7 Effective Techniques to Prevent Duplicate Requests in Your Application
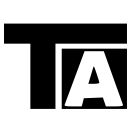
5 min read
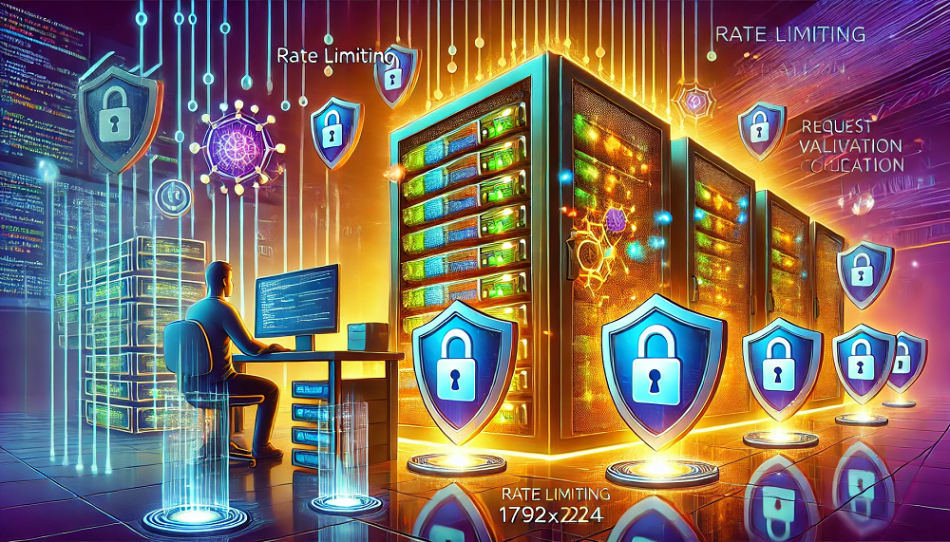
1. Understanding Duplicate Requests
Before diving into solutions, let’s first understand what duplicate requests are, why they occur, and how they can impact your application.
1.1 What Are Duplicate Requests?
Duplicate requests occur when the same request is sent multiple times to the server within a short period. This can happen intentionally or unintentionally, and they often lead to unnecessary processing and inconsistent data states.
1.2 Common Causes of Duplicate Requests
- User Interactions: Users double-clicking or refreshing pages can send multiple requests.
- Network Issues: Poor or unstable network connections can lead to resending requests.
- Client-Side Bugs: Errors in the front-end code can sometimes cause requests to fire off repeatedly.
1.3 The Impact of Duplicate Requests
Duplicate requests can lead to:
- Increased server load
- Higher operational costs
- Inaccurate data processing
- Negative user experiences
2. Techniques to Prevent Duplicate Requests
Let's explore some of the most effective techniques to prevent duplicate requests and mitigate their impact on your application.
2.1 Implementing Client-Side Guards
Preventing duplicate requests should start from the client-side. Using techniques like disabling buttons after clicks and leveraging JavaScript libraries can go a long way in minimizing duplicates.
Code Example:
document.getElementById('submitButton').addEventListener('click', function() {
this.disabled = true;
// proceed with sending request
});
This simple code disables the button after the first click, ensuring no further requests can be initiated until re-enabled.
2.2 Leveraging Debouncing
Debouncing helps by delaying the processing of user actions until a specified wait time has passed. This is particularly effective in scenarios where actions like typing or scrolling can trigger repeated requests.
Code Example:
function debounce(func, delay) {
let timer;
return function(...args) {
clearTimeout(timer);
timer = setTimeout(() => func.apply(this, args), delay);
};
}
const sendRequest = debounce(() => {
// perform the request
}, 300);
With this code, the sendRequest function will only execute once the user stops triggering it for 300 milliseconds.
2.3 Using Unique Request IDs
One of the most reliable server-side methods for detecting and preventing duplicate requests is by generating unique request IDs. These can be created on the client-side and sent with each request.
Code Example:
import java.util.UUID;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestHeader;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class RequestController {
@PostMapping("/process")
public ResponseEntity<String> processRequest(@RequestHeader("Request-ID") String requestId) {
if (requestAlreadyProcessed(requestId)) {
return ResponseEntity.status(HttpStatus.CONFLICT).body("Duplicate request");
}
// Process request
return ResponseEntity.ok("Request processed successfully");
}
private boolean requestAlreadyProcessed(String requestId) {
// Check if the request ID has already been handled
return false;
}
}
This code shows how a unique Request-ID header can be used to track requests and reject duplicates.
2.4 Implementing Server-Side Rate Limiting
Rate limiting helps to control the frequency of requests from a single user or IP address, which can be an effective strategy for preventing duplicates caused by spamming or malicious behavior.
Code Example:
Rate limiting can be done using libraries like Redis with Java, where you set a limit for a particular IP or user.
// Assuming Redis is configured
public boolean isRateLimited(String userId) {
String key = "user:" + userId + ":requests";
long requestCount = redis.incr(key);
if (requestCount == 1) {
redis.expire(key, 60); // set TTL for 1 minute
}
return requestCount > MAX_REQUESTS;
}
2.5 Using Mutex or Lock Mechanisms
In cases where requests can modify shared resources, mutex or lock mechanisms can be employed to prevent concurrent modifications by ensuring that only one request accesses the resource at a time.
Code Example:
Using Java's synchronized keyword or ReentrantLock can provide effective locking.
import java.util.concurrent.locks.ReentrantLock;
public class ResourceController {
private ReentrantLock lock = new ReentrantLock();
public void accessResource() {
lock.lock();
try {
// Perform resource modification
} finally {
lock.unlock();
}
}
}
2.6 Utilizing Idempotent Requests
An idempotent request guarantees that performing the same operation multiple times has the same effect as doing it once. This is useful when certain endpoints need to handle retries gracefully.
2.7 Using Message Queues
Message queues can be configured to detect duplicate messages and only process unique requests, which is particularly useful in distributed systems.
3. Conclusion
Preventing duplicate requests is critical for maintaining application efficiency and delivering a seamless user experience. The techniques outlined here, from client-side debouncing to server-side rate limiting, will help you to manage and prevent duplicate requests effectively. As you implement these strategies, consider your application's specific requirements and experiment with different approaches for the best results.
If you have any questions or other techniques you’ve found effective, feel free to comment below! Let’s discuss.
Read more at : 7 Effective Techniques to Prevent Duplicate Requests in Your Application
0
Subscribe to my newsletter
Read articles from Tuanhdotnet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
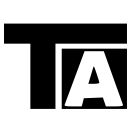
Tuanhdotnet
Tuanhdotnet
I am Tuanh.net. As of 2024, I have accumulated 8 years of experience in backend programming. I am delighted to connect and share my knowledge with everyone.