Create a Scalable Web App with Docker Compose in Under 5 Minutes
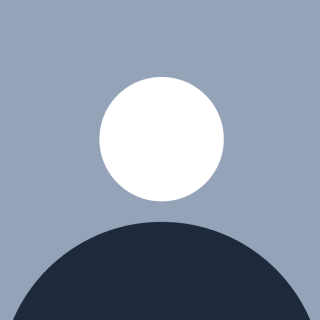
Hey devs! If you've ever found yourself juggling multiple docker run
commands or struggling to connect services together in development, Docker Compose is your new best friend.
In this post, I’ll walk you through:
What Docker Compose is
Why it's a game-changer
A hands-on demo using Flask + Redis
How to run it locally in 3 simple steps
Let’s dive in! 🐳
🧠 What is Docker Compose?
Docker Compose is a tool that allows you to define and run multi-container Docker applications using a YAML file.
Rather than starting each container individually with docker run
, you define all services (like web apps, databases, and caches) in one file—then start everything with a single command:
docker-compose up
That’s it. Your entire app spins up.
💡 Perfect for:
Web apps that use backend + database + cache
Microservices
Development and testing environments
🧠 Why Use Docker Compose?
✅ One command to run everything
✅ Simple service linking (like Flask talking to Redis or MySQL)
✅ Easier sharing and collaboration
✅ Reproducible environments
📦 Project Overview
We’ll create:
A Flask app that shows how many times it has been visited
A Redis cache that stores the count
🧱 Project Structure
myapp/
├── app.py
├── requirements.txt
├── Dockerfile
└── docker-compose.yml
🧾 Step 1: Flask App Code
Create a file named app.py
:
from flask import Flask
import redis
app = Flask(__name__)
cache = redis.Redis(host='redis', port=6379)
@app.route('/')
def hello():
count = cache.incr('hits')
return f'Hello! You have visited this page {count} times.\n'
if __name__ == "__main__":
app.run(host="0.0.0.0", port=5000)
🔍 This does 3 things:
Connects to Redis (
host='redis'
)Increments a key
hits
Returns how many times the page is visited
📦 Step 2: Add Requirements
Create a file named requirements.txt
:
flask
redis
🐋 Step 3: Create Dockerfile
FROM python:3.9-alpine
WORKDIR /app
COPY . .
RUN pip install -r requirements.txt
CMD ["python", "app.py"]
This builds your Flask app into a Docker container using a lightweight Python image.
⚙️ Step 4: Create docker-compose.yml
version: "3.9"
services:
web:
build: .
ports:
- "5000:5000"
depends_on:
- redis
redis:
image: "redis:alpine"
web
is your Flask appredis
is a Redis cache pulled from Docker Hubdepends_on
ensures Redis starts before Flask tries to connect
🚀 Run the App
Step-by-step:
Open terminal and navigate to the project directory
cd myapp
Run Docker Compose
docker-compose up --build
Open in your browser:
http://localhost:5000
You’ll see:
Hello! You have visited this page 1 times.
Keep refreshing! Redis increments the counter.
🧪 Bonus: Tear Down the App
To stop and remove containers:
docker-compose down
To stop and delete containers, volumes, and networks:
docker-compose down -v
💡 What You Learned
✅ How Docker Compose links multiple services
✅ Creating and connecting a Flask app with Redis
✅ Dockerizing and running everything with a single command
🛠️ Ideas to Extend This Demo
Add PostgreSQL or MySQL to store persistent data
Use Docker Volumes to persist Redis across reboots
Deploy the app on a cloud VPS using Docker Compose
📈 Want to Boost Your DevOps Skills?
If you liked this tutorial, follow me for:
More beginner-friendly Docker guides
End-to-end DevOps project walkthroughs
Deployment, CI/CD, and cloud tutorials
💬 Got stuck somewhere? Drop your questions in the comments — I reply fast!
📣 Before You Go...
👉 Share this with a friend who's learning Docker
👉 Bookmark this blog for your future reference
👉 Follow me on Hashnode for upcoming content
Subscribe to my newsletter
Read articles from Loga Rajeshwaran Karthikeyan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by