Deploying Your First ML Model with Flask: A No-Jargon Guide
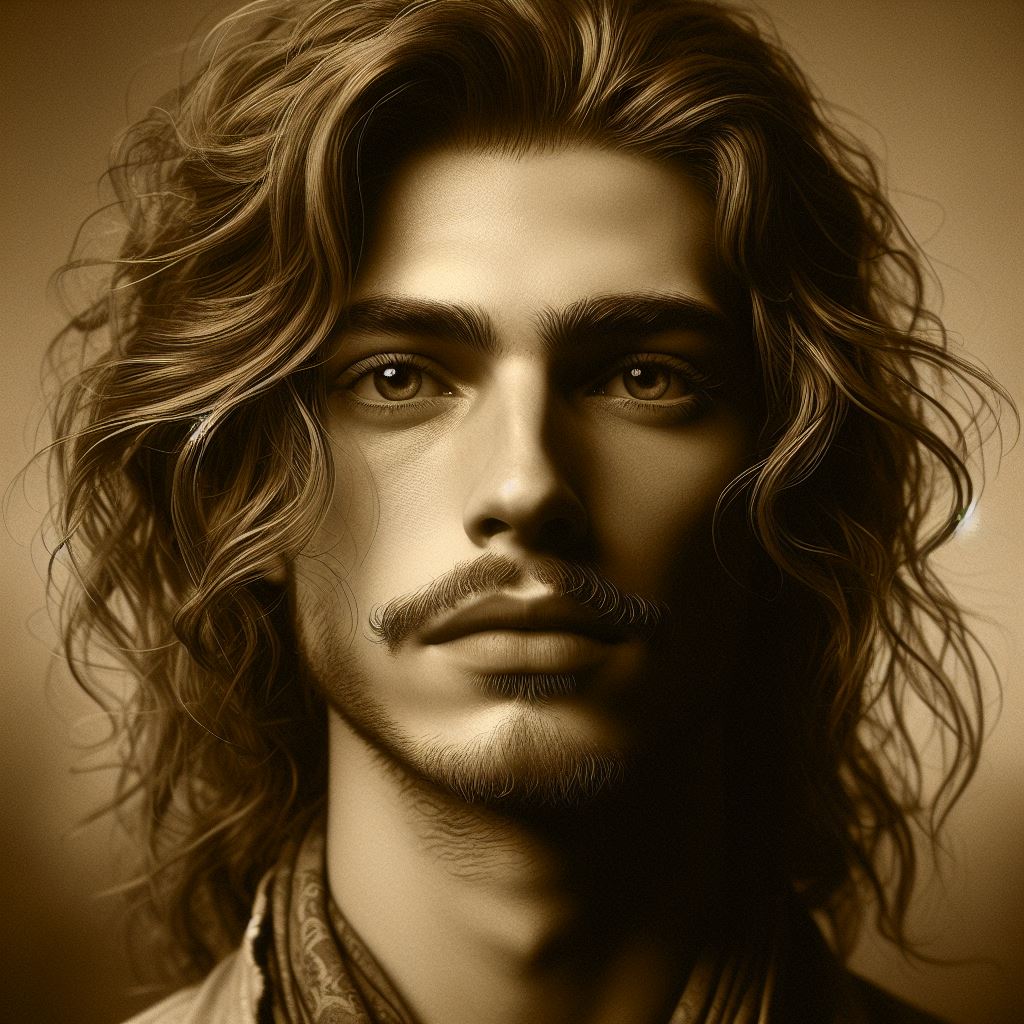

So you've trained your first machine learning model and it’s chilling in your Jupyter Notebook. But now what? How do you actually use it in real life?
Don’t worry—this post will walk you through the simplest way to turn your ML model into something the world can use using Flask, a lightweight web framework for Python. No buzzwords. No corporate lingo. Just pure magic (and a bit of code).
What We’re Building
We’ll create a mini web app that:
Takes input from a user
Uses your trained ML model to make a prediction
Shows the result back to the user
Step 1: Train a Simple ML Model
Let’s use a quick model with sklearn
to keep things smooth. You can replace this later with your own model.
# train_model.py
from sklearn.datasets import load_iris
from sklearn.linear_model import LogisticRegression
import pickle
# Load sample data
iris = load_iris()
X, y = iris.data, iris.target
# Train a simple model
model = LogisticRegression()
model.fit(X, y)
# Save it to disk
with open('model.pkl', 'wb') as file:
pickle.dump(model, file)
✅ Run this once. It saves model.pkl
, which we’ll use in our Flask app.
🌐 Step 2: Create a Flask App
Now, let's bring Flask into the picture.
# app.py
from flask import Flask, request, jsonify, render_template
import pickle
import numpy as np
# Load model
model = pickle.load(open('model.pkl', 'rb'))
# Create app
app = Flask(__name__)
@app.route('/')
def home():
return render_template('index.html') # Simple form to take input
@app.route('/predict', methods=['POST'])
def predict():
try:
# Get input from form
input_values = [float(x) for x in request.form.values()]
final_input = [np.array(input_values)]
# Make prediction
prediction = model.predict(final_input)
return render_template('index.html', prediction_text=f'Predicted Class: {prediction[0]}')
except:
return render_template('index.html', prediction_text="⚠️ Invalid input. Please try again.")
if __name__ == "__main__":
app.run(debug=True)
🧾 Step 3: Add an HTML Frontend
Create a folder named templates
and add this file inside:
templates/index.html
<!DOCTYPE html>
<html>
<head>
<title>ML Predictor</title>
<style>
body {
font-family: Arial;
background-color: #f0f2f5;
padding: 50px;
}
.container {
background: white;
padding: 20px;
border-radius: 10px;
width: 300px;
margin: auto;
box-shadow: 0 0 10px rgba(0,0,0,0.1);
}
input {
width: 100%;
margin: 8px 0;
padding: 8px;
}
button {
background: #007bff;
color: white;
border: none;
padding: 10px;
width: 100%;
border-radius: 5px;
cursor: pointer;
}
</style>
</head>
<body>
<div class="container">
<h2>Enter Features</h2>
<form action="/predict" method="POST">
<input type="text" name="feature1" placeholder="Feature 1" required>
<input type="text" name="feature2" placeholder="Feature 2" required>
<input type="text" name="feature3" placeholder="Feature 3" required>
<input type="text" name="feature4" placeholder="Feature 4" required>
<button type="submit">Predict</button>
</form>
<h3>{{ prediction_text }}</h3>
</div>
</body>
</html>
⚙️ Step 4: Run the App
n your terminal, run:
python app.py
Open http://127.0.0.1:5000/
in your browser, enter your inputs, and BOOM 💥—you’ll see your model's prediction!
Example Test Inputs
Try these:
5.1, 3.5, 1.4, 0.2
→ Likely Class 06.7, 3.1, 4.4, 1.4
→ Likely Class 17.2, 3.6, 6.1, 2.5
→ Likely Class 2
Bonus: Convert it to an API
Want to use it from JavaScript or another app? Just add this route:
@app.route('/api', methods=['POST'])
def api():
data = request.get_json(force=True)
prediction = model.predict([np.array(list(data.values()))])
return jsonify({'prediction': int(prediction[0])})
Then you can do:
curl -X POST http://127.0.0.1:5000/api -H "Content-Type: application/json" -d '{"feature1":5.1,"feature2":3.5,"feature3":1.4,"feature4":0.2}'
Next Steps
You’ve now deployed your first ML model locally. You can:
💬 Final Thoughts
This isn’t just about ML. It’s about shipping your ideas.
You trained a model. You wrapped it in Flask. You made it usable.
Stay curious. Stay shipping. 🚀
Subscribe to my newsletter
Read articles from Sanchit Agrawal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
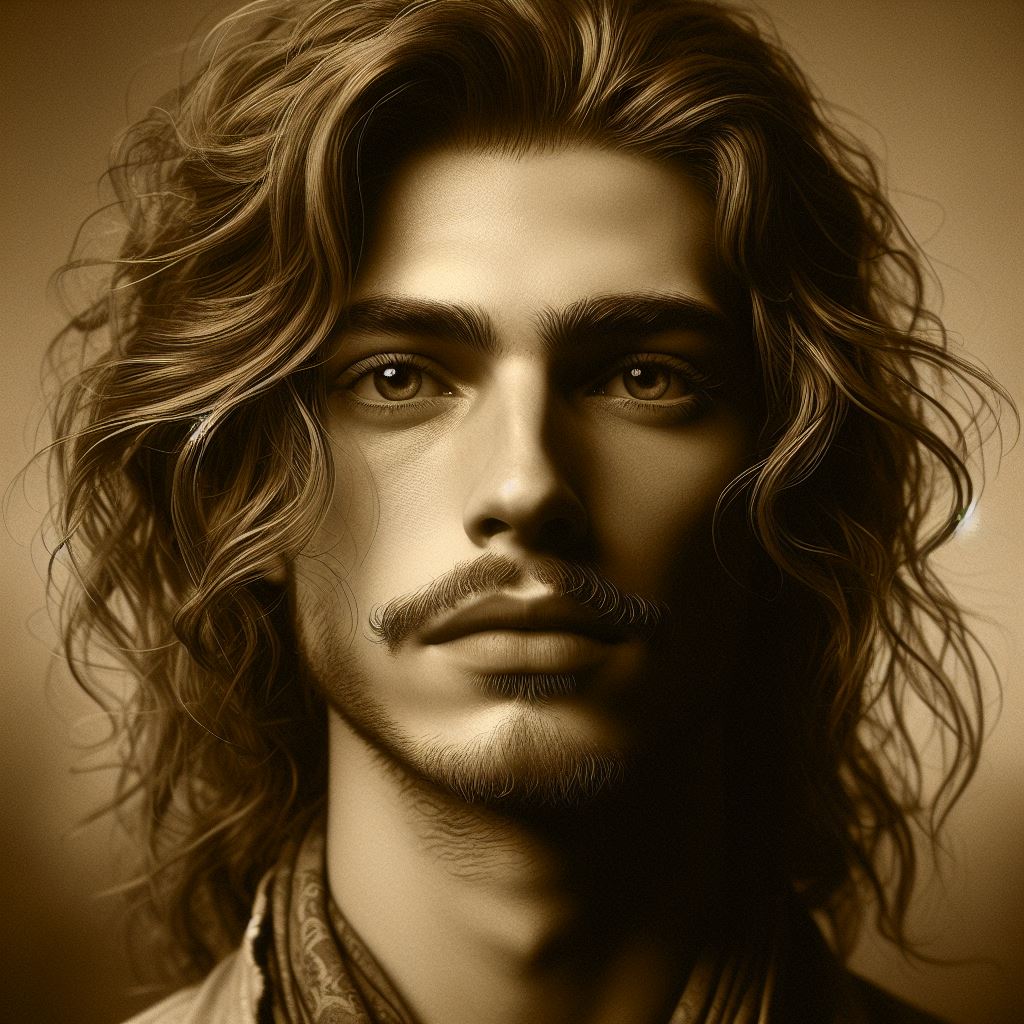
Sanchit Agrawal
Sanchit Agrawal
I am a dedicated learner who is eager to acquire knowledge in new technologies.