Introduction to Vue.js
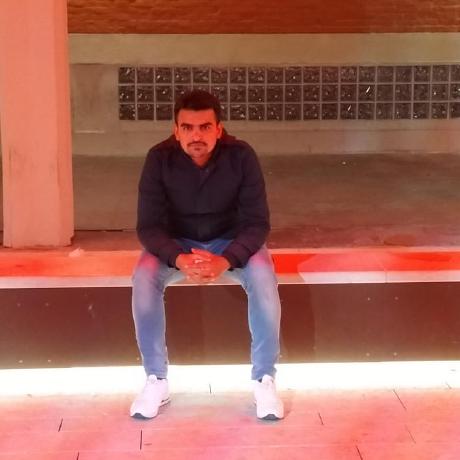

Vue.js is a progressive JavaScript framework used for building user interfaces. Created by Evan You in 2014, Vue.js has gained immense popularity due to its simplicity, flexibility, and ability to integrate with existing projects. It is designed to be incrementally adoptable, meaning you can use as much or as little of it as you need.
Key Features of Vue.js
Reactive Data Binding: Vue's core feature allows automatic synchronization between the model and the view.
Component-Based Architecture: It enables developers to break down the UI into reusable components.
Directives: Special attributes like
v-bind
andv-for
make it easy to manipulate the DOM.Vue CLI: A powerful tool for scaffolding and managing Vue.js projects.
Two-Way Data Binding: Achieved using the
v-model
directive, simplifying form handling.Integration: Can be integrated into projects alongside other libraries or used for full-fledged Single Page Applications (SPAs).
Vue.js Syntax with Examples
To understand Vue.js, let’s start with a simple example.
Setting Up Vue.js
You can include Vue.js in your project via a CDN link or install it via npm. Here's an example using the CDN:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Vue.js Example</title>
<script src="https://cdn.jsdelivr.net/npm/vue@2"></script>
</head>
<body>
<div id="app">
<h1>{{ message }}</h1>
<input v-model="message" placeholder="Type something here">
<p>Character count: {{ message.length }}</p>
</div>
<script>
new Vue({
el: '#app',
data: {
message: 'Hello, Vue.js!'
}
});
</script>
</body>
</html>
Explanation of the Code
The
div
withid="app"
: This is the root element where Vue will control the DOM.{{ message }}
: This is an example of Vue's template syntax for data binding.v-model
: A directive that creates two-way data binding between the input field and themessage
data property.Vue Instance: Created using
new Vue()
, whereel
specifies the element to mount Vue, anddata
contains the reactive properties.
Building a Counter Component
Vue's component system lets you create reusable elements. Here's an example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Vue.js Component Example</title>
<script src="https://cdn.jsdelivr.net/npm/vue@2"></script>
</head>
<body>
<div id="app">
<counter></counter>
</div>
<script>
Vue.component('counter', {
data: function() {
return {
count: 0
};
},
template: `
<div>
<button @click="count++">Click me</button>
<p>Button clicked {{ count }} times.</p>
</div>
`
});
new Vue({
el: '#app'
});
</script>
</body>
</html>
Explanation
Vue.component
: Defines a new component calledcounter
.Template: Includes a button and a paragraph to display the count.
Event Listener (
@click
): Binds the click event to increment the count.Reusable: The
counter
component can be used multiple times within the app.
Learning Vue.js from the Official Source
For an authoritative and comprehensive guide to learning Vue.js, visit the official Vue.js documentation. It provides tutorials, API references, and examples for beginners and advanced users.
Conclusion
Vue.js is a robust framework that simplifies the process of building interactive and dynamic user interfaces. Its lightweight nature, coupled with powerful features like two-way data binding and component-based architecture, makes it a popular choice for developers worldwide. By mastering Vue.js, you can create efficient, maintainable, and scalable web applications.
Subscribe to my newsletter
Read articles from Ahmed Raza directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
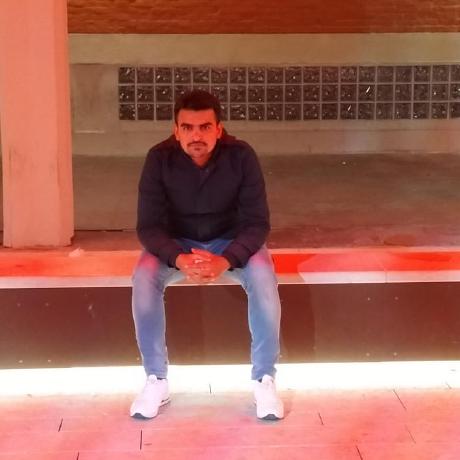
Ahmed Raza
Ahmed Raza
Ahmed Raza is a versatile full-stack developer with extensive experience in building APIs through both REST and GraphQL. Skilled in Golang, he uses gqlgen to create optimized GraphQL APIs, alongside Redis for effective caching and data management. Ahmed is proficient in a wide range of technologies, including YAML, SQL, and MongoDB for data handling, as well as JavaScript, HTML, and CSS for front-end development. His technical toolkit also includes Node.js, React, Java, C, and C++, enabling him to develop comprehensive, scalable applications. Ahmed's well-rounded expertise allows him to craft high-performance solutions that address diverse and complex application needs.