JavaScript Cheatsheet: Everything You Need in One Place
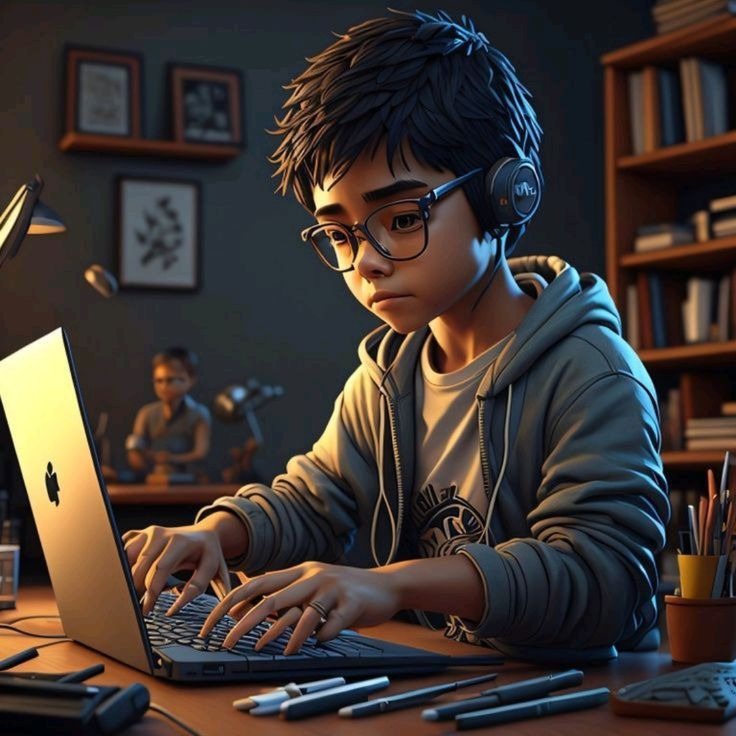
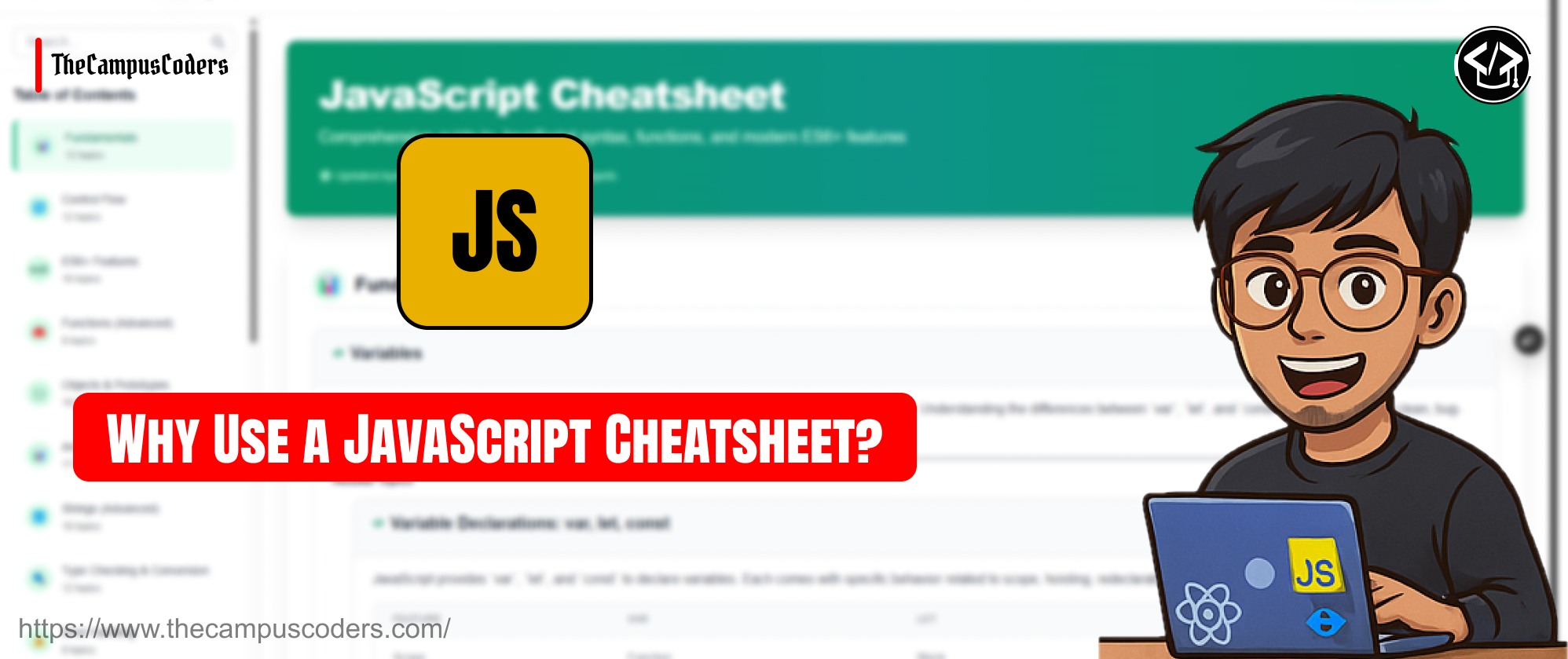
If you’re diving into the world of JavaScript or prepping for your next dev interview, having a go-to cheatsheet can save you tons of time. Whether you're a beginner or brushing up after a break, this one-stop reference from The Campus Coders is designed to cover the most essential JavaScript concepts — fast, clear, and practical.
Let’s jump right in. 👇
Why Use a JavaScript Cheatsheet?
In the real world, devs don’t memorize everything — they build fast by recalling patterns. A cheatsheet gives you:
A quick refresher when you're stuck
Cleaner, copy-paste-ready syntax
Real-world code snippets and use-cases
Less time Googling, more time building
What This Cheatsheet Covers
Here’s what you’ll find inside our JavaScript Cheatsheet:
1. Variables & Data Types
let
,const
,var
→ When to use whatPrimitive Types:
string
,number
,boolean
,null
,undefined
,symbol
,bigint
Reference Types:
Object
,Array
,Function
2. Loops & Conditionals
if
,else
,switch
for
,while
,do-while
,for...of
,for...in
Ternary Operators for cleaner logic
3. Functions
Function declaration vs expression
Arrow functions &
this
contextCallback, Higher-order functions
4. Objects & Arrays
Object destructuring
Spread and Rest operators
Array methods:
map
,filter
,reduce
,find
,some
,every
5. Asynchronous JS
setTimeout
,setInterval
Promises →
.then()
,.catch()
async/await
patternFetch API & error handling
6. Scope, Hoisting, Closures
Lexical scope
Function and block scoping
Closure examples you’ll actually use
7. DOM Manipulation
document.querySelector
,getElementById
Event listeners:
click
,submit
,keydown
Changing styles, text, and attributes
8. ES6+ Features
Template literals
Modules (
import
,export
)Default parameters
Optional chaining
?.
9. Debugging & Console
console.log()
,warn()
,error()
,table()
typeof
,instanceof
,JSON.stringify()
10. Type Conversion
Implicit vs explicit conversion
Truthy & Falsy values
Unary + operator,
parseInt
,parseFloat
,Number()
Pro Tip: How to Use This Cheatsheet Effectively
Bookmark it in your dev browser
Practice by re-typing snippets (not just copy-paste)
Pair each section with mini projects
Use it as a daily warm-up when learning JavaScript
What Makes The Campus Coders Cheatsheet Different?
Unlike generic cheatsheets, we built this with:
Real-world devs in mind (not just theory)
Hinglish-style tooltips for added relatability
Interactive code blocks (coming soon!)
Printable PDF version so you can learn offline too
Ready to Code Smarter?
This isn’t just a cheatsheet — it’s your JavaScript companion for coding faster, learning deeper, and preparing better for projects and interviews.
Make sure to follow us on all platforms @thecampuscoders for daily JavaScript tips, tutorials, and bite-sized learning!
Subscribe to my newsletter
Read articles from Raaj Aryan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
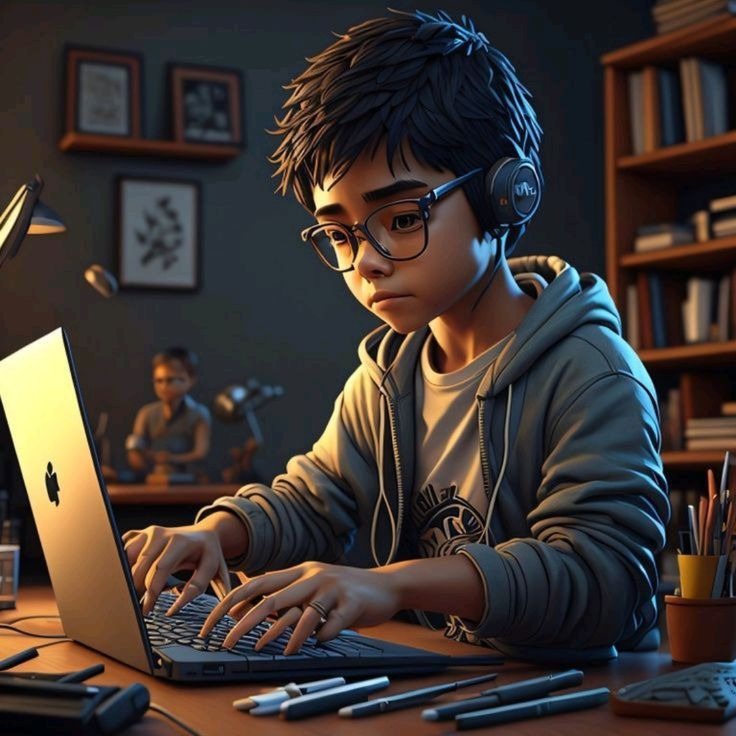
Raaj Aryan
Raaj Aryan
MERN Stack Developer • Open Source Contributor • DSA With Java • Freelancer • Youtuber • Problem-solving •