How I Cut My App’s Build Size by 50% in Flutter
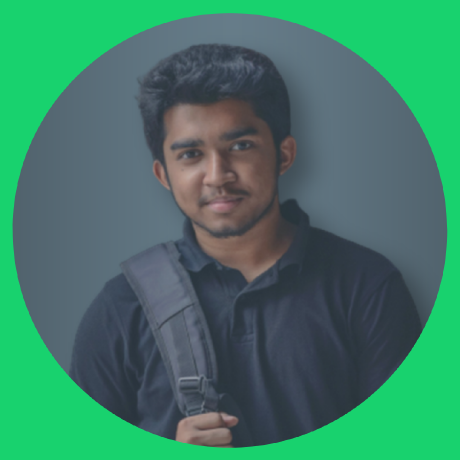

Build size is one of the most critical aspects of mobile app development, especially if you're targeting users in regions with limited internet access or data caps. Recently, I managed to reduce my Flutter app’s release build size by nearly 50%, and in this post, I’ll share exactly how I did it — step by step.
Why Build Size Matters
A bloated app build not only takes longer to download but can also impact your app’s install rate and even ranking on app stores. Optimizing your app size leads to:
Faster downloads
Lower storage usage
Better performance on low-end devices
Improved user experience
Initial Build Size Analysis
To start, I ran the following command to analyze my app's current size:
flutter build apk --analyze-size
This generated a .json
file I could open in the Flutter DevTools size analyzer.
Results:
Initial APK size: 36.2 MB
After optimizations: 18.1 MB
Let’s break down the optimizations I applied.
1. Remove Unused Packages
I checked my pubspec.yaml
and removed unused dependencies:
# Before
dependencies:
http:
provider:
firebase_analytics:
charts_flutter:
flutter_html:
I removed charts_flutter
and flutter_html
, which were not in active use. Then ran:
flutter pub get
Savings: ~2.5 MB
2. Use Tree Shaking for Icons
If you're using MaterialIcons
, Flutter includes the whole icon set by default.
To fix this, I used flutter_launcher_icons
and custom icon fonts instead of importing the entire icon library.
Also, add this in pubspec.yaml
:
flutter:
uses-material-design: false
Savings: ~1.8 MB
3. Split Per ABI
Instead of generating a fat APK that supports all architectures, I created APKs per ABI:
flutter build apk --split-per-abi
This gave me separate APKs for armeabi-v7a
, arm64-v8a
, and x86_64
.
Savings: Up to 60% for specific architectures
4. Compress and Resize Assets
I had several high-res images in the assets folder. I:
Compressed PNGs using TinyPNG
Resized large images
Used WebP format where possible
Savings: ~3.2 MB
5. Use ProGuard (for Android)
ProGuard helps strip unused code from dependencies. I enabled it by editing android/app/build.gradle
:
buildTypes {
release {
shrinkResources true
minifyEnabled true
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
Savings: ~4 MB
6. Remove Debug Info
Always build with the --release
flag and ensure no debug info is bundled.
flutter build apk --release
Also, avoid logging in production builds.
7. Use Deferred Components (Optional)
Flutter now supports deferred components (Android only), letting you load parts of the app on demand. This requires setup on both the Flutter and Android side.
More here: Flutter Deferred Components
Final Thoughts
Cutting down your Flutter app's build size doesn't have to be rocket science. Start with an analysis, then iterate through removal of unused packages, asset optimization, ABI splitting, and enabling ProGuard.
Total size saved: ~18 MB (50%)
If your users are in bandwidth-sensitive markets, this can make a huge difference in adoption and retention.
Over to You!
Have you tried optimizing your Flutter app size? Got any other tricks up your sleeve? Let me know in the comments or connect with me.
Subscribe to my newsletter
Read articles from Emran Khandaker Evan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
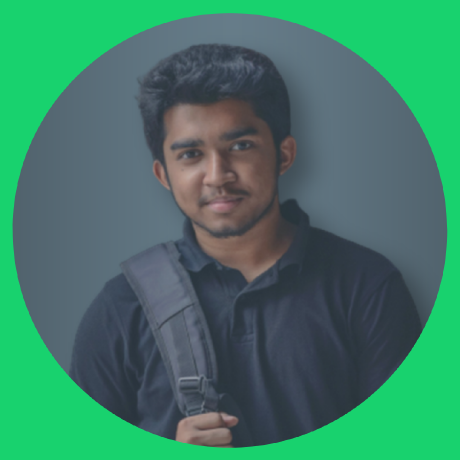
Emran Khandaker Evan
Emran Khandaker Evan
Software Engineer | Content Creator