🚀 Mastering HTTP Requests & Routing in Node.js: The Backend Developer’s Guide
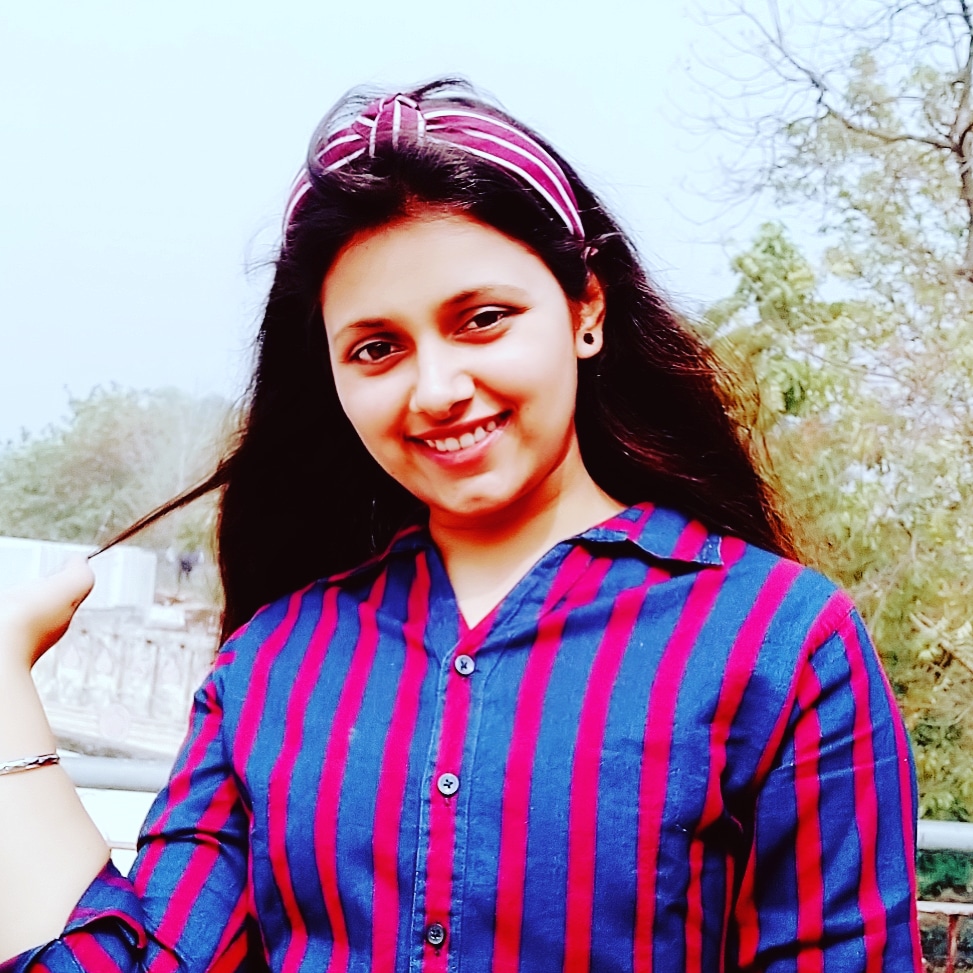

The Request-Response Cycle and HTTP Methods in Node.js
Understanding how HTTP requests and responses work is fundamental to backend development. In this blog, we'll explore the request-response cycle, HTTP methods, and basic routing in Express.js, along with tools to test your APIs.
The Request-Response Cycle
When a client (e.g., a web browser or mobile app) sends a request to a server, the backend (Express.js in this case) processes the request and returns a response. This cycle consists of:
- Client sends a request: Includes an HTTP method (GET, POST, etc.), headers, and optional data (body).
- Server processes the request: The backend logic (routes, middleware, databases) handles the request.
- Server sends a response: Contains a status code (e.g.,
200 OK
,404 Not Found
) and optional data (e.g., JSON, HTML).
HTTP Methods
HTTP methods define the type of operation the client wants to perform on the server:
Method | Description | Example Use Case |
GET | Retrieve data from the server. | Fetching a list of movies. |
POST | Create new data on the server. | Adding a new movie. |
PUT | Update entire existing data. | Replacing a movie's details. |
PATCH | Update part of existing data. | Changing only a movie's title. |
DELETE | Remove data from the server. | Deleting a movie. |
Basic Routing in Express.js
Routing refers to how an application responds to client requests for specific endpoints (URL paths) and HTTP methods.
Creating a Route
Here’s a simple GET
route in Express:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Welcome to API!');
});
Key Components of a Route
- Endpoint: The URL path (e.g.,
/movies
). - HTTP Method: Defines the action (GET, POST, etc.).
- Request (
req
): Contains data from the client (e.g., query params, body, headers). - Response (
res
): Used to send data back to the client.
Example: POST Route
app.post('/movies', (req, res) => {
res.send('Movie created successfully!');
});
API Testing Tools: Postman and Insomnia
Testing APIs manually via a frontend can be tedious. Tools like Postman and Insomnia simplify this process by allowing you to:
- Send requests to any endpoint (GET, POST, etc.).
- Test API responses without building a frontend.
- Simulate edge cases (e.g., invalid data, authentication errors).
Why Use These Tools?
- Debugging: Check if your backend handles requests correctly.
- Documentation: Share API workflows with your team.
- Automation: Write tests for your endpoints.
Important Notes
- Always validate data in POST, PUT, and PATCH requests to avoid malformed inputs.
- Use proper HTTP status codes (e.g., 200 for success, 404 for not found, 500 for server errors).
- Keep routes organized using Express Router for larger applications.
- Secure your APIs with middleware like helmet and cors.
- Test thoroughly before deploying to production.
In the next blog, we’ll dive into middleware, req
and res
objects, and error handling in Express.js. Stay tuned!
Subscribe to my newsletter
Read articles from Anjali Saini directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
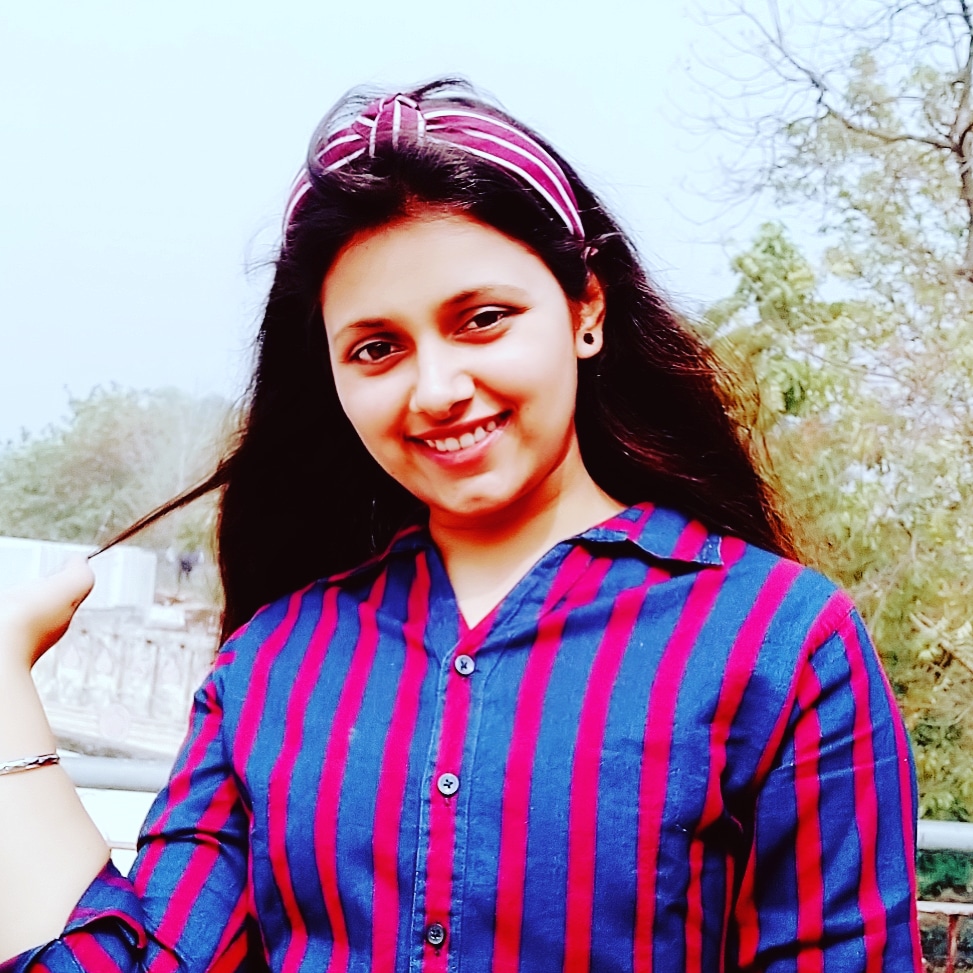
Anjali Saini
Anjali Saini
I am an Enthusiastic and self-motivated web-Developer . Currently i am learning to build end-to-end web-apps.