Techniques to Decide: Is Java or Kotlin the Right Choice for Your Project?
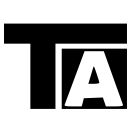
4 min read
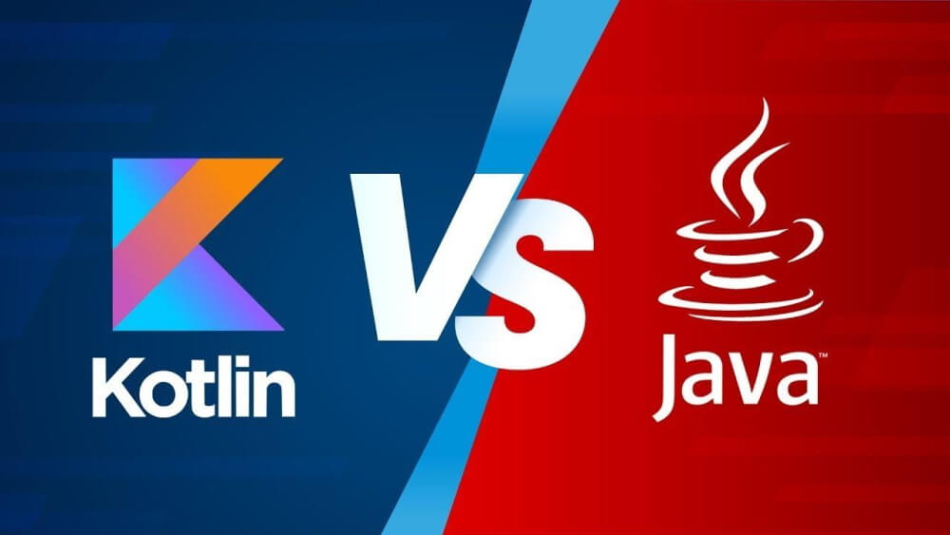
1. Language Fundamentals
Understanding the core aspects of each language is essential to gauge which might be more suitable for your project.
1.1 Syntax and Readability
- Java: Known for its verbosity, Java has a more extensive syntax, which can lead to larger codebases.
- Kotlin: Offers a concise syntax, reducing boilerplate code significantly. Here’s a simple comparison:
// Java
public class Person {
private String name;
public Person(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
// Kotlin
class Person(val name: String)
1.2 Null Safety
- Java: Lacks built-in null safety, which can lead to NullPointerExceptions.
- Kotlin: Introduces null safety directly in the language, significantly reducing the likelihood of runtime errors related to null values.
// Kotlin example
var name: String? = null
println(name?.length) // Safe call, no exception
1.3 Data Classes
- Java: Requires explicit code for getters, setters, equals(), hashCode(), and toString() methods.
- Kotlin: Provides data classes, which automatically generate these methods, simplifying the code:
data class User(val name: String, val age: Int)
1.4 Compatibility and Interoperability
- Java: Can work with any JVM-compatible language.
- Kotlin: Fully interoperable with Java, allowing you to call Java code from Kotlin and vice versa. This feature is advantageous when migrating or gradually integrating Kotlin into an existing Java codebase.
2. Performance and Efficiency
Evaluating performance aspects is key to making an informed decision. Both languages compile to JVM bytecode, so the performance is often similar. However, there are distinctions.
2.1 Compilation Speed
- Java: Tends to compile faster than Kotlin, especially on larger projects. This can be important for CI/CD pipelines where build times impact deployment.
- Kotlin: While slower in compilation, incremental compilation has improved, especially for Android.
2.2 Runtime Performance
Java and Kotlin: Both languages typically have comparable runtime performance since they compile down to bytecode. However, Kotlin's additional features (such as lambdas and coroutines) can introduce slight overhead but often optimize code readability and maintainability.
2.3 Coroutines and Concurrency
- Java: Relies on traditional threading and the new java.util.concurrent package for concurrency.
- Kotlin: Introduces coroutines, enabling efficient, lightweight, and more readable concurrency:
// Kotlin coroutine example
GlobalScope.launch {
delay(1000L)
println("Coroutine!")
}
3. Ecosystem and Tooling
3.1 Android Development
- Java: Initially, the official language for Android, with a mature ecosystem and extensive resources.
- Kotlin: Now preferred for Android, it is officially recommended by Google and offers concise syntax and modern features, making it popular for mobile app development.
3.2 Server-Side Development
- Java: Dominates the server-side domain, with mature frameworks like Spring, Hibernate, and a vast developer community.
- Kotlin: Fully compatible with Java frameworks, allowing its use in Spring Boot, Ktor, and other frameworks. Kotlin also provides a more modern approach to server-side programming.
3.3 Tooling and IDE Support
- Java: Has robust support across all major IDEs, with tools specifically tailored to Java development.
- Kotlin: Initially best supported in IntelliJ IDEA, but support has expanded significantly, including Android Studio.
4. Community and Learning Curve
4.1 Java Community
Java has a vast developer community with ample resources, libraries, frameworks, and documentation. This makes it easier to find solutions and community support.
4.2 Kotlin Community
While smaller, the Kotlin community is rapidly growing. Kotlin’s primary documentation and resources are well-maintained, and its modern features often make it easier to learn for beginners familiar with Java.
5. Conclusion
Both Java and Kotlin have their advantages. Java’s established ecosystem and larger community make it ideal for long-term, enterprise-level projects with extensive requirements. On the other hand, Kotlin’s concise syntax, null safety, and modern features make it an attractive choice for Android development and newer JVM projects. Ultimately, the best choice depends on your project's specific requirements and the team’s familiarity with the language.
If you have questions or need further clarification on which language suits your project, feel free to comment below!
Read more at : Techniques to Decide: Is Java or Kotlin the Right Choice for Your Project?
0
Subscribe to my newsletter
Read articles from Tuanhdotnet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
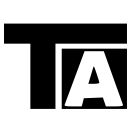
Tuanhdotnet
Tuanhdotnet
I am Tuanh.net. As of 2024, I have accumulated 8 years of experience in backend programming. I am delighted to connect and share my knowledge with everyone.