How to Display Images on a Website Without Source Links Using Vue.js
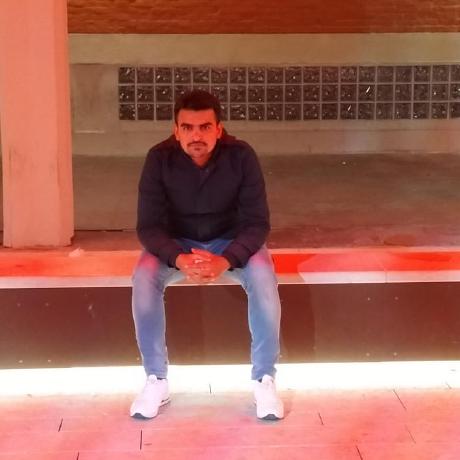

Images play a vital role in modern web design, enhancing the visual appeal and user experience of a website. However, there are instances where you might want to show images without revealing their source link or invoking unnecessary actions such as the default browser behavior when an image is clicked. For Vue.js developers, achieving this while maintaining lightweight, performant, and clean code is essential.
In this article, we’ll explore how to achieve this and provide practical tips for ensuring a seamless user experience.
Why Hide Source Links for Images?
When images are displayed on a website, their <img>
elements can inadvertently act as clickable links if not handled properly. This behavior can:
Distract users with unnecessary actions.
Make images appear interactive when they’re not intended to be.
Lead to security or copyright concerns if the image source URL is exposed.
To prevent this, Vue.js provides a versatile framework for implementing a clean, link-free image display with minimal overhead.
Implementing Image Display in Vue.js
Here's a lightweight Vue.js implementation to render images while avoiding source links:
1. Use the <img>
Tag Correctly
To display an image in Vue.js, bind the src
attribute dynamically while ensuring no href
or default clickable behavior is associated with it. Here's how:
<template>
<div class="image-container">
<img :src="imageSource" :alt="imageAlt" />
</div>
</template>
<script>
export default {
data() {
return {
imageSource: 'path/to/your/image.jpg', // Replace with your image path
imageAlt: 'A description of the image', // For accessibility
};
},
};
</script>
<style scoped>
.image-container img {
display: block;
max-width: 100%;
height: auto;
cursor: default; /* Prevents the pointer from showing like a link */
}
</style>
This example ensures:
Dynamic Loading: The image source can be updated dynamically.
Accessibility: The
alt
attribute improves accessibility for visually impaired users and SEO.
2. Prevent Clickable Behavior
To avoid default actions such as opening the image URL in a new tab when users accidentally right-click or drag, additional styling and event handling can be applied.
<template>
<div class="image-wrapper" @dragstart.prevent>
<img :src="imageSource" :alt="imageAlt" @contextmenu.prevent />
</div>
</template>
<style scoped>
.image-wrapper img {
pointer-events: none; /* Disables all mouse interactions */
user-select: none; /* Prevents text/image selection */
}
</style>
Here:
@contextmenu.prevent
disables the right-click context menu for the image.pointer-events: none
ensures that the image is entirely non-interactive.user-select: none
disables selection to prevent accidental drag-and-drop.
3. Using Base64-Encoded Images (Optional)
For scenarios where you don’t want to expose a direct image URL, Base64 encoding can be a viable option. This approach embeds the image data directly within the HTML, avoiding external references.
<template>
<div class="image-container">
<img :src="base64Image" alt="Embedded Image" />
</div>
</template>
<script>
export default {
data() {
return {
base64Image: 'data:image/png;base64,iVBORw0KGgoAAAANSUhEUg...', // Replace with actual Base64 string
};
},
};
</script>
Although Base64 encoding is effective for small images, it’s not recommended for large or multiple images due to increased HTML size and slower rendering.
4. Optimize for Performance
Displaying images without source links should not compromise performance. Here are some best practices:
Lazy Loading: Load images only when they’re in the viewport to reduce initial load time. Vue.js has a wide range of lazy-loading plugins or can leverage the native
loading="lazy"
attribute:<img :src="imageSource" alt="Lazy Loaded Image" loading="lazy" />
Image Compression: Use optimized formats like WebP or compressed JPEG/PNG images.
CDN Integration: Deliver images from a content delivery network (CDN) for faster loading and improved caching.
Conclusion
Displaying images without exposing source links in Vue.js is straightforward with the right combination of clean HTML, CSS, and JavaScript. By following the strategies outlined here, you can create a polished and professional image display that aligns with your website’s goals.
Vue.js developers can implement these techniques to ensure images are presented in a user-friendly manner without unnecessary distractions, all while maintaining optimal performance.
Happy coding!
Subscribe to my newsletter
Read articles from Ahmed Raza directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
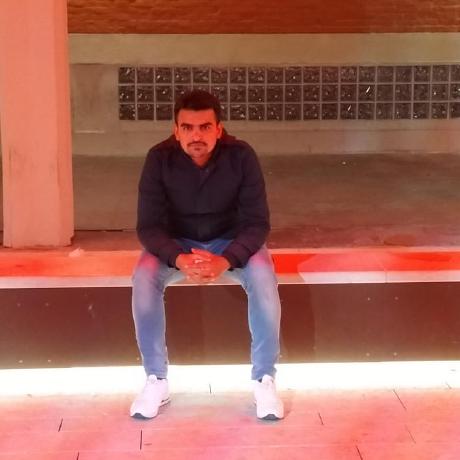
Ahmed Raza
Ahmed Raza
Ahmed Raza is a versatile full-stack developer with extensive experience in building APIs through both REST and GraphQL. Skilled in Golang, he uses gqlgen to create optimized GraphQL APIs, alongside Redis for effective caching and data management. Ahmed is proficient in a wide range of technologies, including YAML, SQL, and MongoDB for data handling, as well as JavaScript, HTML, and CSS for front-end development. His technical toolkit also includes Node.js, React, Java, C, and C++, enabling him to develop comprehensive, scalable applications. Ahmed's well-rounded expertise allows him to craft high-performance solutions that address diverse and complex application needs.