🧠 Mastering Node.js Performance with Clustering in Express.js + TypeScript
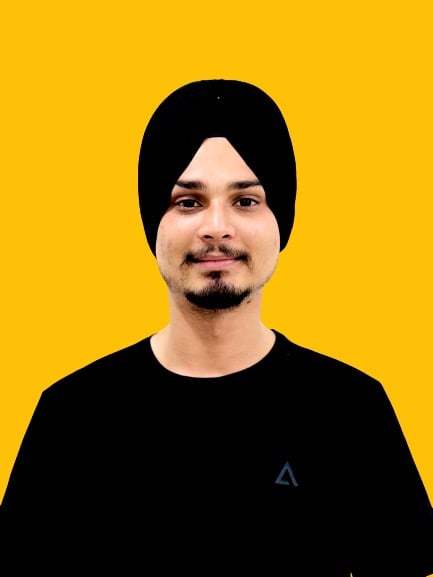
Table of contents
- After spending so much time in building high-performance Node.js applications, one thing I’ve learned the hard way is this:
- ⚙️ Optimizing Your App Before Scaling
- 🔍 What is Node.js Clustering?
- 💥 Why You Need Clustering (Real Example)
- 🛠️ Implementing Clustering in Express.js with TypeScript
- 📦 Production Advice (From the Trenches)
- ⚠️ Where Clustering Doesn’t Work
- 🧠 Final Thoughts

After spending so much time in building high-performance Node.js applications, one thing I’ve learned the hard way is this:
Your backend might run, but that doesn’t mean it’s ready to scale.
It’s one of those lessons that hits you when traffic surges, CPU usage spikes, and your single-threaded Node.js app quietly chokes under the load.
⚙️ Optimizing Your App Before Scaling
When your app starts running slow or struggling under heavier traffic, the first instinct is to look for bottlenecks. Here are a few common things we often optimize before scaling:
Code Optimization
We refactor code to remove redundant processes and ensure efficiency.
We implement caching strategies where necessary.
Database Query Optimization
- We look for slow queries and optimize them by indexing, limiting result sets, or utilizing more efficient query patterns.
Middleware Optimization
- We reduce unnecessary middlewares and ensure the ones we use are lightweight and optimized.
Load Balancing
- We introduce load balancing to distribute requests across multiple instances or servers.
Memory Management
- We profile and optimize memory usage to ensure our app doesn’t run into memory leaks under load.
These are just a few things we tackle first. But as you scale up, optimizing code and database queries isn’t enough. This is where Node.js clustering comes into play.
🔍 What is Node.js Clustering?
At its core, Node.js is single-threaded — it processes all incoming requests using a single CPU core. This is fine for lightweight or low-traffic apps, but in real-world scenarios, it becomes a bottleneck.
Now, most modern machines have 4, 8, 16, or more CPU cores, but Node only uses one.
Clustering is a native Node.js feature that allows your app to:
Spawn multiple worker processes
Utilize all available CPU cores
Share a common port to handle incoming traffic
Recover gracefully if a worker crashes
In short: you scale your app horizontally on a single machine.
💥 Why You Need Clustering (Real Example)
Failure points can vary, but I believe the ones listed below are the most common and are ones that everyone has likely encountered at least once in their career.
we deployed a simple TypeScript+Express API for a fast-growing startup. At launch, things ran fine — response times were great, logs were clean.
In several hours, the app got hit with 800+ concurrent users.
Our single-threaded Node.js process maxed out at 100% CPU.
Requests started queuing up, and then — total meltdown.
Users got 502s. Logs froze. We couldn’t even SSH into the box.
All because we never enabled clustering.
We had an 8-core machine, and used only 1/8th of its potential.
That lesson became a rule on my teams:
If it’s production and not serverless, it’s clustered.
🛠️ Implementing Clustering in Express.js with TypeScript
Let me show you the exact boilerplate I use to cluster any Express + TypeScript app.
📁 Folder Structure
src/
├── index.ts // clustering logic
└── server.ts // app definition and other stuff
🔹 server.ts
– Your Standard Express App
import express from 'express';
export const createServer = () => {
const app = express();
app.get('/', (_req, res) => {
res.send(`Handled by worker ${process.pid}`);
});
return app;
};
🔹 index.ts
– The Clustering Logic
import cluster from 'cluster';
import os from 'os';
import { createServer } from './server';
const numCPUs = os.cpus().length;
if (cluster.isPrimary) {
console.log(`Primary process ${process.pid} is running`);
for (let i = 0; i < numCPUs; i++) {
cluster.fork();
}
cluster.on('exit', (worker) => {
console.log(`Worker ${worker.process.pid} exited. Spawning a new one...`);
cluster.fork();
});
} else {
const app = createServer();
const PORT = process.env.PORT || 3000;
app.listen(PORT, () =>
console.log(`Worker ${process.pid} is listening on port ${PORT}`)
);
}
Now when you run this index.ts/index.js file. You’ll now see multiple workers handling requests in parallel — leveraging every core your machine offers.
📦 Production Advice (From the Trenches)
If you’re serious about building scalable Node.js services, clustering should be part of your baseline architecture. Here are my personal opinions:
✅ Always cluster Express apps in production
✅ Use PM2 or Docker for process management
✅ Monitor CPU/Memory per worker
✅ Restart failed workers automatically
✅ Log the process.pid to identify bottlenecks
✅ Keep app logic stateless where possible
✅ Use Redis or shared caches for session management
⚠️ Where Clustering Doesn’t Work
While clustering is a killer feature, it’s not universal.
It doesn’t work on serverless platforms like:
Vercel
Netlify Functions
AWS Lambda
Cloudflare Workers
These environments run stateless functions, which are spun up and torn down per request. You don’t control the process — so cluster.fork()
has no effect.
If you're using serverless, clustering logic will simply be ignored. For these environments, trust their native auto-scaling — and focus on optimizing cold starts and keeping things stateless.
🧠 Final Thoughts
Clustering is not a “nice-to-have” — it’s a core scalability tool in the real world.
If you’re building APIs with Express and TypeScript/Javascript, and deploying to a host where you manage the process — enable clustering. Your future self (and your uptime metrics) will thank you.
Feel free to use this pattern across your projects. It’s helped me ship everything from MVPs to mission-critical financial APIs — and saved me from more 502s than I care to admit.
If this helped you — share it, bookmark it, or even better: implement it.
Let’s build smarter, faster, and more resilient Node.js apps together.
Subscribe to my newsletter
Read articles from Amandeep Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
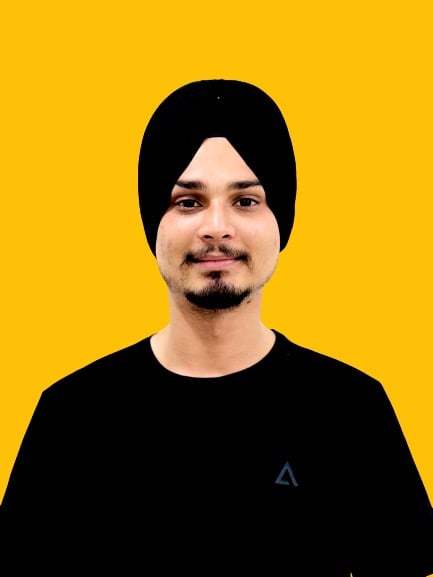
Amandeep Singh
Amandeep Singh
Proficient in a diverse range of technologies, I bring a dynamic skill set to every project I undertake. Whether it's optimising performance, implementing new features, or solving complex technical challenges, I thrive in dynamic environments where collaboration and creativity are paramount.