HTML Role Attribute Tutorial
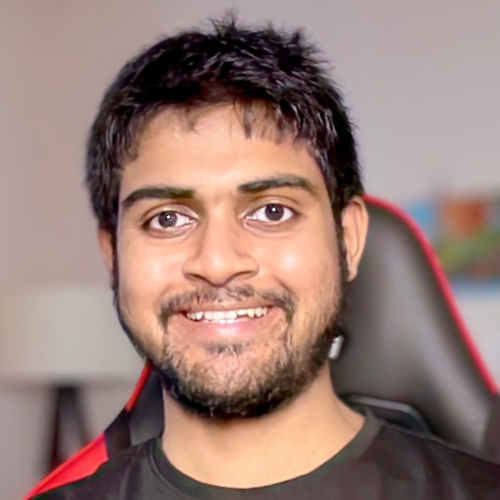

What is the HTML Role Attribute?
The role
attribute is part of the Web Accessibility Initiative - Accessible Rich Internet Applications (WAI-ARIA) specification. It is used to define the purpose or function of an HTML element to assistive technologies, such as screen readers, to enhance web accessibility for users with disabilities.
The role
attribute provides semantic meaning to elements, especially when native HTML elements do not sufficiently convey their purpose or when custom components are created using non-semantic elements like <div>
or <span>
.
Example:
<div role="button">Click me</div>
Here, the div
is assigned the button
role to indicate it behaves like a button, even though it’s not a native <button>
element.
Why Use the Role Attribute?
The role
attribute is crucial for:
Improving Accessibility: It helps assistive technologies understand the purpose of elements, enabling users with disabilities to navigate and interact with web content effectively.
Enhancing Semantics: It adds meaning to elements that lack native semantic roles, such as custom UI components in JavaScript frameworks.
Supporting Dynamic Content: For web applications with dynamic or non-standard interfaces,
role
ensures that assistive technologies can interpret interactive elements correctly.
When to Use the Role Attribute
Use the role
attribute in the following scenarios:
Custom Components: When building custom widgets (e.g., a
<div>
acting as a checkbox or tab) that don’t use native HTML elements.Dynamic Web Applications: In single-page applications (SPAs) or frameworks like React, Angular, or Vue, where non-semantic elements are often used for interactivity.
Fallback for Older Browsers: When using HTML5 semantic elements (e.g.,
<nav>
,<main>
) that may not be fully supported by older assistive technologies, you can addrole
as a fallback.Clarifying Ambiguous Elements: When an element’s purpose isn’t clear from its native HTML tag or context.
When NOT to Use the Role Attribute
Avoid Redundancy: Do not add a
role
to an element that already has a clear native semantic meaning. For example,<button role="button">
is unnecessary because<button>
already implies thebutton
role.Use Native Elements First: Prefer native HTML elements (e.g.,
<button>
,<nav>
) over non-semantic elements with roles unless absolutely necessary.
Common Role Values
The ARIA specification defines many roles, categorized as follows:
Landmark Roles: Identify key sections of a page.
- Examples:
role="banner"
,role="navigation"
,role="main"
,role="footer"
.
- Examples:
Widget Roles: Describe interactive components.
- Examples:
role="button"
,role="checkbox"
,role="dialog"
,role="tab"
.
- Examples:
Document Structure Roles: Provide context for content.
- Examples:
role="article"
,role="heading"
,role="listitem"
.
- Examples:
Abstract Roles: Used for framework development, not directly in HTML (e.g.,
role="widget"
).
Example of Landmark Roles:
<header role="banner">
<h1>Website Title</h1>
</header>
<nav role="navigation">
<ul>
<li><a href="/">Home</a></li>
<li><a href="/about">About</a></li>
</ul>
</nav>
<main role="main">
<h2>Main Content</h2>
<p>This is the primary content of the page.</p>
</main>
Best Practices for Using the Role Attribute
Use Native HTML Elements First:
Prefer
<button>
over<div role="button">
.Use semantic HTML5 elements like
<nav>
,<main>
,<article>
instead of<div>
with roles whenever possible.
Combine with ARIA Attributes:
Pair
role
with relevant ARIA attributes to provide additional context. For example:<div role="alert" aria-live="assertive">Error: Invalid input!</div>
Here,
aria-live="assertive"
ensures the alert is announced immediately by screen readers.
Avoid Overriding Native Roles:
- Do not change the implied role of a native element. For example,
<button role="heading">
is incorrect and confusing to assistive technologies.
- Do not change the implied role of a native element. For example,
Test with Assistive Technologies:
Use tools like NVDA, VoiceOver, or JAWS to test how your
role
attributes are interpreted.Browser extensions like axe DevTools or WAVE can help identify ARIA misuse.
Follow the ARIA Authoring Practices:
Refer to the WAI-ARIA Authoring Practices for guidance on implementing roles correctly.
Ensure interactive roles (e.g.,
button
,checkbox
) are keyboard-accessible and support expected behaviors (e.g., pressingEnter
orSpace
on arole="button"
).
Limit Use of Roles on Non-Interactive Elements:
- Avoid adding interactive roles (e.g.,
button
) to static elements unless they are programmatically interactive.
- Avoid adding interactive roles (e.g.,
Use Landmark Roles Sparingly:
- Apply landmark roles like
navigation
,main
, orbanner
only once per page unless the context justifies multiple instances (e.g., multiplenavigation
regions).
- Apply landmark roles like
Validate ARIA Usage:
- Ensure roles are valid and follow the ARIA specification. Invalid roles (e.g.,
role="invalidrole"
) are ignored by assistive technologies.
- Ensure roles are valid and follow the ARIA specification. Invalid roles (e.g.,
Example: Custom Checkbox
<div role="checkbox" aria-checked="false" tabindex="0" onclick="toggleCheckbox(this)">
Custom Checkbox
</div>
<script>
function toggleCheckbox(element) {
const isChecked = element.getAttribute('aria-checked') === 'true';
element.setAttribute('aria-checked', !isChecked);
}
</script>
The
role="checkbox"
indicates the element is a checkbox.aria-checked
tracks the state (checked/unchecked).tabindex="0"
makes it focusable via keyboard.JavaScript handles the toggle behavior.
Common Mistakes to Avoid
Redundant Roles:
Incorrect:
<nav role="navigation">
(redundant, as<nav>
impliesnavigation
).Correct:
<nav>
.
Incorrect Role Combinations:
Incorrect:
<div role="button" aria-checked="true">
(button
does not supportaria-checked
).Correct: Use
role="checkbox"
for elements witharia-checked
.
Missing ARIA States/Properties:
Incomplete:
<div role="dialog">
(lacksaria-labelledby
oraria-describedby
).Correct:
<div role="dialog" aria-labelledby="dialogTitle"> <h2 id="dialogTitle">Dialog Title</h2> <p>Content</p> </div>
Overusing Roles:
- Avoid adding roles to every element. Use them only when necessary to clarify purpose or enhance accessibility.
Testing and Validation Tools
Screen Readers: Test with NVDA (Windows), VoiceOver (macOS/iOS), or TalkBack (Android).
Browser DevTools: Use Chrome’s Accessibility panel or Firefox’s Accessibility Inspector.
Automated Tools: Run audits with Lighthouse, axe DevTools, or WAVE.
Manual Testing: Ensure keyboard navigation and ARIA announcements work as expected.
Comprehensive List of ARIA Roles
Below is a reference list of all WAI-ARIA roles with a one-line, easy-to-understand description for each. Use this as a quick guide when applying roles to your HTML elements.
Landmark Roles
banner: A header section, typically containing the site logo or title.
complementary: A supporting section, like a sidebar, that complements the main content.
contentinfo: A footer section with metadata, like copyright or contact info.
form: A section containing a form for user input.
main: The primary content area of the page.
navigation: A section with links for navigating the site or app.
region: A distinct section of the page that deserves its own label.
search: A section containing a search form or input.
Widget Roles
alert: A message with urgent information, like an error or warning.
alertdialog: A dialog box for urgent messages that requires user action.
button: An interactive element that triggers an action when clicked.
checkbox: A toggleable input that can be checked or unchecked.
dialog: A pop-up window that requires user interaction.
gridcell: A single cell within a grid or table.
link: A clickable element that navigates to another resource or page.
log: A section that displays a stream of updates, like a chat log.
marquee: A scrolling or changing text area, like a news ticker.
menu: A list of interactive options, like a dropdown menu.
menubar: A horizontal bar containing menu options.
menuitem: A single option within a menu.
menuitemcheckbox: A menu item that can be toggled on or off.
menuitemradio: A menu item that is part of a mutually exclusive group.
option: A selectable item in a listbox or dropdown.
progressbar: A visual indicator of a task’s progress.
radio: A single selectable option within a group of mutually exclusive choices.
scrollbar: A control for scrolling content in a container.
searchbox: An input field specifically for search queries.
slider: A control for selecting a value within a range.
spinbutton: An input for selecting a value by incrementing or decrementing.
status: A container for non-urgent status updates or messages.
switch: A toggleable control with on/off states, like a checkbox.
tab: A single item in a tabbed interface.
tabpanel: The content area associated with a specific tab.
textbox: A single-line or multi-line text input field.
timer: A display for countdowns or elapsed time.
tooltip: A small pop-up with additional information about an element.
treeitem: An item within a hierarchical tree structure.
Document Structure Roles
article: A self-contained piece of content, like a blog post or news story.
cell: A single cell in a table (not part of a grid).
columnheader: A header for a table or grid column.
definition: A term or concept being defined.
directory: A list of references or links, like a table of contents.
document: A main content area representing a document.
feed: A scrollable list of articles or updates, like a social media feed.
figure: A referenced image, diagram, or illustration.
group: A collection of related elements, like a set of form fields.
heading: A title or subtitle for a section of content.
img: An image or graphic.
list: A group of items, like a bulleted or numbered list.
listbox: A selectable list of options, like a dropdown.
listitem: A single item within a list.
math: A mathematical expression or equation.
note: Additional or supplementary information.
presentation: An element that should be ignored by assistive technologies.
row: A single row in a table or grid.
rowgroup: A group of rows in a table, like a header or footer group.
rowheader: A header for a table or grid row.
separator: A divider between sections or items.
table: A data table with rows and columns.
term: A word or phrase being defined or explained.
toolbar: A collection of interactive tools or controls.
tree: A hierarchical list of items, like a file explorer.
treegrid: A grid that also supports hierarchical navigation.
Abstract Roles (Not for Direct Use)
command: A generic role for actionable elements (use specific roles like
button
instead).composite: A widget that contains multiple interactive elements (use specific roles like
grid
).input: A generic input element (use specific roles like
textbox
).landmark: A generic navigation region (use specific roles like
main
).range: An element representing a range of values (use specific roles like
slider
).roletype: The base role for all ARIA roles (not used directly).
section: A generic content area (use specific roles like
article
).sectionhead: A generic heading (use
heading
instead).select: A generic selectable list (use
listbox
ormenu
).structure: A generic document structure (use specific roles like
list
).widget: A generic interactive component (use specific roles like
button
).window: A generic window or dialog (use
dialog
instead).
Conclusion
The role
attribute is a powerful tool for making web content accessible, especially for custom components and dynamic applications. By following best practices—using native elements first, pairing roles with appropriate ARIA attributes, and testing thoroughly—you can ensure your web content is inclusive and usable for all users. The comprehensive list of roles above serves as a quick reference for selecting the appropriate role for your elements.
For more details, refer to the WAI-ARIA specification and the ARIA Authoring Practices Guide.
Subscribe to my newsletter
Read articles from Junaid Bin Jaman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
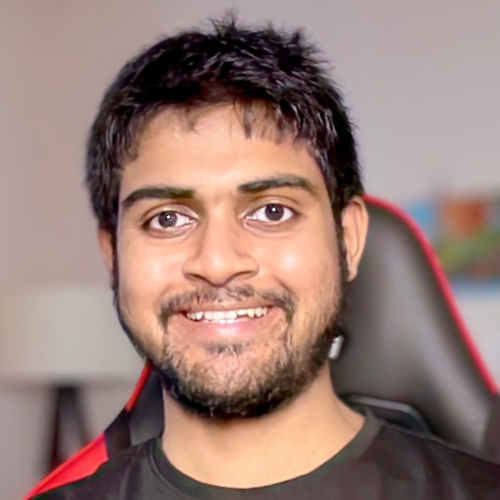
Junaid Bin Jaman
Junaid Bin Jaman
Hello! I'm a software developer with over 6 years of experience, specializing in React and WordPress plugin development. My passion lies in crafting seamless, user-friendly web applications that not only meet but exceed client expectations. I thrive on solving complex problems and am always eager to embrace new challenges. Whether it's building robust WordPress plugins or dynamic React applications, I bring a blend of creativity and technical expertise to every project.