Caching in Java Using Caffeine: A Comprehensive Guide.
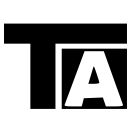
3 min read
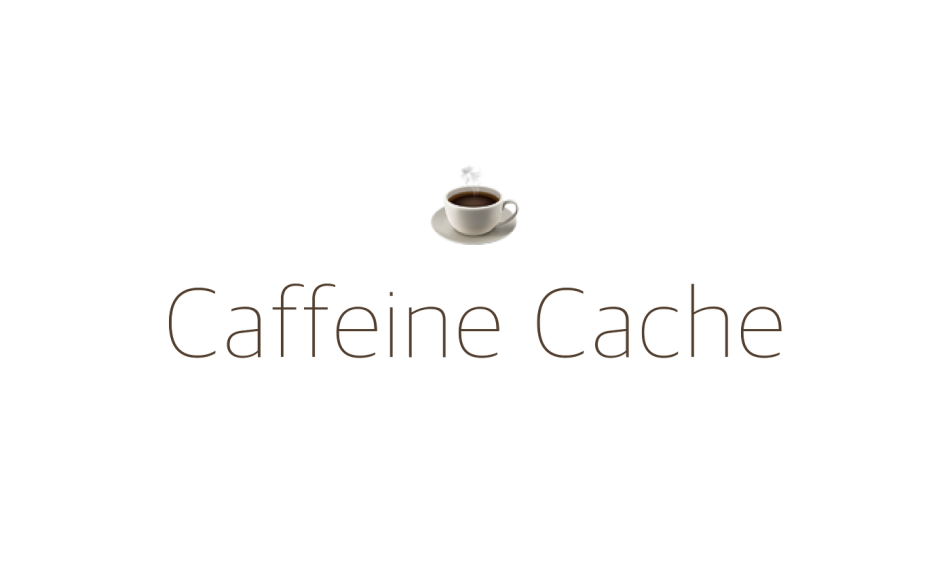
1. What is Caffeine?
Caffeine is a Java library for caching, designed to be both high-performing and easy to use. It’s similar to Google Guava but with notable improvements in speed and flexibility.
1.1 Features of Caffeine
- Automatic Loading: It loads data in the cache automatically.
- Flexible Expiration Policies: Supports time-based expiration, size-based eviction, and more.
- High Performance: Designed for speed and optimized for high read/write throughput.
1.2 Why Use Caffeine?
Caffeine is not only efficient but also incredibly customizable, providing many tools to fine-tune caching behavior based on application needs.
2. Steps to Implement Caffeine Caching in Java
Implementing Caffeine is straightforward with the right guidance. Follow these steps to get started.
2.1 Step 1: Add Caffeine Dependency
First, include the Caffeine dependency in your project. In a Maven project, you would add it like this:
<dependency>
<groupId>com.github.ben-manes.caffeine</groupId>
<artifactId>caffeine</artifactId>
<version>3.0.4</version>
</dependency>
This dependency will allow us to use Caffeine in our Java application.
2.2 Step 2: Create a Caffeine Cache Instance
Next, create a basic cache instance with common configuration settings:
import com.github.benmanes.caffeine.cache.Cache;
import com.github.benmanes.caffeine.cache.Caffeine;
Cache<String, Object> cache = Caffeine.newBuilder()
.expireAfterWrite(10, TimeUnit.MINUTES)
.maximumSize(100)
.build();
Here, we configure the cache to evict entries 10 minutes after creation, with a maximum of 100 entries.
2.3 Step 3: Cache Data
You can easily put and get data from the cache as follows:
// Putting data in the cache
cache.put("key1", "This is cached data");
// Retrieving data from the cache
String value = (String) cache.getIfPresent("key1");
System.out.println(value); // Output: This is cached data
2.4 Step 4: Using get with a Loading Function
Use the get method with a loading function to retrieve or compute the value if it’s not already cached:
String value = cache.get("key2", key -> loadFromDatabase(key));
System.out.println(value);
The loadFromDatabase method will only be called if "key2" is not present in the cache.
3. Best Practices for Caffeine Caching
To maximize the effectiveness of Caffeine in your application, keep the following best practices in mind:
3.1 Monitor Cache Usage
Use Caffeine’s built-in statistics to monitor cache usage:
CacheStats stats = cache.stats();
System.out.println("Hit Rate: " + stats.hitRate());
3.2 Choose Appropriate Expiration Policies
Selecting an expiration policy depends on how data is accessed. For example:
- Time-Based Expiration: Use when data validity is time-sensitive.
- Size-Based Eviction: Set a maximum size when memory usage is a concern.
3.3 Use Async Caching for Non-Critical Loads
Use asynchronous caching for non-blocking operations, which can improve overall system responsiveness:
AsyncCache<String, Object> asyncCache = Caffeine.newBuilder()
.expireAfterWrite(10, TimeUnit.MINUTES)
.maximumSize(100)
.buildAsync();
4. Conclusion
Caching is essential for creating high-performance applications, and Caffeine offers a robust solution for Java developers. From its flexible configuration options to its high-speed operations, Caffeine is a tool that can transform your application’s efficiency.
Got questions? Leave a comment below, and I'll be happy to help!
Read more at : Caching in Java Using Caffeine: A Comprehensive Guide.
0
Subscribe to my newsletter
Read articles from Tuanhdotnet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
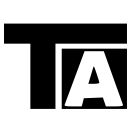
Tuanhdotnet
Tuanhdotnet
I am Tuanh.net. As of 2024, I have accumulated 8 years of experience in backend programming. I am delighted to connect and share my knowledge with everyone.