Why Your Node.js App Freezes (And How Worker Threads Can Save You)
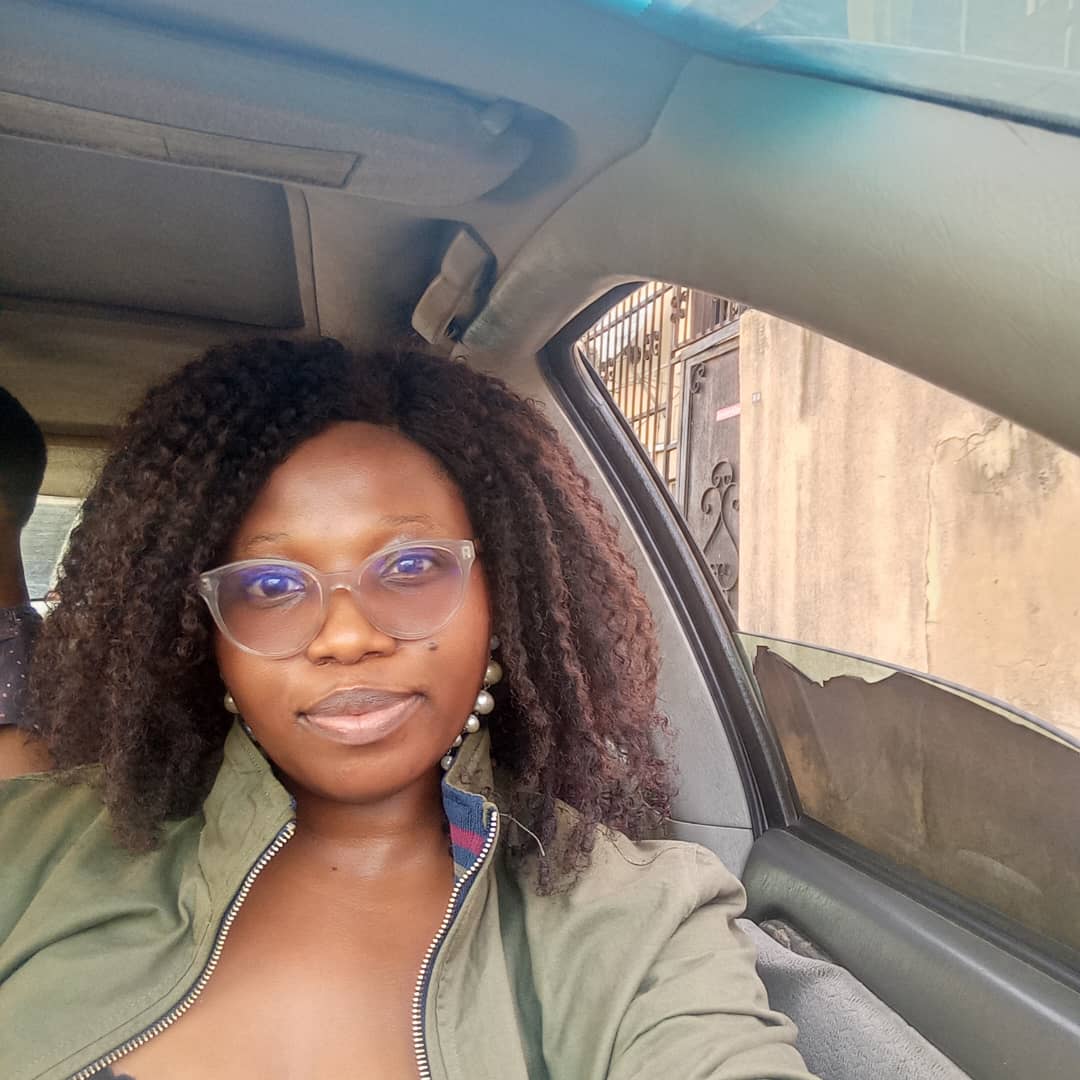

You've built an awesome Node.js app that your friends love using. Then disaster strikes - someone tries to generate a report and your entire application freezes. Everyone's experience grinds to a halt.
This happens because Node.js runs on a single thread by default. When something CPU-intensive comes along, everything else has to wait.
Enter worker threads: your solution to keeping Node.js applications responsive.
Worker Threads Explained (For Real Humans)
Imagine you're running a pizza restaurant:
Without Worker Threads:
You're taking orders, making pizzas, serving customers, and handling payments all by yourself
When a large catering order comes in, you stop everything else to make 20 pizzas
Regular customers get frustrated and leave while waiting
With Worker Threads:
You still take orders and handle payments
But you've hired specialized pizza chefs to handle the cooking
When that big catering order arrives, you send it to your chefs
You keep serving other customers without missing a beat
That's exactly what worker threads do in Node.js. They let you delegate heavy tasks to separate "workers" while keeping your main application responsive.
When Should You Use Worker Threads? Real Examples:
1. Image Processing
Before: Your app lets users upload profile pictures, but resizing and optimizing them freezes the entire site for several seconds.
After: With worker threads, the main application keeps running smoothly while a worker thread handles the image processing in the background.
2. Reports Generation
Before: When a user generates a monthly sales report that analyzes thousands of transactions, your app becomes completely unresponsive.
After: Your main thread instantly acknowledges the report request, then a worker thread crunches the numbers while users continue browsing.
3. Search Functionality
Before: Searching through a large database makes your app stutter for everyone.
After: The search processing happens in a worker thread, keeping the UI snappy and responsive.
Let's Build Something Real: A Simple Worker Thread Example
Here's how to implement worker threads in a way that actually makes sense. We'll create a simple web server that can handle intensive calculations without freezing.
Step 1: Set Up Your Project
Create a new folder and initialize your project:
mkdir my-responsive-app
cd my-responsive-app
npm init -y
Step 2: Create Your Main Application File (app.js)
const http = require('http');
const { Worker } = require('worker_threads');
// Create a simple HTTP server
const server = http.createServer((req, res) => {
// This route will do heavy calculations
if (req.url === '/calculate') {
console.log('Calculation requested - sending to worker thread');
// Create a new worker thread
const worker = new Worker('./worker.js');
// Listen for the result from our worker
worker.on('message', (result) => {
res.writeHead(200, { 'Content-Type': 'application/json' });
res.end(JSON.stringify({ result }));
});
// Handle any errors
worker.on('error', (error) => {
res.writeHead(500, { 'Content-Type': 'application/json' });
res.end(JSON.stringify({ error: error.message }));
});
}
// This route stays super fast
else if (req.url === '/hello') {
res.writeHead(200, { 'Content-Type': 'text/plain' });
res.end('Hello, I respond instantly because I\'m not blocked!');
}
// Homepage
else {
res.writeHead(200, { 'Content-Type': 'text/html' });
res.end(`
<h1>Worker Threads Demo</h1>
<p>Try these links:</p>
<ul>
<li><a href="/calculate">Run heavy calculation</a> (processed in worker thread)</li>
<li><a href="/hello">Quick response</a> (shows main thread stays responsive)</li>
</ul>
`);
}
});
// Start the server
const PORT = 3000;
server.listen(PORT, () => {
console.log(`Server running at http://localhost:${PORT}`);
});
Step 3: Create Your Worker Thread File (worker.js)
const { parentPort } = require('worker_threads');
// This function simulates an intensive calculation
function doHeavyCalculation() {
console.log('Worker: Starting heavy calculation...');
// Simulate CPU-intensive work
let result = 0;
for (let i = 0; i < 5_000_000_000; i++) {
// This purposely inefficient loop will keep CPU busy
if (i % 1_000_000_000 === 0) {
console.log(`Worker: ${i/1_000_000_000}/5 billion iterations completed`);
}
result += Math.sqrt(i);
}
return {
result: result,
completedAt: new Date().toISOString()
};
}
// Start the heavy calculation
console.log('Worker: I was created to do the heavy lifting!');
const result = doHeavyCalculation();
// Send the result back to the main thread
parentPort.postMessage(result);
console.log('Worker: Done! Result sent back to main thread');
Common Mistakes and How to Avoid Them
Creating too many workers
Problem: Each worker consumes memory and resources
Solution: Consider using a worker pool (like
node:worker_threads
withpiscina
package)
Sending too much data between threads
Problem: Large data transfers between threads can slow things down
Solution: Only transfer the minimal data needed
Using workers for I/O tasks
Problem: Worker threads don't help much with I/O (file/network) operations
Solution: Use async/await and promises for I/O instead
When NOT to Use Worker Threads
Worker threads aren't always the answer. They're best for CPU-intensive work, but unnecessary for:
Database operations (use async/await instead)
API requests (use promises instead)
File operations (use the fs promises API instead)
Simple calculations (the overhead isn't worth it)
Next Steps: Level Up Your Worker Thread Skills
Ready to take your worker thread knowledge further? Try these challenges:
Create a worker pool to manage multiple workers
Implement two-way communication with your workers
Share memory between threads for better performance
Conclusion
Worker threads are your secret weapon for keeping Node.js applications fast and responsive when handling CPU-intensive tasks. By offloading heavy work to separate threads, you ensure your users never experience those frustrating freezes.
Have you implemented worker threads in your own projects? Share your experience in the comments below!
Subscribe to my newsletter
Read articles from Abigeal Afolabi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
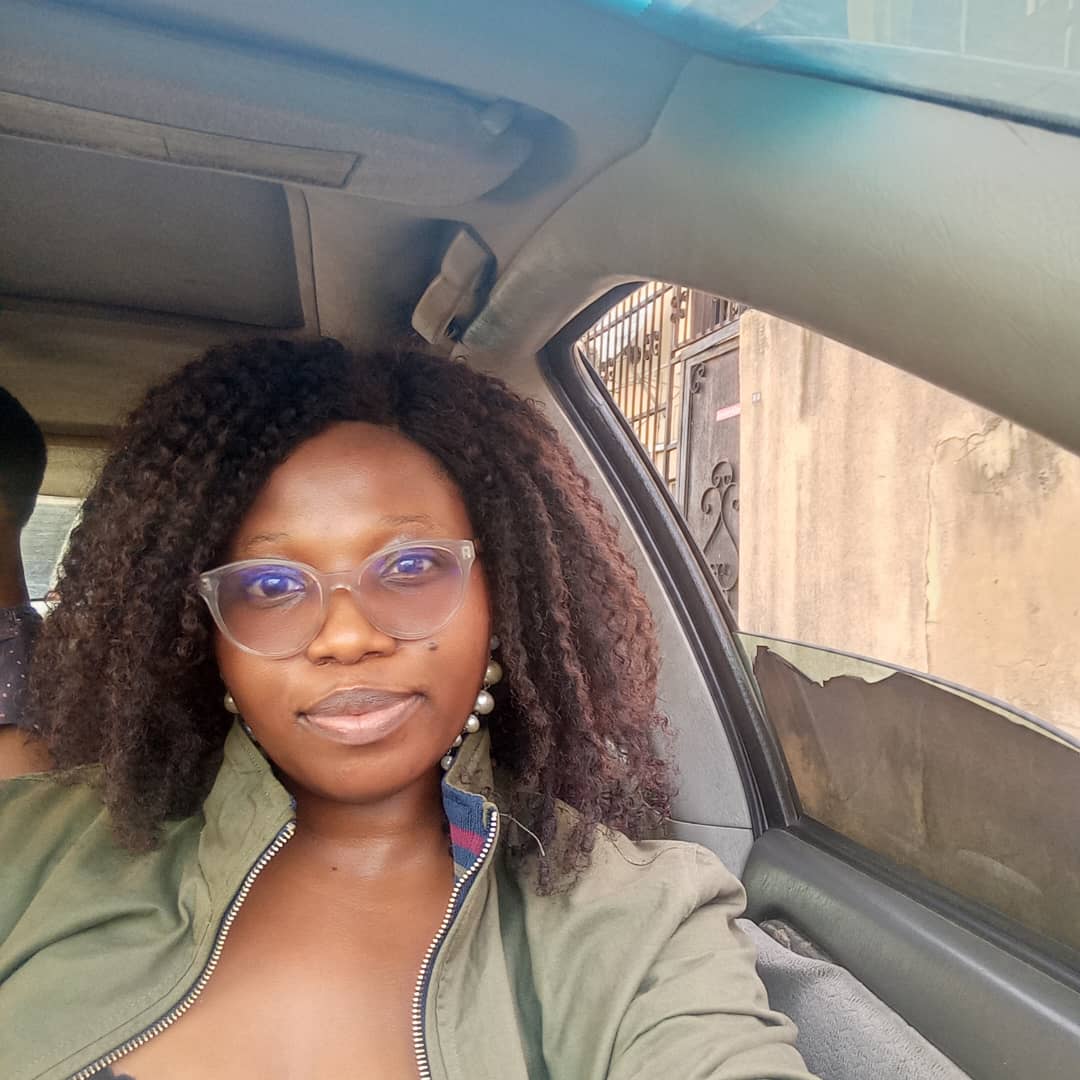
Abigeal Afolabi
Abigeal Afolabi
๐ Software Engineer by day, SRE magician by night! โจ Tech enthusiast with an insatiable curiosity for data. ๐ Harvard CS50 Undergrad igniting my passion for code. Currently delving into the MERN stack โ because who doesn't love crafting seamless experiences from front to back? Join me on this exhilarating journey of embracing technology, penning insightful tech chronicles, and unraveling the mysteries of data! ๐๐ง Let's build, let's write, let's explore โ all aboard the tech express! ๐๐ #CodeAndCuriosity