Deploying a Simple React and Node.js Application on Heroku with GitHub Integration
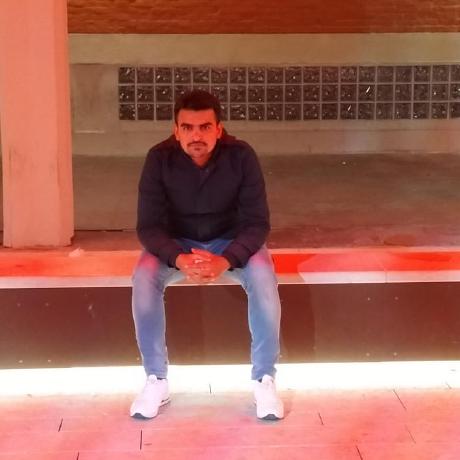

In modern software development, Continuous Deployment (CD) has become a critical practice, enabling developers to automatically deploy their applications to production whenever changes are made. One of the most popular cloud platforms for deploying web applications is Heroku, which offers seamless integration with GitHub to automate deployment processes. This guide will walk you through the steps to deploy a simple React and Node.js application to Heroku, using GitHub for version control and automatic deployment.
Prerequisites
Before we begin, ensure you have the following tools installed:
Node.js and npm (Node Package Manager) – To build and run the application.
Heroku CLI – Command line tool to interact with Heroku.
Git – For version control and connecting your project with GitHub.
A GitHub account – To store and manage the source code of your project.
A Heroku account – To host and deploy the application.
Step 1: Setting Up Your React and Node.js Application
We will start by creating a simple React frontend and a Node.js backend. In this example, the React app will communicate with the Node.js backend through API endpoints.
Initialize the Node.js Backend
Create a new directory for your project:
mkdir react-node-heroku cd react-node-heroku
Initialize the Node.js backend:
mkdir backend cd backend npm init -y npm install express
Create an
index.js
file in thebackend
folder:const express = require('express'); const app = express(); const port = process.env.PORT || 5000; app.get('/api', (req, res) => { res.json({ message: 'Hello from the Node.js backend!' }); }); app.listen(port, () => { console.log(`Server running on port ${port}`); });
Initialize the React Frontend
Navigate back to the root directory of your project and create the React app:
npx create-react-app frontend cd frontend
Inside the
frontend/src/App.js
file, modify it to fetch data from your Node.js backend:import React, { useEffect, useState } from 'react'; function App() { const [message, setMessage] = useState(''); useEffect(() => { fetch('/api') .then((response) => response.json()) .then((data) => setMessage(data.message)); }, []); return ( <div className="App"> <h1>{message}</h1> </div> ); } export default App;
Proxying React to the Node.js Backend
In frontend/package.json
, add a proxy to redirect API calls to the backend:
"proxy": "http://localhost:5000",
Run the Application Locally
To test locally, first run the backend:
cd backend
node index.js
Then, in a separate terminal, start the React app:
cd frontend
npm start
You should now be able to access the React frontend at http://localhost:3000
, and it should successfully fetch data from the Node.js backend.
Step 2: Prepare for Deployment on Heroku
Initialize Git in Your Project
In the root of your project, initialize a Git repository:
git init
Add and commit your project files:
git add . git commit -m "Initial commit"
Create a Procfile
for Heroku
A Procfile
is required by Heroku to know how to run your application. In the root directory, create a file named Procfile
(without any extension) and add the following line:
web: node backend/index.js
This tells Heroku to start the Node.js server when deploying the application.
Prepare the React App for Production
Inside the
frontend
folder, build the React app for production:npm run build
After building the React app, copy the contents of the
build
folder into thebackend
folder, so it can be served by the Node.js backend. You can automate this step using a post-install script by adding the following line topackage.json
inside thefrontend
folder:"scripts": { "postinstall": "cp -r build/* ../backend/public/" }
This command will copy the React build output to the
public
folder of the backend each time you install dependencies.
Add Start Script for Heroku
In the backend/package.json
, add a start
script to serve the React build:
"scripts": {
"start": "node index.js"
}
Step 3: Push the Application to GitHub
Create a new repository on GitHub.
Push your local project to GitHub:
git remote add origin https://github.com/yourusername/your-repository.git git branch -M main git push -u origin main
Step 4: Deploy the Application on Heroku
Login to Heroku using the Heroku CLI:
heroku login
Create a new Heroku app:
heroku create
Link your GitHub repository to Heroku:
Go to the Heroku Dashboard, find your application, and navigate to the Deploy tab.
Choose GitHub as the deployment method and connect to your GitHub account.
Select the repository you want to deploy.
Enable Automatic Deployments:
- In the Deploy tab, scroll down to Automatic Deploys and enable it for the
main
branch. This ensures that every time you push changes to GitHub, Heroku will automatically deploy your app.
- In the Deploy tab, scroll down to Automatic Deploys and enable it for the
Deploy the Application:
- If you want to trigger the first deployment immediately, click on the Deploy Branch button.
Step 5: Verify the Deployment
Once the deployment is complete, Heroku will provide a URL for your live application. You can visit this URL in your browser to check if the app is running successfully.
Step 6: Pushing Changes and Automatic Deployment
From now on, every time you make changes to your React or Node.js code, follow these steps:
Commit your changes to GitHub:
git add . git commit -m "Your commit message" git push origin main
Heroku will automatically deploy the new changes as soon as they are pushed to GitHub.
Conclusion
By integrating GitHub with Heroku, you can automate the deployment process for your React and Node.js application. This approach improves the development workflow and ensures that your app is always up to date with the latest changes. With every push to GitHub, Heroku will automatically handle deployment, ensuring your app is always running the most recent version without manual intervention.
Subscribe to my newsletter
Read articles from Ahmed Raza directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
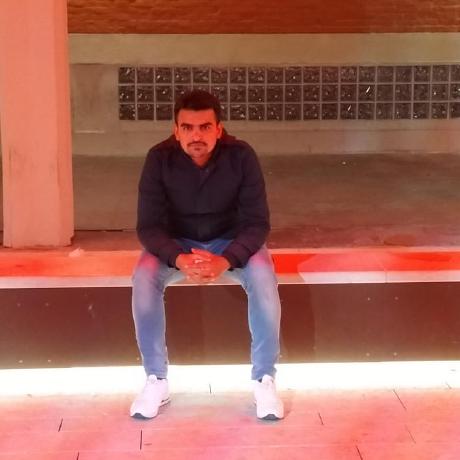
Ahmed Raza
Ahmed Raza
Ahmed Raza is a versatile full-stack developer with extensive experience in building APIs through both REST and GraphQL. Skilled in Golang, he uses gqlgen to create optimized GraphQL APIs, alongside Redis for effective caching and data management. Ahmed is proficient in a wide range of technologies, including YAML, SQL, and MongoDB for data handling, as well as JavaScript, HTML, and CSS for front-end development. His technical toolkit also includes Node.js, React, Java, C, and C++, enabling him to develop comprehensive, scalable applications. Ahmed's well-rounded expertise allows him to craft high-performance solutions that address diverse and complex application needs.