Context API vs Redux Toolkit
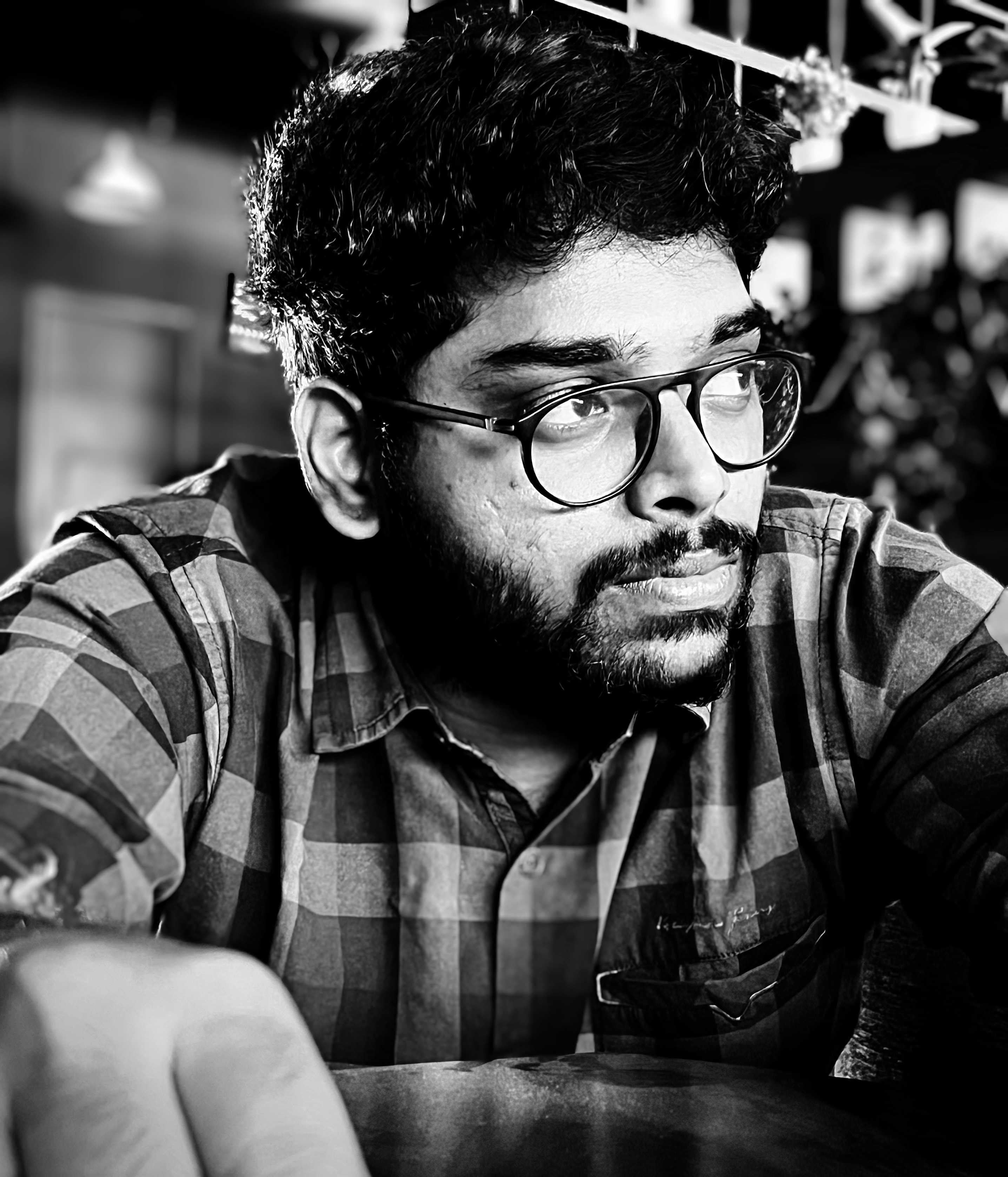
๐ง High-Level Overview
Feature | Context API | Redux |
Purpose | Prop drilling replacement | Scalable state management |
Type | React feature (built-in) | External library |
State | Typically global static values | Global dynamic state (app-wide) |
Updating State | useState , useReducer | Actions โ Reducers โ Store |
Middleware Support | โ Not built-in | โ
Supports middleware like redux-thunk |
Devtools | โ Limited | โ Redux DevTools are ๐ฅ |
Performance | Poor with frequent updates | Highly optimized with selective subscriptions |
Scalability | Suitable for small to medium apps | Built for large-scale apps |
๐ Key Differences (In Depth)
1. Philosophy & Use Case
Context API is meant for infrequently changing values โ like theme, user auth info, locale โ and avoiding prop drilling.
Redux is a centralized state container. It's ideal when your app has complex interactions, frequent updates, and multiple views/components needing access to and updating the same state.
โ Use Context for: theme, language, auth.
โ Use Redux for: shopping carts, complex forms, async data fetching, multi-step workflows.
2. State Update Performance
Context re-renders all consumers when state updates, even if they donโt use the updated value.
Redux optimizes with connect/selectors, so only components using the updated state re-render.
// Context problem:
<AuthContext.Provider value={user}>
<Header /> // re-renders when user changes
<Footer /> // re-renders too, even if it doesnโt use user
</AuthContext.Provider>
This can lead to performance bottlenecks as the app scales.
3. Middleware, Devtools & Debugging
Context API has no middleware support (like logging, async logic).
Redux has a robust middleware ecosystem (
redux-thunk
,redux-saga
) + devtools that show time travel, action history, diffs, etc.
This makes debugging and async flows far easier in Redux.
4. Code Structure & Scalability
Context gets messy when:
You have multiple contexts
They depend on each other
You need to nest them
<ThemeContext.Provider value={theme}>
<AuthContext.Provider value={user}>
<LangContext.Provider value={lang}>
<App />
</LangContext.Provider>
</AuthContext.Provider>
</ThemeContext.Provider>
- Redux centralizes everything in a single store, making it easier to maintain and test.
5. Testing and Maintainability
Redux's pure functions (reducers, actions) are easily testable.
Context relies more on hooks and nesting, which can complicate unit tests.
โ Why You Should NOT Use Context API Alone (in large apps)
Performance: Context re-renders can cause unnecessary updates.
Scalability: Context nesting becomes unmanageable in large apps.
Debugging: No time-travel devtools, no logging middleware.
Async logic: Difficult to handle with only
useReducer
oruseState
.No Middleware: No way to intercept, log, or chain actions.
๐ก When to Combine Them
You can actually use them together smartly:
Use Context API to wrap your app with one-time configuration (theme, auth, store).
Use Redux for the appโs dynamic state and complex flows.
<AuthContext.Provider value={user}>
<ReduxProvider store={store}>
<App />
</ReduxProvider>
</AuthContext.Provider>
TL;DR
Use Case | Use Context API | Use Redux |
Static global state (theme, auth) | โ | โ |
Complex, dynamic state | โ | โ |
Needs middleware / async handling | โ | โ |
Small-scale app | โ | โ |
Large-scale app | โ | โ |
Subscribe to my newsletter
Read articles from Sridhar Murali directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
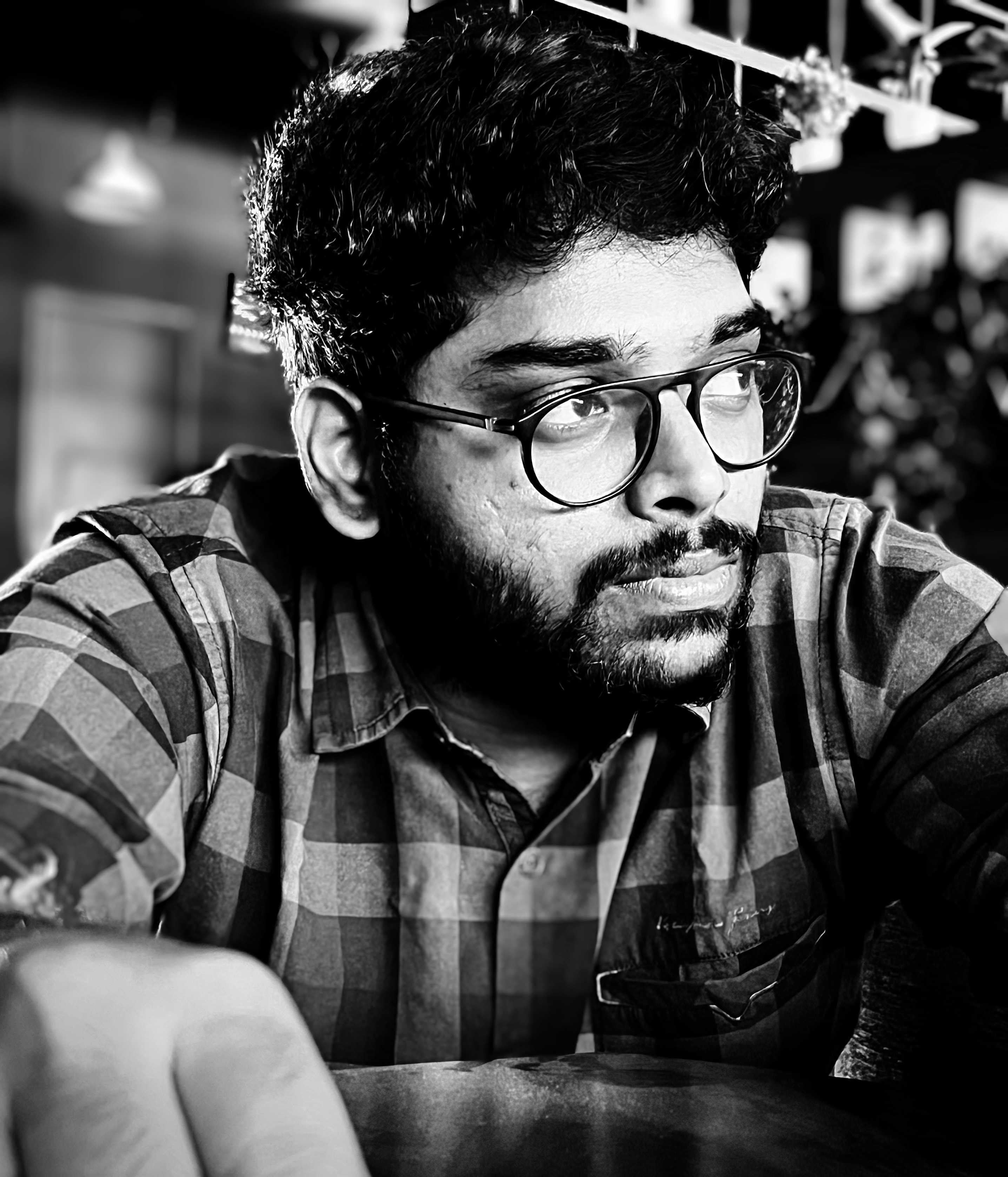
Sridhar Murali
Sridhar Murali
Passionate Frontend Engineer with over 4.5 years of experience in frontend development and a strong previous experience in Quality Assurance of 4 years (2016-2020). Skilled in building responsive, high-quality applications using JavaScript frameworks like Ember.js and React.js, combining meticulous attention to detail from a QA background with a commitment to delivering seamless user experiences. Recognized for writing efficient, accessible code, strong debugging skills