Unit– V Graphics with Turtle
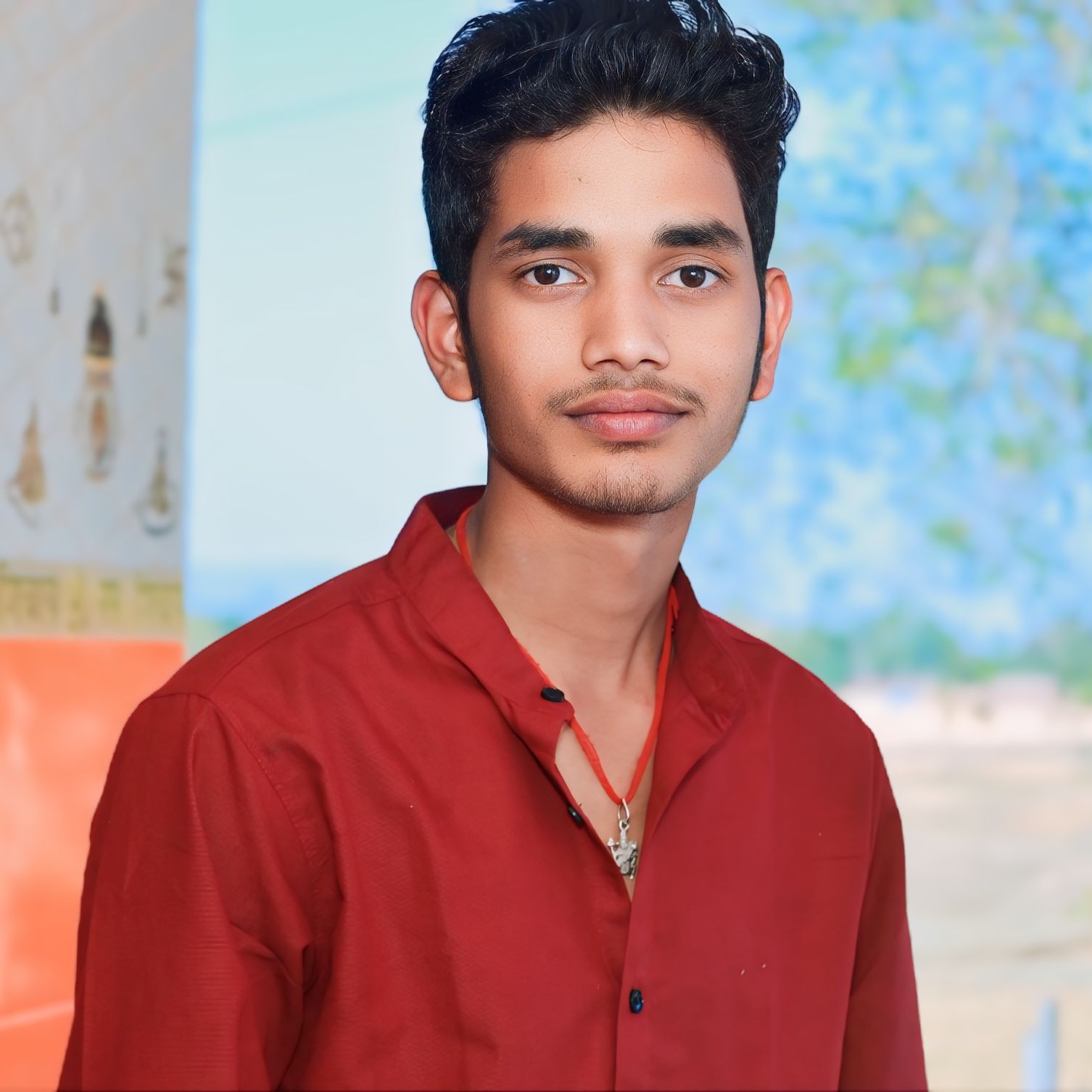

Introduction to Turtle Graphics
Turtle graphics is a popular and beginner-friendly way to learn programming, especially for younger audiences or those new to coding. It provides a simple environment where users can control a "turtle" (a graphical cursor) that moves around the screen, drawing lines and shapes as it moves. The turtle responds to a set of commands in the Python programming language, such as moving forward, turning, and changing the pen's state.
By using turtle graphics, individuals can visually understand concepts like loops, functions, and conditionals, while also creating drawings ranging from basic geometric shapes to complex patterns and designs. This combination of interactivity and creativity makes turtle graphics an engaging way to introduce programming concepts.
Turtles are just Python objects, so you can use any Python constructs in turtle programs: selection, loops, recursion, etc.
Turtles are objects that move about on a screen (window). Various methods allow you to direct the turtle’s movement.
The turtle screen can be thought of as a reference to the Cartesian plane in many ways. The turtle screen is a two-dimensional space where the Cartesian plane principles are applied, allowing users to control and visualize movement and drawing through coordinates.
5.2 Turtle Methods
Turtle graphics offer several useful methods that control the turtle's movement, drawing, and appearance. Below are some common methods:
5.2.1 Turtle Methods
forward(distance): Moves the turtle forward by the specified distance.
backward(distance): Moves the turtle backward by the specified distance.
right(angle): Turns the turtle right by the given angle in degrees.
left(angle): Turns the turtle left by the given angle in degrees.
penup(): Lifts the pen off the screen so the turtle doesn't leave a trail.
pendown(): Lowers the pen, so the turtle starts drawing.
setposition(x, y): Moves the turtle to the specified position (x, y).
color(colorname): Changes the color of the turtle's pen.
begin_fill(): Starts filling a shape with color.
end_fill(): Ends the filling of a shape.
Example: Moving the turtle.
import turtle
t = turtle.Turtle()
t.forward(100) # Move the turtle forward by 100 units
t.left(90) # Turn the turtle left by 90 degrees
t.forward(100) # Move the turtle forward again
5.2.2 Turtle Screen Methods
Turtle also provides methods to control the screen itself, such as setting its background color, size, title, and more.
Some common screen methods include:
bgcolor(color): Sets the background color of the screen.
title(title): Sets the title of the turtle graphics window.
screensize(width, height): Sets the size of the turtle graphics window.
exitonclick(): Closes the window when the user clicks on it.
import turtle
screen = turtle.Screen()
screen.bgcolor("lightblue") # Change background color
screen.title("Trial Graphics") # Set window title
screen.exitonclick() # Close the window on click
5.3 Using Loops and Conditional Statements to Draw Graphics
Loops and conditional statements are key to making turtle graphics more dynamic and interactive. Loops help repeat certain movements or shapes, and conditionals can be used to control the turtle's behavior based on certain conditions.
5.3.1 Turtle Programming: Loops and Conditional Statements
Using Loops:
Loops are used to repeat a set of commands multiple times. For example, drawing a square involves turning and moving the turtle in a specific pattern, which can be achieved using a for loop.
Example: Drawing a square using a loop
import turtle
t = turtle.Turtle()
for _ in range(4): # Repeat 4 times
t.forward(100) # Move forward by 100 units
t.left(90) # Turn left by 90 degrees
Using Conditional Statements:
Conditional statements (if, else, elif) can be used to control the flow of the program. For instance, you can make the turtle draw a shape only if a certain condition is met.
Example: Drawing different shapes based on the user's input
import turtle
t = turtle.Turtle()
shape = input("Enter a shape (square/triangle): ")
if shape == "square":
for _ in range(4):
t.forward(100)
t.left(90)
elif shape == "triangle":
for _ in range(3):
t.forward(100)
t.left(120)
else:
print("Unknown shape")
》Example of how to draw a smiley face with turtle graphics:
import turtle
def draw_circle(radius, color, x, y):
t.penup()
t.goto(x, y)
t.pendown()
t.begin_fill()
t.color(color)
t.circle(radius)
t.end_fill()
def draw_smile(radius, angle, x, y):
t.penup()
t.goto(x, y)
t.setheading(-60)
t.pendown()
t.circle(radius, angle)
Set up the screen
screen = turtle.Screen()
screen.bgcolor("white")
Create a turtle
t = turtle.Turtle()
t.speed(10)
Draw face, eyes, and smile using functions
draw_circle(100, "yellow", 0, -100) # Face
draw_circle(10, "black", -40, 30) # Left eye
draw_circle(10, "black", 40, 30) # Right eye
draw_smile(50, 120, -40, -20) # Smile
t.hideturtle()
screen.exitonclick()
Explanation:
Functions:
draw_circle: Draws a filled circle with specified radius, color, and position.
draw_smile: Draws a smile (arc) with a given radius and angle.
Main Drawing:
A yellow circle is drawn for the face.
Two black circles are drawn for the eyes.
A curved smile is drawn as an arc.
Execution:
- The turtle window stays open until clicked with screen.exitonclick().
》Example to creating a simple chessboard using Python's turtle module is a great way to practice drawing with code. Below is an example of how you can draw an 8x8 chessboard with alternating black and white squares.
import turtle
# Set up the screen
screen = turtle.Screen()
screen.bgcolor("white")
# Create the turtle
chessboard = turtle.Turtle()
chessboard.speed(0)
chessboard.penup()
# Chessboard size and square size
board_size = 8
square_size = 50 # Size of each square on the chessboard
# Function to draw a single square
def draw_square(color, x, y):
chessboard.goto(x, y)
chessboard.pendown()
chessboard.begin_fill()
chessboard.fillcolor(color)
for _ in range(4):
chessboard.forward(square_size)
chessboard.left(90)
chessboard.end_fill()
chessboard.penup()
# Draw the chessboard
def draw_chessboard():
start_x = -square_size * (board_size // 2)
start_y = square_size * (board_size // 2)
# Loop through rows and columns to draw the squares
for row in range(board_size):
for col in range(board_size):
# Determine the color of the square
if (row + col) % 2 == 0:
color = "white"
else:
color = "black"
x = start_x + col * square_size
y = start_y - row * square_size
draw_square(color, x, y)
# Call the function to draw the chessboard
draw_chessboard()
# Hide the turtle after drawing
chessboard.hideturtle()
# Keep the window open
turtle.done()
Explanation:
Chessboard Size: The chessboard is an 8x8 grid of squares. Each square is 50x50 pixels.
Looping: The code uses two loops to go through each row and column, determining whether each square should be black or white by checking the sum of the row and column indices (if even, white; if odd, black).
Drawing: The draw_square function is used to draw each individual square at the correct position on the screen.
When you run this code, you'll see an 8x8 chessboard with alternating black and white squares. This is a great starting point for creating a more interactive chess game if you wanted to add pieces or make it functional.
Subscribe to my newsletter
Read articles from Sagar Sangam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
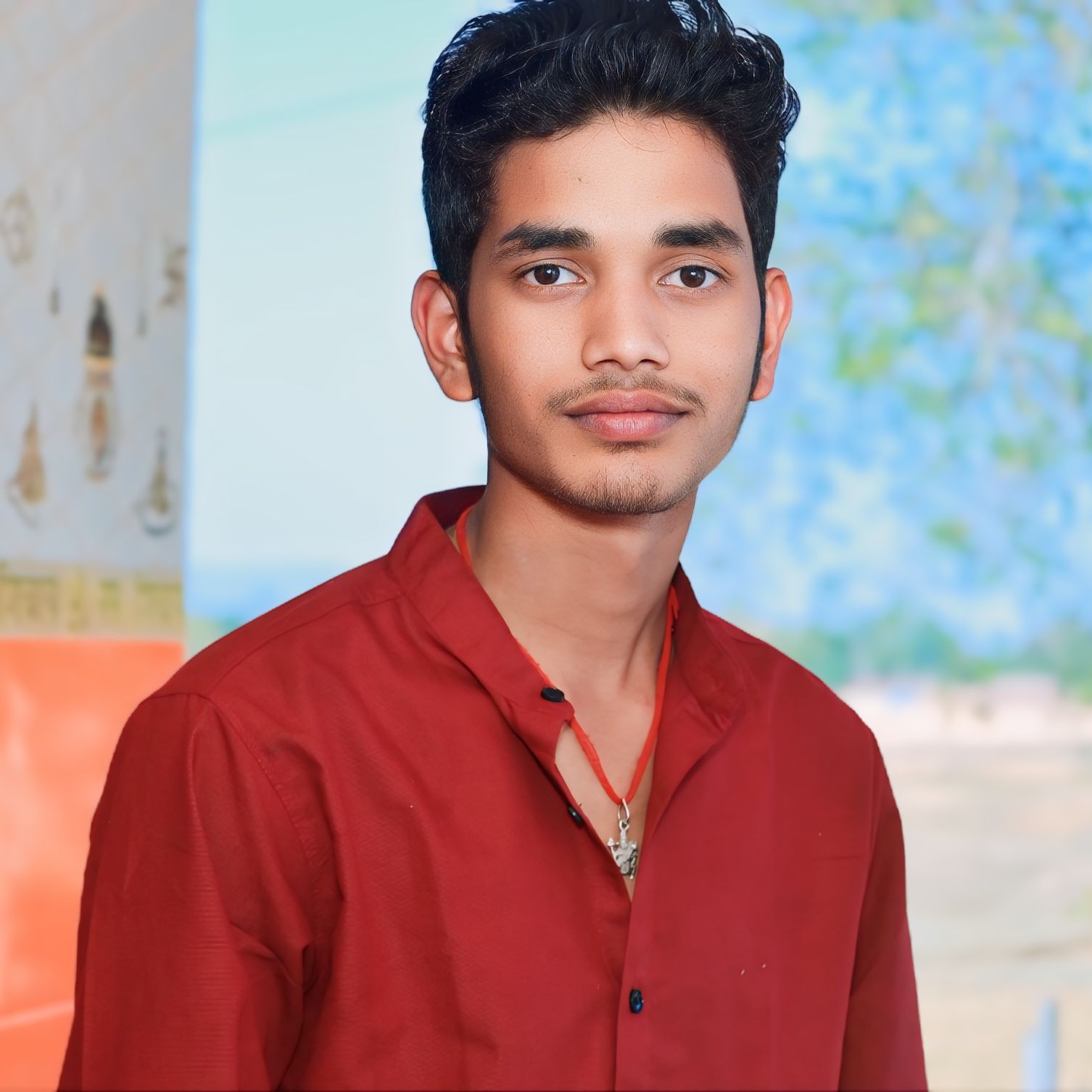