We could also pass AWS CLI profile in cdk deploy command
cdk deploy BudgetCsvTransformStack-Test --profile my-profile
Here we are telling AWS CDK to take our AWS account information from AWS CLI my-profile profile. So both CDK_DEFAULT_ACCOUNT
and CDK_DEFAULT_REGION
environment variables will be defined based this profile instead of default one.
Before using AWS CLI profile in cdk deploy command it is required to bootstrap cdk configuration for this specific profile by running following command
cdk bootstrap --profile my-profile
When we want to destroy the app in a specific environment, we can use a command like the following:
cdk destroy BudgetCsvTransformStack-Test
I think this is a very convenient way to separate testing and production environments.
Here you could find full code used in this blog post
https://github.com/jrsokolow/budget_planner/blob/main/budget-csv-transform/app.py
Subscribe to my newsletter
Read articles from Jakub Sokolowski directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
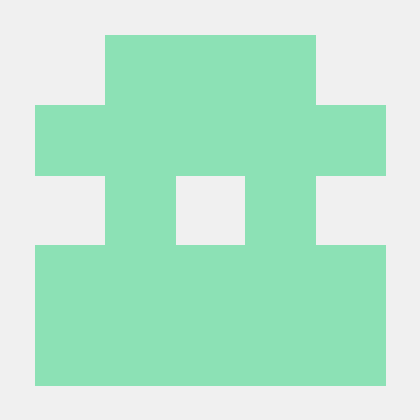