Using Java Reflection: When, Why, and How It’s Beneficial
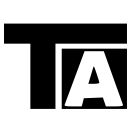
4 min read
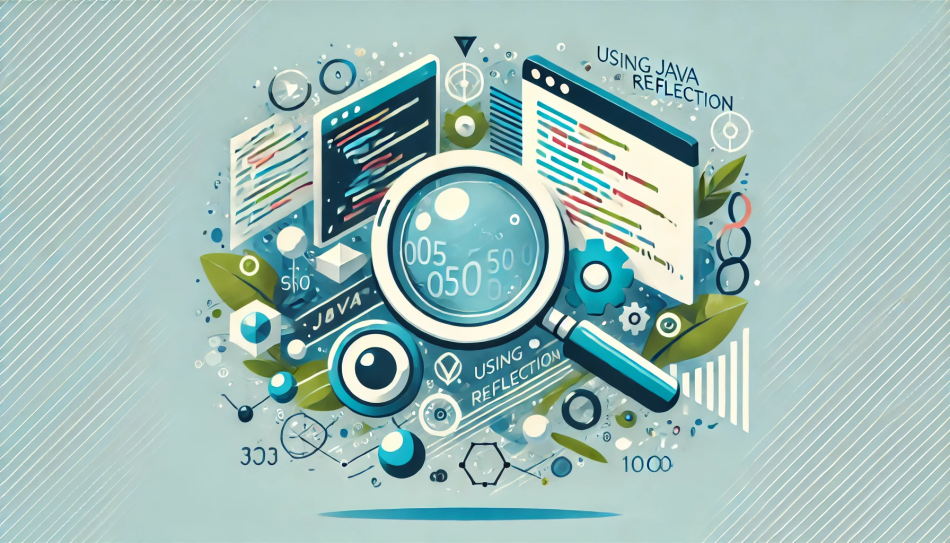
1. What is Java Reflection?
1.1 Definition and Purpose
Explain Java Reflection as the ability to inspect and modify the runtime behavior of applications. Describe how it provides runtime access to information about classes, methods, fields, and interfaces.
1.2 How Does Reflection Work?
Discuss the underlying mechanics of Reflection in Java, focusing on the java.lang.reflect package. Explain with a simple code example:
import java.lang.reflect.Method;
public class ReflectionDemo {
public static void main(String[] args) {
try {
Class<?> clazz = Class.forName("java.util.ArrayList");
Method[] methods = clazz.getDeclaredMethods();
for (Method method : methods) {
System.out.println("Method Name: " + method.getName());
}
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
}
The output lists all methods of the ArrayList class. This example helps demonstrate Reflection’s core ability to inspect class details at runtime.
1.3 Key Reflection Classes and Interfaces
Provide an overview of the Class, Method, Field, and Constructor classes, including examples showing how each is used.
2. When and Why to Use Java Reflection
2.1 Dynamic Class Loading
Discuss scenarios where dynamic class loading is required, such as plugins or custom scripts that need to load classes without explicitly knowing their details at compile time.
Code Example: Show how Reflection enables dynamic loading and instantiation of classes based on configuration files.
2.2 Accessing Private Fields and Methods for Testing
Explain how Reflection is useful in testing by accessing private fields and methods for unit testing purposes.
import java.lang.reflect.Field;
public class PrivateFieldAccess {
private String secret = "Hidden Message";
public static void main(String[] args) {
try {
PrivateFieldAccess obj = new PrivateFieldAccess();
Field field = PrivateFieldAccess.class.getDeclaredField("secret");
field.setAccessible(true);
System.out.println("Secret: " + field.get(obj));
} catch (Exception e) {
e.printStackTrace();
}
}
}
Result: Outputs the value of a private field. This example demonstrates how Reflection helps bypass access restrictions during testing, a common Reflection use case.
2.3 Framework Development
Discuss how frameworks like Spring and Hibernate rely on Reflection to perform Dependency Injection (DI) and Object-Relational Mapping (ORM).
Explanation: Explain DI and ORM briefly and how Reflection makes these powerful techniques possible.
3. Reflection Considerations and Performance Impact
3.1 Performance Overhead
Reflection is slower than direct code execution. Explain the impact with benchmarks (can be hypothetical, or use an external chart).
Example Code: Compare the execution time of a regular method call with an equivalent Reflection-based call.
3.2 Security Implications
Reflection can bypass access modifiers, potentially exposing sensitive data. Discuss security best practices and how to mitigate Reflection misuse.
Tips for Secure Reflection: Only use Reflection when necessary, sanitize input when dealing with user-defined classes, etc.
3.3 When Not to Use Reflection
Summarize the scenarios where Reflection might not be appropriate due to performance, readability, or maintainability issues.
4. Practical Examples of Java Reflection in Real-World Applications
4.1 Dependency Injection Example
Demonstrate how a basic Dependency Injection framework could be built using Reflection. Show code that automatically injects dependencies into fields marked with a custom annotation.
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.FIELD)
public @interface Inject {}
public class DIContainer {
public static void initialize(Object obj) throws Exception {
for (Field field : obj.getClass().getDeclaredFields()) {
if (field.isAnnotationPresent(Inject.class)) {
field.setAccessible(true);
field.set(obj, field.getType().newInstance());
}
}
}
}
Explanation: This demonstrates a simplified version of DI used in frameworks like Spring.
4.2 Building a Simple Object Mapper
Illustrate how to use Reflection to create a simple object mapper that converts data from a JSON-like structure to an object.
public class ObjectMapper {
public <T> T mapToObject(Map<String, Object> data, Class<T> clazz) throws Exception {
T obj = clazz.getDeclaredConstructor().newInstance();
for (Field field : clazz.getDeclaredFields()) {
field.setAccessible(true);
field.set(obj, data.get(field.getName()));
}
return obj;
}
}
Result and Use Case: Describe how this can be applied in JSON parsing frameworks or ORM frameworks, showcasing the versatility of Reflection in data mapping.
5. Conclusion
Summarize the main points and emphasize best practices. Encourage readers to use Reflection responsibly and understand its impact.
Got questions? Feel free to ask in the comments below!
Read more at : Using Java Reflection: When, Why, and How It’s Beneficial
0
Subscribe to my newsletter
Read articles from Tuanhdotnet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
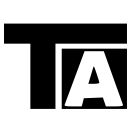
Tuanhdotnet
Tuanhdotnet
I am Tuanh.net. As of 2024, I have accumulated 8 years of experience in backend programming. I am delighted to connect and share my knowledge with everyone.