Controller → Model → View (CMV): The Java Pattern You Didn't Know You Needed ⚙️
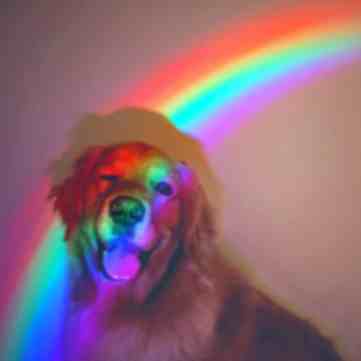

Here's a LinkedIn-style post explaining CMV (Controller-Model-View) in contrast to the more common MVC (Model-View-Controller) pattern, with a focus on Java and full explanation:
🧠 Let’s Talk About CMV: Controller – Model – View in Java ☕
Most of us are familiar with MVC – Model-View-Controller. But have you ever thought of flipping the sequence to CMV – Controller-Model-View?
Sounds similar? Not quite. Let’s break it down and see how CMV can offer a cleaner flow, especially when working with Java frameworks like Spring MVC, Jakarta EE, or even custom architecture in Java desktop apps.
🔁 MVC (Traditional Flow)
Model: Represents the data or business logic.
View: The UI – what the user sees.
Controller: Handles input, updates the model, and selects the view.
✅ This works well for simple apps. ❌ But as the app grows, things can get messy, especially when the view and controller are too tightly coupled.
✅ CMV – Why this order?
In CMV, the flow is Controller ➡️ Model ➡️ View — and the control stays with the controller until the final output.
🔍 CMV in Java – Explained
1. Controller
The entry point. It handles requests (from UI or API), does validation, manages sessions, and invokes services or models.
@GetMapping("/user/{id}")
public String getUser(@PathVariable Long id, Model model) {
User user = userService.getUserById(id); // → Model
model.addAttribute("user", user); // → View binding
return "userView"; // → View
}
2. Model
This layer is all about data and business logic. Could be a simple POJO or full-blown service layer.
public class User {
private Long id;
private String name;
private String email;
// Getters and Setters
}
Or a service that fetches data:
public User getUserById(Long id) {
return userRepository.findById(id).orElseThrow(...);
}
3. View
In Java (Spring), this could be Thymeleaf, JSP, or any templating engine that renders the final HTML.
<!-- userView.html -->
<h1>Welcome, [[${user.name}]]!</h1>
🔄 Flow Recap:
Controller receives the request 👉 calls the Model to get/process data 👉 passes that to the View for rendering.
This decouples responsibilities better and follows the natural sequence of user interaction: input ➡ logic ➡ output.
🚀 Benefits of CMV in Java:
✅ Better separation of concerns
✅ Easier to test each layer
✅ More intuitive flow in modern backend frameworks
✅ Scalable for complex apps (REST APIs, microservices, etc.)
💬 Have you ever structured your application this way — Controller → Model → View?
Drop your thoughts or experience with architecture patterns in the comments! 👇
#Java #SpringBoot #SoftwareArchitecture #MVC #CMV #DesignPatterns #WebDevelopment #CleanCode #BackendDevelopment
Subscribe to my newsletter
Read articles from Bhavik Prajapati directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
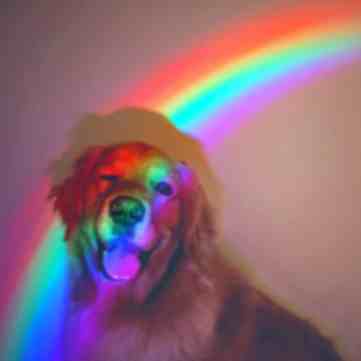
Bhavik Prajapati
Bhavik Prajapati
Software Developer at Konze India Pvt Ltd | MERN/MEAN Stack Enthusiast | Founder of Code with World I'm Bhavik Prajapati, a passionate software developer and a Computer Science graduate from LD College of Engineering. Currently, I specialize in full-stack development using React.js, Angular, Express.js, and Node.js at Konze India Pvt Ltd, where I thrive on solving complex problems and optimizing solutions. As the founder of Code with World and an avid tech blogger, I regularly share insights on Java, Data Structures, and cutting-edge optimization techniques to empower others in the tech community. I’m driven by the belief that “You are not what you think you are, but what you think, YOU ARE!” Let’s connect, collaborate, and code! Together, we can shape the future of technology.