π Session Token vs Refresh Token β The Simplest Explanation!
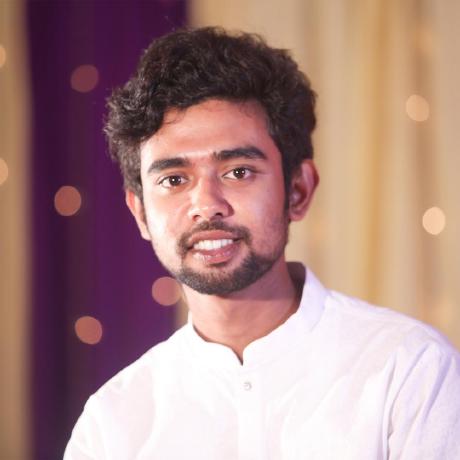

Modern web apps run on tokens. But which one does what? Let's break down Session Tokens and Refresh Tokens so clearly that youβll never forget, and confidently implement them in any project.
Letβs go from concept to code and build your solid understanding with:
π§ What to Build (Token System Essentials)
π Functions Youβll Need
π Algorithms/Flows
π οΈ Tools & Libraries (with alternatives)
π Trendy/Best Practices
π’ What Big Tech Uses
π§ 1. WHAT TO BUILD β Token Auth System in Any App
Any software (web, mobile, API-based) with token authentication will have 3 main parts:
Step | Action |
π 1 | Login (generate access + refresh tokens) |
π 2 | Refresh token (get new access token) |
π 3 | Logout (invalidate refresh token) |
π 2. REQUIRED FUNCTIONS (in pseudo + JS-style)
You typically need to write 5 core functions:
// 1. Login
function login(email, password) {
// validate user
// generate accessToken + refreshToken
// store refreshToken securely (DB or cookie)
}
// 2. Generate Access Token
function generateAccessToken(user) {
// return jwt.sign(user, secret, { expiresIn: '15m' })
}
// 3. Generate Refresh Token
function generateRefreshToken(user) {
// return jwt.sign(user, refreshSecret, { expiresIn: '7d' })
}
// 4. Refresh Token Endpoint
function refresh(req) {
// validate refreshToken
// if valid => issue new accessToken
}
// 5. Logout
function logout(req) {
// remove/invalidate refreshToken
}
Add:
β
middleware
to check access token on every API callπ Token rotation strategy
π 3. ALGORITHM FLOW (Pseudocode)
A. Login Flow
User submits email + password
β
If valid:
β generate access token (15 mins)
β generate refresh token (7 days)
β send access in body, refresh in HTTP-only cookie
B. API Request Flow
Frontend sends access token in headers
β
Backend verifies token
β
If valid β grant access
If expired β ask frontend to refresh token
C. Token Refresh Flow
Frontend sends refresh token (cookie)
β
Backend verifies it
β
If valid β issue new access token (maybe refresh token too)
β
Frontend replaces old token and continues
D. Logout Flow
User clicks logout
β
Frontend deletes tokens (cookie/localStorage)
β
Backend blacklists or deletes refresh token from DB
π οΈ 4. TOOLS TO USE
π Token Generator
jsonwebtoken
(Node.js)pyjwt
(Python)nimbus-jose-jwt
(Java)
π¦ Session Store (Optional)
Redis (store refresh token or blacklist tokens)
In-memory (for demo)
Database (Mongo, Postgres)
πͺ Cookie Management
cookie-parser
(Node)HttpOnly
+SameSite=Strict
for refresh tokens
π Auth Libs (if you want ready-made)
NextAuth.js
(Next.js)Passport.js
(Node)Firebase Auth
(Google, prebuilt solution)Supabase Auth
(Backendless)
β‘ 5. TRENDY BEST PRACTICES
β
Use short-lived access tokens (15m to 1h)
β
Use refresh tokens with rotation (and maybe detection of reuse)
β
Store refresh token in HTTP-only secure cookies, never in localStorage
β
Add a logout-all-devices or token revoke option
β
Use middleware/auth guard in APIs/routes
β¨ Extra: Use a queue (e.g., Redis) to store a blacklist of used refresh tokens (detect hijacking)
π’ 6. BIG TECH STRATEGY
Company | Auth System | Notes |
Session cookie-based (internal), tokens for APIs | Uses long-lived refresh system | |
OAuth2 + OpenID + JWT | Access & Refresh tokens, stored securely | |
Discord | Access token + refresh token flow | Like OAuth2 spec |
Spotify | Strict refresh token rotation, OAuth2 | Modern best practices |
Netflix | Short-lived access token, secure refresh handling | High emphasis on device-level auth |
π¬ Even big companies don't keep users logged in forever. They refresh tokens in the background to make UX smooth.
β What You Should Write (Almost Any Software Needs):
Backend
Login route
Token generation utilities
Token refresh route
Logout route
Auth middleware
Optional: Token storage in DB or Redis
Frontend
Store access token (memory/localStorage)
Auto-refresh tokens on expiration
Logout flow
Attach token to API headers
π§ Side-by-Side Snapshot
Feature | Session Token (Access) | Refresh Token |
Purpose | Access APIs | Get new access tokens |
Lifespan | Short (15mβ1h) | Long (daysβweeks) |
Sent with requests | β Yes | β No |
Risk if stolen | High (frequently exposed) | Low (stored securely) |
Storage | Memory/localStorage/cookie | HTTP-only cookie (preferred) |
Rotation | β Optional | β Recommended |
π― Quick Summary
Use access tokens for immediate API calls.
Use refresh tokens to silently renew access without asking the user to log in again.
Store refresh tokens securely. Rotate them. Invalidate them on logout.
Access token is your key to the house. Refresh token is your ability to get a new key if you lose the old one.
Subscribe to my newsletter
Read articles from Rajon Dey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
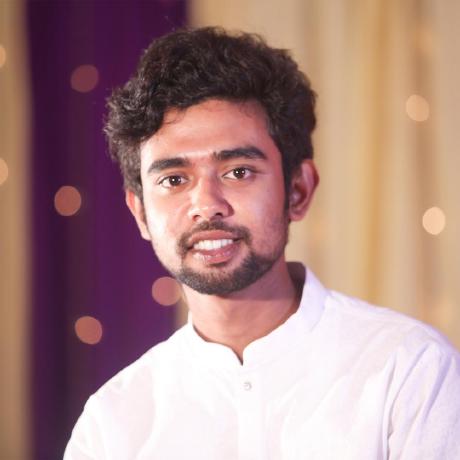
Rajon Dey
Rajon Dey
I'm a Software Developer, my passion is crafting smart, meaningful, and professional websites that enhance the online presence of individuals and businesses, making the web a more engaging, effective, and better platform. Get the data - https://developer-data.beehiiv.com/