Shallow Copy vs Deep Copy in Java
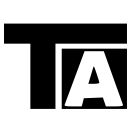
4 min read
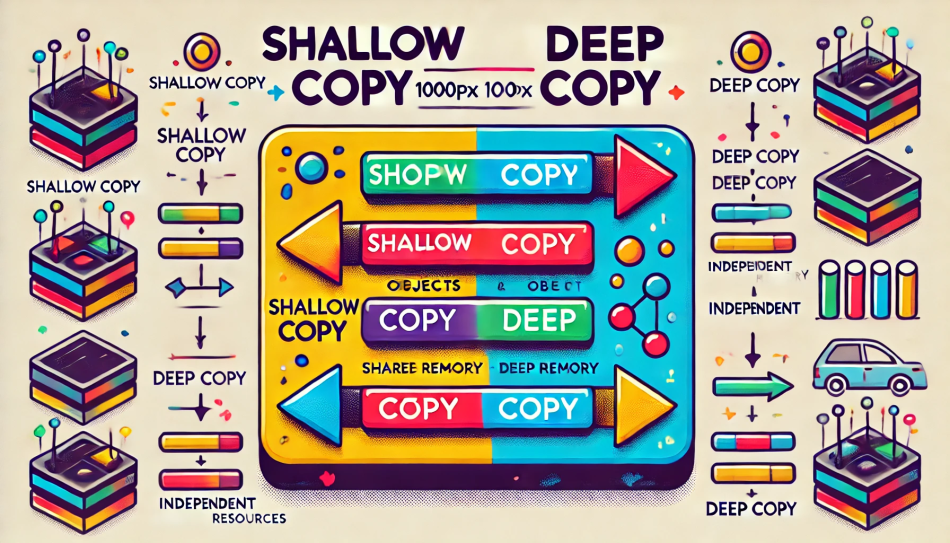
1. Shallow Copy in Java
A shallow copy of an object is a new object where the fields are copied directly from the original. But beware: for fields that are references, only the reference itself is copied, not the object it refers to. This section covers how to create a shallow copy and the implications of its use.
1.1 What is Shallow Copy?
A shallow copy duplicates the top-level structure of the original object, leaving any nested objects as references. It works well for simpler structures but can cause issues when objects are deeply nested.
1.2 How to Create a Shallow Copy
In Java, the clone() method (if implemented) is the primary way to perform a shallow copy. Here’s how it works:
class ShallowCopyExample implements Cloneable {
int value;
int[] array;
public ShallowCopyExample(int value, int[] array) {
this.value = value;
this.array = array;
}
@Override
protected Object clone() throws CloneNotSupportedException {
return super.clone();
}
}
public class Main {
public static void main(String[] args) throws CloneNotSupportedException {
int[] array = {1, 2, 3};
ShallowCopyExample original = new ShallowCopyExample(10, array);
ShallowCopyExample copy = (ShallowCopyExample) original.clone();
System.out.println("Original array before change: " + original.array[0]); // 1
copy.array[0] = 99;
System.out.println("Original array after change: " + original.array[0]); // 99
}
}
1.3 Pros and Cons of Shallow Copy
- Pros: Fast and memory-efficient, useful for simple objects.
- Cons: Not ideal for objects with nested or mutable references.
1.4 Common Mistakes with Shallow Copy
Failing to understand shallow copies can lead to bugs. For instance, when one element of a copied object is changed, and it inadvertently affects the original object. This section demonstrates how to avoid such pitfalls.
2. Deep Copy in Java
A deep copy duplicates an object and all objects it references, recursively. This method prevents unintentional changes, making it ideal for complex object structures.
2.1 What is Deep Copy?
A deep copy creates a distinct object where nested objects are also duplicated, ensuring no reference sharing. This guarantees complete independence between the original and copied objects.
2.2 How to Create a Deep Copy
Java does not natively support deep copying as easily as shallow copying, but it can be achieved through custom cloning methods or serialization. Here’s an example using a custom copy constructor:
class DeepCopyExample {
int value;
int[] array;
public DeepCopyExample(int value, int[] array) {
this.value = value;
this.array = array.clone();
}
}
public class Main {
public static void main(String[] args) {
int[] array = {1, 2, 3};
DeepCopyExample original = new DeepCopyExample(10, array);
DeepCopyExample copy = new DeepCopyExample(original.value, original.array);
System.out.println("Original array before change: " + original.array[0]); // 1
copy.array[0] = 99;
System.out.println("Original array after change: " + original.array[0]); // 1
}
}
2.3 Pros and Cons of Deep Copy
- Pros: Prevents unintended modifications to the original data.
- Cons: Can be slower and more memory-intensive than shallow copies, especially for large, deeply nested objects.
2.4 Common Mistakes with Deep Copy
Deep copying can lead to performance issues if not managed carefully. Explore various optimization techniques, such as avoiding redundant deep copies where not necessary.
3. When to Use Shallow Copy vs. Deep Copy
This section provides guidelines on when to choose shallow vs. deep copying. For example, use shallow copies for simple data transfers, while deep copies are preferable for data manipulation where integrity is essential.
4. Conclusion
Understanding shallow and deep copies helps prevent bugs in Java and ensures that object manipulation is both efficient and intentional. Are you ready to implement these techniques in your projects? If you have questions or need further clarification, feel free to leave a comment below!
Read more at : Shallow Copy vs Deep Copy in Java
0
Subscribe to my newsletter
Read articles from Tuanhdotnet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
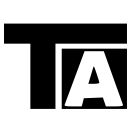
Tuanhdotnet
Tuanhdotnet
I am Tuanh.net. As of 2024, I have accumulated 8 years of experience in backend programming. I am delighted to connect and share my knowledge with everyone.