⚛️ React Topic 4: Props (Properties) ⚛️ React Topic 5: State

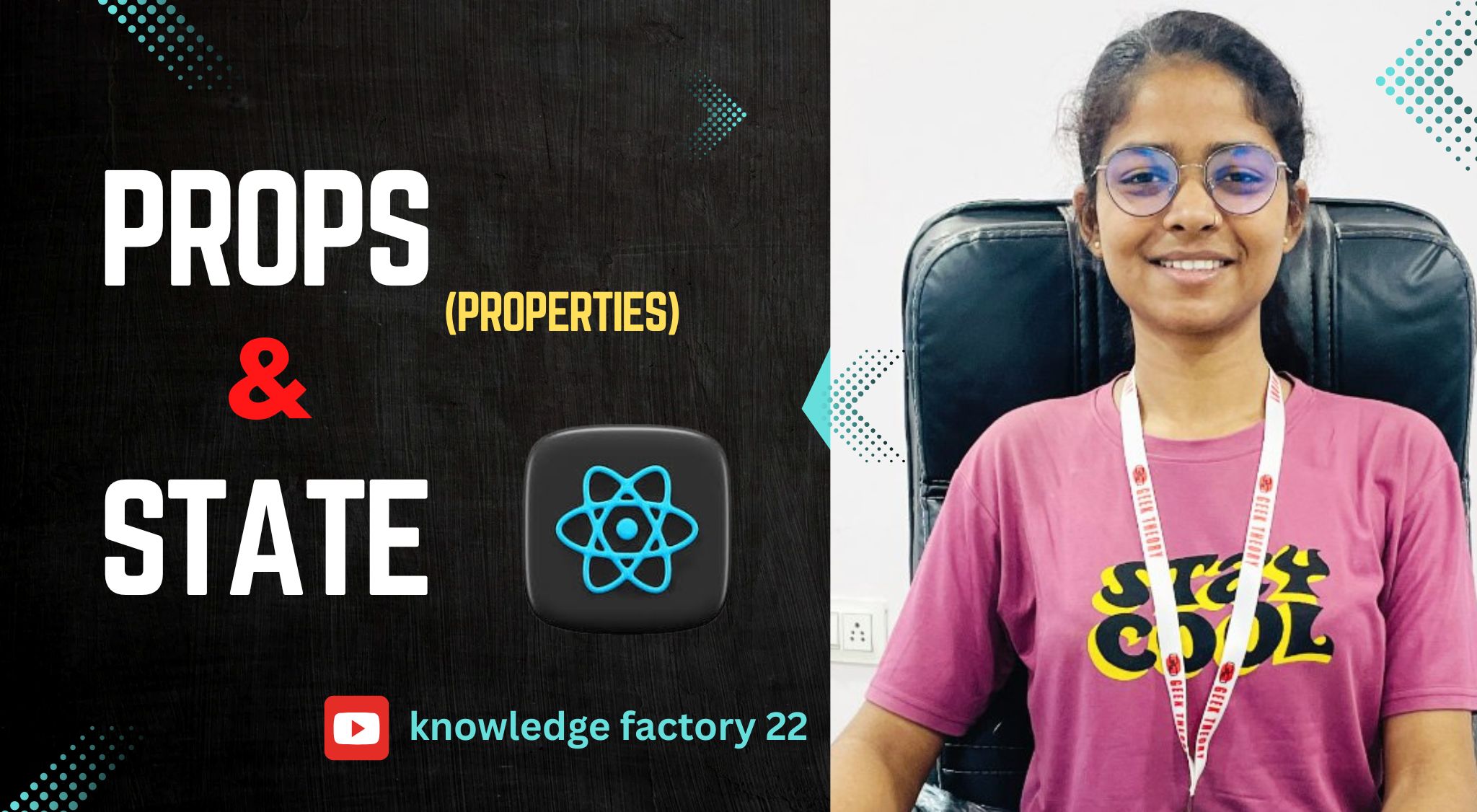
These two concepts form the backbone of dynamic React apps. I’ve covered everything in simple language with real-life examples — perfect for both students and working professionals.
🔹 Topic 4: Props (Properties)
✅ What are Props?
Props means "properties".
They are used to pass data from one component to another — like sending arguments to a function.
📦 Props are read-only. You can use them, but you can’t change them from inside the component.
🧑🍳 Real-Life Analogy:
Imagine you are ordering food online.
You select a pizza with size: “Large”, and topping: “Paneer”
The delivery app sends that info to the restaurant
🧠 Similarly, React sends props (data) from parent to child component.
🧑💻 Example: Passing Props to Component
👉 App.js
//jsx
import React from 'react';
import ProfileCard from './components/ProfileCard';
function App() {
return (
<div>
<h1>Our Team</h1>
<ProfileCard name="Amit" role="Frontend Developer" />
<ProfileCard name="Riya" role="UI/UX Designer" />
</div>
);
}
export default App;
👉 ProfileCard.js
//jsx
import React from 'react';
function ProfileCard(props) {
return (
<div className="card">
<h2>{props.name}</h2>
<p>{props.role}</p>
</div>
);
}
export default ProfileCard;
🔥 Destructuring Props (Cleaner way)
//jsx
function ProfileCard({ name, role }) {
return (
<div className="card">
<h2>{name}</h2>
<p>{role}</p>
</div>
);
}
📌 Props Are:
Passed from parent → child
Read-only
Useful to reuse components with different data
🆚 Props vs State
Feature | Props | State |
Data Source | Passed from parent | Managed inside component |
Editable | ❌ Read-only | ✅ Mutable |
Usage | Reuse, pass data | Store dynamic values |
Example Use | User info, product name | Counter, toggle, input field |
✅ Summary of Props
Props help pass data into components
Props make components dynamic and reusable
Props are read-only and must be passed from the parent
⚛️ React Topic 5: State
✅ What is State?
State is a built-in object in React that is used to store and manage dynamic data within a component.
Whenever state changes, the UI automatically updates.
🧠 Real-Life Analogy:
Imagine a light switch:
ON → light is on
OFF → light is off
🧠 You can think of state as the “current situation”. You click to change the state → and the light (UI) updates.
🧪 useState Hook (Functional Component)
We use useState
hook to create state in a function component.
//jsx
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0); // default value 0
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>Click Me</button>
</div>
);
}
🧯 Explanation:
count
→ current value of statesetCount
→ function to update stateuseState(0)
→ initial value is 0
Every time you click the button, state is updated and UI re-renders.
🔄 Updating State
You can update state using the setter function (like setCount
).
//jsx
setCount(count + 1);
✅ State updates are asynchronous — React batches them for performance.
🆚 State vs Props
Feature | Props | State |
Passed from | Parent Component | Inside Component |
Editable | ❌ No | ✅ Yes |
Purpose | Reusability | Interactivity |
Example | User info, theme | Counter, modal open/close |
🧠 Real-Life Use Cases
Use Case | Use |
Click counter | State |
Show user profile details | Props |
Toggle sidebar open/close | State |
List of products passed to card | Props |
✅ Summary of State
State is used to store dynamic values
We use
useState()
in function componentsWhen state changes → UI updates automatically
Use state for interactions, use props for data transfer
⚛️ Props & State – Mini Projects + Assignment
🧪 Mini Project 1: Digital Visiting Cards using Props
🎯 Objective:
Learn to pass dynamic data (name, profession, image, etc.) using props.
🧱 What You'll Build:
A list of digital visiting cards where each card is a reusable component and displays different user details passed via props.
📁 File Structure:
src/
├── App.js
└── components/
└── VisitingCard.js
🔸 VisitingCard.js
// jsx
import React from 'react';
function VisitingCard({ name, profession, image }) {
return (
<div className="card">
<img src={image} alt={name} width="100" />
<h2>{name}</h2>
<p>{profession}</p>
</div>
);
}
export default VisitingCard;
🔹 App.js
//jsx
import React from 'react';
import VisitingCard from './components/VisitingCard';
function App() {
return (
<div>
<h1>Our Team</h1>
<VisitingCard name="Ankit" profession="React Developer" image="https://randomuser.me/api/portraits/men/1.jpg" />
<VisitingCard name="Neha" profession="UI Designer" image="https://randomuser.me/api/portraits/women/2.jpg" />
</div>
);
}
export default App;
✅ Concepts Covered:
Props passing (name, profession, image)
Reusability of components
Props destructuring
🧪 Mini Project 2: Counter App using State
🎯 Objective:
Understand how to use and update state using useState
.
🔸 App.js
//jsx
import React, { useState } from 'react';
function App() {
const [count, setCount] = useState(0);
return (
<div style={{ textAlign: 'center' }}>
<h1>Counter App</h1>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
<button onClick={() => setCount(count - 1)}>Decrement</button>
</div>
);
}
export default App;
✅ Concepts Covered:
useState Hook
Updating UI dynamically
Button click + state update
Clean layout using inline styles
🧪 Mini Project 3: Toggle Profile using State
🎯 Objective:
Learn how state can control visibility of elements.
🔸 App.js
//jsx
import React, { useState } from 'react';
function App() {
const [showProfile, setShowProfile] = useState(true);
return (
<div style={{ textAlign: 'center' }}>
<h1>Toggle Profile</h1>
<button onClick={() => setShowProfile(!showProfile)}>
{showProfile ? "Hide" : "Show"} Profile
</button>
{showProfile && (
<div>
<img src="https://randomuser.me/api/portraits/men/75.jpg" width="100" alt="profile" />
<h3>Rahul Sharma</h3>
<p>Full Stack Developer</p>
</div>
)}
</div>
);
}
export default App;
✅ Concepts Covered:
useState + conditional rendering
Button toggle
Real-world toggle functionality (show/hide)
📚 Assignment: Profile List with Toggle and Counter
🧩 Task:
Create a
ProfileCard
component that accepts name, role, and image using propsIn
App.js
, render 3 different cards by passing different props.Add a “Show Details” toggle button inside each card using state to show/hide a description.
Add a “Like” button using state to track and display how many likes each profile received.
✨ Bonus (For Working Professionals):
Use
useEffect()
to log “Card updated” in console when like count changes.Add a reset button to reset likes to 0.
✅ Concepts Practiced:
Props (passing data)
State (toggle + counter)
Conditional Rendering
Combining multiple concepts into one clean UI
🔔 Stay Connected
If you found this article helpful and want to receive more such beginner-friendly and industry-relevant React notes, tutorials, and project ideas —
📩 Subscribe to our newsletter by entering your email below.
And if you're someone who wants to prepare for tech interviews while having a little fun and entertainment,
🎥 Don’t forget to subscribe to my YouTube channel – Knowledge Factory 22 – for regular content on tech concepts, career tips, and coding insights!
Stay curious. Keep building. 🚀
Subscribe to my newsletter
Read articles from Payal Porwal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Payal Porwal
Payal Porwal
Hi there, tech enthusiasts! I'm a passionate Software Developer driven by a love for continuous learning and innovation. I thrive on exploring new tools and technologies, pushing boundaries, and finding creative solutions to complex problems. What You'll Find Here On my Hashnode blog, I share: 🚀 In-depth explorations of emerging technologies 💡 Practical tutorials and how-to guides 🔧Insights on software development best practices 🚀Reviews of the latest tools and frameworks 💡 Personal experiences from real-world projects. Join me as we bridge imagination and implementation in the tech world. Whether you're a seasoned pro or just starting out, there's always something new to discover! Let’s connect and grow together! 🌟