TS2317: Global type '{0}' must have {1} type parameter(s)
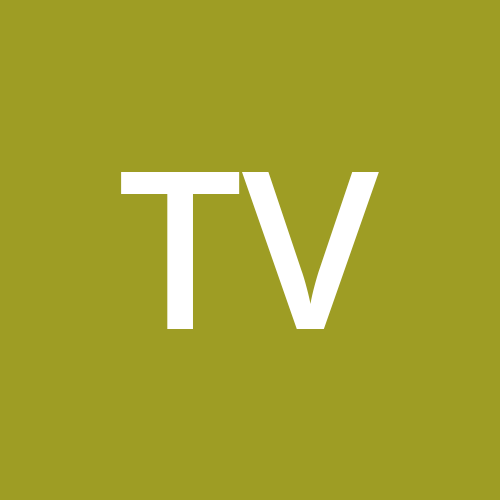
TS2317: Global type '{0}' must have {1} type parameter(s)
TypeScript is a strongly-typed superset of JavaScript that brings optional static typing to the language. Unlike JavaScript, which is dynamically typed, TypeScript allows developers to define types explicitly, making code more predictable, easier to debug, and safer. By introducing these type-checking capabilities, TypeScript helps prevent runtime errors before they occur. Types in TypeScript can represent everything from simple data structures like numbers and strings to complex constructs like interfaces, enums, and generics.
Types describe the shape and structure of data in TypeScript. They define how variables, functions, or objects should behave in your code, ensuring you use values as intended. For example, if you declare a variable as a string
, TypeScript ensures you don’t accidentally assign a number to it.
If you’re interested in mastering TypeScript or learning coding best practices using AI-powered tools, consider subscribing to our blog or exploring platforms like GPTeach to enhance your skills.
What are Types?
A "type" in TypeScript is a way of defining what structure or value a variable, function, or object must adhere to. It serves as a contract that ensures that operations are performed correctly. For example, defining a variable's type tells TypeScript what kind of value it can hold:
let age: number = 25; // age must be a number
let name: string = "Alice"; // name must be a string
Using types makes your code clearer and helps others (or even yourself) understand what functions or variables expect. Additionally, advanced types like generics allow you to write reusable and flexible code by defining constraints on various data structures.
TS2317: Global type '{0}' must have {1} type parameter(s)
The error TS2317: Global type '{0}' must have {1} type parameter(s)
occurs when a global generic type is used incorrectly with an invalid number of type parameters. Generic types (flexible types that accept parameters) in TypeScript are designed to take one or more type arguments to operate. When you fail to provide the required type parameters or provide an incorrect count, you encounter this error.
This error often occurs due to a mismatch between type definitions and their usage.
What Causes TS2317: Global type '{0}' must have {1} type parameter(s)?
To understand the error, we need to examine generic types. Generics act as placeholders for types, enabling the creation of reusable components that work with various data types. Here's a simple generic type:
type GenericType<T> = T[];
In this example, GenericType
requires one type parameter (<T>
), which represents the type for each element in the array. If you fail to provide or incorrectly supply the required parameter, the compiler generates the error: TS2317: Global type '{0}' must have {1} type parameter(s)
.
Example Error
Here’s a code snippet that causes the TS2317 error:
type MyArray<T, U> = [T, U];
let invalid: MyArray = [1, "string"];
This code produces the following error:
TS2317: Global type 'MyArray' must have 2 type parameter(s).
Why? Because MyArray
expects two type parameters (e.g., MyArray<number, string>
), but none are provided.
How to Fix TS2317: Global type '{0}' must have {1} type parameter(s)
To resolve this error, you need to ensure that you specify the exact number of type parameters required by the generic type. Here’s how you can fix the above code:
type MyArray<T, U> = [T, U];
let valid: MyArray<number, string> = [1, "string"]; // Correct usage
Now, TypeScript knows the types of both elements in the tuple, and the error is resolved.
Important to Know!
- Generic types are flexible and allow for reusable code, but they must adhere to their defined signature.
- If a type requires one or more parameters, all of them must be explicitly provided unless there is a default value set for the type parameters.
Common Scenarios for TS2317
Missing Type Arguments
type Pair<T, U> = [T, U];
let example: Pair; // Error: TS2317: Global type 'Pair' must have 2 type parameter(s).
Fix:
type Pair<T, U> = [T, U];
let example: Pair<number, string> = [3, "test"];
Excess Type Arguments
Some generic types have a fixed number of parameters, and providing more than required also leads to the TS2317 error.
type SingleParameter<T> = T;
let example: SingleParameter<number, string>; // Error: TS2317
Fix:
Ensure you follow the generic type’s expected signature:
type SingleParameter<T> = T;
let example: SingleParameter<number> = 42; // Correct usage
Important to Know!
- Generic types can be nested within other types or declarations. Understand the hierarchy of your types.
- Check the official TypeScript documentation or your custom type definition for the expected number of parameters to avoid this error.
FAQ: TS2317 Error
1. What are type parameters?
Type parameters are placeholders (like <T>
or <T, U>
) that allow generics to accept different types dynamically. For example, Array<T>
means the array can accept any type (T
) and operate on it.
2. Can I set default values for type parameters?
Yes! TypeScript allows you to define default values for type parameters, which can help you avoid the TS2317 error if no parameter is explicitly provided. For example:
type ReadonlyArray<T = string> = T[];
let example: ReadonlyArray = ["default"]; // Works due to default type 'string'
Conclusion
The TypeScript error TS2317: Global type '{0}' must have {1} type parameter(s)
occurs when you use generic types improperly with too few or too many type parameters. Generic types are one of TypeScript's most powerful features, enabling the creation of reusable and type-safe components. However, they require careful use to avoid such errors. Always consult the type’s signature and ensure the correct number of type arguments are supplied.
If you’re passionate about learning TypeScript in-depth or want to harness tools like AI to polish your coding skills, visit GPTeach or subscribe to this blog for more tips and tutorials.
Subscribe to my newsletter
Read articles from Turing Vang directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
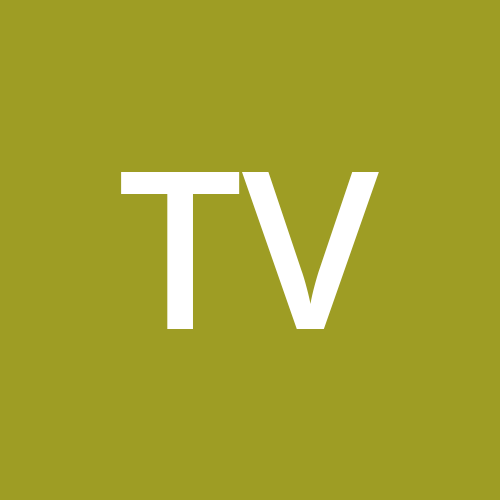