That Moment When XML Just… Felt Wrong 😩
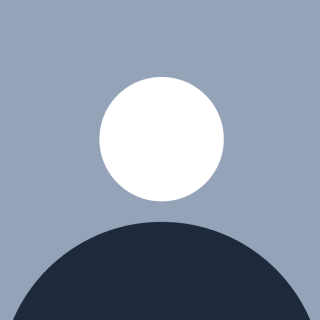

Okay, so I’ll admit it — I was that developer, clinging to XML like it was some kind of safety blanket. Layout files here, findViewById
there, and don't even get me started on updating views when state changed. It was all... too much.
Then I met Jetpack Compose. And yeah, the learning curve? Definitely a thing. But once it clicked, the way I built UIs completely changed.
Let me break it down from the beginning — from the point where everything started to make sense.
UI That’s All About Description, Not Construction 🛠️
Here’s the first thing that blew my mind: in Compose, you don’t build the UI. You describe it.
Like, literally:
@Composable
fun Greeting(name: String) {
Text("Hey there, $name!")
}
That’s it. That’s your UI. You tell Compose what it should look like given some state, and it figures out the how. No more manually updating views, no more fighting RecyclerView just to get something dynamic on screen.
It’s declarative UI, and it’s a whole different mindset.
Composables Are Like Recipes 🍲 (But Don’t Spill the Sauce)
Here’s what I didn’t get at first: these @Composable
functions? They’re meant to be idempotent. Fancy word, but it just means this:
Given the same input, they always produce the same output.
No side effects. Don’t sneak in a network call or a toast — that’ll backfire. Trust me 🙃
I totally messed that up early on. Tried fetching data inside a composable... let’s just say that function got called a lot, and not when I wanted.
The Composer Is the Real MVP 🧠
Behind the scenes, there's this mysterious object called the Composer. It tracks what UI is currently on screen, what data it's using, and when that data changes, it figures out exactly what needs to be redrawn.
And guess what? It doesn’t redraw everything. That’s where recomposition comes in.
Let’s say I have this:
@Composable
fun MyProfile(name: String, isOnline: Boolean) {
Text(text = if (isOnline) "$name is online" else "$name is offline")
}
If only isOnline
changes, Compose doesn’t rebuild everything else. It’s smart like that. It skips the stuff that didn’t change — which means faster UI, better performance, and fewer headaches for me 😌
Composition: The UI Tree You Never See 🌲
When Compose runs your @Composable
functions, it builds something called a Composition. Think of it like an invisible blueprint of your UI. Every piece of UI you describe becomes a node in this tree.
If you've ever seen a UI hierarchy in Android Studio — imagine that, but faster, lighter, and built completely in memory.
Also cool? Each setContent {}
in your app gets its own Composition. So everything stays scoped and clean.
TL;DR for My Fellow Scroll-Through-ers 🏃♂️
Compose = declarative UI → You describe the UI with Kotlin functions.
Composable functions should be stateless and idempotent.
The Composer tracks state and optimizes what gets redrawn.
Composition is the internal tree structure of your UI.
Recomposition updates only what's changed — no more full redraws 🔥
What tripped you up when first trying Compose? Or if you haven't tried it yet… what's stopping you? 👇
Let's chat in the comments — I’m still learning too! 😊
Subscribe to my newsletter
Read articles from Kavearhasi Viswanathan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by