Hosting a Static Website on AWS S3 Using AWS CLI – No ClickOps, Just DevOps 💻
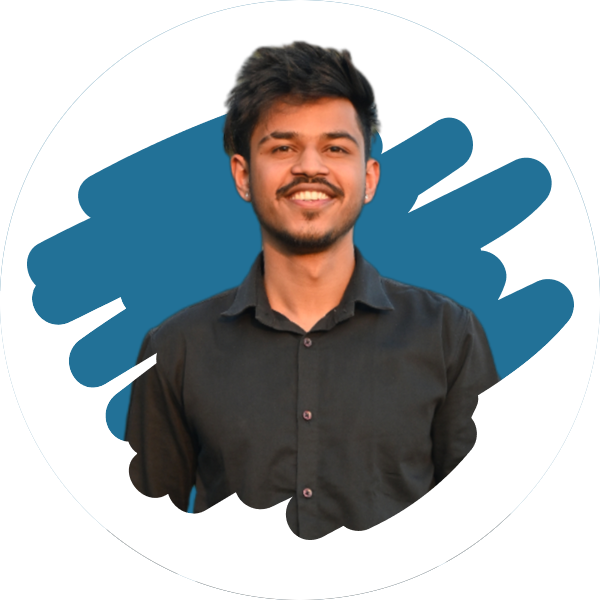

💡 Introduction
Hola Amigos! 👋
Welcome to the world of DevOps and automation! Today, we’re diving into a hands-on project that’s both simple and powerful—we’ll be creating and hosting a static website on Amazon S3.
Now you might be thinking, “Aren’t there already a million blogs on this?” And you're absolutely right! But here’s what sets this one apart: we’re not using ClickOps.
That’s right—no AWS Console clicking, no point-and-shoot setup. While the Console might be convenient for quick testing, it’s not how real-world DevOps workflows are built. As DevOps engineers, we’re expected to automate everything—using AWS CLI, SDKs, CDKs, or Infrastructure as Code (IaC) tools like Terraform.
In this blog, we’ll focus purely on automation using the AWS Command Line Interface (CLI) to get our static site live. It’s clean, repeatable, and the kind of thing you’d actually do in a professional environment.
Sounds exciting? Let’s get right into it—no fluff, just DevOps stuff. 👨💻🔥
🛠️ Prerequisites
Before we roll up our sleeves and get started, let’s make sure our environment is ready. Here’s what you’ll need to follow along smoothly:
✅ 1. AWS Account & CLI Configuration
Since we’re working with Amazon S3, you’ll need an AWS account. Make sure you have an IAM user with the necessary permissions and security credentials. Once that’s in place:
Install the AWS CLI on your system (v2 is recommended).
Configure it using
aws configure
and provide your Access Key, Secret Key, region, and output format
✅ 2. Basic Bash Knowledge
We’ll be writing a Bash script to automate the creation and configuration of the S3 bucket. If you're familiar with basic scripting, loops, and conditionals—you’re good to go. Nothing too fancy, just enough to glue the commands together.
✅ 3. AWS CLI Auto-Prompt (Highly Recommended)
Typing long CLI commands can get tedious—and prone to errors. To make things easier, you can enable auto-prompt mode by exporting the following environment variable:
export AWS_CLI_AUTO_PROMPT="on-partial"
This feature gives you an interactive suggestion prompt whenever you partially type a command—super helpful when you can’t recall all the flags or structure.
🧠 Writing the Bash Script
Let’s get to the fun part—automation.
As someone preparing for the AWS Solutions Architect Associate Certification, I’ve been documenting my learning through real-world labs. I’ve created a GitHub repository named AWS_EXAMPLES
, where I share scripts and solutions for various AWS use-cases I experiment with. For this tutorial, the Bash script used to host a static site on S3 is available here:
🔗 View the deploy script on GitHub
Let’s break down the contents of this script and what it does:
#!/bin/bash
# Exit immediately if a command exits with a non-zero status
set -e
# === CONFIGURATION ===
BUCKET_NAME="pravesh-static-site-$(date +%s)" # Ensures a unique bucket name
REGION="us-east-1" # Change if needed
INDEX_FILE="index.html"
# === STEP 1: Create the S3 bucket ===
echo "Creating bucket: $BUCKET_NAME"
aws s3api create-bucket \
--bucket "$BUCKET_NAME" \
--region "$REGION"
# === STEP 2: Disable block public access ===
echo "Disabling block public access..."
aws s3api put-public-access-block \
--bucket "$BUCKET_NAME" \
--public-access-block-configuration BlockPublicAcls=false,IgnorePublicAcls=false,BlockPublicPolicy=false,RestrictPublicBuckets=false
# === STEP 3: Set up bucket policy for public read ===
echo "Applying public read bucket policy..."
POLICY=$(cat <<EOF
{
"Version": "2012-10-17",
"Statement": [
{
"Sid": "PublicReadGetObject",
"Effect": "Allow",
"Principal": "*",
"Action": "s3:GetObject",
"Resource": "arn:aws:s3:::$BUCKET_NAME/*"
}
]
}
EOF
)
aws s3api put-bucket-policy --bucket "$BUCKET_NAME" --policy "$POLICY"
# === STEP 4: Enable static website hosting ===
echo "Enabling static website hosting..."
aws s3 website s3://$BUCKET_NAME/ --index-document index.html
# === STEP 5: Upload index.html file ===
echo "Uploading $INDEX_FILE..."
aws s3 cp "$INDEX_FILE" "s3://$BUCKET_NAME/"
# === STEP 6: Print the website URL ===
echo "Static site is now hosted at:"
echo "http://$BUCKET_NAME.s3-website-$REGION.amazonaws.com"
📌 Note
If you’re using a region other than us-east-1
, you’ll need to include a --create-bucket-configuration
flag with a location constraint like so:
--create-bucket-configuration LocationConstraint="$REGION"
🛠️ Running the Script
Save the script in a file named
deploy
in your working directory.Give it execute permissions using:
chmod u+x deploy
- Make sure you have an
index.html
file in the same directory as the script. You can use my version:
...or simply create your own.
- Finally, run the script:
./deploy
You’ll see the script do its magic—create a bucket, configure permissions, upload your site, and print out the final URL. Just copy that link, paste it into your browser, and voilà—your static website is live! 🎉
🏁 Conclusion
And that’s a wrap!
In this blog, we explored how to host a static website on Amazon S3 using nothing but the AWS CLI. Specifically, we learned how to:
Create a uniquely named S3 bucket using
s3api
Disable block public access for the bucket
Write and apply an inline bucket policy to allow public reads
Enable static website hosting on the bucket
Upload our
index.html
fileRetrieve the final URL to access the website
All of this—done seamlessly from the terminal. No console clicking, just pure DevOps automation vibes! 😎
This was a fun little project, and I hope you enjoyed following along as much as I enjoyed building it.
If you found this blog helpful, feel free to connect with me on LinkedIn, Twitter, and Dev.to—I regularly share hands-on DevOps content, tutorials, and real-world experiments from my learning journey.
Until next time—happy learning and keep automating! 🚀
Subscribe to my newsletter
Read articles from Pravesh Sudha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
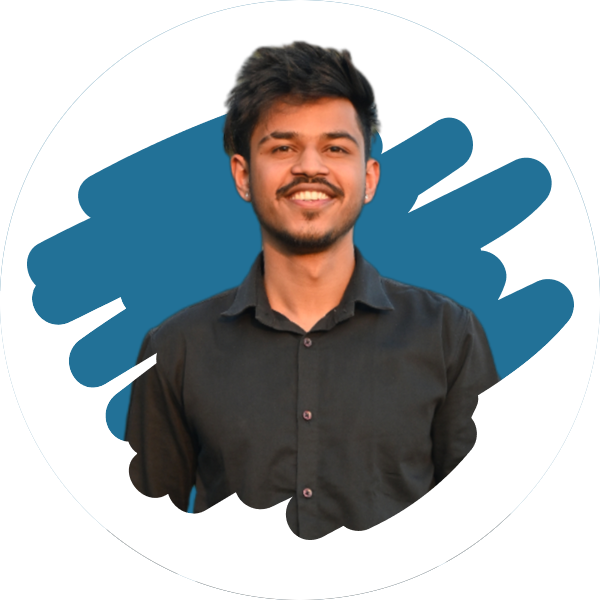
Pravesh Sudha
Pravesh Sudha
Bridging critical thinking and innovation, from philosophy to DevOps.