Alerting for AWS EC2 Instances Not Managed by SSM Using AWS Config and CDK
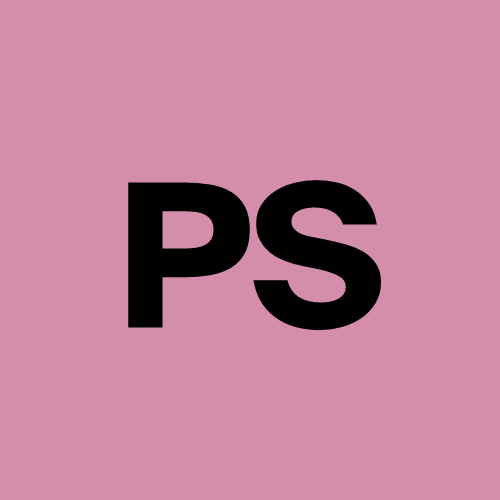

Monitoring your EC2 instances to ensure they’re managed by AWS Systems Manager (SSM) is crucial for security, compliance, and smooth operations. In this post, we’ll explain why SSM-managed instances are so important, how instances can become “unmanaged,” and walk through a step-by-step guide to automatically alert you about any EC2 instance that isn’t managed by SSM. We’ll use a combination of AWS Config, Amazon EventBridge, AWS Lambda, and Amazon SES – all provisioned with the AWS CDK in TypeScript – to build an automated compliance alert system. By the end, you’ll have a CDK stack that detects non-compliant EC2 instances and emails you their details, helping you uphold DevSecOps best practices and maintain AWS environment hygiene.
Why Ensure EC2 Instances Are Managed by SSM
AWS Systems Manager (SSM) is a service that allows you to automate operational tasks, manage instances at scale, and perform detailed system-level monitoring for EC2 instances . When your instances are SSM-managed (often called “managed instances”), you unlock a host of benefits:
• Security & Patch Management: SSM lets you automate patching and apply updates to your instances, keeping them secure. With SSM’s Patch Manager and Automation documents, you can ensure instances receive timely security patches and configuration updates. Instances not in SSM may miss critical updates, leaving vulnerabilities unaddressed.
• Compliance & Auditing: Many compliance frameworks (e.g., NIST CSF) and internal policies require centralized management of servers. By utilizing AWS Systems Manager, you ensure EC2 instances are continuously monitored for security compliance, patch management, software inventory, and regulatory adherence . This helps maintain a secure and compliant environment by centralizing and streamlining EC2 management .
• Operational Efficiency: SSM provides tools like Run Command, Session Manager, and Inventory. These enable you to remotely execute commands, manage configurations, and track software inventory across all your instances. If an instance isn’t SSM-managed, you lose these capabilities for that instance, making troubleshooting and administration harder.
• Consistency: SSM-managed instances can be part of Automation workflows and State Manager associations to enforce desired states. Unmanaged instances might drift from your standard configurations.
In short, an EC2 instance managed by SSM (i.e., a “managed instance”) is any instance that has been configured for Systems Manager . Ensuring all instances are managed means you have consistent control and visibility, which is key to good DevSecOps practices.
How EC2 Instances Become “Unmanaged”
Even in well-run environments, it’s possible for EC2 instances to fall out of SSM management and become “unmanaged.” Here are common scenarios:
• SSM Agent Not Running: The AWS SSM Agent is the software on the instance that communicates with Systems Manager. If this agent is not installed, has been accidentally uninstalled, or is stopped/disabled, the instance will not report to SSM. According to AWS Config’s managed rule documentation, an instance is flagged NON_COMPLIANT if the instance is running but the SSM Agent is stopped or terminated . In other words, a running EC2 with no active SSM agent is considered unmanaged.
• Missing IAM Role/Permissions: For an EC2 instance to be managed by SSM, it must have an IAM instance profile with the necessary permissions (typically the AmazonSSMManagedInstanceCore policy). If an instance was launched without this IAM role or the role’s policies were removed, the SSM agent cannot connect to the Systems Manager service. The instance will then drop out of SSM’s managed instances list.
• Network Misconfiguration: SSM Agent needs network access to communicate with AWS endpoints. If the instance has no internet access or no VPC endpoints for SSM, it may fail to connect. For example, an EC2 in a private subnet without the required VPC endpoints (or NAT) for SSM will be unable to register, effectively unmanaged.
• Outdated or Misconfigured Agent: In some cases, an outdated SSM agent might crash or behave unexpectedly, or the instance might be misconfigured (e.g., firewall blocking SSM traffic). This can cause previously managed instances to stop reporting.
• Instances Launched Outside Standard Processes: You might have automation to auto-register instances with SSM, but if someone launches an EC2 instance outside of those processes (for instance, in a new account or region without SSM setup), that instance might come up unmanaged.
It’s important to catch these situations. Unmanaged instances won’t receive your automated patches or configurations, and they won’t show up in SSM Inventory or Compliance views. This could lead to security gaps or operational blind spots. Our goal is to set up an automated alert whenever an instance becomes unmanaged so that you can remediate it (for example, reinstall the agent, fix the IAM role, or shut down the instance if it’s not approved).
How can we detect unmanaged instances automatically?
AWS offers a managed AWS Config rule exactly for this: “EC2 instances managed by Systems Manager.” This Config rule (EC2_INSTANCE_MANAGED_BY_SSM) evaluates all EC2 instances and checks if each is managed by SSM. The rule is marked NON_COMPLIANT if an EC2 instance is running and the SSM Agent is stopped or not communicating . We will use this rule as the trigger for our alerting system.
Solution Overview and Architecture
To automate alerts for unmanaged EC2 instances, we’ll use the following AWS services working in tandem:
• AWS Config Managed Rule: We’ll enable the ec2-instance-managed-by-systems-manager rule, which flags any running EC2 that isn’t SSM-managed as non-compliant.
• Amazon EventBridge (CloudWatch Events): We’ll create an EventBridge rule to listen for compliance change events from AWS Config. Specifically, it will catch events when the above Config rule evaluates an instance as NON_COMPLIANT. By filtering the event, we ensure we react only to instances becoming non-compliant (unmanaged).
• AWS Lambda Function: The EventBridge rule will trigger a Lambda function. This function will gather details about the offending EC2 instance (like its instance ID and Name tag) and send out an alert email.
• Amazon Simple Email Service (SES): Our Lambda will use Amazon SES to send the actual email notification to specified recipients. We’ll use SES because it’s reliable and designed for such use cases. (SNS could be an alternative for simple text notifications, but SES allows a direct email with customizable content.)
• AWS CDK (TypeScript): We’ll implement all the infrastructure above using the AWS CDK, making it easy to deploy repeatably. This includes the Config rule, EventBridge rule, Lambda function code, and necessary permissions and SES setup.
Below is a conceptual diagram of the architecture:
Event-driven architecture: AWS Config (via EventBridge) triggers a Lambda Function when an instance becomes non-compliant, and the Lambda sends an email using Amazon SES.
In this flow, AWS Config continuously evaluates instances against the SSM management rule. If an instance fails (becomes unmanaged), EventBridge immediately triggers the Lambda. The Lambda logs the event (CloudWatch Logs) and sends an email via SES. Using CDK ensures this entire setup is defined as code, version-controlled, and can be deployed in multiple environments – a big win for DevSecOps practices.
Now, let’s dive into the step-by-step implementation.
Prerequisites
Before we start building, make sure you have the following:
• AWS Account & CLI Access: You’ll need an AWS account with permissions to create the resources (Config rules, Lambda, SES, etc.). Configure AWS CLI or AWS CDK with appropriate credentials (e.g., Admin or a role with necessary rights).
• AWS CDK Toolkit: Install the AWS CDK if you haven’t already. For example, via npm: npm install -g aws-cdk. This post uses CDK v2 with TypeScript.
• AWS Config Enabled: Ensure AWS Config is enabled in the region you’re deploying to. You should have a Configuration Recorder and Delivery Channel set up (with an S3 bucket for config data). If not, you can enable AWS Config in the console by choosing a bucket/role. (CDK can create these too, but it’s outside our main scope.)
• SES Email Verification: Decide which email address (or domain) will be used as the sender for alerts, and which address will receive the alerts. In SES, verify the sender email (and recipient as well, if your SES is in sandbox mode). In SES, you need to verify both the sender and recipient email addresses to ensure a secure and controlled email-sending environment when in sandbox. We’ll assume an email (e.g., alerts@example.com) is verified to send, and you have access to an inbox to receive the notifications.
With that out of the way, let’s start building our solution with CDK!
Step 1: Initialize a New AWS CDK Project
First, set up a new CDK project for our infrastructure:
1. Create a project directory (e.g., aws-ssm-alert) and initialize a CDK TypeScript app:
mkdir aws-ssm-alert && cd aws-ssm-alert
cdk init app --language typescript
This will generate a baseline CDK project with TypeScript. You’ll see files like package.json, cdk.json, a /bin folder with the app’s entry point, and a /lib folder with a stack class.
2. Explore the project structure: After init, your project should look like:
aws-ssm-alert/
├── bin/
│ └── aws-ssm-alert.ts # CDK app entry point
├── lib/
│ └── aws-ssm-alert-stack.ts # Your main stack definition
├── package.json
├── cdk.json
├── tsconfig.json
└── etc...
The CDK app in bin/aws-ssm-alert.ts instantiates the stack defined in lib/aws-ssm-alert-stack.ts. We will be writing our infrastructure code in the stack class.
3. Install dependencies: The template already includes aws-cdk-lib and constructs in package.json. Run npm install if it wasn’t run automatically. Also, ensure the CDK libraries are up to date (you can use npm install aws-cdk-lib@latest if needed).
4. Bootstrap (if necessary): If you haven’t used CDK in this AWS account/region before, run cdk bootstrap to set up the necessary CDK resources (this creates a CDK toolkit stack with an S3 bucket for assets, etc.). This only needs to be done once per account/region.
With the CDK project ready, we can start defining our AWS resources in code.
Step 2: Define the AWS Config Rule (SSM Managed Instances)
The core of our alerting system is the AWS Config rule that checks for SSM-managed instances. AWS provides a managed rule for this, so we don’t have to write custom logic – we just need to enable it in our account via CDK.
Open the stack file (lib/aws-ssm-alert-stack.ts) and add the following code to create the AWS Config rule:
import * as config from 'aws-cdk-lib/aws-config';
// ... other imports ...
export class AwsSsmAlertStack extends Stack {
constructor(scope: Construct, id: string, props?: StackProps) {
super(scope, id, props);
// AWS Config rule to check if EC2 instances are managed by SSM
const ssmManagedRule = new config.ManagedRule(this, 'SSMManagedInstancesRule', {
identifier: config.ManagedRuleIdentifiers.EC2_INSTANCE_MANAGED_BY_SSM,
configRuleName: 'ec2-instance-managed-by-systems-manager',
description: 'Checks if EC2 instances are managed by AWS Systems Manager (SSM).'
});
// ... (we will add more resources below) ...
}
}
A few notes on this code:
• ManagedRuleIdentifiers.EC2_INSTANCE_MANAGED_BY_SSM
is a constant for the managed rule identifier. This corresponds to the AWS Config rule that “checks whether the Amazon EC2 instances in your account are managed by AWS Systems Manager” .
• We set configRuleName to ec2-instance-managed-by-systems-manager
. This is the display name of the rule (the one you’ll see in the AWS Config console). AWS’s documentation notes that the rule identifier and name differ for this rule ; using the documented name avoids confusion.
• The rule has no additional parameters – it will evaluate all EC2 instances in the account/region by default. The rule triggers on configuration changes (such as an instance starting or an agent status change).
When this rule finds a non-compliant instance (SSM agent off), it will report NON_COMPLIANT to AWS Config. However, just creating the rule doesn’t send any alerts by itself – that’s where the next components come in.
Important: Make sure AWS Config is active. If AWS Config is not yet enabled in this region, deploy a Configuration Recorder and Delivery Channel (or enable via console) before deploying this rule. Without an active recorder, the rule won’t run.
Step 3: Create the Lambda Function to Send Alerts
Next, we’ll create a Lambda function that will be triggered on non-compliance events and send an email alert via SES. We’ll implement the function in Python for simplicity.
Lambda Function Code (Python): Create a new directory (e.g., lambda) in the project, and inside it create a file ssm_alert_function.py with the following code:
import os
import boto3
def lambda_handler(event, context):
# Extract relevant details from the AWS Config event
detail = event.get('detail', {})
instance_id = detail.get('resourceId')
region = detail.get('awsRegion')
account = detail.get('awsAccountId')
rule_name = detail.get('configRuleName')
compliance = detail.get('newEvaluationResult', {}).get('complianceType')
# Default values if data is missing
instance_name = "Unknown"
# Get the EC2 instance's Name tag (if available) for a human-friendly identifier
if instance_id and region:
ec2 = boto3.client('ec2', region_name=region)
try:
resp = ec2.describe_instances(InstanceIds=[instance_id])
reservations = resp.get('Reservations', [])
if reservations:
tags = reservations[0]['Instances'][0].get('Tags', [])
for tag in tags:
if tag['Key'] == 'Name':
instance_name = tag['Value']
break
except Exception as e:
print(f"Error fetching instance name for {instance_id}: {e}")
# Compose the email subject and body
subject = f"Alert: EC2 instance {instance_id} is NOT managed by SSM"
body_text = (
f"EC2 instance {instance_id} (Name: {instance_name}) in account {account}, region {region} "
f"is {compliance} with AWS Systems Manager compliance (rule: {rule_name}).\n"
"This means the instance is not managed by AWS Systems Manager (SSM). "
"Please check the instance's SSM agent and IAM role to restore management."
)
# Send the email via Amazon SES
ses = boto3.client('ses', region_name=region)
response = ses.send_email(
Source=os.environ['SENDER_EMAIL'],
Destination={'ToAddresses': [os.environ['ALERT_EMAIL']]},
Message={
'Subject': {'Data': subject},
'Body': {'Text': {'Data': body_text}}
}
)
print(f"SES send_email response: {response['MessageId']}")
return {"status": "sent", "messageId": response['MessageId']}
Let’s break down what this function does:
• It expects the event to be an AWS Config compliance change event. It parses out details like instance_id, AWS region, AWS account, the rule_name, and the compliance status. These are nested under event['detail'].
• It then uses the EC2 API (describe_instances) to get the instance’s tags, searching for the Name tag. This is optional but makes the alert more informative (e.g., “Instance i-0123456789 (Name: WebServer1) is not managed by SSM”). If anything goes wrong or the tag isn’t found, we default to “Unknown”.
• Next, it composes an email message. We use environment variables SENDER_EMAIL and ALERT_EMAIL (we will set these in the CDK) to avoid hard-coding addresses. The email includes the instance ID, name, account, region, and a brief note that it’s not managed by SSM.
• Finally, it uses boto3 (AWS SDK for Python) to call ses.send_email() with the given subject and body. The result includes a MessageId which we log for reference.
This is a straightforward function – essentially, gather info and send an email. Using SES through boto3 in Lambda is a common pattern (just ensure your region supports SES and the emails are verified).
Now we need to integrate this Lambda function into our CDK stack:
In the CDK stack code (aws-ssm-alert-stack.ts), add the Lambda function resource:
import * as lambda from 'aws-cdk-lib/aws-lambda';
import * as path from 'path';
import * as iam from 'aws-cdk-lib/aws-iam';
// ... (make sure to import these at top)
// Within the AwsSsmAlertStack constructor, after defining ssmManagedRule:
// Lambda function to handle non-compliance events and send email alerts
const alertFunction = new lambda.Function(this, 'SSMAlertFunction', {
runtime: lambda.Runtime.PYTHON_3_9,
handler: 'ssm_alert_function.lambda_handler',
code: lambda.Code.fromAsset(path.join(__dirname, '../lambda')), // assumes code is in lambda/ directory
environment: {
'SENDER_EMAIL': 'alerts@example.com', // replace with your verified sender
'ALERT_EMAIL': 'admin@example.com' // replace with your recipient email
}
});
// Grant permissions to the Lambda to describe EC2 instances and send SES email
alertFunction.addToRolePolicy(new iam.PolicyStatement({
actions: ['ec2:DescribeInstances'],
resources: ['*'] // describing is read-only; cannot easily restrict by resource ID
}));
alertFunction.addToRolePolicy(new iam.PolicyStatement({
actions: ['ses:SendEmail', 'ses:SendRawEmail'],
resources: ['*'] // allow sending email via SES from any identity (we will restrict via verified identity in SES itself)
}));
Key points about this CDK code:
• We package the Lambda code using lambda.Code.fromAsset. This will zip the contents of the lambda directory (which contains our ssm_alert_function.py) and deploy it. Make sure the path is correct relative to your CDK project structure.
• We set the runtime to Python 3.9 (you can use 3.10 or 3.8 as supported) and the handler to ssm_alert_function.lambda_handler (file name and function name).
• In environment, plug in the verified SES sender and the desired recipient. Use your actual emails here: for instance, if you verified ops-team@mycompany.com in SES, set SENDER_EMAIL to that. For ALERT_EMAIL, you can use a distribution list or your email (and ensure it’s verified if in sandbox).
• We then add IAM permissions for the Lambda:
• ec2:DescribeInstances so the function can look up instance tags. (We scope to all resources; you could theoretically scope to the specific instance ARN if the event provided it, but since any instance ID could come through, we allow all. This action is read-only.)
• ses:SendEmail and ses:SendRawEmail so the function can send emails via SES. We allow all resources (*) which means it can use any verified identity in this account. For tighter security, you could specify the ARN of the SES identity (your verified email/domain), but that can vary by region. Simplicity is fine here, given that only our function has this role.
By default, the Lambda gets a basic execution role that allows CloudWatch Logs, so it can write logs (the print statements) to CloudWatch.
Now we have the Config rule and a Lambda function to handle alerts. Next, we connect them with EventBridge.
Step 4: Set Up an EventBridge Rule to Trigger the Lambda
AWS Config emits events on state changes of rules. We’ll create an EventBridge rule (formerly CloudWatch Events rule) that listens for our Config rule becoming NON_COMPLIANT and invokes the Lambda function.
Add the following to the stack code:
import * as events from 'aws-cdk-lib/aws-events';
import * as targets from 'aws-cdk-lib/aws-events-targets';
// ... (ensure these imports are present)
// Within the constructor, after defining alertFunction:
// EventBridge rule to catch Config rule non-compliance and trigger the Lambda
const configNonComplianceRule = new events.Rule(this, 'ConfigNonComplianceRule', {
description: 'Triggers on EC2 SSM Managed Instances rule NON_COMPLIANT evaluations',
eventPattern: {
source: ['aws.config'],
detailType: ['Config Rules Compliance Change'],
detail: {
// Only target our specific Config rule by name:
configRuleName: [ 'ec2-instance-managed-by-systems-manager' ],
// Only trigger when the new evaluation is NON_COMPLIANT
newEvaluationResult: {
complianceType: [ 'NON_COMPLIANT' ]
}
}
}
});
// Set the Lambda as the target for the EventBridge rule
configNonComplianceRule.addTarget(new targets.LambdaFunction(alertFunction));
Let’s explain the event pattern:
• source: We filter events from aws.config only.
• detailType: We specifically look at “Config Rules Compliance Change” events. AWS Config generates this event type when a resource’s compliance status changes for a Config rule .
• detail.configRuleName: We restrict the rule name to our rule (“ec2-instance-managed-by-systems-manager”). This ensures we don’t catch compliance changes from other, unrelated Config rules you might have. (If you want one Lambda to handle multiple rules, you could list multiple names here.)
• detail.newEvaluationResult.complianceType: We only care about transitions to NON_COMPLIANT. This way, when an instance becomes non-compliant (unmanaged), we trigger the Lambda. We ignore transitions to COMPLIANT or other states. By filtering on the complianceType, we handle only the “went non-compliant” direction .
With this pattern, whenever AWS Config flags an instance as not managed by SSM, an event will match and our alertFunction will be invoked. The event data (as we saw in the Lambda code) includes the instance ID, etc.
We’ve now defined all the main components in our CDK stack: the Config rule, the EventBridge rule, and the Lambda with SES permissions. The last piece is making sure SES is configured to allow our emails to be sent.
Step 5: Configure Amazon SES for Email Sending
Amazon SES (Simple Email Service) will actually deliver our alert emails. We need to ensure SES is set up properly:
• Verify the Sender Email: In the AWS SES console, verify the email address you intend to send from (the one we set as SENDER_EMAIL in the Lambda environment). SES will send a verification link to that email; once you confirm it, the email is registered as a verified identity in SES. If you have a domain verified in SES, you could use an address on that domain as well.
• Verify the Recipient Email (if needed): If your SES is in the sandbox (the default for new accounts), you must also verify any recipient email address. Verify the address that you set as ALERT_EMAIL. (In production SES – after you request a sending limit increase – you can send to any address. But initially, sandbox mode restrictions apply.)
• Region Consideration: Note which AWS region you verified the identities in. SES identities are regional. Make sure the Lambda uses the same region for the SES client. In our code, we used region_name=event['detail']['awsRegion'] to initialize the boto3 SES client to the region where the Config event came from (which should be your deployment region). If your SES is in a different region, you might want to explicitly set the region or adjust accordingly. It’s simplest to deploy everything in one region.
If you haven’t used SES before, the AWS documentation provides guidance on verifying identities . The key point is that SES must know you own the sender (and for sandbox, the receiver). After verification, our Lambda’s ses.send_email call will be able to send the message.
📝 Tip: You can test SES sending manually by using the “Send Test Email” feature in the SES console with your verified identities, just to ensure email delivery works, before relying on the Lambda.
Now our infrastructure and configurations are all set. Let’s deploy and see it in action.
Step 6: Deploy the CDK Stack and Test the Setup
With our CDK code ready, we can deploy it to AWS:
1. Synthesize the CloudFormation template: Run cdk synth in your project directory. This will compile your TypeScript and output the CloudFormation template. Check that there are no errors and the resources (Config rule, Lambda, EventBridge rule, IAM policies) are present in the synthesized output.
2. Deploy to AWS: Run cdk deploy. You’ll be asked to confirm IAM changes (since we’re creating roles and a Config rule that involves AWS Config service). Type “y” to approve. CDK will then create the stack in your AWS account.
• The deployment will output progress. It should create the Config rule, Lambda function (and an S3 asset upload for the code), the EventBridge rule, etc.
• Once complete, note any output or confirmations. There might not be custom outputs, but you can verify in the AWS console that the resources are now live:
• In AWS Config console, under Rules, you should see ec2-instance-managed-by-systems-manager with a green check if all instances are compliant (or a red exclamation if not).
• In the Lambda console, the function SSMAlertFunction should exist with the environment variables set.
• In EventBridge (or CloudWatch Events) console, a rule ConfigNonComplianceRule should be present targeting the Lambda.
• In IAM, the Lambda’s role should have the SES and EC2 read permissions we defined.
3. Test the functionality: Now the fun part – validate that the alert works. There are a couple of ways to test:
• Immediate Compliance Check: As soon as the Config rule is active, it will evaluate your instances. If you already have an unmanaged EC2 instance, the rule should flag it. AWS Config might periodically re-evaluate or evaluate on state change. To force a quick evaluation, you can go to the AWS Config console, select the rule, and trigger a re-evaluation (or stop/start an instance to generate a config change).
• Simulate a Non-Compliant Instance: If all your instances are compliant, you can simulate a violation. For example, take a test EC2 instance and try to break its SSM management:
• If it’s Windows or Linux with SSM agent running, you could stop the SSM agent service on that instance (for a quick test). On Amazon Linux, sudo systemctl stop amazon-ssm-agent will do it. Within a few minutes, AWS Config should detect the agent is not responding.
• Alternatively, remove the IAM instance profile from the instance (or create a new instance without an IAM role for SSM). The agent will lose access and should drop off.
• Wait for AWS Config Evaluation: AWS Config will mark the instance as NON_COMPLIANT on the next evaluation cycle or config change trigger. When that happens, EventBridge should catch it and invoke the Lambda. This is usually quite fast (near real-time on config change).
• Check for Email: Monitor the recipient inbox (and possibly spam folder, just in case) for the alert email. The email subject should start with “Alert: EC2 instance i-XXXX is NOT managed by SSM”. The body will list the instance ID and name, account, region, and advise to check the agent/role.
• Verify CloudWatch Logs: If you don’t see an email, go to CloudWatch Logs for the Lambda (/aws/lambda/SSMAlertFunction) to troubleshoot. You should see logs of the event and any errors. Common issues could be SES not sending (check that the email is verified and in correct region) or permissions.
If everything is set up correctly, you should receive the notification email shortly after an instance becomes unmanaged. Success! 🎉 You’ve got an automated watcher on your EC2 instances’ SSM status.
Conclusion
By following this guide, you have implemented an automated alert system for unmanaged EC2 instances using AWS Systems Manager and AWS CDK. This solution ensures that if any EC2 in your environment loses SSM management (whether due to agent issues or misconfiguration), you’ll promptly get an email alert with the details. The combination of AWS Config and EventBridge provides a powerful event-driven way to monitor compliance in near real-time, and using Lambda+SES gives a flexible notification mechanism.
This setup isn’t just about notifications – it’s about embracing DevSecOps principles. We’ve automated a compliance check and integrated it into your operations workflow, reducing the need for manual monitoring. Such automation helps maintain infrastructure hygiene and frees engineers to focus on proactive improvements rather than reactively putting out fires. As noted earlier, automation of compliance checks saves time and lowers risk , which is exactly what we achieved here.
You can extend this approach further by adding automatic remediation (for example, triggering a Systems Manager Automation document to reinstall the agent or notify via Slack or create a ServiceNow ticket, etc.). AWS Config Rules even support automatic remediation actions via SSM documents , which could be a next step.
In production environments, it’s highly recommended to adopt this kind of guardrail. It provides quick visibility into potential misconfigurations (like someone launching an instance without proper IAM role or an agent crash going unnoticed). With AWS CDK, you also have this infrastructure as code, making it easy to version control and deploy to multiple accounts or regions as needed.
In summary, by setting up AWS alerts for unmanaged EC2 instances, you bolster your cloud environment’s security and compliance posture. We encourage you to integrate this solution (and others like it for different compliance rules) into your environments. It’s a small investment of time that pays off with continuous, hands-off monitoring and peace of mind knowing no EC2 instance will silently drift out of management without you knowing. Happy automating and stay secure!
Subscribe to my newsletter
Read articles from Pawan Sawalani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
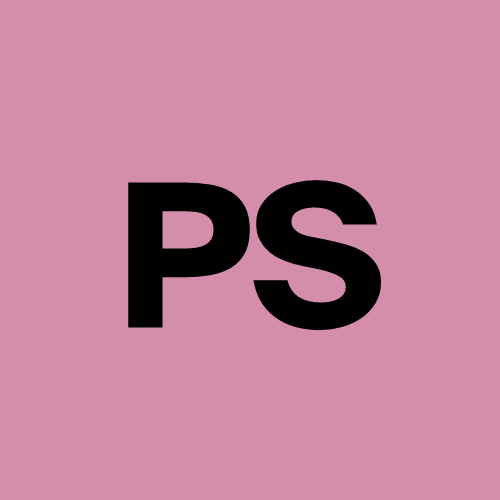