๐ง Understanding Spring Bean Scopes โ What I Learned This Week
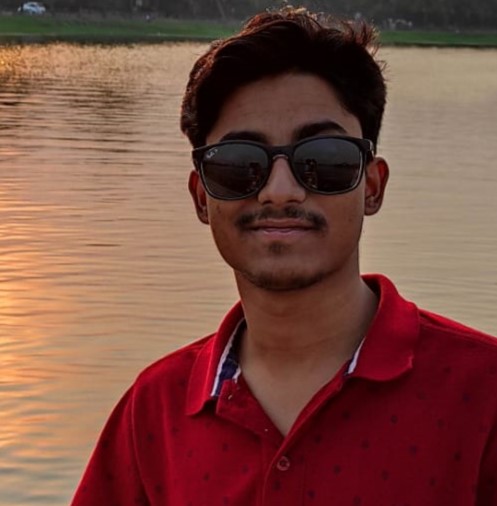

Last week, I delved deeper into Spring, focusing on a concept that frequently appeared in tutorials โ Bean Scopes. Initially, I thought, "Isn't every bean just created once and used everywhere?" But I discovered that Spring provides significant control over how many times a bean is created, how long it lives, and where it's used โ that's the essence of bean scopes. Here's my learning breakdown from a fellow learner trying to get comfortable with the Spring ecosystem one step at a time ๐.
๐ Resources I Used
๐ฑ So What Are Bean Scopes?
In Spring, a bean scope defines the lifecycle and visibility of a bean. Basically, it tells Spring how many instances of a bean should be created and where those instances should be stored.
When you annotate a class with @Component
or declare it as a bean, itโs by default singleton โ which means one shared instance is created and reused.
But thatโs not always ideal. Different use cases need different scopes.
๐ Types of Bean Scopes I Learned About
1. ๐ง Singleton (Default)
What it means: Only one instance of the bean exists in the Spring container.
When to use: For stateless services or components that don't hold user-specific data.
Config:
@Scope("singleton") @Component public class MyBean { ... }
2. ๐งช Prototype
What it means: A new bean instance is created every time itโs requested.
When to use: When you need separate state for each use (e.g., a form object).
Config:
@Scope("prototype") @Component public class MyBean { ... }
โ The container only creates it โ no further lifecycle management (like destroy).
3. ๐ Request (Web Only)
What it means: One bean per HTTP request.
When to use: Useful in web apps when you want one bean per user request.
Config:
@Scope("request") @Component public class MyBean { ... }
4. ๐ฌ Session (Web Only)
What it means: One bean per user session.
When to use: When you want to maintain user state (like a shopping cart).
Config:
@Scope("session") @Component public class MyBean { ... }
5. ๐ GlobalSession (Web + Portlet Context)
What it means: Like
session
, but for global session scope in a portlet-based environment.Not used much in basic Spring apps โ just good to know it exists.
๐ XML vs Annotation-Based Config
I mostly worked with annotations, but hereโs how they look in both styles:
โ Annotation-based
@Component
@Scope("prototype") // or "singleton", "request", "session"
public class MyBean { ... }
๐ XML-based
<bean id="myBean" class="com.example.MyBean" scope="prototype" />
Honestly, I prefer the annotation style. Feels cleaner and easier to manage โ but both get the job done.
๐ก Real-World Use Cases I Noted
Scope | When I'd Use It |
Singleton | Shared services, loggers, utility classes |
Prototype | Stateful objects (like form data) |
Request | Web controller request-specific data |
Session | User preferences, carts, or temporary settings |
๐ How Scope Affects Lifecycle?
This was an interesting part. I thought all beans go through the same lifecycle, but the scope actually changes how Spring manages the bean lifecycle:
Singleton beans are fully managed (init + destroy).
Prototype beans are only created โ Spring doesnโt call their
@PreDestroy
.For request/session/globalSession, Spring manages them based on HTTP lifecycle, not your
main()
method.
So if youโre using @PreDestroy
or custom destroy-method
, it wonโt work for prototype or request/session beans unless you're doing manual handling.
๐ What's Next?
Still continuing my Spring journey. Up next, Iโm planning to:
Dive into
@ComponentScan
and how Spring auto-wires thingsStart working with Spring Boot and REST APIs
Understand better how all this config works behind the scenes
This week was mostly about understanding the behavior of beans โ next week will be about putting them to work in real apps.
๐พ My Practice Code
Everything I tried out for this topic is here:
๐ GitHub - Spring Bean Scopes & Lifecycle Code
๐ฅ In a nutshell (TL;DR)
Spring gives multiple bean scopes like
singleton
,prototype
,request
,session
, andglobalsession
.Scope decides how many instances of a bean exist and for how long.
singleton
is default and ideal for stateless services.Use
prototype
when you want a new object every time.Request/session scopes are perfect for web apps.
Lifecycle management differs depending on the scope.
You can configure scope using annotations (
@Scope
) or XML.Understanding scopes = writing better, memory-friendly, bug-free Spring apps.
Hope this helped someone whoโs just getting started like me! Spring has a lot of layers, but bit by bit, itโs making more sense.
If youโre learning Spring too โ letโs connect and share learnings! ๐
Subscribe to my newsletter
Read articles from Arkadipta Kundu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
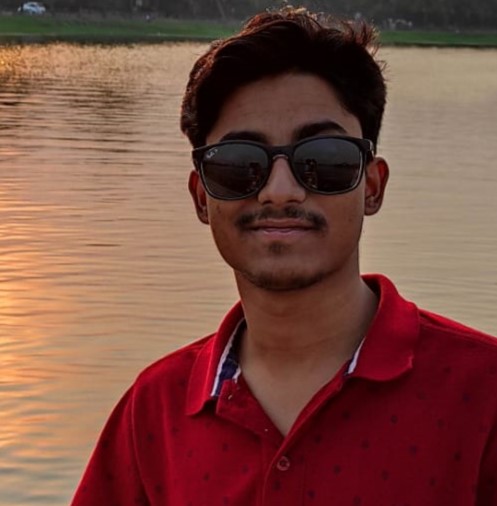
Arkadipta Kundu
Arkadipta Kundu
Iโm a Computer Science undergrad from India with a passion for coding and building things that make an impact. Skilled in Java, Data Structures and Algorithms (DSA), and web development, I love diving into problem-solving challenges and constantly learning. Right now, Iโm focused on sharpening my DSA skills and expanding my expertise in Java development.