TS2316: Global type '{0}' must be a class or interface type
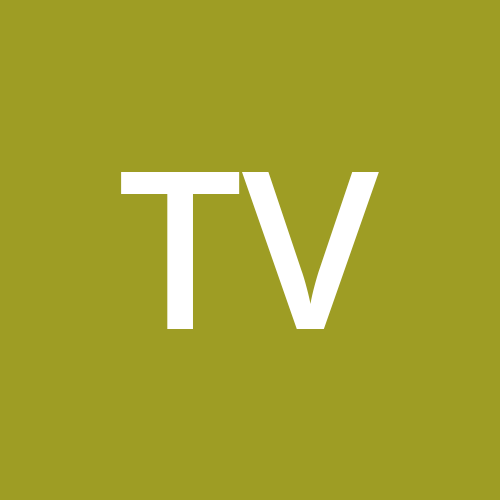
TS2316: Global type '{0}' must be a class or interface type
Introduction to TypeScript and Types
TypeScript is a powerful, statically typed superset of JavaScript. Put simply, TypeScript builds on JavaScript by allowing developers to add type annotations, making their code more robust, maintainable, and less prone to runtime errors. Since TypeScript is a superset language, all valid JavaScript code is valid TypeScript code, meaning you can incrementally adopt it into existing JavaScript projects.
One of the foundational concepts in TypeScript is the notion of types. In TypeScript, a type defines the shape of data — it indicates what kind of value a variable, parameter, or return value should have. For example, string
is a type that ensures a value is text, and number
is a type for numerical values.
If you're just starting with TypeScript or want to sharpen your coding skills further, make sure to follow our blog for more examples and explanations, and check out GPTeach to start learning how to code effectively using AI-driven tools.
What Are Interfaces in TypeScript?
Before diving into the error, TS2316: Global type '{0}' must be a class or interface type, let’s first address what interfaces are. Interfaces in TypeScript are used to define the structure or "shape" of an object. They provide a set of rules or a contract that objects must adhere to. By using interfaces, you can create more readable, reusable, and scalable code.
For example:
interface User {
name: string;
age: number;
}
const user: User = {
name: "Alice",
age: 30,
};
Here, the User
interface ensures that user
has both a name
(of type string
) and an age
(of type number
). If this structure isn't followed, TypeScript will throw an error. Interfaces are commonly used for object type annotations, adding stricter type-checking to your codebase.
Understanding TS2316: Global type '{0}' must be a class or interface type
What Does This Error Mean?
The compiler error TS2316: Global type '{0}' must be a class or interface type occurs when TypeScript expects a class or interface type in a specific context, but it encounters something invalid instead. This problem is often tied to the way developers define or use types.
To explain it in simpler terms: TypeScript is trying to ensure that what you're working with (typically an object or structure) is well-defined, using either a class
or an interface
. If what you're providing doesn't meet this requirement, the TypeScript compiler throws this error.
Code Example: Reproducing the Error
Here's a simple example of how TS2316: Global type '{0}' must be a class or interface type might occur:
type Shape = "circle" | "square";
class Circle {
radius: number;
constructor(radius: number) {
this.radius = radius;
}
}
class Square {
side: number;
constructor(side: number) {
this.side = side;
}
}
// Attempt to define a class or extend something invalid
class MyShape extends Shape {} // Error: TS2316
In the above example, Shape
is defined as a union type (a special type operator that allows multiple types), not a class or interface. When you try to extend it using class MyShape extends Shape
, the compiler throws the error TS2316: Global type '{0}' must be a class or interface type.
Fixing the Error: TS2316: Global type '{0}' must be a class or interface type
There are a few ways to fix this problem based on how you're trying to structure your code:
Use a Class or Interface Instead of Union Types
If you're trying to extend functionality, you need to use a class or an interface:interface Shape { getArea(): number; } class Circle implements Shape { radius: number; constructor(radius: number) { this.radius = radius; } getArea(): number { return Math.PI * this.radius * this.radius; } } class Square implements Shape { side: number; constructor(side: number) { this.side = side; } getArea(): number { return this.side * this.side; } }
Here, the
Shape
interface is used to define a common contract (getArea()
) that each class must adhere to. There’s no conflict, and you avoid TS2316 entirely.Avoid Extending Non-Class/Interface Types
If you don't need polymorphism, simply avoid extending something that's not a class or interface:type Shape = "circle" | "square"; // No `extends` needed for this use case function printShape(shape: Shape): void { console.log("Shape:", shape); }
In this case, the
Shape
union type can be used as-is without inheritance since it was incorrectly being used to extend functionality.
Important to Know!
Interfaces vs. Types: While interfaces and types in TypeScript share some similarities, interfaces are typically used for object and class definitions, while
type
is used for unions, intersections, and primitive annotations.Extending Classes and Interfaces: Always ensure that what you're extending is actually an interface or a class. You cannot extend primitive types (
number
,string
, etc.) or union types like ("circle" | "square"
).TS2316 Occurs During Compile-Time: This error is specifically a compile-time error. It won't show up in JavaScript because JavaScript doesn’t have the concept of
types
orinterfaces
. This is why TypeScript is so useful—it catches potential bugs or abuses of the language early on.
Frequently Asked Questions (FAQs)
What is the difference between type
and interface
in TypeScript?
type
can define a wide variety of constructs, like primitives, union types, arrays, or object shapes.interface
is specifically meant for describing the structure of an object or a class. Useinterface
when working with object shapes andtype
for more flexible utilities.
Why can't I extend union types or primitive types?
Union types like string | number
or primitives like string
don't have an inheritance hierarchy (they aren’t objects or structures). TypeScript requires a class
or interface
for inheritance because these define reusable object contracts.
How do I avoid TS2316: Global type '{0}' must be a class or interface type?
- Always ensure that what you're extending or implementing is an interface or class.
- When defining reusable logical types (like union types), don’t treat them as if they are part of an object-oriented hierarchy. Use functional or compositional approaches instead.
By understanding TS2316: Global type '{0}' must be a class or interface type and its context, you can write more robust TypeScript code and avoid common pitfalls around type misuse.
Subscribe to my newsletter
Read articles from Turing Vang directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
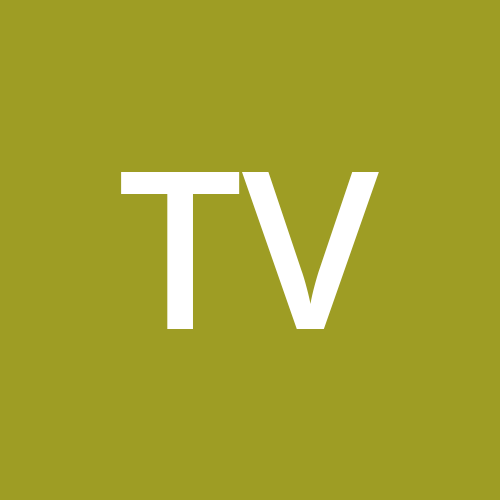