Automatically Generating Resolvers from a GraphQL Schema in Node.js
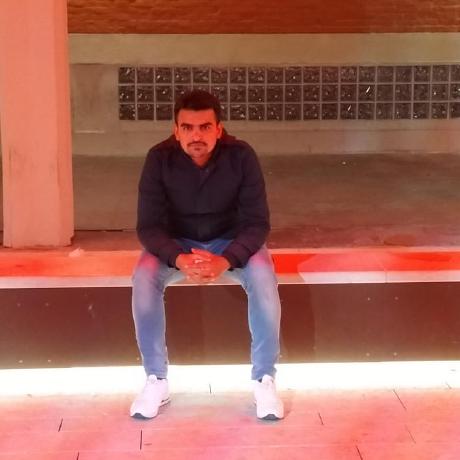

In modern API development, GraphQL has emerged as a powerful and flexible way to query and manipulate data. However, when implementing a GraphQL server, one of the most repetitive tasks is writing resolvers for each type and field in your schema. These resolvers define the behavior for fetching or modifying data when a query or mutation is executed. While this is a crucial part of setting up your GraphQL server, it can often become cumbersome, especially when dealing with large schemas.
Fortunately, there are ways to automate the generation of resolvers, saving time and reducing the potential for human error. This article will demonstrate how to automatically generate GraphQL resolvers from an existing GraphQL schema in a Node.js environment.
1. Prerequisites
Before proceeding, ensure the following prerequisites are met:
Node.js installed on your machine. You can download and install it from here.
A basic understanding of GraphQL, including schema definitions and resolvers.
Familiarity with Node.js and npm for dependency management.
If you're new to GraphQL, we recommend going through the official GraphQL documentation to get up to speed with the fundamentals.
2. Setting Up the Project
The first step in this process is to create a new Node.js project. Open a terminal and run the following commands:
mkdir graphql-resolver-generator
cd graphql-resolver-generator
npm init -y
This will initialize a new Node.js project with a package.json
file.
3. Installing Required Dependencies
To interact with GraphQL, we will need a few core dependencies:
graphql
: The core GraphQL library.apollo-server
: A popular GraphQL server implementation for Node.js.graphql-tools
: A utility to help manipulate and work with GraphQL schemas.
Install these dependencies by running the following command:
npm install graphql apollo-server graphql-tools
Next, we will need additional tools for generating resolvers from the schema. We'll use a simple approach to auto-generate basic resolvers using the schema definition.
To simplify the process, install a resolver generation utility such as graphql-resolvers
or create a custom utility script.
npm install graphql-resolvers
4. Generating Resolvers Automatically
The goal is to generate resolvers automatically based on your GraphQL schema. We will write a script that extracts the types from the schema and generates corresponding resolvers for each type.
Sample GraphQL Schema
First, define a simple GraphQL schema that we will use to demonstrate resolver generation. Create a file named schema.graphql
:
type Query {
getUser(id: ID!): User
getPost(id: ID!): Post
}
type User {
id: ID!
name: String
email: String
}
type Post {
id: ID!
title: String
content: String
author: User
}
Script to Generate Resolvers
Create a file generateResolvers.js
where we will automatically generate resolvers based on the schema. Below is a simple script that generates resolvers for the Query
type and each field in the User
and Post
types:
const { makeExecutableSchema } = require('graphql-tools');
const { readFileSync } = require('fs');
// Load the schema from the file
const schemaString = readFileSync('./schema.graphql', 'utf-8');
// Create an executable schema
const schema = makeExecutableSchema({ typeDefs: schemaString });
// Function to generate resolvers
const generateResolvers = (schema) => {
const resolvers = {};
// Loop through each type in the schema
schema.getTypeMap().forEach((type) => {
if (type.astNode.kind === 'ObjectTypeDefinition') {
resolvers[type.name] = {};
// Generate resolvers for each field in the type
type.fields.forEach((field) => {
resolvers[type.name][field.name] = (parent, args, context, info) => {
console.log(`Fetching ${field.name} for ${type.name}`);
return null; // For simplicity, returning null
};
});
}
});
return resolvers;
};
// Generate resolvers
const resolvers = generateResolvers(schema);
console.log(resolvers);
This script does the following:
Loads the GraphQL schema from a
.graphql
file.Generates an executable schema using
graphql-tools
.Iterates over the schema types and generates default resolvers for each field.
Prints the resolvers to the console.
5. Testing the Implementation
Once the script is ready, you can test the automatic generation of resolvers by running the following command:
node generateResolvers.js
This will log the generated resolvers for each field in your schema. For instance, it will generate a resolver for getUser
under the Query
type, and resolvers for id
, name
, and email
under the User
type.
You can now integrate these resolvers with your GraphQL server implementation. For instance, you could combine them with Apollo Server:
const { ApolloServer } = require('apollo-server');
// Create an Apollo Server instance
const server = new ApolloServer({
typeDefs: schemaString,
resolvers: generateResolvers(schema),
});
server.listen().then(({ url }) => {
console.log(`Server ready at ${url}`);
});
6. Conclusion
Automatically generating GraphQL resolvers from your schema can significantly speed up development and reduce the chances of introducing errors, especially when dealing with large or evolving schemas. By leveraging the graphql-tools
package and some custom logic, you can quickly generate basic resolvers, allowing you to focus more on business logic and data interactions.
While this approach covers basic use cases, it can be extended further. You could, for example, integrate more advanced features such as authentication, authorization, or more sophisticated resolver functions that interact with databases or external APIs.
This method can be applied to various GraphQL projects and is a great starting point for automating the GraphQL development process in a Node.js environment.
Subscribe to my newsletter
Read articles from Ahmed Raza directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
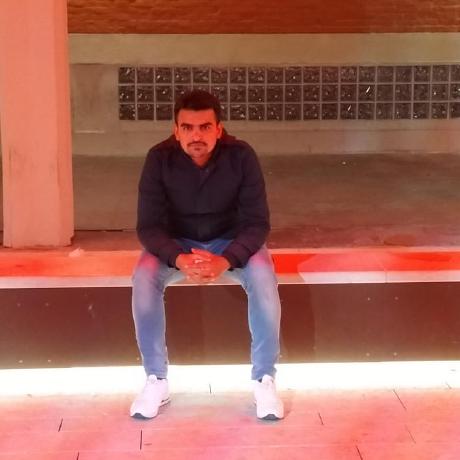
Ahmed Raza
Ahmed Raza
Ahmed Raza is a versatile full-stack developer with extensive experience in building APIs through both REST and GraphQL. Skilled in Golang, he uses gqlgen to create optimized GraphQL APIs, alongside Redis for effective caching and data management. Ahmed is proficient in a wide range of technologies, including YAML, SQL, and MongoDB for data handling, as well as JavaScript, HTML, and CSS for front-end development. His technical toolkit also includes Node.js, React, Java, C, and C++, enabling him to develop comprehensive, scalable applications. Ahmed's well-rounded expertise allows him to craft high-performance solutions that address diverse and complex application needs.