Protocols: Nothing Works Without Rules
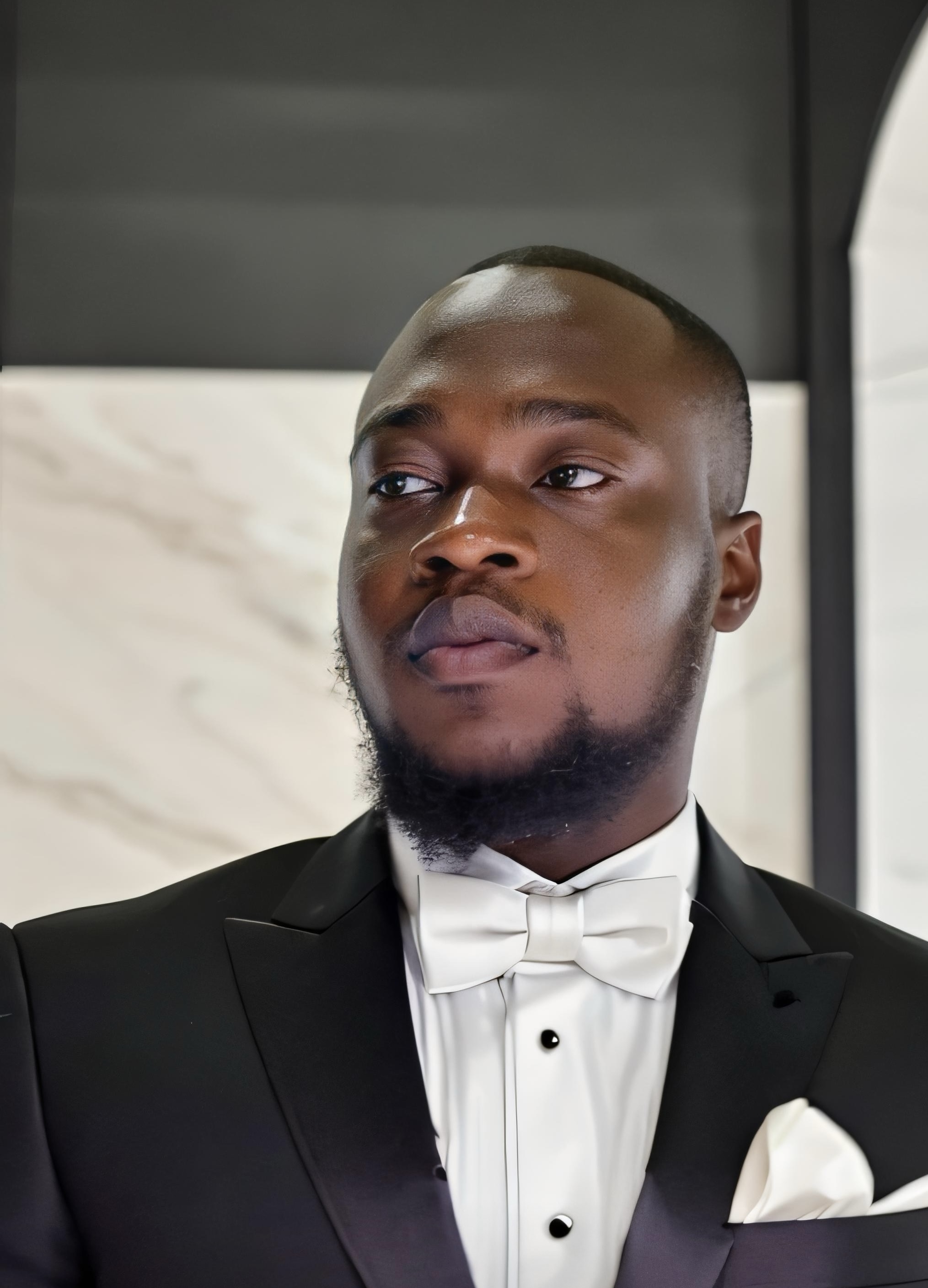
Table of contents

If you've spent any time in the trenches of software engineering, especially wrestling with distributed systems or large-scale architectures, you know that complexity is the name of the game. We build layers upon layers, abstractions over abstractions, trying to tame the beast. But have you ever stopped to think about the absolute bedrock upon which all this complexity rests?
It’s not design patterns, not fancy frameworks or syntactic sugar, just good old rules. It's something much more fundamental, almost invisible, yet utterly pervasive: Protocols. They are really just those invisible boundaries and structures that define how things should work, and more importantly, how they shouldn’t.
Lately, I’ve been reflecting a lot on the core of our discipline, and I’ve come to a conclusion that feels both obvious and philosophical at the same time: everything is protocol. Strip away all the abstraction, peel back the layers of your clean architecture, and what you’ll find underneath. if it’s well-engineered, it is a set of protocols, a defined, agreed-upon ways of interaction.
Introduction
Protocols aren't just about HTTP
requests or TCP
handshakes; they are the fundamental rule sets that govern any interaction, from the subatomic level to sprawling global networks. They are the source of immense power, enabling collaboration and complexity (the "Good"), but also the origin of frustrating constraints, baffling bugs, and security nightmares (the "Evil"). They are, quite literally, how anything is built on anything.
At the simplest level, a protocol is a rule or set of rules that define how entities communicate and interact. In software, that could be as high-level as HTTP or as low-level as TCP/IP. But this idea goes beyond networking.
Consider object-oriented programming, what is an interface if not a protocol? A promise: “Any class that implements this will behave this way.” Same thing with APIs. Same thing with serialization formats. Protocols are everywhere. They are not optional, and they are not “nice to haves”. They are the very things that enable systems to be built on top of one another.
What Are We Really Talking About When We Say "Protocol"?
Forget RFCs and formal definitions for a second. At its heart, a protocol is simply an agreement. It's a set of rules that defines how two or more entities will interact. Think about the simplest human interaction: a handshake.
Initiation: One person extends their hand.
Syntax: The hand is usually open, palm facing inwards or slightly up.
Response: The other person mirrors the action, extending their own hand.
Semantics: The hands clasp. This signifies greeting, agreement, or farewell.
Action: A brief shake (the timing and pressure are also subtle parts of the protocol!).
Termination: The hands release.
Break any of these implicit rules, and the interaction feels off, fails, or conveys a different message entirely. Offer a closed fist, hold on too long, use the wrong hand in some cultures – you've violated the protocol.
This simple example highlights the core components of any protocol, whether social or technical:
Syntax: The structure or format of the messages/actions (e.g., the layout of bits in a network packet, the required fields in a JSON payload, the posture of a handshake).
Semantics: The meaning of the messages/actions (e.g.,
SYN
means "I want to connect,"200 OK
means "Request successful," a clasped hand means "Greeting acknowledged").Timing/Ordering: When messages/actions should happen and in what sequence (e.g., you must send a
SYN
before anACK
in TCP, you offer your hand before shaking).
Without these agreed-upon rules, communication and interaction descend into chaos. Nothing gets built. Nothing functions.
Read more on how Stateless HTTP protocol was built on TCP here.
What are these rules?
These can be low-level like TCP, or application-level like gRPC or GraphQL. You can even think of REST conventions or Kafka message schemas as protocols.
Take this example of a client-server interaction over HTTP:
GET /api/users HTTP/1.1
Host: example.com
Accept: application/json
Authorization: Bearer token123
If the server doesn’t follow the HTTP protocol, and maybe responds with a malformed header, your well-behaved client might crash or throw a cryptic error. The contract is broken.
Protocols are beautiful because they create predictability. Predictability means stability, and in a large system, that’s gold.
But protocols are also strict. They don’t care about your business logic or your fancy framework. If the data doesn’t conform, the request is rejected. If a handshake isn’t done right, the connection dies. If the quorum isn’t met, no consensus is reached. It’s brutal, but it’s necessary.
I’ve seen well-meaning developers treat protocols as if they were guidelines. That’s a mistake. Protocols aren’t guidelines. They’re contracts. Break them, and the consequences range from silent failures to catastrophic outages.
For example, consider the following rust code which expects a json response (contract):
use reqwest::header::ACCEPT;
use std::error::Error;
#[tokio::main]
async fn main() -> Result<(), Box<dyn Error>> {
let client = reqwest::Client::new();
let res = client
.get("https://malformed.example.com/api/user/42")
.header(ACCEPT, "application/json")
.send()
.await;
match res {
Ok(response) => {
if response.status().is_success() {
let json = response.json::<serde_json::Value>().await;
match json {
Ok(data) => println!("User profile: {:#?}", data),
Err(e) => eprintln!("⚠️ Failed to parse JSON: {}", e),
}
} else {
eprintln!("❌ HTTP error: {}", response.status());
}
}
Err(e) => {
eprintln!("🚨 Protocol error: {}", e);
// e.g. "error reading response headers: invalid HTTP header"
}
}
Ok(())
}
This code looks innocent and robust. You handle errors based on status codes and expect a clean JSON body.
But here’s what could break everything:
Scenario:
The server responds like this:
HTTP/1.1 200 OK
Content-Type: application/json
Content-Length: 32
X-Custom-Header
{ "name": "Sofwan", "role": "Admin" }
Notice that malformed header? X-Custom-Header
is missing a value.
What happens?
The HTTP parser chokes.
fetch()
throws a low-level error likeSyntaxError: Unexpected end of JSON input
or worse, a native crash.Your graceful business logic never gets a chance to run.
Lesson:
Your client followed the rules.
Your code was clean.
But the server broke the protocol contract.
And the protocol doesn’t bend. There’s no “maybe” or “almost correct.” It either complies or it fails—hard.
One time, in a project where we were building a real-time event pipeline, we switched from one message broker to another, both supporting the same messaging semantics. Except, as it turned out, one of them was more strict about message acknowledgment order. That slight difference in protocol adherence exposed a lurking race condition that had been hiding in our consumer logic for weeks. We only found out because production messages started disappearing. Just like that.
Protocols in Engineering: The Lifeblood of Distributed Systems
Nowhere is the power and peril of protocols more evident than in distributed computing. When you have multiple machines, potentially separated by unreliable networks, trying to coordinate and achieve a common goal, unambiguous, robust protocols are not just helpful; they are essential.
Consensus Protocols (Raft, Paxos): How do multiple nodes agree on a value or a state transition, even if some nodes crash or messages get lost? These protocols are incredibly intricate sets of rules defining message types (AppendEntries, RequestVote), state transitions, and leader election logic. Get the protocol implementation slightly wrong, and you get split-brain scenarios, data corruption, or total system unavailability.
Protocols like Raft are designed to be unambiguous, because ambiguity in distributed consensus leads to split-brain scenarios, data loss, or total collapse. An example is,if self.state == Follower && election_timeout_expired() { self.state = Candidate; self.current_term += 1; self.votes = 1; // vote for self broadcast_vote_request(); }
This is not a simple if-statement. It’s part of a carefully defined state machine, where every node must transition the same way for the cluster to remain consistent.
Replication Protocols: How does a primary database node ensure its replicas have the same data? Synchronous, asynchronous, semi-synchronous replication – these are all protocols defining the interaction and guarantees between the primary and its followers.
Messaging Protocols (AMQP, MQTT, Kafka Protocol): How do producers send messages and consumers receive them reliably and efficiently via a broker? These protocols define message formats, delivery guarantees (at-least-once, at-most-once, exactly-once – each a different protocol!), acknowledgments, and topic/queue semantics.
Remote Procedure Call (RPC) Protocols (gRPC, Thrift): How does one service invoke a function on another service across a network as if it were local? These involve protocols for serialization (Protocol Buffers, Avro – protocols themselves!), request/response mapping, error handling, and connection management.
API Contracts (REST, GraphQL): While often seen as architectural styles, the specific way you structure your URLs, use HTTP verbs, format your JSON/GraphQL queries and responses is a protocol between your frontend and backend, or between microservices. A poorly defined or inconsistently implemented API protocol leads to endless integration headaches.
In distributed systems, protocols are the invisible threads holding everything together over a chasm of network latency and potential failures. You cannot fake it. You cannot fake correctness. Either your protocols are sound, or your system is broken. They are the system, in many ways.
The Grand Tapestry: Anything Built on Anything
It’s almost poetic, right? But it’s also very literal in software engineering. This is where the magic, and sometimes the madness, truly lies. Our world, both natural and artificial, is a stack of protocols. It’s no exaggeration to say that the internet itself is a stack of protocols, beautifully layered, each one building on the constraints and guarantees of the one below. That’s not an accident. That’s engineering.
Think about physics. The fundamental forces and particles interact according to strict rules (protocols). These rules allow atoms to form. The rules governing atomic interactions (chemistry protocols) allow molecules to form. Molecular interactions (biochemical protocols) allow cells to function. Cell interactions allow organisms. Organism interactions (social protocols, language) allow societies.
It’s protocols all the way down.
Now, map this to our world of software engineering (using the OSI model)
Physical Layer: How voltages or light pulses represent bits on a wire or fiber. That's a protocol.
Data Link Layer: How bits are grouped into frames, how to detect errors, how to manage access to the physical medium (e.g., Ethernet protocol). Built on the physical layer protocol.
Network Layer: How to route packets across multiple networks (e.g., IP protocol). Built on the data link layer protocol. It doesn't care if it's Ethernet or Wi-Fi underneath, as long as the lower layer adheres to its expected protocol.
Transport Layer: How to provide reliable (TCP) or unreliable (UDP) end-to-end communication, manage flow control, and segment data. Built on the network layer protocol.
Application Layer: How specific applications communicate (e.g., HTTP for web, SMTP for email, gRPC for RPC). Built on the transport layer protocol.
Each layer relies on the guarantees provided by the layer below it, interacting with it through a well-defined protocol (an interface, essentially). It abstracts away the details of the lower layers, allowing engineers working on the Application Layer (like many of us) to think about application logic without worrying about voltage levels or frame collisions.
This layering, enabled entirely by protocols, is the only reason we can build systems as complex as the modern internet or large-scale distributed databases. Imagine trying to write a web application if you had to manually manage packet routing and error correction for every single request! Protocols are the great abstraction enablers.
A message broker speaks AMQP or MQTT. Your backend talks JSON over HTTPS. Inside your services, gRPC messages dance over HTTP/2. Below all of that, it's TCP. Beneath that, IP. Below that, Ethernet. Each layer is built on a protocol, defined to the letter, specifying behaviour, expectations, constraints.
Systems can only interoperate if they speak the same language, and the language is defined by a protocol.
Take distributed systems for example. The moment you split a system across network boundaries, you’ve walked into a land ruled by protocols. Consistency? Availability? Partition tolerance? These CAP theorem elements are not abstract ideas, they manifest in how your nodes agree (consensus protocols), how they replicate (replication protocols), how they detect faults (heartbeat protocols), and how they recover (failure and healing protocols).
Take the following code snippet for example
// Application Layer – Developer's perspective
async function sendWelcomeEmail(user: User) {
const message = {
to: user.email,
subject: "Welcome to Our Platform",
body: `Hi ${user.name}, thanks for joining us!`
};
// Message is serialized into JSON over HTTPS
await fetch("https://email-service.internal/api/send", {
method: "POST",
headers: {
"Content-Type": "application/json",
"Authorization": `Bearer ${process.env.API_TOKEN}`
},
body: JSON.stringify(message)
});
}
That looks simple, right? But here’s what’s actually happening underneath:
HTTP Layer (Application → Transport)
The
fetch()
call constructs an HTTP request—following the HTTP/1.1 or HTTP/2 protocol spec.Headers are formatted, body is encoded, request line is created.
TLS Layer (Transport → Network)
- If HTTPS is used, TLS handles encryption, handshake, and certificate validation—following the TLS protocol.
TCP Layer
- Below that, the request is chunked into packets and sent over TCP, which manages ordering, packet loss, and retry mechanisms.
IP Layer
- TCP hands data to IP, which handles addressing and routing packets across networks.
Link Layer (Ethernet)
- The network adapter frames the IP packets and sends them as electrical signals or photons using Ethernet or Wi-Fi protocols.
On the flip side;
If the TLS handshake fails, the entire request fails.
If TCP drops a packet, but retries work, the developer never notices.
If Ethernet collisions aren’t handled by the protocol, your entire application breaks.
That’s the beauty of protocol layering: every layer abstracts away the horror of the layer beneath it, while strictly enforcing contracts.
And if any layer doesn’t speak the exact expected protocol? Miscommunication. Failure. Silence.
The Protocols We Create
Protocols aren’t just things we consume, they’re also things we design. When you're designing a protocol, be it an internal API, an event contract, or a distributed consensus mechanism, you are shaping the interface between people.
You create a REST API? That’s a protocol. You publish a Kafka event format? That’s a protocol. You define a SQL schema? That’s a protocol between your code and the database.
Here’s a mini example of a custom internal protocol for idempotent request handling:
POST /api/charge HTTP/1.1
Idempotency-Key: a5c7efb4-91f4-11e5-bf7f-feff819cdc9f
Content-Type: application/json
The contract:
If the same
Idempotency-Key
is received again, return the original result.Must store response against the key for a period of time.
Must hash body to detect replay attacks.
If your backend ignores any part of this protocol, duplicate charges could happen. Or worse, undetectable bugs.
Great engineers don’t just design systems. They design agreements that enable systems to survive change, growth, and human error. And that’s what protocols really are.
The Engineer's Burden: Crafting Better Rules
As software engineers, particularly those working on distributed or foundational systems, we are often not just consumers of protocols, but also designers. Whether defining an API contract, creating an internal RPC mechanism, or developing a new distributed algorithm, we are crafting the rules of interaction.
This is a significant responsibility. A poorly designed protocol can inflict pain for years, hindering development, causing production issues, and limiting future evolution. Contrary, a clean, well-defined, extensible protocol is a gift to future developers (including our future selves).
What makes a "good" protocol?
Clarity & Unambiguity: Leave no room for interpretation. Define states, transitions, message formats, and error conditions precisely.
Simplicity (where possible): Favor simplicity unless complexity is truly justified by the requirements.
Extensibility: Think about future evolution. How will you add features? How will you version the protocol? (e.g., using feature flags, well-defined version negotiation).
Efficiency: Consider the performance implications – serialization overhead, number of round trips, etc.
Robustness: Define how errors are handled. What happens if a message is lost, duplicated, or corrupted?
Security: Build security considerations in from the start, don't bolt them on later.
Conclusion: Masters of the Rules
Protocols are the invisible architecture of our connected world and our complex software systems. They are the embodiment of the "anything built on anything" principle, enabling layers of abstraction and interoperability that make modern technology feasible. They are the source of immense "Good," allowing systems to communicate, coordinate, and scale.
But they also carry the potential for "Evil" – the rigidity of legacy, the complexity that breeds bugs, the ambiguities that cause friction, and the vulnerabilities that expose us.
As engineers, understanding protocols isn't just about knowing TCP vs. UDP or REST vs. gRPC. It's about recognizing the fundamental role of agreed-upon rules in any system we build. It's about appreciating the trade-offs inherent in their design and striving to be thoughtful, meticulous creators of the rules that will govern the interactions within our own complex creations. Because ultimately, the quality of our systems often comes down to the quality of the protocols holding them together.
Subscribe to my newsletter
Read articles from Sofwan A. Lawal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
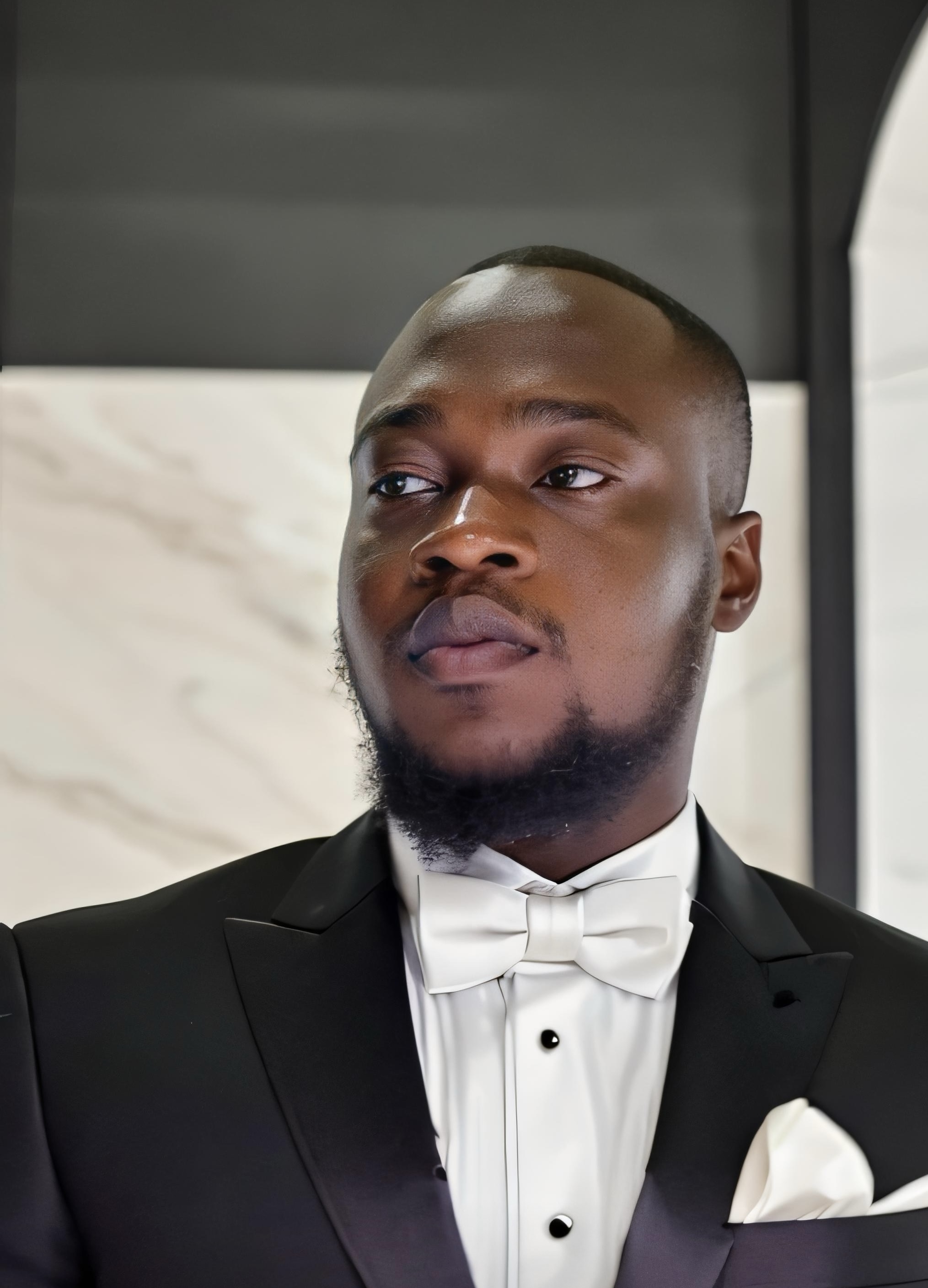
Sofwan A. Lawal
Sofwan A. Lawal
I am a seasoned Software Engineer with over 8 years of diverse experience, primarily focusing on Backend Software Engineering and Complex systems.