MongoDB Cheat Sheet
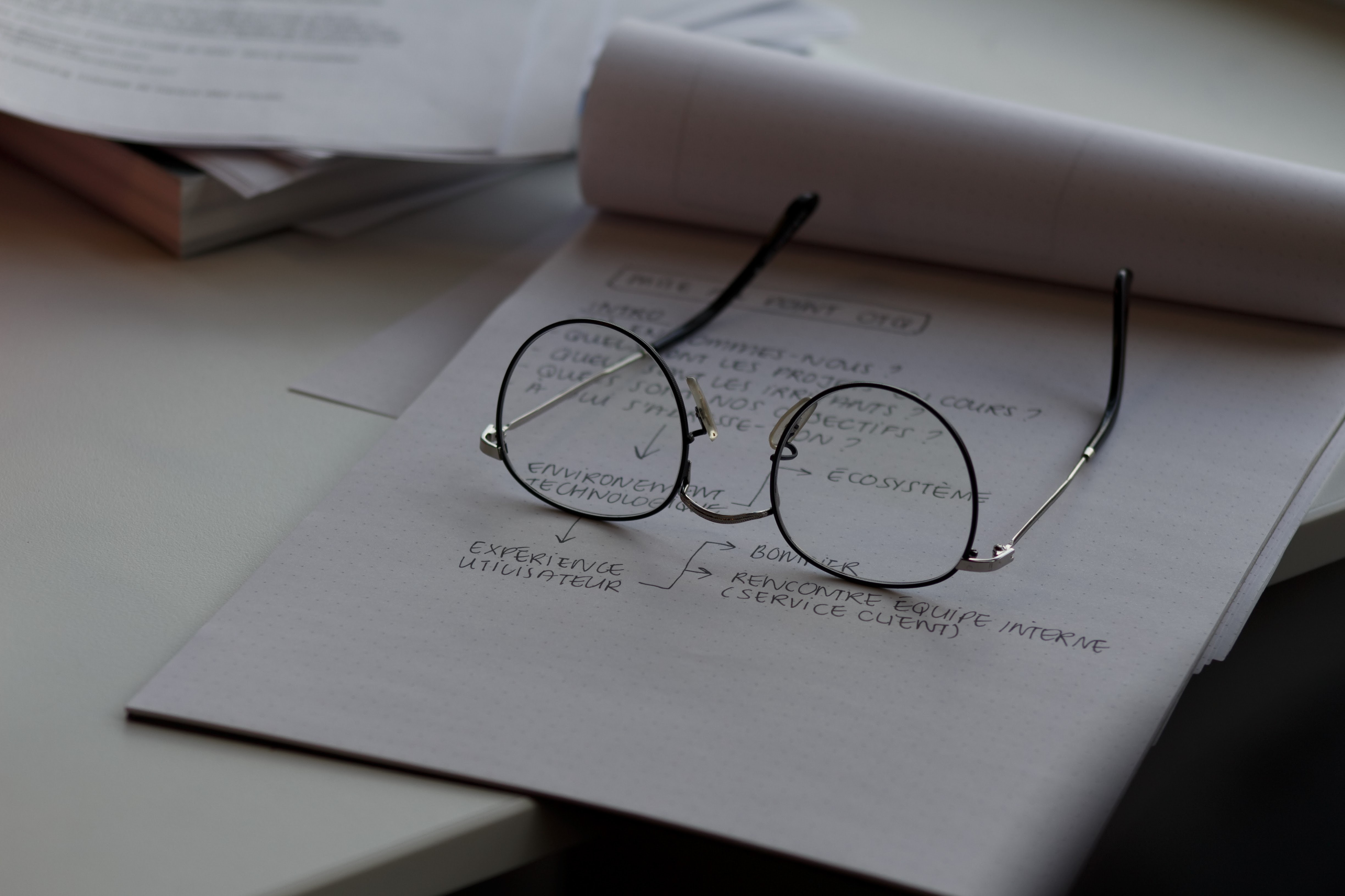

I’ve been working with MongoDB since the beginning of my career, and I finally decided to put together a cheat sheet— I’ve created for myself and others who might need a quick reminder.
While brushing up on MongoDB recently, I realized how helpful it would be to have everything in one place. So here it is—a handy, practical reference that I’ll keep updating as I go.
Hope you find it useful!
🔹 Basic Commands
show dbs // List all databases
use dbName // Switch to (or create) a database
show collections // List all collections in the current DB
🔹 Inserting Data
db.collection.insertOne({name: "Ram"})
db.collection.insertMany([{name: "John"}, {name: "Jane"}])
🔹 Reading Data
db.collection.find() // Find all documents
db.collection.findOne({name: "Ram"}) // Find one document
db.collection.find().limit(5) // Limit number of results
db.collection.find().forEach(doc => print(doc)) // Loop through documents
db.collection.countDocuments() // Count documents
🔹 Updating Data
db.collection.updateOne(
{ name: "Ram" },
{ $set: { idCards: { hasPancard: true, hasAdharCard: true } } }
)
db.collection.updateMany(
{},
{ $set: { hobbies: "anime" } }
)
🔹 Deleting Data
db.collection.deleteOne({ name: "Ram" })
db.collection.deleteMany({}) // Deletes all documents in collection
🔹 Query Operators
$lt // Less than
$lte // Less than or equal to
$gt // Greater than
$gte // Greater than or equal to
$in // Matches any value in an array
$nin // Not in
$not // Not operator
$and // Logical AND
$or // Logical OR
$nor // Logical NOR
🔹 Other Useful Commands
db.collection.drop() // Drop a collection
db.dropDatabase() // Drop the current database
🔹 Concepts
Schemaless: MongoDB collections don’t enforce document structure.
Data types: String, Number, Boolean, Array, Object, Date, etc
Subscribe to my newsletter
Read articles from Nidhin M directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
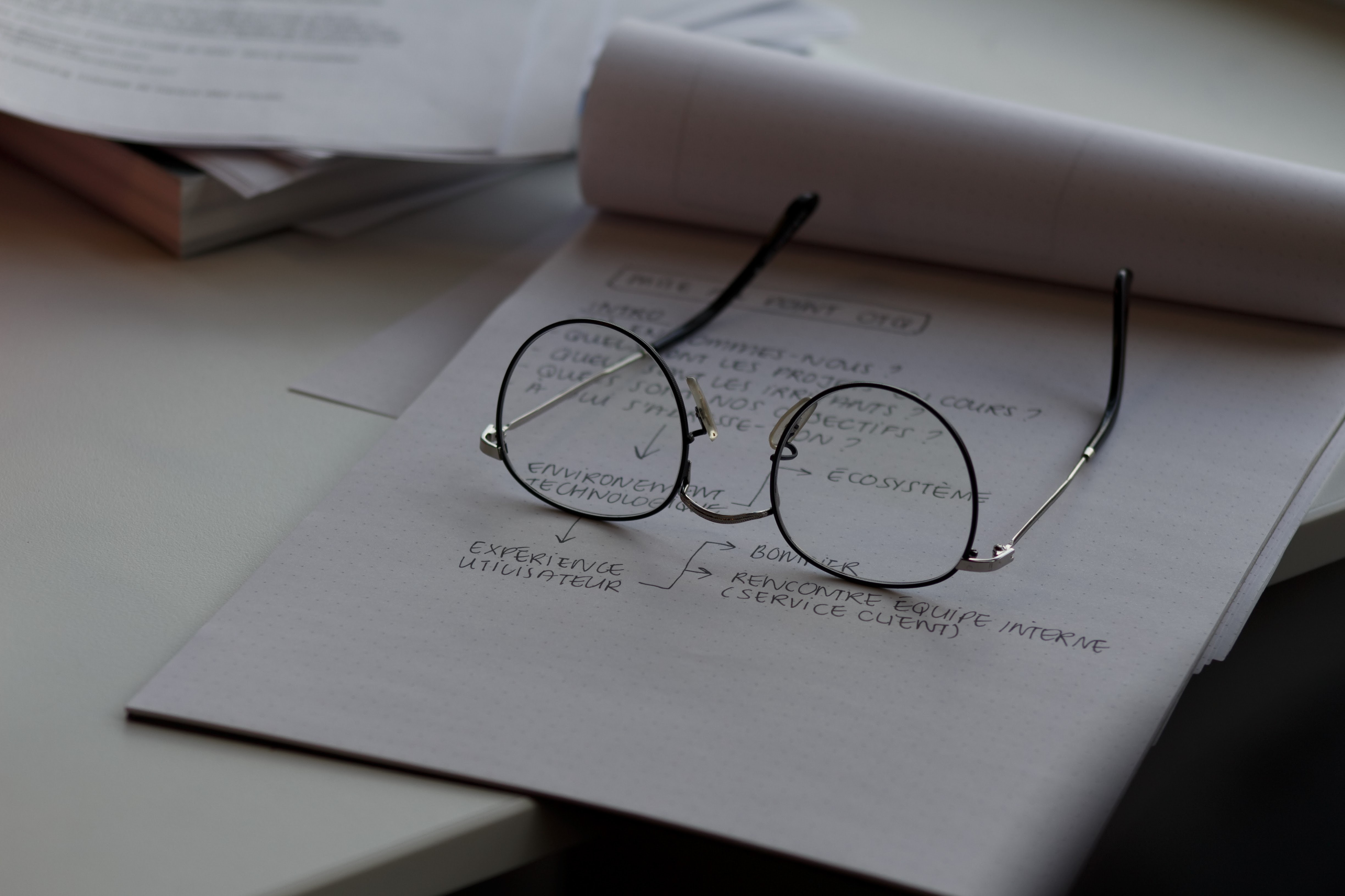
Nidhin M
Nidhin M
Hey there! I'm a passionate software developer who thrives in the TypeScript world and its companions. I enjoy creating tutorials, sharing tech tips, and finding ways to boost performance. Let's explore coding and tech together! 🚀