Codewars #1: Fusion Chamber Shutdown
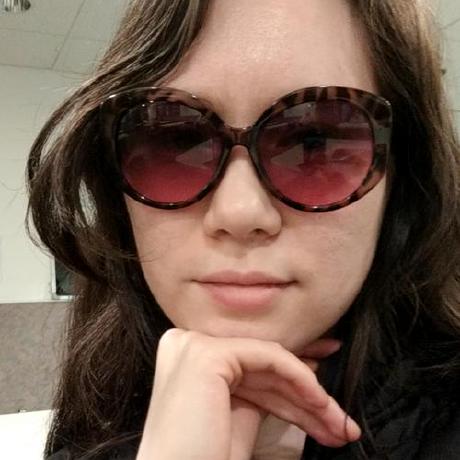
Resource: Codewars
Kyu: 7
Go to description
Practices:
- 4.14.25
Working out the Problem
Given an array, the contents entail [C, H, O], quantities of carbon, hydrogen, and oxygen elements. These elements combine to form the molecules: water (H20), carbon dioxide (CO2), and methane (CH4). They are produced in order of affinity, meaning if there are 20 C, 120 H, 20 O, the H and O will combine to form 20 water molecules, leaving 20 C and 80 H. The remainder will combine into 20 methane molecules.
Water: H2O
H = O x 2eg. H = 20, O = 10 :: H = O x 2
Water = O = 10
H = 0, O = 0eg. H = 17, O = 10 :: H < O x 2
Water = (H - 1) / 2 = 8
H = 1, O = 2eg. H = 22, O = 10 :: H > O x 2
Water = O = 10
H = 2, O = 0
Carbon dioxide: CO2
O = C x 2
Methane: CH4
H = C x 4
const burner = (c, h, o) => {
const mole = []
if (h >= o * 2)
mole.push(o)
else
mole.push(Math.floor(h / 2))
o -= mole[0]
h -= mole[0] * 2
if (o >= c * 2)
mole.push(c)
else
mole.push(Math.floor(o / 2))
c -= mole[1]
o -= mole[1] * 2
if (h >= c * 4)
mole.push(c)
else
mole.push(Math.floor(h / 4))
return mole
}
Submitted:
function burner(c, h, o) {
const water = h >= o * 2 ? o : Math.floor(h / 2)
o -= water
h -= water * 2
const co2 = o >= c * 2 ? c : Math.floor(o / 2)
c -= co2
o -= co2 * 2
const methane = h >= c * 4 ? c : Math.floor(h / 4)
return [water, co2, methane];
}
Iterative
Rather than the math, use incrementation and count how many iterations before exceeding either number. For example, in water, there are 2H for every O, so incrementing H +2 and O +1, regardless of whether even or odd, and whichever is higher, the max number of iterations is the number of molecules produced. However, to use the end sums to determine remaining H and O, the sum prior to exceeding the max has to be used, so there is a check, else while incrementing for each iteration, decrement from the amount of H and O available.
let water = 0
do {
o--
h -= 2
water++
} while (h - 2 > 0 && o - 1 > 0
// [5, 44, 1] should be [5, 45, 0]
// [1, 1, 352] should be [0, 0, 354]
for (h, o; h > 0 && o > 0; h -= 2, o--)
water++
// [ 5, 44, +0 ] should be [ 5, 45, +0 ]
// [ +0, +0, 353 ] should be [ +0, +0, 354 ]
while (h - 2 >= 0 && o > 0) {
water++
h -= 2
o--
}
Mininum
The thinking I missed: Water is always 2H for every O, and whichever number is lower naturally fits into the other and can is the number of molecules made.
I didn’t know this before: ~~ provides integer closer to 0
Refer clever solution
const water = Math.min(~~(H / 2), O)
const carbon = Math.min(~~((O - water) / 2), C)
const methane = Math.min(~~((H - water * 2) / 4), C - carbon)
Solutions
Compare and Decrement
const burner = (c, h, o) => { const water = h >= o * 2 ? o : Math.floor(h / 2) o -= water h -= water * 2 const co2 = o >= c * 2 ? c : Math.floor(o / 2) c -= co2 o -= co2 * 2 const methane = h >= c * 4 ? c : Math.floor(h / 4) return [water, co2, methane] }
Iterative
const burner = (c, h, o) => { let water = 0, co2 = 0, methane = 0 while (h - 2 >= 0 && o > 0) { water++ h -= 2 o-- } while (o - 2 >= 0 && c > 0) { co2++ o -= 2 c-- } while (h - 4 >= 0 && c > 0) { methane++ h -= 4 c-- } return [water, co2, methane] }
Minimum
const burner = (c, h, o) => { const water = Math.min(~~(h / 2), o) h -= water * 2 o -= water const co2 = Math.min(~~(o / 2), c) c -= co2 const methane = Math.min(~~(h / 4), c) return [water, co2, methane] }
Subscribe to my newsletter
Read articles from Stella Marie directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
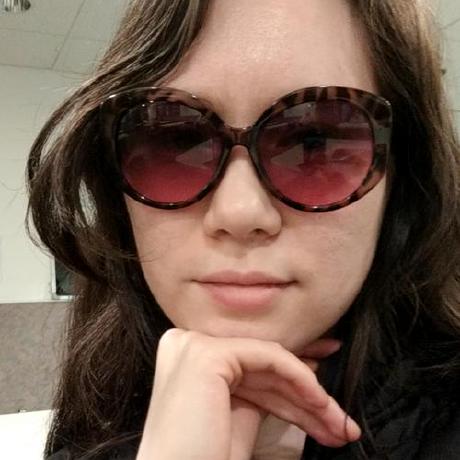
Stella Marie
Stella Marie
Interests: Frontend D&D Abstract designs Storytelling in games and writing BS Informatics, specialization: User Experience design [USA] MA Design, specialization: Interaction design [China]